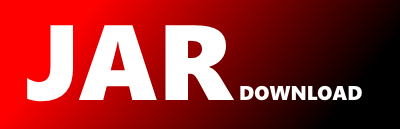
com.pulumi.gcp.alloydb.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.alloydb.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterAutomatedBackupPolicy
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterBackupSource
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterContinuousBackupConfig
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterContinuousBackupInfo
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterEncryptionConfig
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterEncryptionInfo
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterInitialUser
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterMaintenanceUpdatePolicy
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterMigrationSource
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterNetworkConfig
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterPscConfig
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterRestoreBackupSource
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterRestoreContinuousBackupSource
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterSecondaryConfig
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterAutomatedBackupPolicy.Companion.toKotlin as clusterAutomatedBackupPolicyToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterBackupSource.Companion.toKotlin as clusterBackupSourceToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterContinuousBackupConfig.Companion.toKotlin as clusterContinuousBackupConfigToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterContinuousBackupInfo.Companion.toKotlin as clusterContinuousBackupInfoToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterEncryptionConfig.Companion.toKotlin as clusterEncryptionConfigToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterEncryptionInfo.Companion.toKotlin as clusterEncryptionInfoToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterInitialUser.Companion.toKotlin as clusterInitialUserToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterMaintenanceUpdatePolicy.Companion.toKotlin as clusterMaintenanceUpdatePolicyToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterMigrationSource.Companion.toKotlin as clusterMigrationSourceToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterNetworkConfig.Companion.toKotlin as clusterNetworkConfigToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterPscConfig.Companion.toKotlin as clusterPscConfigToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterRestoreBackupSource.Companion.toKotlin as clusterRestoreBackupSourceToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterRestoreContinuousBackupSource.Companion.toKotlin as clusterRestoreContinuousBackupSourceToKotlin
import com.pulumi.gcp.alloydb.kotlin.outputs.ClusterSecondaryConfig.Companion.toKotlin as clusterSecondaryConfigToKotlin
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource = com.pulumi.gcp.alloydb.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* ## Example Usage
* ### Alloydb Cluster Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultNetwork = new gcp.compute.Network("default", {name: "alloydb-cluster"});
* const _default = new gcp.alloydb.Cluster("default", {
* clusterId: "alloydb-cluster",
* location: "us-central1",
* networkConfig: {
* network: defaultNetwork.id,
* },
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_network = gcp.compute.Network("default", name="alloydb-cluster")
* default = gcp.alloydb.Cluster("default",
* cluster_id="alloydb-cluster",
* location="us-central1",
* network_config={
* "network": default_network.id,
* })
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultNetwork = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-cluster",
* });
* var @default = new Gcp.Alloydb.Cluster("default", new()
* {
* ClusterId = "alloydb-cluster",
* Location = "us-central1",
* NetworkConfig = new Gcp.Alloydb.Inputs.ClusterNetworkConfigArgs
* {
* Network = defaultNetwork.Id,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultNetwork, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-cluster"),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "default", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-cluster"),
* Location: pulumi.String("us-central1"),
* NetworkConfig: &alloydb.ClusterNetworkConfigArgs{
* Network: defaultNetwork.ID(),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterNetworkConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultNetwork = new Network("defaultNetwork", NetworkArgs.builder()
* .name("alloydb-cluster")
* .build());
* var default_ = new Cluster("default", ClusterArgs.builder()
* .clusterId("alloydb-cluster")
* .location("us-central1")
* .networkConfig(ClusterNetworkConfigArgs.builder()
* .network(defaultNetwork.id())
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-cluster
* location: us-central1
* networkConfig:
* network: ${defaultNetwork.id}
* defaultNetwork:
* type: gcp:compute:Network
* name: default
* properties:
* name: alloydb-cluster
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Alloydb Cluster Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "alloydb-cluster-full"});
* const full = new gcp.alloydb.Cluster("full", {
* clusterId: "alloydb-cluster-full",
* location: "us-central1",
* networkConfig: {
* network: _default.id,
* },
* databaseVersion: "POSTGRES_15",
* initialUser: {
* user: "alloydb-cluster-full",
* password: "alloydb-cluster-full",
* },
* continuousBackupConfig: {
* enabled: true,
* recoveryWindowDays: 14,
* },
* automatedBackupPolicy: {
* location: "us-central1",
* backupWindow: "1800s",
* enabled: true,
* weeklySchedule: {
* daysOfWeeks: ["MONDAY"],
* startTimes: [{
* hours: 23,
* minutes: 0,
* seconds: 0,
* nanos: 0,
* }],
* },
* quantityBasedRetention: {
* count: 1,
* },
* labels: {
* test: "alloydb-cluster-full",
* },
* },
* labels: {
* test: "alloydb-cluster-full",
* },
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="alloydb-cluster-full")
* full = gcp.alloydb.Cluster("full",
* cluster_id="alloydb-cluster-full",
* location="us-central1",
* network_config={
* "network": default.id,
* },
* database_version="POSTGRES_15",
* initial_user={
* "user": "alloydb-cluster-full",
* "password": "alloydb-cluster-full",
* },
* continuous_backup_config={
* "enabled": True,
* "recovery_window_days": 14,
* },
* automated_backup_policy={
* "location": "us-central1",
* "backup_window": "1800s",
* "enabled": True,
* "weekly_schedule": {
* "days_of_weeks": ["MONDAY"],
* "start_times": [{
* "hours": 23,
* "minutes": 0,
* "seconds": 0,
* "nanos": 0,
* }],
* },
* "quantity_based_retention": {
* "count": 1,
* },
* "labels": {
* "test": "alloydb-cluster-full",
* },
* },
* labels={
* "test": "alloydb-cluster-full",
* })
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-cluster-full",
* });
* var full = new Gcp.Alloydb.Cluster("full", new()
* {
* ClusterId = "alloydb-cluster-full",
* Location = "us-central1",
* NetworkConfig = new Gcp.Alloydb.Inputs.ClusterNetworkConfigArgs
* {
* Network = @default.Id,
* },
* DatabaseVersion = "POSTGRES_15",
* InitialUser = new Gcp.Alloydb.Inputs.ClusterInitialUserArgs
* {
* User = "alloydb-cluster-full",
* Password = "alloydb-cluster-full",
* },
* ContinuousBackupConfig = new Gcp.Alloydb.Inputs.ClusterContinuousBackupConfigArgs
* {
* Enabled = true,
* RecoveryWindowDays = 14,
* },
* AutomatedBackupPolicy = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyArgs
* {
* Location = "us-central1",
* BackupWindow = "1800s",
* Enabled = true,
* WeeklySchedule = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyWeeklyScheduleArgs
* {
* DaysOfWeeks = new[]
* {
* "MONDAY",
* },
* StartTimes = new[]
* {
* new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs
* {
* Hours = 23,
* Minutes = 0,
* Seconds = 0,
* Nanos = 0,
* },
* },
* },
* QuantityBasedRetention = new Gcp.Alloydb.Inputs.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs
* {
* Count = 1,
* },
* Labels =
* {
* { "test", "alloydb-cluster-full" },
* },
* },
* Labels =
* {
* { "test", "alloydb-cluster-full" },
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-cluster-full"),
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "full", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-cluster-full"),
* Location: pulumi.String("us-central1"),
* NetworkConfig: &alloydb.ClusterNetworkConfigArgs{
* Network: _default.ID(),
* },
* DatabaseVersion: pulumi.String("POSTGRES_15"),
* InitialUser: &alloydb.ClusterInitialUserArgs{
* User: pulumi.String("alloydb-cluster-full"),
* Password: pulumi.String("alloydb-cluster-full"),
* },
* ContinuousBackupConfig: &alloydb.ClusterContinuousBackupConfigArgs{
* Enabled: pulumi.Bool(true),
* RecoveryWindowDays: pulumi.Int(14),
* },
* AutomatedBackupPolicy: &alloydb.ClusterAutomatedBackupPolicyArgs{
* Location: pulumi.String("us-central1"),
* BackupWindow: pulumi.String("1800s"),
* Enabled: pulumi.Bool(true),
* WeeklySchedule: &alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleArgs{
* DaysOfWeeks: pulumi.StringArray{
* pulumi.String("MONDAY"),
* },
* StartTimes: alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArray{
* &alloydb.ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs{
* Hours: pulumi.Int(23),
* Minutes: pulumi.Int(0),
* Seconds: pulumi.Int(0),
* Nanos: pulumi.Int(0),
* },
* },
* },
* QuantityBasedRetention: &alloydb.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs{
* Count: pulumi.Int(1),
* },
* Labels: pulumi.StringMap{
* "test": pulumi.String("alloydb-cluster-full"),
* },
* },
* Labels: pulumi.StringMap{
* "test": pulumi.String("alloydb-cluster-full"),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterNetworkConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterInitialUserArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyWeeklyScheduleArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("alloydb-cluster-full")
* .build());
* var full = new Cluster("full", ClusterArgs.builder()
* .clusterId("alloydb-cluster-full")
* .location("us-central1")
* .networkConfig(ClusterNetworkConfigArgs.builder()
* .network(default_.id())
* .build())
* .databaseVersion("POSTGRES_15")
* .initialUser(ClusterInitialUserArgs.builder()
* .user("alloydb-cluster-full")
* .password("alloydb-cluster-full")
* .build())
* .continuousBackupConfig(ClusterContinuousBackupConfigArgs.builder()
* .enabled(true)
* .recoveryWindowDays(14)
* .build())
* .automatedBackupPolicy(ClusterAutomatedBackupPolicyArgs.builder()
* .location("us-central1")
* .backupWindow("1800s")
* .enabled(true)
* .weeklySchedule(ClusterAutomatedBackupPolicyWeeklyScheduleArgs.builder()
* .daysOfWeeks("MONDAY")
* .startTimes(ClusterAutomatedBackupPolicyWeeklyScheduleStartTimeArgs.builder()
* .hours(23)
* .minutes(0)
* .seconds(0)
* .nanos(0)
* .build())
* .build())
* .quantityBasedRetention(ClusterAutomatedBackupPolicyQuantityBasedRetentionArgs.builder()
* .count(1)
* .build())
* .labels(Map.of("test", "alloydb-cluster-full"))
* .build())
* .labels(Map.of("test", "alloydb-cluster-full"))
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* full:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-cluster-full
* location: us-central1
* networkConfig:
* network: ${default.id}
* databaseVersion: POSTGRES_15
* initialUser:
* user: alloydb-cluster-full
* password: alloydb-cluster-full
* continuousBackupConfig:
* enabled: true
* recoveryWindowDays: 14
* automatedBackupPolicy:
* location: us-central1
* backupWindow: 1800s
* enabled: true
* weeklySchedule:
* daysOfWeeks:
* - MONDAY
* startTimes:
* - hours: 23
* minutes: 0
* seconds: 0
* nanos: 0
* quantityBasedRetention:
* count: 1
* labels:
* test: alloydb-cluster-full
* labels:
* test: alloydb-cluster-full
* default:
* type: gcp:compute:Network
* properties:
* name: alloydb-cluster-full
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ### Alloydb Cluster Restore
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const default = gcp.compute.getNetwork({
* name: "alloydb-network",
* });
* const source = new gcp.alloydb.Cluster("source", {
* clusterId: "alloydb-source-cluster",
* location: "us-central1",
* network: _default.then(_default => _default.id),
* initialUser: {
* password: "alloydb-source-cluster",
* },
* });
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-source-cluster",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.then(_default => _default.id),
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.then(_default => _default.id),
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* const sourceInstance = new gcp.alloydb.Instance("source", {
* cluster: source.name,
* instanceId: "alloydb-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* }, {
* dependsOn: [vpcConnection],
* });
* const sourceBackup = new gcp.alloydb.Backup("source", {
* backupId: "alloydb-backup",
* location: "us-central1",
* clusterName: source.name,
* }, {
* dependsOn: [sourceInstance],
* });
* const restoredFromBackup = new gcp.alloydb.Cluster("restored_from_backup", {
* clusterId: "alloydb-backup-restored",
* location: "us-central1",
* networkConfig: {
* network: _default.then(_default => _default.id),
* },
* restoreBackupSource: {
* backupName: sourceBackup.name,
* },
* });
* const restoredViaPitr = new gcp.alloydb.Cluster("restored_via_pitr", {
* clusterId: "alloydb-pitr-restored",
* location: "us-central1",
* network: _default.then(_default => _default.id),
* restoreContinuousBackupSource: {
* cluster: source.name,
* pointInTime: "2023-08-03T19:19:00.094Z",
* },
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.get_network(name="alloydb-network")
* source = gcp.alloydb.Cluster("source",
* cluster_id="alloydb-source-cluster",
* location="us-central1",
* network=default.id,
* initial_user={
* "password": "alloydb-source-cluster",
* })
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-source-cluster",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* source_instance = gcp.alloydb.Instance("source",
* cluster=source.name,
* instance_id="alloydb-instance",
* instance_type="PRIMARY",
* machine_config={
* "cpu_count": 2,
* },
* opts = pulumi.ResourceOptions(depends_on=[vpc_connection]))
* source_backup = gcp.alloydb.Backup("source",
* backup_id="alloydb-backup",
* location="us-central1",
* cluster_name=source.name,
* opts = pulumi.ResourceOptions(depends_on=[source_instance]))
* restored_from_backup = gcp.alloydb.Cluster("restored_from_backup",
* cluster_id="alloydb-backup-restored",
* location="us-central1",
* network_config={
* "network": default.id,
* },
* restore_backup_source={
* "backup_name": source_backup.name,
* })
* restored_via_pitr = gcp.alloydb.Cluster("restored_via_pitr",
* cluster_id="alloydb-pitr-restored",
* location="us-central1",
* network=default.id,
* restore_continuous_backup_source={
* "cluster": source.name,
* "point_in_time": "2023-08-03T19:19:00.094Z",
* })
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Compute.GetNetwork.Invoke(new()
* {
* Name = "alloydb-network",
* });
* var source = new Gcp.Alloydb.Cluster("source", new()
* {
* ClusterId = "alloydb-source-cluster",
* Location = "us-central1",
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* InitialUser = new Gcp.Alloydb.Inputs.ClusterInitialUserArgs
* {
* Password = "alloydb-source-cluster",
* },
* });
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-source-cluster",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* var sourceInstance = new Gcp.Alloydb.Instance("source", new()
* {
* Cluster = source.Name,
* InstanceId = "alloydb-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* vpcConnection,
* },
* });
* var sourceBackup = new Gcp.Alloydb.Backup("source", new()
* {
* BackupId = "alloydb-backup",
* Location = "us-central1",
* ClusterName = source.Name,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* sourceInstance,
* },
* });
* var restoredFromBackup = new Gcp.Alloydb.Cluster("restored_from_backup", new()
* {
* ClusterId = "alloydb-backup-restored",
* Location = "us-central1",
* NetworkConfig = new Gcp.Alloydb.Inputs.ClusterNetworkConfigArgs
* {
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* },
* RestoreBackupSource = new Gcp.Alloydb.Inputs.ClusterRestoreBackupSourceArgs
* {
* BackupName = sourceBackup.Name,
* },
* });
* var restoredViaPitr = new Gcp.Alloydb.Cluster("restored_via_pitr", new()
* {
* ClusterId = "alloydb-pitr-restored",
* Location = "us-central1",
* Network = @default.Apply(@default => @default.Apply(getNetworkResult => getNetworkResult.Id)),
* RestoreContinuousBackupSource = new Gcp.Alloydb.Inputs.ClusterRestoreContinuousBackupSourceArgs
* {
* Cluster = source.Name,
* PointInTime = "2023-08-03T19:19:00.094Z",
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _default, err := compute.LookupNetwork(ctx, &compute.LookupNetworkArgs{
* Name: "alloydb-network",
* }, nil)
* if err != nil {
* return err
* }
* source, err := alloydb.NewCluster(ctx, "source", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-source-cluster"),
* Location: pulumi.String("us-central1"),
* Network: pulumi.String(_default.Id),
* InitialUser: &alloydb.ClusterInitialUserArgs{
* Password: pulumi.String("alloydb-source-cluster"),
* },
* })
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-source-cluster"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: pulumi.String(_default.Id),
* })
* if err != nil {
* return err
* }
* vpcConnection, err := servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: pulumi.String(_default.Id),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* sourceInstance, err := alloydb.NewInstance(ctx, "source", &alloydb.InstanceArgs{
* Cluster: source.Name,
* InstanceId: pulumi.String("alloydb-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* vpcConnection,
* }))
* if err != nil {
* return err
* }
* sourceBackup, err := alloydb.NewBackup(ctx, "source", &alloydb.BackupArgs{
* BackupId: pulumi.String("alloydb-backup"),
* Location: pulumi.String("us-central1"),
* ClusterName: source.Name,
* }, pulumi.DependsOn([]pulumi.Resource{
* sourceInstance,
* }))
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "restored_from_backup", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-backup-restored"),
* Location: pulumi.String("us-central1"),
* NetworkConfig: &alloydb.ClusterNetworkConfigArgs{
* Network: pulumi.String(_default.Id),
* },
* RestoreBackupSource: &alloydb.ClusterRestoreBackupSourceArgs{
* BackupName: sourceBackup.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "restored_via_pitr", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-pitr-restored"),
* Location: pulumi.String("us-central1"),
* Network: pulumi.String(_default.Id),
* RestoreContinuousBackupSource: &alloydb.ClusterRestoreContinuousBackupSourceArgs{
* Cluster: source.Name,
* PointInTime: pulumi.String("2023-08-03T19:19:00.094Z"),
* },
* })
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetNetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterInitialUserArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.alloydb.Backup;
* import com.pulumi.gcp.alloydb.BackupArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterNetworkConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterRestoreBackupSourceArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterRestoreContinuousBackupSourceArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = ComputeFunctions.getNetwork(GetNetworkArgs.builder()
* .name("alloydb-network")
* .build());
* var source = new Cluster("source", ClusterArgs.builder()
* .clusterId("alloydb-source-cluster")
* .location("us-central1")
* .network(default_.id())
* .initialUser(ClusterInitialUserArgs.builder()
* .password("alloydb-source-cluster")
* .build())
* .build());
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-source-cluster")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* var sourceInstance = new Instance("sourceInstance", InstanceArgs.builder()
* .cluster(source.name())
* .instanceId("alloydb-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(vpcConnection)
* .build());
* var sourceBackup = new Backup("sourceBackup", BackupArgs.builder()
* .backupId("alloydb-backup")
* .location("us-central1")
* .clusterName(source.name())
* .build(), CustomResourceOptions.builder()
* .dependsOn(sourceInstance)
* .build());
* var restoredFromBackup = new Cluster("restoredFromBackup", ClusterArgs.builder()
* .clusterId("alloydb-backup-restored")
* .location("us-central1")
* .networkConfig(ClusterNetworkConfigArgs.builder()
* .network(default_.id())
* .build())
* .restoreBackupSource(ClusterRestoreBackupSourceArgs.builder()
* .backupName(sourceBackup.name())
* .build())
* .build());
* var restoredViaPitr = new Cluster("restoredViaPitr", ClusterArgs.builder()
* .clusterId("alloydb-pitr-restored")
* .location("us-central1")
* .network(default_.id())
* .restoreContinuousBackupSource(ClusterRestoreContinuousBackupSourceArgs.builder()
* .cluster(source.name())
* .pointInTime("2023-08-03T19:19:00.094Z")
* .build())
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* source:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-source-cluster
* location: us-central1
* network: ${default.id}
* initialUser:
* password: alloydb-source-cluster
* sourceInstance:
* type: gcp:alloydb:Instance
* name: source
* properties:
* cluster: ${source.name}
* instanceId: alloydb-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* options:
* dependson:
* - ${vpcConnection}
* sourceBackup:
* type: gcp:alloydb:Backup
* name: source
* properties:
* backupId: alloydb-backup
* location: us-central1
* clusterName: ${source.name}
* options:
* dependson:
* - ${sourceInstance}
* restoredFromBackup:
* type: gcp:alloydb:Cluster
* name: restored_from_backup
* properties:
* clusterId: alloydb-backup-restored
* location: us-central1
* networkConfig:
* network: ${default.id}
* restoreBackupSource:
* backupName: ${sourceBackup.name}
* restoredViaPitr:
* type: gcp:alloydb:Cluster
* name: restored_via_pitr
* properties:
* clusterId: alloydb-pitr-restored
* location: us-central1
* network: ${default.id}
* restoreContinuousBackupSource:
* cluster: ${source.name}
* pointInTime: 2023-08-03T19:19:00.094Z
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-source-cluster
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* default:
* fn::invoke:
* Function: gcp:compute:getNetwork
* Arguments:
* name: alloydb-network
* ```
*
* ### Alloydb Secondary Cluster Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "alloydb-secondary-cluster"});
* const primary = new gcp.alloydb.Cluster("primary", {
* clusterId: "alloydb-primary-cluster",
* location: "us-central1",
* network: _default.id,
* });
* const privateIpAlloc = new gcp.compute.GlobalAddress("private_ip_alloc", {
* name: "alloydb-secondary-cluster",
* addressType: "INTERNAL",
* purpose: "VPC_PEERING",
* prefixLength: 16,
* network: _default.id,
* });
* const vpcConnection = new gcp.servicenetworking.Connection("vpc_connection", {
* network: _default.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [privateIpAlloc.name],
* });
* const primaryInstance = new gcp.alloydb.Instance("primary", {
* cluster: primary.name,
* instanceId: "alloydb-primary-instance",
* instanceType: "PRIMARY",
* machineConfig: {
* cpuCount: 2,
* },
* }, {
* dependsOn: [vpcConnection],
* });
* const secondary = new gcp.alloydb.Cluster("secondary", {
* clusterId: "alloydb-secondary-cluster",
* location: "us-east1",
* network: _default.id,
* clusterType: "SECONDARY",
* continuousBackupConfig: {
* enabled: false,
* },
* secondaryConfig: {
* primaryClusterName: primary.name,
* },
* }, {
* dependsOn: [primaryInstance],
* });
* const project = gcp.organizations.getProject({});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="alloydb-secondary-cluster")
* primary = gcp.alloydb.Cluster("primary",
* cluster_id="alloydb-primary-cluster",
* location="us-central1",
* network=default.id)
* private_ip_alloc = gcp.compute.GlobalAddress("private_ip_alloc",
* name="alloydb-secondary-cluster",
* address_type="INTERNAL",
* purpose="VPC_PEERING",
* prefix_length=16,
* network=default.id)
* vpc_connection = gcp.servicenetworking.Connection("vpc_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[private_ip_alloc.name])
* primary_instance = gcp.alloydb.Instance("primary",
* cluster=primary.name,
* instance_id="alloydb-primary-instance",
* instance_type="PRIMARY",
* machine_config={
* "cpu_count": 2,
* },
* opts = pulumi.ResourceOptions(depends_on=[vpc_connection]))
* secondary = gcp.alloydb.Cluster("secondary",
* cluster_id="alloydb-secondary-cluster",
* location="us-east1",
* network=default.id,
* cluster_type="SECONDARY",
* continuous_backup_config={
* "enabled": False,
* },
* secondary_config={
* "primary_cluster_name": primary.name,
* },
* opts = pulumi.ResourceOptions(depends_on=[primary_instance]))
* project = gcp.organizations.get_project()
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "alloydb-secondary-cluster",
* });
* var primary = new Gcp.Alloydb.Cluster("primary", new()
* {
* ClusterId = "alloydb-primary-cluster",
* Location = "us-central1",
* Network = @default.Id,
* });
* var privateIpAlloc = new Gcp.Compute.GlobalAddress("private_ip_alloc", new()
* {
* Name = "alloydb-secondary-cluster",
* AddressType = "INTERNAL",
* Purpose = "VPC_PEERING",
* PrefixLength = 16,
* Network = @default.Id,
* });
* var vpcConnection = new Gcp.ServiceNetworking.Connection("vpc_connection", new()
* {
* Network = @default.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* privateIpAlloc.Name,
* },
* });
* var primaryInstance = new Gcp.Alloydb.Instance("primary", new()
* {
* Cluster = primary.Name,
* InstanceId = "alloydb-primary-instance",
* InstanceType = "PRIMARY",
* MachineConfig = new Gcp.Alloydb.Inputs.InstanceMachineConfigArgs
* {
* CpuCount = 2,
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* vpcConnection,
* },
* });
* var secondary = new Gcp.Alloydb.Cluster("secondary", new()
* {
* ClusterId = "alloydb-secondary-cluster",
* Location = "us-east1",
* Network = @default.Id,
* ClusterType = "SECONDARY",
* ContinuousBackupConfig = new Gcp.Alloydb.Inputs.ClusterContinuousBackupConfigArgs
* {
* Enabled = false,
* },
* SecondaryConfig = new Gcp.Alloydb.Inputs.ClusterSecondaryConfigArgs
* {
* PrimaryClusterName = primary.Name,
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* primaryInstance,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/alloydb"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("alloydb-secondary-cluster"),
* })
* if err != nil {
* return err
* }
* primary, err := alloydb.NewCluster(ctx, "primary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-primary-cluster"),
* Location: pulumi.String("us-central1"),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* privateIpAlloc, err := compute.NewGlobalAddress(ctx, "private_ip_alloc", &compute.GlobalAddressArgs{
* Name: pulumi.String("alloydb-secondary-cluster"),
* AddressType: pulumi.String("INTERNAL"),
* Purpose: pulumi.String("VPC_PEERING"),
* PrefixLength: pulumi.Int(16),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* vpcConnection, err := servicenetworking.NewConnection(ctx, "vpc_connection", &servicenetworking.ConnectionArgs{
* Network: _default.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* privateIpAlloc.Name,
* },
* })
* if err != nil {
* return err
* }
* primaryInstance, err := alloydb.NewInstance(ctx, "primary", &alloydb.InstanceArgs{
* Cluster: primary.Name,
* InstanceId: pulumi.String("alloydb-primary-instance"),
* InstanceType: pulumi.String("PRIMARY"),
* MachineConfig: &alloydb.InstanceMachineConfigArgs{
* CpuCount: pulumi.Int(2),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* vpcConnection,
* }))
* if err != nil {
* return err
* }
* _, err = alloydb.NewCluster(ctx, "secondary", &alloydb.ClusterArgs{
* ClusterId: pulumi.String("alloydb-secondary-cluster"),
* Location: pulumi.String("us-east1"),
* Network: _default.ID(),
* ClusterType: pulumi.String("SECONDARY"),
* ContinuousBackupConfig: &alloydb.ClusterContinuousBackupConfigArgs{
* Enabled: pulumi.Bool(false),
* },
* SecondaryConfig: &alloydb.ClusterSecondaryConfigArgs{
* PrimaryClusterName: primary.Name,
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* primaryInstance,
* }))
* if err != nil {
* return err
* }
* _, err = organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.alloydb.Cluster;
* import com.pulumi.gcp.alloydb.ClusterArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.alloydb.Instance;
* import com.pulumi.gcp.alloydb.InstanceArgs;
* import com.pulumi.gcp.alloydb.inputs.InstanceMachineConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterContinuousBackupConfigArgs;
* import com.pulumi.gcp.alloydb.inputs.ClusterSecondaryConfigArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("alloydb-secondary-cluster")
* .build());
* var primary = new Cluster("primary", ClusterArgs.builder()
* .clusterId("alloydb-primary-cluster")
* .location("us-central1")
* .network(default_.id())
* .build());
* var privateIpAlloc = new GlobalAddress("privateIpAlloc", GlobalAddressArgs.builder()
* .name("alloydb-secondary-cluster")
* .addressType("INTERNAL")
* .purpose("VPC_PEERING")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var vpcConnection = new Connection("vpcConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(privateIpAlloc.name())
* .build());
* var primaryInstance = new Instance("primaryInstance", InstanceArgs.builder()
* .cluster(primary.name())
* .instanceId("alloydb-primary-instance")
* .instanceType("PRIMARY")
* .machineConfig(InstanceMachineConfigArgs.builder()
* .cpuCount(2)
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(vpcConnection)
* .build());
* var secondary = new Cluster("secondary", ClusterArgs.builder()
* .clusterId("alloydb-secondary-cluster")
* .location("us-east1")
* .network(default_.id())
* .clusterType("SECONDARY")
* .continuousBackupConfig(ClusterContinuousBackupConfigArgs.builder()
* .enabled(false)
* .build())
* .secondaryConfig(ClusterSecondaryConfigArgs.builder()
* .primaryClusterName(primary.name())
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(primaryInstance)
* .build());
* final var project = OrganizationsFunctions.getProject();
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-primary-cluster
* location: us-central1
* network: ${default.id}
* primaryInstance:
* type: gcp:alloydb:Instance
* name: primary
* properties:
* cluster: ${primary.name}
* instanceId: alloydb-primary-instance
* instanceType: PRIMARY
* machineConfig:
* cpuCount: 2
* options:
* dependson:
* - ${vpcConnection}
* secondary:
* type: gcp:alloydb:Cluster
* properties:
* clusterId: alloydb-secondary-cluster
* location: us-east1
* network: ${default.id}
* clusterType: SECONDARY
* continuousBackupConfig:
* enabled: false
* secondaryConfig:
* primaryClusterName: ${primary.name}
* options:
* dependson:
* - ${primaryInstance}
* default:
* type: gcp:compute:Network
* properties:
* name: alloydb-secondary-cluster
* privateIpAlloc:
* type: gcp:compute:GlobalAddress
* name: private_ip_alloc
* properties:
* name: alloydb-secondary-cluster
* addressType: INTERNAL
* purpose: VPC_PEERING
* prefixLength: 16
* network: ${default.id}
* vpcConnection:
* type: gcp:servicenetworking:Connection
* name: vpc_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${privateIpAlloc.name}
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Cluster can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/clusters/{{cluster_id}}`
* * `{{project}}/{{location}}/{{cluster_id}}`
* * `{{location}}/{{cluster_id}}`
* * `{{cluster_id}}`
* When using the `pulumi import` command, Cluster can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default projects/{{project}}/locations/{{location}}/clusters/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{project}}/{{location}}/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{location}}/{{cluster_id}}
* ```
* ```sh
* $ pulumi import gcp:alloydb/cluster:Cluster default {{cluster_id}}
* ```
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.gcp.alloydb.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* Annotations to allow client tools to store small amount of arbitrary data. This is distinct from labels. https://google.aip.dev/128
* An object containing a list of "key": value pairs. Example: { "name": "wrench", "mass": "1.3kg", "count": "3" }.
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
*/
public val annotations: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy