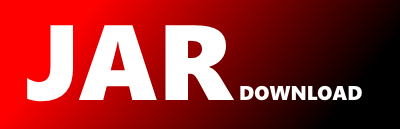
com.pulumi.gcp.compute.kotlin.InstanceTemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.InstanceTemplateArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateAdvancedMachineFeaturesArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateAdvancedMachineFeaturesArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateConfidentialInstanceConfigArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateConfidentialInstanceConfigArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateDiskArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateDiskArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateGuestAcceleratorArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateGuestAcceleratorArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateNetworkInterfaceArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateNetworkInterfaceArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateNetworkPerformanceConfigArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateNetworkPerformanceConfigArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateReservationAffinityArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateReservationAffinityArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateSchedulingArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateSchedulingArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateServiceAccountArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateServiceAccountArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateShieldedInstanceConfigArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateShieldedInstanceConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* > **Note**: Global instance templates can be used in any region. To lower the impact of outages outside your region and gain data residency within your region, use google_compute_region_instance_template.
* Manages a VM instance template resource within GCE. For more information see
* [the official documentation](https://cloud.google.com/compute/docs/instance-templates)
* and
* [API](https://cloud.google.com/compute/docs/reference/latest/instanceTemplates).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.serviceaccount.Account("default", {
* accountId: "service-account-id",
* displayName: "Service Account",
* });
* const myImage = gcp.compute.getImage({
* family: "debian-11",
* project: "debian-cloud",
* });
* const foobar = new gcp.compute.Disk("foobar", {
* name: "existing-disk",
* image: myImage.then(myImage => myImage.selfLink),
* size: 10,
* type: "pd-ssd",
* zone: "us-central1-a",
* });
* const dailyBackup = new gcp.compute.ResourcePolicy("daily_backup", {
* name: "every-day-4am",
* region: "us-central1",
* snapshotSchedulePolicy: {
* schedule: {
* dailySchedule: {
* daysInCycle: 1,
* startTime: "04:00",
* },
* },
* },
* });
* const defaultInstanceTemplate = new gcp.compute.InstanceTemplate("default", {
* name: "appserver-template",
* description: "This template is used to create app server instances.",
* tags: [
* "foo",
* "bar",
* ],
* labels: {
* environment: "dev",
* },
* instanceDescription: "description assigned to instances",
* machineType: "e2-medium",
* canIpForward: false,
* scheduling: {
* automaticRestart: true,
* onHostMaintenance: "MIGRATE",
* },
* disks: [
* {
* sourceImage: "debian-cloud/debian-11",
* autoDelete: true,
* boot: true,
* resourcePolicies: dailyBackup.id,
* },
* {
* source: foobar.name,
* autoDelete: false,
* boot: false,
* },
* ],
* networkInterfaces: [{
* network: "default",
* }],
* metadata: {
* foo: "bar",
* },
* serviceAccount: {
* email: _default.email,
* scopes: ["cloud-platform"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.serviceaccount.Account("default",
* account_id="service-account-id",
* display_name="Service Account")
* my_image = gcp.compute.get_image(family="debian-11",
* project="debian-cloud")
* foobar = gcp.compute.Disk("foobar",
* name="existing-disk",
* image=my_image.self_link,
* size=10,
* type="pd-ssd",
* zone="us-central1-a")
* daily_backup = gcp.compute.ResourcePolicy("daily_backup",
* name="every-day-4am",
* region="us-central1",
* snapshot_schedule_policy={
* "schedule": {
* "daily_schedule": {
* "days_in_cycle": 1,
* "start_time": "04:00",
* },
* },
* })
* default_instance_template = gcp.compute.InstanceTemplate("default",
* name="appserver-template",
* description="This template is used to create app server instances.",
* tags=[
* "foo",
* "bar",
* ],
* labels={
* "environment": "dev",
* },
* instance_description="description assigned to instances",
* machine_type="e2-medium",
* can_ip_forward=False,
* scheduling={
* "automatic_restart": True,
* "on_host_maintenance": "MIGRATE",
* },
* disks=[
* {
* "source_image": "debian-cloud/debian-11",
* "auto_delete": True,
* "boot": True,
* "resource_policies": daily_backup.id,
* },
* {
* "source": foobar.name,
* "auto_delete": False,
* "boot": False,
* },
* ],
* network_interfaces=[{
* "network": "default",
* }],
* metadata={
* "foo": "bar",
* },
* service_account={
* "email": default.email,
* "scopes": ["cloud-platform"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.ServiceAccount.Account("default", new()
* {
* AccountId = "service-account-id",
* DisplayName = "Service Account",
* });
* var myImage = Gcp.Compute.GetImage.Invoke(new()
* {
* Family = "debian-11",
* Project = "debian-cloud",
* });
* var foobar = new Gcp.Compute.Disk("foobar", new()
* {
* Name = "existing-disk",
* Image = myImage.Apply(getImageResult => getImageResult.SelfLink),
* Size = 10,
* Type = "pd-ssd",
* Zone = "us-central1-a",
* });
* var dailyBackup = new Gcp.Compute.ResourcePolicy("daily_backup", new()
* {
* Name = "every-day-4am",
* Region = "us-central1",
* SnapshotSchedulePolicy = new Gcp.Compute.Inputs.ResourcePolicySnapshotSchedulePolicyArgs
* {
* Schedule = new Gcp.Compute.Inputs.ResourcePolicySnapshotSchedulePolicyScheduleArgs
* {
* DailySchedule = new Gcp.Compute.Inputs.ResourcePolicySnapshotSchedulePolicyScheduleDailyScheduleArgs
* {
* DaysInCycle = 1,
* StartTime = "04:00",
* },
* },
* },
* });
* var defaultInstanceTemplate = new Gcp.Compute.InstanceTemplate("default", new()
* {
* Name = "appserver-template",
* Description = "This template is used to create app server instances.",
* Tags = new[]
* {
* "foo",
* "bar",
* },
* Labels =
* {
* { "environment", "dev" },
* },
* InstanceDescription = "description assigned to instances",
* MachineType = "e2-medium",
* CanIpForward = false,
* Scheduling = new Gcp.Compute.Inputs.InstanceTemplateSchedulingArgs
* {
* AutomaticRestart = true,
* OnHostMaintenance = "MIGRATE",
* },
* Disks = new[]
* {
* new Gcp.Compute.Inputs.InstanceTemplateDiskArgs
* {
* SourceImage = "debian-cloud/debian-11",
* AutoDelete = true,
* Boot = true,
* ResourcePolicies = dailyBackup.Id,
* },
* new Gcp.Compute.Inputs.InstanceTemplateDiskArgs
* {
* Source = foobar.Name,
* AutoDelete = false,
* Boot = false,
* },
* },
* NetworkInterfaces = new[]
* {
* new Gcp.Compute.Inputs.InstanceTemplateNetworkInterfaceArgs
* {
* Network = "default",
* },
* },
* Metadata =
* {
* { "foo", "bar" },
* },
* ServiceAccount = new Gcp.Compute.Inputs.InstanceTemplateServiceAccountArgs
* {
* Email = @default.Email,
* Scopes = new[]
* {
* "cloud-platform",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewAccount(ctx, "default", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("service-account-id"),
* DisplayName: pulumi.String("Service Account"),
* })
* if err != nil {
* return err
* }
* myImage, err := compute.LookupImage(ctx, &compute.LookupImageArgs{
* Family: pulumi.StringRef("debian-11"),
* Project: pulumi.StringRef("debian-cloud"),
* }, nil)
* if err != nil {
* return err
* }
* foobar, err := compute.NewDisk(ctx, "foobar", &compute.DiskArgs{
* Name: pulumi.String("existing-disk"),
* Image: pulumi.String(myImage.SelfLink),
* Size: pulumi.Int(10),
* Type: pulumi.String("pd-ssd"),
* Zone: pulumi.String("us-central1-a"),
* })
* if err != nil {
* return err
* }
* dailyBackup, err := compute.NewResourcePolicy(ctx, "daily_backup", &compute.ResourcePolicyArgs{
* Name: pulumi.String("every-day-4am"),
* Region: pulumi.String("us-central1"),
* SnapshotSchedulePolicy: &compute.ResourcePolicySnapshotSchedulePolicyArgs{
* Schedule: &compute.ResourcePolicySnapshotSchedulePolicyScheduleArgs{
* DailySchedule: &compute.ResourcePolicySnapshotSchedulePolicyScheduleDailyScheduleArgs{
* DaysInCycle: pulumi.Int(1),
* StartTime: pulumi.String("04:00"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = compute.NewInstanceTemplate(ctx, "default", &compute.InstanceTemplateArgs{
* Name: pulumi.String("appserver-template"),
* Description: pulumi.String("This template is used to create app server instances."),
* Tags: pulumi.StringArray{
* pulumi.String("foo"),
* pulumi.String("bar"),
* },
* Labels: pulumi.StringMap{
* "environment": pulumi.String("dev"),
* },
* InstanceDescription: pulumi.String("description assigned to instances"),
* MachineType: pulumi.String("e2-medium"),
* CanIpForward: pulumi.Bool(false),
* Scheduling: &compute.InstanceTemplateSchedulingArgs{
* AutomaticRestart: pulumi.Bool(true),
* OnHostMaintenance: pulumi.String("MIGRATE"),
* },
* Disks: compute.InstanceTemplateDiskArray{
* &compute.InstanceTemplateDiskArgs{
* SourceImage: pulumi.String("debian-cloud/debian-11"),
* AutoDelete: pulumi.Bool(true),
* Boot: pulumi.Bool(true),
* ResourcePolicies: dailyBackup.ID(),
* },
* &compute.InstanceTemplateDiskArgs{
* Source: foobar.Name,
* AutoDelete: pulumi.Bool(false),
* Boot: pulumi.Bool(false),
* },
* },
* NetworkInterfaces: compute.InstanceTemplateNetworkInterfaceArray{
* &compute.InstanceTemplateNetworkInterfaceArgs{
* Network: pulumi.String("default"),
* },
* },
* Metadata: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* ServiceAccount: &compute.InstanceTemplateServiceAccountArgs{
* Email: _default.Email,
* Scopes: pulumi.StringArray{
* pulumi.String("cloud-platform"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetImageArgs;
* import com.pulumi.gcp.compute.Disk;
* import com.pulumi.gcp.compute.DiskArgs;
* import com.pulumi.gcp.compute.ResourcePolicy;
* import com.pulumi.gcp.compute.ResourcePolicyArgs;
* import com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyArgs;
* import com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyScheduleArgs;
* import com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyScheduleDailyScheduleArgs;
* import com.pulumi.gcp.compute.InstanceTemplate;
* import com.pulumi.gcp.compute.InstanceTemplateArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateSchedulingArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateNetworkInterfaceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateServiceAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Account("default", AccountArgs.builder()
* .accountId("service-account-id")
* .displayName("Service Account")
* .build());
* final var myImage = ComputeFunctions.getImage(GetImageArgs.builder()
* .family("debian-11")
* .project("debian-cloud")
* .build());
* var foobar = new Disk("foobar", DiskArgs.builder()
* .name("existing-disk")
* .image(myImage.applyValue(getImageResult -> getImageResult.selfLink()))
* .size(10)
* .type("pd-ssd")
* .zone("us-central1-a")
* .build());
* var dailyBackup = new ResourcePolicy("dailyBackup", ResourcePolicyArgs.builder()
* .name("every-day-4am")
* .region("us-central1")
* .snapshotSchedulePolicy(ResourcePolicySnapshotSchedulePolicyArgs.builder()
* .schedule(ResourcePolicySnapshotSchedulePolicyScheduleArgs.builder()
* .dailySchedule(ResourcePolicySnapshotSchedulePolicyScheduleDailyScheduleArgs.builder()
* .daysInCycle(1)
* .startTime("04:00")
* .build())
* .build())
* .build())
* .build());
* var defaultInstanceTemplate = new InstanceTemplate("defaultInstanceTemplate", InstanceTemplateArgs.builder()
* .name("appserver-template")
* .description("This template is used to create app server instances.")
* .tags(
* "foo",
* "bar")
* .labels(Map.of("environment", "dev"))
* .instanceDescription("description assigned to instances")
* .machineType("e2-medium")
* .canIpForward(false)
* .scheduling(InstanceTemplateSchedulingArgs.builder()
* .automaticRestart(true)
* .onHostMaintenance("MIGRATE")
* .build())
* .disks(
* InstanceTemplateDiskArgs.builder()
* .sourceImage("debian-cloud/debian-11")
* .autoDelete(true)
* .boot(true)
* .resourcePolicies(dailyBackup.id())
* .build(),
* InstanceTemplateDiskArgs.builder()
* .source(foobar.name())
* .autoDelete(false)
* .boot(false)
* .build())
* .networkInterfaces(InstanceTemplateNetworkInterfaceArgs.builder()
* .network("default")
* .build())
* .metadata(Map.of("foo", "bar"))
* .serviceAccount(InstanceTemplateServiceAccountArgs.builder()
* .email(default_.email())
* .scopes("cloud-platform")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:serviceaccount:Account
* properties:
* accountId: service-account-id
* displayName: Service Account
* defaultInstanceTemplate:
* type: gcp:compute:InstanceTemplate
* name: default
* properties:
* name: appserver-template
* description: This template is used to create app server instances.
* tags:
* - foo
* - bar
* labels:
* environment: dev
* instanceDescription: description assigned to instances
* machineType: e2-medium
* canIpForward: false
* scheduling:
* automaticRestart: true
* onHostMaintenance: MIGRATE
* disks:
* - sourceImage: debian-cloud/debian-11
* autoDelete: true
* boot: true
* resourcePolicies: ${dailyBackup.id}
* - source: ${foobar.name}
* autoDelete: false
* boot: false
* networkInterfaces:
* - network: default
* metadata:
* foo: bar
* serviceAccount:
* email: ${default.email}
* scopes:
* - cloud-platform
* foobar:
* type: gcp:compute:Disk
* properties:
* name: existing-disk
* image: ${myImage.selfLink}
* size: 10
* type: pd-ssd
* zone: us-central1-a
* dailyBackup:
* type: gcp:compute:ResourcePolicy
* name: daily_backup
* properties:
* name: every-day-4am
* region: us-central1
* snapshotSchedulePolicy:
* schedule:
* dailySchedule:
* daysInCycle: 1
* startTime: 04:00
* variables:
* myImage:
* fn::invoke:
* Function: gcp:compute:getImage
* Arguments:
* family: debian-11
* project: debian-cloud
* ```
*
* ### Automatic Envoy Deployment
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const default = gcp.compute.getDefaultServiceAccount({});
* const myImage = gcp.compute.getImage({
* family: "debian-11",
* project: "debian-cloud",
* });
* const foobar = new gcp.compute.InstanceTemplate("foobar", {
* name: "appserver-template",
* machineType: "e2-medium",
* canIpForward: false,
* tags: [
* "foo",
* "bar",
* ],
* disks: [{
* sourceImage: myImage.then(myImage => myImage.selfLink),
* autoDelete: true,
* boot: true,
* }],
* networkInterfaces: [{
* network: "default",
* }],
* scheduling: {
* preemptible: false,
* automaticRestart: true,
* },
* metadata: {
* "gce-software-declaration": `{
* "softwareRecipes": [{
* "name": "install-gce-service-proxy-agent",
* "desired_state": "INSTALLED",
* "installSteps": [{
* "scriptRun": {
* "script": "#! /bin/bash\\nZONE=(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\\nexport SERVICE_PROXY_AGENT_DIRECTORY=(mktemp -d)\\nsudo gsutil cp gs://gce-service-proxy-"ZONE"/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "SERVICE_PROXY_AGENT_DIRECTORY" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "SERVICE_PROXY_AGENT_DIRECTORY"\\nsudo tar -xzf "SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent-0.2.tgz -C "SERVICE_PROXY_AGENT_DIRECTORY"\\n"SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent/service-proxy-agent-bootstrap.sh"
* }
* }]
* }]
* }
* `,
* "gce-service-proxy": `{
* "api-version": "0.2",
* "proxy-spec": {
* "proxy-port": 15001,
* "network": "my-network",
* "tracing": "ON",
* "access-log": "/var/log/envoy/access.log"
* }
* "service": {
* "serving-ports": [80, 81]
* },
* "labels": {
* "app_name": "bookserver_app",
* "app_version": "STABLE"
* }
* }
* `,
* "enable-guest-attributes": "true",
* "enable-osconfig": "true",
* },
* serviceAccount: {
* email: _default.then(_default => _default.email),
* scopes: ["cloud-platform"],
* },
* labels: {
* "gce-service-proxy": "on",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.get_default_service_account()
* my_image = gcp.compute.get_image(family="debian-11",
* project="debian-cloud")
* foobar = gcp.compute.InstanceTemplate("foobar",
* name="appserver-template",
* machine_type="e2-medium",
* can_ip_forward=False,
* tags=[
* "foo",
* "bar",
* ],
* disks=[{
* "source_image": my_image.self_link,
* "auto_delete": True,
* "boot": True,
* }],
* network_interfaces=[{
* "network": "default",
* }],
* scheduling={
* "preemptible": False,
* "automatic_restart": True,
* },
* metadata={
* "gce-software-declaration": """{
* "softwareRecipes": [{
* "name": "install-gce-service-proxy-agent",
* "desired_state": "INSTALLED",
* "installSteps": [{
* "scriptRun": {
* "script": "#! /bin/bash\nZONE=$(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\nexport SERVICE_PROXY_AGENT_DIRECTORY=$(mktemp -d)\nsudo gsutil cp gs://gce-service-proxy-"$ZONE"/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY"\nsudo tar -xzf "$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent-0.2.tgz -C "$SERVICE_PROXY_AGENT_DIRECTORY"\n"$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent/service-proxy-agent-bootstrap.sh"
* }
* }]
* }]
* }
* """,
* "gce-service-proxy": """{
* "api-version": "0.2",
* "proxy-spec": {
* "proxy-port": 15001,
* "network": "my-network",
* "tracing": "ON",
* "access-log": "/var/log/envoy/access.log"
* }
* "service": {
* "serving-ports": [80, 81]
* },
* "labels": {
* "app_name": "bookserver_app",
* "app_version": "STABLE"
* }
* }
* """,
* "enable-guest-attributes": "true",
* "enable-osconfig": "true",
* },
* service_account={
* "email": default.email,
* "scopes": ["cloud-platform"],
* },
* labels={
* "gce-service-proxy": "on",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Compute.GetDefaultServiceAccount.Invoke();
* var myImage = Gcp.Compute.GetImage.Invoke(new()
* {
* Family = "debian-11",
* Project = "debian-cloud",
* });
* var foobar = new Gcp.Compute.InstanceTemplate("foobar", new()
* {
* Name = "appserver-template",
* MachineType = "e2-medium",
* CanIpForward = false,
* Tags = new[]
* {
* "foo",
* "bar",
* },
* Disks = new[]
* {
* new Gcp.Compute.Inputs.InstanceTemplateDiskArgs
* {
* SourceImage = myImage.Apply(getImageResult => getImageResult.SelfLink),
* AutoDelete = true,
* Boot = true,
* },
* },
* NetworkInterfaces = new[]
* {
* new Gcp.Compute.Inputs.InstanceTemplateNetworkInterfaceArgs
* {
* Network = "default",
* },
* },
* Scheduling = new Gcp.Compute.Inputs.InstanceTemplateSchedulingArgs
* {
* Preemptible = false,
* AutomaticRestart = true,
* },
* Metadata =
* {
* { "gce-software-declaration", @"{
* ""softwareRecipes"": [{
* ""name"": ""install-gce-service-proxy-agent"",
* ""desired_state"": ""INSTALLED"",
* ""installSteps"": [{
* ""scriptRun"": {
* ""script"": ""#! /bin/bash\nZONE=$(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\nexport SERVICE_PROXY_AGENT_DIRECTORY=$(mktemp -d)\nsudo gsutil cp gs://gce-service-proxy-""$ZONE""/service-proxy-agent/releases/service-proxy-agent-0.2.tgz ""$SERVICE_PROXY_AGENT_DIRECTORY"" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz ""$SERVICE_PROXY_AGENT_DIRECTORY""\nsudo tar -xzf ""$SERVICE_PROXY_AGENT_DIRECTORY""/service-proxy-agent-0.2.tgz -C ""$SERVICE_PROXY_AGENT_DIRECTORY""\n""$SERVICE_PROXY_AGENT_DIRECTORY""/service-proxy-agent/service-proxy-agent-bootstrap.sh""
* }
* }]
* }]
* }
* " },
* { "gce-service-proxy", @"{
* ""api-version"": ""0.2"",
* ""proxy-spec"": {
* ""proxy-port"": 15001,
* ""network"": ""my-network"",
* ""tracing"": ""ON"",
* ""access-log"": ""/var/log/envoy/access.log""
* }
* ""service"": {
* ""serving-ports"": [80, 81]
* },
* ""labels"": {
* ""app_name"": ""bookserver_app"",
* ""app_version"": ""STABLE""
* }
* }
* " },
* { "enable-guest-attributes", "true" },
* { "enable-osconfig", "true" },
* },
* ServiceAccount = new Gcp.Compute.Inputs.InstanceTemplateServiceAccountArgs
* {
* Email = @default.Apply(@default => @default.Apply(getDefaultServiceAccountResult => getDefaultServiceAccountResult.Email)),
* Scopes = new[]
* {
* "cloud-platform",
* },
* },
* Labels =
* {
* { "gce-service-proxy", "on" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _default, err := compute.GetDefaultServiceAccount(ctx, nil, nil)
* if err != nil {
* return err
* }
* myImage, err := compute.LookupImage(ctx, &compute.LookupImageArgs{
* Family: pulumi.StringRef("debian-11"),
* Project: pulumi.StringRef("debian-cloud"),
* }, nil)
* if err != nil {
* return err
* }
* _, err = compute.NewInstanceTemplate(ctx, "foobar", &compute.InstanceTemplateArgs{
* Name: pulumi.String("appserver-template"),
* MachineType: pulumi.String("e2-medium"),
* CanIpForward: pulumi.Bool(false),
* Tags: pulumi.StringArray{
* pulumi.String("foo"),
* pulumi.String("bar"),
* },
* Disks: compute.InstanceTemplateDiskArray{
* &compute.InstanceTemplateDiskArgs{
* SourceImage: pulumi.String(myImage.SelfLink),
* AutoDelete: pulumi.Bool(true),
* Boot: pulumi.Bool(true),
* },
* },
* NetworkInterfaces: compute.InstanceTemplateNetworkInterfaceArray{
* &compute.InstanceTemplateNetworkInterfaceArgs{
* Network: pulumi.String("default"),
* },
* },
* Scheduling: &compute.InstanceTemplateSchedulingArgs{
* Preemptible: pulumi.Bool(false),
* AutomaticRestart: pulumi.Bool(true),
* },
* Metadata: pulumi.StringMap{
* "gce-software-declaration": pulumi.String(`{
* "softwareRecipes": [{
* "name": "install-gce-service-proxy-agent",
* "desired_state": "INSTALLED",
* "installSteps": [{
* "scriptRun": {
* "script": "#! /bin/bash\nZONE=$(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\nexport SERVICE_PROXY_AGENT_DIRECTORY=$(mktemp -d)\nsudo gsutil cp gs://gce-service-proxy-"$ZONE"/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY"\nsudo tar -xzf "$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent-0.2.tgz -C "$SERVICE_PROXY_AGENT_DIRECTORY"\n"$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent/service-proxy-agent-bootstrap.sh"
* }
* }]
* }]
* }
* `),
* "gce-service-proxy": pulumi.String(`{
* "api-version": "0.2",
* "proxy-spec": {
* "proxy-port": 15001,
* "network": "my-network",
* "tracing": "ON",
* "access-log": "/var/log/envoy/access.log"
* }
* "service": {
* "serving-ports": [80, 81]
* },
* "labels": {
* "app_name": "bookserver_app",
* "app_version": "STABLE"
* }
* }
* `),
* "enable-guest-attributes": pulumi.String("true"),
* "enable-osconfig": pulumi.String("true"),
* },
* ServiceAccount: &compute.InstanceTemplateServiceAccountArgs{
* Email: pulumi.String(_default.Email),
* Scopes: pulumi.StringArray{
* pulumi.String("cloud-platform"),
* },
* },
* Labels: pulumi.StringMap{
* "gce-service-proxy": pulumi.String("on"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetDefaultServiceAccountArgs;
* import com.pulumi.gcp.compute.inputs.GetImageArgs;
* import com.pulumi.gcp.compute.InstanceTemplate;
* import com.pulumi.gcp.compute.InstanceTemplateArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateNetworkInterfaceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateSchedulingArgs;
* import com.pulumi.gcp.compute.inputs.InstanceTemplateServiceAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = ComputeFunctions.getDefaultServiceAccount();
* final var myImage = ComputeFunctions.getImage(GetImageArgs.builder()
* .family("debian-11")
* .project("debian-cloud")
* .build());
* var foobar = new InstanceTemplate("foobar", InstanceTemplateArgs.builder()
* .name("appserver-template")
* .machineType("e2-medium")
* .canIpForward(false)
* .tags(
* "foo",
* "bar")
* .disks(InstanceTemplateDiskArgs.builder()
* .sourceImage(myImage.applyValue(getImageResult -> getImageResult.selfLink()))
* .autoDelete(true)
* .boot(true)
* .build())
* .networkInterfaces(InstanceTemplateNetworkInterfaceArgs.builder()
* .network("default")
* .build())
* .scheduling(InstanceTemplateSchedulingArgs.builder()
* .preemptible(false)
* .automaticRestart(true)
* .build())
* .metadata(Map.ofEntries(
* Map.entry("gce-software-declaration", """
* {
* "softwareRecipes": [{
* "name": "install-gce-service-proxy-agent",
* "desired_state": "INSTALLED",
* "installSteps": [{
* "scriptRun": {
* "script": "#! /bin/bash\nZONE=$(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\nexport SERVICE_PROXY_AGENT_DIRECTORY=$(mktemp -d)\nsudo gsutil cp gs://gce-service-proxy-"$ZONE"/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY"\nsudo tar -xzf "$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent-0.2.tgz -C "$SERVICE_PROXY_AGENT_DIRECTORY"\n"$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent/service-proxy-agent-bootstrap.sh"
* }
* }]
* }]
* }
* """),
* Map.entry("gce-service-proxy", """
* {
* "api-version": "0.2",
* "proxy-spec": {
* "proxy-port": 15001,
* "network": "my-network",
* "tracing": "ON",
* "access-log": "/var/log/envoy/access.log"
* }
* "service": {
* "serving-ports": [80, 81]
* },
* "labels": {
* "app_name": "bookserver_app",
* "app_version": "STABLE"
* }
* }
* """),
* Map.entry("enable-guest-attributes", "true"),
* Map.entry("enable-osconfig", "true")
* ))
* .serviceAccount(InstanceTemplateServiceAccountArgs.builder()
* .email(default_.email())
* .scopes("cloud-platform")
* .build())
* .labels(Map.of("gce-service-proxy", "on"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foobar:
* type: gcp:compute:InstanceTemplate
* properties:
* name: appserver-template
* machineType: e2-medium
* canIpForward: false
* tags:
* - foo
* - bar
* disks:
* - sourceImage: ${myImage.selfLink}
* autoDelete: true
* boot: true
* networkInterfaces:
* - network: default
* scheduling:
* preemptible: false
* automaticRestart: true
* metadata:
* gce-software-declaration: |
* {
* "softwareRecipes": [{
* "name": "install-gce-service-proxy-agent",
* "desired_state": "INSTALLED",
* "installSteps": [{
* "scriptRun": {
* "script": "#! /bin/bash\nZONE=$(curl --silent http://metadata.google.internal/computeMetadata/v1/instance/zone -H Metadata-Flavor:Google | cut -d/ -f4 )\nexport SERVICE_PROXY_AGENT_DIRECTORY=$(mktemp -d)\nsudo gsutil cp gs://gce-service-proxy-"$ZONE"/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY" || sudo gsutil cp gs://gce-service-proxy/service-proxy-agent/releases/service-proxy-agent-0.2.tgz "$SERVICE_PROXY_AGENT_DIRECTORY"\nsudo tar -xzf "$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent-0.2.tgz -C "$SERVICE_PROXY_AGENT_DIRECTORY"\n"$SERVICE_PROXY_AGENT_DIRECTORY"/service-proxy-agent/service-proxy-agent-bootstrap.sh"
* }
* }]
* }]
* }
* gce-service-proxy: |
* {
* "api-version": "0.2",
* "proxy-spec": {
* "proxy-port": 15001,
* "network": "my-network",
* "tracing": "ON",
* "access-log": "/var/log/envoy/access.log"
* }
* "service": {
* "serving-ports": [80, 81]
* },
* "labels": {
* "app_name": "bookserver_app",
* "app_version": "STABLE"
* }
* }
* enable-guest-attributes: 'true'
* enable-osconfig: 'true'
* serviceAccount:
* email: ${default.email}
* scopes:
* - cloud-platform
* labels:
* gce-service-proxy: on
* variables:
* default:
* fn::invoke:
* Function: gcp:compute:getDefaultServiceAccount
* Arguments: {}
* myImage:
* fn::invoke:
* Function: gcp:compute:getImage
* Arguments:
* family: debian-11
* project: debian-cloud
* ```
*
* ## Deploying the Latest Image
* A common way to use instance templates and managed instance groups is to deploy the
* latest image in a family, usually the latest build of your application. There are two
* ways to do this in the provider, and they have their pros and cons. The difference ends
* up being in how "latest" is interpreted. You can either deploy the latest image available
* when the provider runs, or you can have each instance check what the latest image is when
* it's being created, either as part of a scaling event or being rebuilt by the instance
* group manager.
* If you're not sure, we recommend deploying the latest image available when the provider runs,
* because this means all the instances in your group will be based on the same image, always,
* and means that no upgrades or changes to your instances happen outside of a `pulumi up`.
* You can achieve this by using the `gcp.compute.Image`
* data source, which will retrieve the latest image on every `pulumi apply`, and will update
* the template to use that specific image:
* ```tf
* data "google_compute_image" "my_image" {
* family = "debian-11"
* project = "debian-cloud"
* }
* resource "google_compute_instance_template" "instance_template" {
* name_prefix = "instance-template-"
* machine_type = "e2-medium"
* region = "us-central1"
* // boot disk
* disk {
* source_image = data.google_compute_image.my_image.self_link
* }
* }
* ```
* To have instances update to the latest on every scaling event or instance re-creation,
* use the family as the image for the disk, and it will use GCP's default behavior, setting
* the image for the template to the family:
* ```tf
* resource "google_compute_instance_template" "instance_template" {
* name_prefix = "instance-template-"
* machine_type = "e2-medium"
* region = "us-central1"
* // boot disk
* disk {
* source_image = "debian-cloud/debian-11"
* }
* }
* ```
* ## Import
* Instance templates can be imported using any of these accepted formats:
* * `projects/{{project}}/global/instanceTemplates/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, instance templates can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/instanceTemplate:InstanceTemplate default projects/{{project}}/global/instanceTemplates/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/instanceTemplate:InstanceTemplate default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/instanceTemplate:InstanceTemplate default {{name}}
* ```
* @property advancedMachineFeatures Configure Nested Virtualisation and Simultaneous Hyper Threading on this VM. Structure is documented below
* @property canIpForward Whether to allow sending and receiving of
* packets with non-matching source or destination IPs. This defaults to false.
* @property confidentialInstanceConfig Enable [Confidential Mode](https://cloud.google.com/compute/confidential-vm/docs/about-cvm) on this VM. Structure is documented below
* @property description A brief description of this resource.
* @property disks Disks to attach to instances created from this template.
* This can be specified multiple times for multiple disks. Structure is
* documented below.
* @property enableDisplay Enable [Virtual Displays](https://cloud.google.com/compute/docs/instances/enable-instance-virtual-display#verify_display_driver) on this instance.
* **Note**: `allow_stopping_for_update` must be set to true in order to update this field.
* @property guestAccelerators List of the type and count of accelerator cards attached to the instance. Structure documented below.
* @property instanceDescription A brief description to use for instances
* created from this template.
* @property labels A set of key/value label pairs to assign to instances
* created from this template.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field 'effective_labels' for all of the labels present on the resource.
* @property machineType The machine type to create.
* To create a machine with a [custom type](https://cloud.google.com/dataproc/docs/concepts/compute/custom-machine-types) (such as extended memory), format the value like `custom-VCPUS-MEM_IN_MB` like `custom-6-20480` for 6 vCPU and 20GB of RAM.
* - - -
* @property metadata Metadata key/value pairs to make available from
* within instances created from this template.
* @property metadataStartupScript An alternative to using the
* startup-script metadata key, mostly to match the compute_instance resource.
* This replaces the startup-script metadata key on the created instance and
* thus the two mechanisms are not allowed to be used simultaneously.
* @property minCpuPlatform Specifies a minimum CPU platform. Applicable values are the friendly names of CPU platforms, such as
* `Intel Haswell` or `Intel Skylake`. See the complete list [here](https://cloud.google.com/compute/docs/instances/specify-min-cpu-platform).
* @property name The name of the instance template. If you leave
* this blank, the provider will auto-generate a unique name.
* @property namePrefix Creates a unique name beginning with the specified
* prefix. Conflicts with `name`.
* @property networkInterfaces Networks to attach to instances created from
* this template. This can be specified multiple times for multiple networks.
* Structure is documented below.
* @property networkPerformanceConfig (Optional, Configures network performance settings for the instance created from the
* template. Structure is documented below. **Note**: `machine_type`
* must be a [supported type](https://cloud.google.com/compute/docs/networking/configure-vm-with-high-bandwidth-configuration),
* the `image` used must include the [`GVNIC`](https://cloud.google.com/compute/docs/networking/using-gvnic#create-instance-gvnic-image)
* in `guest-os-features`, and `network_interface.0.nic-type` must be `GVNIC`
* in order for this setting to take effect.
* @property partnerMetadata Beta key/value pair represents partner metadata assigned to instance template where key represent a defined namespace and value is a json string represent the entries associted with the namespace.
* @property project The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
* @property region An instance template is a global resource that is not
* bound to a zone or a region. However, you can still specify some regional
* resources in an instance template, which restricts the template to the
* region where that resource resides. For example, a custom `subnetwork`
* resource is tied to a specific region. Defaults to the region of the
* Provider if no value is given.
* @property reservationAffinity Specifies the reservations that this instance can consume from.
* Structure is documented below.
* @property resourceManagerTags A set of key/value resource manager tag pairs to bind to the instances. Keys must be in the format tagKeys/{tag_key_id}, and values are in the format tagValues/456.
* @property resourcePolicies - A list of self_links of resource policies to attach to the instance. Modifying this list will cause the instance to recreate. Currently a max of 1 resource policy is supported.
* @property scheduling The scheduling strategy to use. More details about
* this configuration option are detailed below.
* @property serviceAccount Service account to attach to the instance. Structure is documented below.
* @property shieldedInstanceConfig Enable [Shielded VM](https://cloud.google.com/security/shielded-cloud/shielded-vm) on this instance. Shielded VM provides verifiable integrity to prevent against malware and rootkits. Defaults to disabled. Structure is documented below.
* **Note**: `shielded_instance_config` can only be used with boot images with shielded vm support. See the complete list [here](https://cloud.google.com/compute/docs/images#shielded-images).
* @property tags Tags to attach to the instance.
*/
public data class InstanceTemplateArgs(
public val advancedMachineFeatures: Output? = null,
public val canIpForward: Output? = null,
public val confidentialInstanceConfig: Output? =
null,
public val description: Output? = null,
public val disks: Output>? = null,
public val enableDisplay: Output? = null,
public val guestAccelerators: Output>? = null,
public val instanceDescription: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy