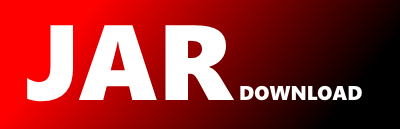
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuild.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudbuild.kotlin.outputs
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property artifacts Artifacts produced by the build that should be uploaded upon successful completion of all build steps.
* Structure is documented below.
* @property availableSecrets Secrets and secret environment variables.
* Structure is documented below.
* @property images A list of images to be pushed upon the successful completion of all build steps.
* The images are pushed using the builder service account's credentials.
* The digests of the pushed images will be stored in the Build resource's results field.
* If any of the images fail to be pushed, the build status is marked FAILURE.
* @property logsBucket Google Cloud Storage bucket where logs should be written.
* Logs file names will be of the format ${logsBucket}/log-${build_id}.txt.
* @property options Special options for this build.
* Structure is documented below.
* @property queueTtl TTL in queue for this build. If provided and the build is enqueued longer than this value,
* the build will expire and the build status will be EXPIRED.
* The TTL starts ticking from createTime.
* A duration in seconds with up to nine fractional digits, terminated by 's'. Example: "3.5s".
* @property secrets Secrets to decrypt using Cloud Key Management Service.
* Structure is documented below.
* @property source The location of the source files to build.
* One of `storageSource` or `repoSource` must be provided.
* Structure is documented below.
* @property steps The operations to be performed on the workspace.
* Structure is documented below.
* @property substitutions Substitutions data for Build resource.
* @property tags Tags for annotation of a Build. These are not docker tags.
* @property timeout Amount of time that this build should be allowed to run, to second granularity.
* If this amount of time elapses, work on the build will cease and the build status will be TIMEOUT.
* This timeout must be equal to or greater than the sum of the timeouts for build steps within the build.
* The expected format is the number of seconds followed by s.
* Default time is ten minutes (600s).
*/
public data class TriggerBuild(
public val artifacts: TriggerBuildArtifacts? = null,
public val availableSecrets: TriggerBuildAvailableSecrets? = null,
public val images: List? = null,
public val logsBucket: String? = null,
public val options: TriggerBuildOptions? = null,
public val queueTtl: String? = null,
public val secrets: List? = null,
public val source: TriggerBuildSource? = null,
public val steps: List,
public val substitutions: Map? = null,
public val tags: List? = null,
public val timeout: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudbuild.outputs.TriggerBuild): TriggerBuild =
TriggerBuild(
artifacts = javaType.artifacts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildArtifacts.Companion.toKotlin(args0)
})
}).orElse(null),
availableSecrets = javaType.availableSecrets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildAvailableSecrets.Companion.toKotlin(args0)
})
}).orElse(null),
images = javaType.images().map({ args0 -> args0 }),
logsBucket = javaType.logsBucket().map({ args0 -> args0 }).orElse(null),
options = javaType.options().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildOptions.Companion.toKotlin(args0)
})
}).orElse(null),
queueTtl = javaType.queueTtl().map({ args0 -> args0 }).orElse(null),
secrets = javaType.secrets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildSecret.Companion.toKotlin(args0)
})
}),
source = javaType.source().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildSource.Companion.toKotlin(args0)
})
}).orElse(null),
steps = javaType.steps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudbuild.kotlin.outputs.TriggerBuildStep.Companion.toKotlin(args0)
})
}),
substitutions = javaType.substitutions().map({ args0 -> args0.key.to(args0.value) }).toMap(),
tags = javaType.tags().map({ args0 -> args0 }),
timeout = javaType.timeout().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy