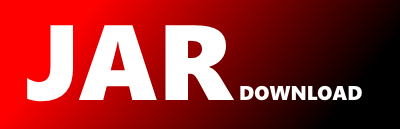
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.composer.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property airflowUri The URI of the Apache Airflow Web UI hosted within this
* environment.
* @property dagGcsPrefix The Cloud Storage prefix of the DAGs for this environment.
* Although Cloud Storage objects reside in a flat namespace, a
* hierarchical file tree can be simulated using '/'-delimited
* object name prefixes. DAG objects for this environment
* reside in a simulated directory with this prefix.
* @property dataRetentionConfig The configuration setting for Airflow data retention mechanism. This field is supported for Cloud Composer environments in versions composer-2.0.32-airflow-2.1.4. or newer
* @property databaseConfig The configuration of Cloud SQL instance that is used by the Apache Airflow software. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property enablePrivateBuildsOnly Optional. If true, builds performed during operations that install Python packages have only private connectivity to Google services. If false, the builds also have access to the internet.
* @property enablePrivateEnvironment Optional. If true, a private Composer environment will be created.
* @property encryptionConfig The encryption options for the Composer environment and its dependencies.
* @property environmentSize The size of the Cloud Composer environment. This field is supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
* @property gkeCluster The Kubernetes Engine cluster used to run this environment.
* @property maintenanceWindow The configuration for Cloud Composer maintenance window.
* @property masterAuthorizedNetworksConfig Configuration options for the master authorized networks feature. Enabled master authorized networks will disallow all external traffic to access Kubernetes master through HTTPS except traffic from the given CIDR blocks, Google Compute Engine Public IPs and Google Prod IPs.
* @property nodeConfig The configuration used for the Kubernetes Engine cluster.
* @property nodeCount The number of nodes in the Kubernetes Engine cluster that will be used to run this environment. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property privateEnvironmentConfig The configuration used for the Private IP Cloud Composer environment.
* @property recoveryConfig The recovery configuration settings for the Cloud Composer environment
* @property resilienceMode Whether high resilience is enabled or not. This field is supported for Cloud Composer environments in versions composer-2.1.15-airflow-*.*.* and newer.
* @property softwareConfig The configuration settings for software inside the environment.
* @property webServerConfig The configuration settings for the Airflow web server App Engine instance. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property webServerNetworkAccessControl Network-level access control policy for the Airflow web server.
* @property workloadsConfig The workloads configuration settings for the GKE cluster associated with the Cloud Composer environment. Supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
public data class EnvironmentConfig(
public val airflowUri: String? = null,
public val dagGcsPrefix: String? = null,
public val dataRetentionConfig: EnvironmentConfigDataRetentionConfig? = null,
public val databaseConfig: EnvironmentConfigDatabaseConfig? = null,
public val enablePrivateBuildsOnly: Boolean? = null,
public val enablePrivateEnvironment: Boolean? = null,
public val encryptionConfig: EnvironmentConfigEncryptionConfig? = null,
public val environmentSize: String? = null,
public val gkeCluster: String? = null,
public val maintenanceWindow: EnvironmentConfigMaintenanceWindow? = null,
public val masterAuthorizedNetworksConfig: EnvironmentConfigMasterAuthorizedNetworksConfig? =
null,
public val nodeConfig: EnvironmentConfigNodeConfig? = null,
public val nodeCount: Int? = null,
public val privateEnvironmentConfig: EnvironmentConfigPrivateEnvironmentConfig? = null,
public val recoveryConfig: EnvironmentConfigRecoveryConfig? = null,
public val resilienceMode: String? = null,
public val softwareConfig: EnvironmentConfigSoftwareConfig? = null,
public val webServerConfig: EnvironmentConfigWebServerConfig? = null,
public val webServerNetworkAccessControl: EnvironmentConfigWebServerNetworkAccessControl? = null,
public val workloadsConfig: EnvironmentConfigWorkloadsConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.composer.outputs.EnvironmentConfig): EnvironmentConfig = EnvironmentConfig(
airflowUri = javaType.airflowUri().map({ args0 -> args0 }).orElse(null),
dagGcsPrefix = javaType.dagGcsPrefix().map({ args0 -> args0 }).orElse(null),
dataRetentionConfig = javaType.dataRetentionConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigDataRetentionConfig.Companion.toKotlin(args0)
})
}).orElse(null),
databaseConfig = javaType.databaseConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigDatabaseConfig.Companion.toKotlin(args0)
})
}).orElse(null),
enablePrivateBuildsOnly = javaType.enablePrivateBuildsOnly().map({ args0 -> args0 }).orElse(null),
enablePrivateEnvironment = javaType.enablePrivateEnvironment().map({ args0 -> args0 }).orElse(null),
encryptionConfig = javaType.encryptionConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigEncryptionConfig.Companion.toKotlin(args0)
})
}).orElse(null),
environmentSize = javaType.environmentSize().map({ args0 -> args0 }).orElse(null),
gkeCluster = javaType.gkeCluster().map({ args0 -> args0 }).orElse(null),
maintenanceWindow = javaType.maintenanceWindow().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigMaintenanceWindow.Companion.toKotlin(args0)
})
}).orElse(null),
masterAuthorizedNetworksConfig = javaType.masterAuthorizedNetworksConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigMasterAuthorizedNetworksConfig.Companion.toKotlin(args0)
})
}).orElse(null),
nodeConfig = javaType.nodeConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigNodeConfig.Companion.toKotlin(args0)
})
}).orElse(null),
nodeCount = javaType.nodeCount().map({ args0 -> args0 }).orElse(null),
privateEnvironmentConfig = javaType.privateEnvironmentConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigPrivateEnvironmentConfig.Companion.toKotlin(args0)
})
}).orElse(null),
recoveryConfig = javaType.recoveryConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigRecoveryConfig.Companion.toKotlin(args0)
})
}).orElse(null),
resilienceMode = javaType.resilienceMode().map({ args0 -> args0 }).orElse(null),
softwareConfig = javaType.softwareConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigSoftwareConfig.Companion.toKotlin(args0)
})
}).orElse(null),
webServerConfig = javaType.webServerConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigWebServerConfig.Companion.toKotlin(args0)
})
}).orElse(null),
webServerNetworkAccessControl = javaType.webServerNetworkAccessControl().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigWebServerNetworkAccessControl.Companion.toKotlin(args0)
})
}).orElse(null),
workloadsConfig = javaType.workloadsConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.composer.kotlin.outputs.EnvironmentConfigWorkloadsConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy