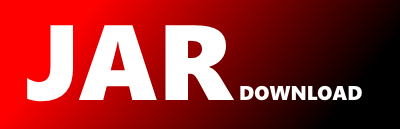
com.pulumi.gcp.accesscontextmanager.kotlin.ServicePerimeterDryRunIngressPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.accesscontextmanager.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.accesscontextmanager.ServicePerimeterDryRunIngressPolicyArgs.builder
import com.pulumi.gcp.accesscontextmanager.kotlin.inputs.ServicePerimeterDryRunIngressPolicyIngressFromArgs
import com.pulumi.gcp.accesscontextmanager.kotlin.inputs.ServicePerimeterDryRunIngressPolicyIngressFromArgsBuilder
import com.pulumi.gcp.accesscontextmanager.kotlin.inputs.ServicePerimeterDryRunIngressPolicyIngressToArgs
import com.pulumi.gcp.accesscontextmanager.kotlin.inputs.ServicePerimeterDryRunIngressPolicyIngressToArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manage a single IngressPolicy in the spec (dry-run) configuration for a service perimeter.
* IngressPolicies match requests based on ingressFrom and ingressTo stanzas. For an ingress policy to match,
* both the ingressFrom and ingressTo stanzas must be matched. If an IngressPolicy matches a request,
* the request is allowed through the perimeter boundary from outside the perimeter.
* For example, access from the internet can be allowed either based on an AccessLevel or,
* for traffic hosted on Google Cloud, the project of the source network.
* For access from private networks, using the project of the hosting network is required.
* Individual ingress policies can be limited by restricting which services and/
* or actions they match using the ingressTo field.
* > **Note:** By default, updates to this resource will remove the IngressPolicy from the
* from the perimeter and add it back in a non-atomic manner. To ensure that the new IngressPolicy
* is added before the old one is removed, add a `lifecycle` block with `create_before_destroy = true` to this resource.
* To get more information about ServicePerimeterDryRunIngressPolicy, see:
* * [API documentation](https://cloud.google.com/access-context-manager/docs/reference/rest/v1/accessPolicies.servicePerimeters#ingresspolicy)
* ## Example Usage
* @property ingressFrom Defines the conditions on the source of a request causing this `IngressPolicy`
* to apply.
* Structure is documented below.
* @property ingressTo Defines the conditions on the `ApiOperation` and request destination that cause
* this `IngressPolicy` to apply.
* Structure is documented below.
* @property perimeter The name of the Service Perimeter to add this resource to.
* - - -
*/
public data class ServicePerimeterDryRunIngressPolicyArgs(
public val ingressFrom: Output? = null,
public val ingressTo: Output? = null,
public val perimeter: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.accesscontextmanager.ServicePerimeterDryRunIngressPolicyArgs = com.pulumi.gcp.accesscontextmanager.ServicePerimeterDryRunIngressPolicyArgs.builder()
.ingressFrom(ingressFrom?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ingressTo(ingressTo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.perimeter(perimeter?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServicePerimeterDryRunIngressPolicyArgs].
*/
@PulumiTagMarker
public class ServicePerimeterDryRunIngressPolicyArgsBuilder internal constructor() {
private var ingressFrom: Output? = null
private var ingressTo: Output? = null
private var perimeter: Output? = null
/**
* @param value Defines the conditions on the source of a request causing this `IngressPolicy`
* to apply.
* Structure is documented below.
*/
@JvmName("parosdftvxchjlea")
public suspend fun ingressFrom(`value`: Output) {
this.ingressFrom = value
}
/**
* @param value Defines the conditions on the `ApiOperation` and request destination that cause
* this `IngressPolicy` to apply.
* Structure is documented below.
*/
@JvmName("nkkqhrvnqruughcb")
public suspend fun ingressTo(`value`: Output) {
this.ingressTo = value
}
/**
* @param value The name of the Service Perimeter to add this resource to.
* - - -
*/
@JvmName("swpiubtwllwtdlhn")
public suspend fun perimeter(`value`: Output) {
this.perimeter = value
}
/**
* @param value Defines the conditions on the source of a request causing this `IngressPolicy`
* to apply.
* Structure is documented below.
*/
@JvmName("mkpqnxxqugrnenqf")
public suspend fun ingressFrom(`value`: ServicePerimeterDryRunIngressPolicyIngressFromArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ingressFrom = mapped
}
/**
* @param argument Defines the conditions on the source of a request causing this `IngressPolicy`
* to apply.
* Structure is documented below.
*/
@JvmName("jealypyjdvcghiih")
public suspend fun ingressFrom(argument: suspend ServicePerimeterDryRunIngressPolicyIngressFromArgsBuilder.() -> Unit) {
val toBeMapped = ServicePerimeterDryRunIngressPolicyIngressFromArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ingressFrom = mapped
}
/**
* @param value Defines the conditions on the `ApiOperation` and request destination that cause
* this `IngressPolicy` to apply.
* Structure is documented below.
*/
@JvmName("mcfiqfgrbipdjink")
public suspend fun ingressTo(`value`: ServicePerimeterDryRunIngressPolicyIngressToArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ingressTo = mapped
}
/**
* @param argument Defines the conditions on the `ApiOperation` and request destination that cause
* this `IngressPolicy` to apply.
* Structure is documented below.
*/
@JvmName("bosupbnbymryklmh")
public suspend fun ingressTo(argument: suspend ServicePerimeterDryRunIngressPolicyIngressToArgsBuilder.() -> Unit) {
val toBeMapped = ServicePerimeterDryRunIngressPolicyIngressToArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ingressTo = mapped
}
/**
* @param value The name of the Service Perimeter to add this resource to.
* - - -
*/
@JvmName("pbvpyrgmyrurxxsw")
public suspend fun perimeter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.perimeter = mapped
}
internal fun build(): ServicePerimeterDryRunIngressPolicyArgs =
ServicePerimeterDryRunIngressPolicyArgs(
ingressFrom = ingressFrom,
ingressTo = ingressTo,
perimeter = perimeter,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy