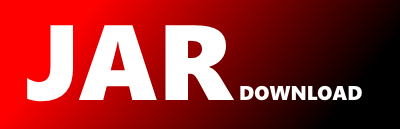
com.pulumi.gcp.cloudrun.kotlin.inputs.ServiceTemplateSpecVolumeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrun.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecVolumeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property csi A filesystem specified by the Container Storage Interface (CSI).
* Structure is documented below.
* @property emptyDir Ephemeral storage which can be backed by real disks (HD, SSD), network storage or memory (i.e. tmpfs). For now only in memory (tmpfs) is supported. It is ephemeral in the sense that when the sandbox is taken down, the data is destroyed with it (it does not persist across sandbox runs).
* Structure is documented below.
* @property name Volume's name.
* @property nfs A filesystem backed by a Network File System share. This filesystem requires the
* run.googleapis.com/execution-environment annotation to be set to "gen2" and
* run.googleapis.com/launch-stage set to "BETA" or "ALPHA".
* Structure is documented below.
* @property secret The secret's value will be presented as the content of a file whose
* name is defined in the item path. If no items are defined, the name of
* the file is the secret_name.
* Structure is documented below.
*/
public data class ServiceTemplateSpecVolumeArgs(
public val csi: Output? = null,
public val emptyDir: Output? = null,
public val name: Output,
public val nfs: Output? = null,
public val secret: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecVolumeArgs =
com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecVolumeArgs.builder()
.csi(csi?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.emptyDir(emptyDir?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.nfs(nfs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secret(secret?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ServiceTemplateSpecVolumeArgs].
*/
@PulumiTagMarker
public class ServiceTemplateSpecVolumeArgsBuilder internal constructor() {
private var csi: Output? = null
private var emptyDir: Output? = null
private var name: Output? = null
private var nfs: Output? = null
private var secret: Output? = null
/**
* @param value A filesystem specified by the Container Storage Interface (CSI).
* Structure is documented below.
*/
@JvmName("kasterkuoqsolcmp")
public suspend fun csi(`value`: Output) {
this.csi = value
}
/**
* @param value Ephemeral storage which can be backed by real disks (HD, SSD), network storage or memory (i.e. tmpfs). For now only in memory (tmpfs) is supported. It is ephemeral in the sense that when the sandbox is taken down, the data is destroyed with it (it does not persist across sandbox runs).
* Structure is documented below.
*/
@JvmName("atiwdjmahoitldkk")
public suspend fun emptyDir(`value`: Output) {
this.emptyDir = value
}
/**
* @param value Volume's name.
*/
@JvmName("gqhvfcragwobllds")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A filesystem backed by a Network File System share. This filesystem requires the
* run.googleapis.com/execution-environment annotation to be set to "gen2" and
* run.googleapis.com/launch-stage set to "BETA" or "ALPHA".
* Structure is documented below.
*/
@JvmName("vgnihyllmbkdxbdh")
public suspend fun nfs(`value`: Output) {
this.nfs = value
}
/**
* @param value The secret's value will be presented as the content of a file whose
* name is defined in the item path. If no items are defined, the name of
* the file is the secret_name.
* Structure is documented below.
*/
@JvmName("gdfyaftipqicpfxd")
public suspend fun secret(`value`: Output) {
this.secret = value
}
/**
* @param value A filesystem specified by the Container Storage Interface (CSI).
* Structure is documented below.
*/
@JvmName("ixfjypikbbglwlgr")
public suspend fun csi(`value`: ServiceTemplateSpecVolumeCsiArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.csi = mapped
}
/**
* @param argument A filesystem specified by the Container Storage Interface (CSI).
* Structure is documented below.
*/
@JvmName("ktlfsemscykdgifl")
public suspend fun csi(argument: suspend ServiceTemplateSpecVolumeCsiArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecVolumeCsiArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.csi = mapped
}
/**
* @param value Ephemeral storage which can be backed by real disks (HD, SSD), network storage or memory (i.e. tmpfs). For now only in memory (tmpfs) is supported. It is ephemeral in the sense that when the sandbox is taken down, the data is destroyed with it (it does not persist across sandbox runs).
* Structure is documented below.
*/
@JvmName("ilwiiftxepwmbbun")
public suspend fun emptyDir(`value`: ServiceTemplateSpecVolumeEmptyDirArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emptyDir = mapped
}
/**
* @param argument Ephemeral storage which can be backed by real disks (HD, SSD), network storage or memory (i.e. tmpfs). For now only in memory (tmpfs) is supported. It is ephemeral in the sense that when the sandbox is taken down, the data is destroyed with it (it does not persist across sandbox runs).
* Structure is documented below.
*/
@JvmName("tuubomvvwnsepmri")
public suspend fun emptyDir(argument: suspend ServiceTemplateSpecVolumeEmptyDirArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecVolumeEmptyDirArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.emptyDir = mapped
}
/**
* @param value Volume's name.
*/
@JvmName("nwtpsjtduvrdrdor")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value A filesystem backed by a Network File System share. This filesystem requires the
* run.googleapis.com/execution-environment annotation to be set to "gen2" and
* run.googleapis.com/launch-stage set to "BETA" or "ALPHA".
* Structure is documented below.
*/
@JvmName("vpitjhoiytujdsoc")
public suspend fun nfs(`value`: ServiceTemplateSpecVolumeNfsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nfs = mapped
}
/**
* @param argument A filesystem backed by a Network File System share. This filesystem requires the
* run.googleapis.com/execution-environment annotation to be set to "gen2" and
* run.googleapis.com/launch-stage set to "BETA" or "ALPHA".
* Structure is documented below.
*/
@JvmName("uxpdxxldywjjfuwu")
public suspend fun nfs(argument: suspend ServiceTemplateSpecVolumeNfsArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecVolumeNfsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.nfs = mapped
}
/**
* @param value The secret's value will be presented as the content of a file whose
* name is defined in the item path. If no items are defined, the name of
* the file is the secret_name.
* Structure is documented below.
*/
@JvmName("dwehlrstrmrqdory")
public suspend fun secret(`value`: ServiceTemplateSpecVolumeSecretArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secret = mapped
}
/**
* @param argument The secret's value will be presented as the content of a file whose
* name is defined in the item path. If no items are defined, the name of
* the file is the secret_name.
* Structure is documented below.
*/
@JvmName("cyhlwdayocwvfikd")
public suspend fun secret(argument: suspend ServiceTemplateSpecVolumeSecretArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecVolumeSecretArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secret = mapped
}
internal fun build(): ServiceTemplateSpecVolumeArgs = ServiceTemplateSpecVolumeArgs(
csi = csi,
emptyDir = emptyDir,
name = name ?: throw PulumiNullFieldException("name"),
nfs = nfs,
secret = secret,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy