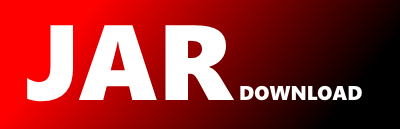
com.pulumi.gcp.container.kotlin.NodePool.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.container.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.container.kotlin.outputs.NodePoolAutoscaling
import com.pulumi.gcp.container.kotlin.outputs.NodePoolManagement
import com.pulumi.gcp.container.kotlin.outputs.NodePoolNetworkConfig
import com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfig
import com.pulumi.gcp.container.kotlin.outputs.NodePoolPlacementPolicy
import com.pulumi.gcp.container.kotlin.outputs.NodePoolQueuedProvisioning
import com.pulumi.gcp.container.kotlin.outputs.NodePoolUpgradeSettings
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.container.kotlin.outputs.NodePoolAutoscaling.Companion.toKotlin as nodePoolAutoscalingToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolManagement.Companion.toKotlin as nodePoolManagementToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolNetworkConfig.Companion.toKotlin as nodePoolNetworkConfigToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolNodeConfig.Companion.toKotlin as nodePoolNodeConfigToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolPlacementPolicy.Companion.toKotlin as nodePoolPlacementPolicyToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolQueuedProvisioning.Companion.toKotlin as nodePoolQueuedProvisioningToKotlin
import com.pulumi.gcp.container.kotlin.outputs.NodePoolUpgradeSettings.Companion.toKotlin as nodePoolUpgradeSettingsToKotlin
/**
* Builder for [NodePool].
*/
@PulumiTagMarker
public class NodePoolResourceBuilder internal constructor() {
public var name: String? = null
public var args: NodePoolArgs = NodePoolArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend NodePoolArgsBuilder.() -> Unit) {
val builder = NodePoolArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): NodePool {
val builtJavaResource = com.pulumi.gcp.container.NodePool(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return NodePool(builtJavaResource)
}
}
/**
* Manages a node pool in a Google Kubernetes Engine (GKE) cluster separately from
* the cluster control plane. For more information see [the official documentation](https://cloud.google.com/container-engine/docs/node-pools)
* and [the API reference](https://cloud.google.com/kubernetes-engine/docs/reference/rest/v1beta1/projects.locations.clusters.nodePools).
* ## Example Usage
* ### Using A Separately Managed Node Pool (Recommended)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.serviceaccount.Account("default", {
* accountId: "service-account-id",
* displayName: "Service Account",
* });
* const primary = new gcp.container.Cluster("primary", {
* name: "my-gke-cluster",
* location: "us-central1",
* removeDefaultNodePool: true,
* initialNodeCount: 1,
* });
* const primaryPreemptibleNodes = new gcp.container.NodePool("primary_preemptible_nodes", {
* name: "my-node-pool",
* cluster: primary.id,
* nodeCount: 1,
* nodeConfig: {
* preemptible: true,
* machineType: "e2-medium",
* serviceAccount: _default.email,
* oauthScopes: ["https://www.googleapis.com/auth/cloud-platform"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.serviceaccount.Account("default",
* account_id="service-account-id",
* display_name="Service Account")
* primary = gcp.container.Cluster("primary",
* name="my-gke-cluster",
* location="us-central1",
* remove_default_node_pool=True,
* initial_node_count=1)
* primary_preemptible_nodes = gcp.container.NodePool("primary_preemptible_nodes",
* name="my-node-pool",
* cluster=primary.id,
* node_count=1,
* node_config={
* "preemptible": True,
* "machine_type": "e2-medium",
* "service_account": default.email,
* "oauth_scopes": ["https://www.googleapis.com/auth/cloud-platform"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.ServiceAccount.Account("default", new()
* {
* AccountId = "service-account-id",
* DisplayName = "Service Account",
* });
* var primary = new Gcp.Container.Cluster("primary", new()
* {
* Name = "my-gke-cluster",
* Location = "us-central1",
* RemoveDefaultNodePool = true,
* InitialNodeCount = 1,
* });
* var primaryPreemptibleNodes = new Gcp.Container.NodePool("primary_preemptible_nodes", new()
* {
* Name = "my-node-pool",
* Cluster = primary.Id,
* NodeCount = 1,
* NodeConfig = new Gcp.Container.Inputs.NodePoolNodeConfigArgs
* {
* Preemptible = true,
* MachineType = "e2-medium",
* ServiceAccount = @default.Email,
* OauthScopes = new[]
* {
* "https://www.googleapis.com/auth/cloud-platform",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/container"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewAccount(ctx, "default", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("service-account-id"),
* DisplayName: pulumi.String("Service Account"),
* })
* if err != nil {
* return err
* }
* primary, err := container.NewCluster(ctx, "primary", &container.ClusterArgs{
* Name: pulumi.String("my-gke-cluster"),
* Location: pulumi.String("us-central1"),
* RemoveDefaultNodePool: pulumi.Bool(true),
* InitialNodeCount: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = container.NewNodePool(ctx, "primary_preemptible_nodes", &container.NodePoolArgs{
* Name: pulumi.String("my-node-pool"),
* Cluster: primary.ID(),
* NodeCount: pulumi.Int(1),
* NodeConfig: &container.NodePoolNodeConfigArgs{
* Preemptible: pulumi.Bool(true),
* MachineType: pulumi.String("e2-medium"),
* ServiceAccount: _default.Email,
* OauthScopes: pulumi.StringArray{
* pulumi.String("https://www.googleapis.com/auth/cloud-platform"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.container.Cluster;
* import com.pulumi.gcp.container.ClusterArgs;
* import com.pulumi.gcp.container.NodePool;
* import com.pulumi.gcp.container.NodePoolArgs;
* import com.pulumi.gcp.container.inputs.NodePoolNodeConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Account("default", AccountArgs.builder()
* .accountId("service-account-id")
* .displayName("Service Account")
* .build());
* var primary = new Cluster("primary", ClusterArgs.builder()
* .name("my-gke-cluster")
* .location("us-central1")
* .removeDefaultNodePool(true)
* .initialNodeCount(1)
* .build());
* var primaryPreemptibleNodes = new NodePool("primaryPreemptibleNodes", NodePoolArgs.builder()
* .name("my-node-pool")
* .cluster(primary.id())
* .nodeCount(1)
* .nodeConfig(NodePoolNodeConfigArgs.builder()
* .preemptible(true)
* .machineType("e2-medium")
* .serviceAccount(default_.email())
* .oauthScopes("https://www.googleapis.com/auth/cloud-platform")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:serviceaccount:Account
* properties:
* accountId: service-account-id
* displayName: Service Account
* primary:
* type: gcp:container:Cluster
* properties:
* name: my-gke-cluster
* location: us-central1
* removeDefaultNodePool: true
* initialNodeCount: 1
* primaryPreemptibleNodes:
* type: gcp:container:NodePool
* name: primary_preemptible_nodes
* properties:
* name: my-node-pool
* cluster: ${primary.id}
* nodeCount: 1
* nodeConfig:
* preemptible: true
* machineType: e2-medium
* serviceAccount: ${default.email}
* oauthScopes:
* - https://www.googleapis.com/auth/cloud-platform
* ```
*
* ### 2 Node Pools, 1 Separately Managed + The Default Node Pool
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.serviceaccount.Account("default", {
* accountId: "service-account-id",
* displayName: "Service Account",
* });
* const primary = new gcp.container.Cluster("primary", {
* name: "marcellus-wallace",
* location: "us-central1-a",
* initialNodeCount: 3,
* nodeLocations: ["us-central1-c"],
* nodeConfig: {
* serviceAccount: _default.email,
* oauthScopes: ["https://www.googleapis.com/auth/cloud-platform"],
* guestAccelerators: [{
* type: "nvidia-tesla-k80",
* count: 1,
* }],
* },
* });
* const np = new gcp.container.NodePool("np", {
* name: "my-node-pool",
* cluster: primary.id,
* nodeConfig: {
* machineType: "e2-medium",
* serviceAccount: _default.email,
* oauthScopes: ["https://www.googleapis.com/auth/cloud-platform"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.serviceaccount.Account("default",
* account_id="service-account-id",
* display_name="Service Account")
* primary = gcp.container.Cluster("primary",
* name="marcellus-wallace",
* location="us-central1-a",
* initial_node_count=3,
* node_locations=["us-central1-c"],
* node_config={
* "service_account": default.email,
* "oauth_scopes": ["https://www.googleapis.com/auth/cloud-platform"],
* "guest_accelerators": [{
* "type": "nvidia-tesla-k80",
* "count": 1,
* }],
* })
* np = gcp.container.NodePool("np",
* name="my-node-pool",
* cluster=primary.id,
* node_config={
* "machine_type": "e2-medium",
* "service_account": default.email,
* "oauth_scopes": ["https://www.googleapis.com/auth/cloud-platform"],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.ServiceAccount.Account("default", new()
* {
* AccountId = "service-account-id",
* DisplayName = "Service Account",
* });
* var primary = new Gcp.Container.Cluster("primary", new()
* {
* Name = "marcellus-wallace",
* Location = "us-central1-a",
* InitialNodeCount = 3,
* NodeLocations = new[]
* {
* "us-central1-c",
* },
* NodeConfig = new Gcp.Container.Inputs.ClusterNodeConfigArgs
* {
* ServiceAccount = @default.Email,
* OauthScopes = new[]
* {
* "https://www.googleapis.com/auth/cloud-platform",
* },
* GuestAccelerators = new[]
* {
* new Gcp.Container.Inputs.ClusterNodeConfigGuestAcceleratorArgs
* {
* Type = "nvidia-tesla-k80",
* Count = 1,
* },
* },
* },
* });
* var np = new Gcp.Container.NodePool("np", new()
* {
* Name = "my-node-pool",
* Cluster = primary.Id,
* NodeConfig = new Gcp.Container.Inputs.NodePoolNodeConfigArgs
* {
* MachineType = "e2-medium",
* ServiceAccount = @default.Email,
* OauthScopes = new[]
* {
* "https://www.googleapis.com/auth/cloud-platform",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/container"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewAccount(ctx, "default", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("service-account-id"),
* DisplayName: pulumi.String("Service Account"),
* })
* if err != nil {
* return err
* }
* primary, err := container.NewCluster(ctx, "primary", &container.ClusterArgs{
* Name: pulumi.String("marcellus-wallace"),
* Location: pulumi.String("us-central1-a"),
* InitialNodeCount: pulumi.Int(3),
* NodeLocations: pulumi.StringArray{
* pulumi.String("us-central1-c"),
* },
* NodeConfig: &container.ClusterNodeConfigArgs{
* ServiceAccount: _default.Email,
* OauthScopes: pulumi.StringArray{
* pulumi.String("https://www.googleapis.com/auth/cloud-platform"),
* },
* GuestAccelerators: container.ClusterNodeConfigGuestAcceleratorArray{
* &container.ClusterNodeConfigGuestAcceleratorArgs{
* Type: pulumi.String("nvidia-tesla-k80"),
* Count: pulumi.Int(1),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = container.NewNodePool(ctx, "np", &container.NodePoolArgs{
* Name: pulumi.String("my-node-pool"),
* Cluster: primary.ID(),
* NodeConfig: &container.NodePoolNodeConfigArgs{
* MachineType: pulumi.String("e2-medium"),
* ServiceAccount: _default.Email,
* OauthScopes: pulumi.StringArray{
* pulumi.String("https://www.googleapis.com/auth/cloud-platform"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.container.Cluster;
* import com.pulumi.gcp.container.ClusterArgs;
* import com.pulumi.gcp.container.inputs.ClusterNodeConfigArgs;
* import com.pulumi.gcp.container.NodePool;
* import com.pulumi.gcp.container.NodePoolArgs;
* import com.pulumi.gcp.container.inputs.NodePoolNodeConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Account("default", AccountArgs.builder()
* .accountId("service-account-id")
* .displayName("Service Account")
* .build());
* var primary = new Cluster("primary", ClusterArgs.builder()
* .name("marcellus-wallace")
* .location("us-central1-a")
* .initialNodeCount(3)
* .nodeLocations("us-central1-c")
* .nodeConfig(ClusterNodeConfigArgs.builder()
* .serviceAccount(default_.email())
* .oauthScopes("https://www.googleapis.com/auth/cloud-platform")
* .guestAccelerators(ClusterNodeConfigGuestAcceleratorArgs.builder()
* .type("nvidia-tesla-k80")
* .count(1)
* .build())
* .build())
* .build());
* var np = new NodePool("np", NodePoolArgs.builder()
* .name("my-node-pool")
* .cluster(primary.id())
* .nodeConfig(NodePoolNodeConfigArgs.builder()
* .machineType("e2-medium")
* .serviceAccount(default_.email())
* .oauthScopes("https://www.googleapis.com/auth/cloud-platform")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:serviceaccount:Account
* properties:
* accountId: service-account-id
* displayName: Service Account
* np:
* type: gcp:container:NodePool
* properties:
* name: my-node-pool
* cluster: ${primary.id}
* nodeConfig:
* machineType: e2-medium
* serviceAccount: ${default.email}
* oauthScopes:
* - https://www.googleapis.com/auth/cloud-platform
* primary:
* type: gcp:container:Cluster
* properties:
* name: marcellus-wallace
* location: us-central1-a
* initialNodeCount: 3
* nodeLocations:
* - us-central1-c
* nodeConfig:
* serviceAccount: ${default.email}
* oauthScopes:
* - https://www.googleapis.com/auth/cloud-platform
* guestAccelerators:
* - type: nvidia-tesla-k80
* count: 1
* ```
*
* ## Import
* Node pools can be imported using the `project`, `location`, `cluster` and `name`. If
* the project is omitted, the project value in the provider configuration will be used. Examples:
* * `{{project_id}}/{{location}}/{{cluster_id}}/{{pool_id}}`
* * `{{location}}/{{cluster_id}}/{{pool_id}}`
* When using the `pulumi import` command, node pools can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:container/nodePool:NodePool default {{project_id}}/{{location}}/{{cluster_id}}/{{pool_id}}
* ```
* ```sh
* $ pulumi import gcp:container/nodePool:NodePool default {{location}}/{{cluster_id}}/{{pool_id}}
* ```
*/
public class NodePool internal constructor(
override val javaResource: com.pulumi.gcp.container.NodePool,
) : KotlinCustomResource(javaResource, NodePoolMapper) {
/**
* Configuration required by cluster autoscaler to adjust
* the size of the node pool to the current cluster usage. Structure is documented below.
*/
public val autoscaling: Output?
get() = javaResource.autoscaling().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
nodePoolAutoscalingToKotlin(args0)
})
}).orElse(null)
})
/**
* The cluster to create the node pool for. Cluster must be present in `location` provided for clusters. May be specified in the format `projects/{{project}}/locations/{{location}}/clusters/{{cluster}}` or as just the name of the cluster.
* - - -
*/
public val cluster: Output
get() = javaResource.cluster().applyValue({ args0 -> args0 })
/**
* The initial number of nodes for the pool. In
* regional or multi-zonal clusters, this is the number of nodes per zone. Changing
* this will force recreation of the resource. WARNING: Resizing your node pool manually
* may change this value in your existing cluster, which will trigger destruction
* and recreation on the next provider run (to rectify the discrepancy). If you don't
* need this value, don't set it. If you do need it, you can use a lifecycle block to
* ignore subsqeuent changes to this field.
*/
public val initialNodeCount: Output
get() = javaResource.initialNodeCount().applyValue({ args0 -> args0 })
/**
* The resource URLs of the managed instance groups associated with this node pool.
*/
public val instanceGroupUrls: Output>
get() = javaResource.instanceGroupUrls().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The location (region or zone) of the cluster.
* - - -
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* List of instance group URLs which have been assigned to this node pool.
*/
public val managedInstanceGroupUrls: Output>
get() = javaResource.managedInstanceGroupUrls().applyValue({ args0 ->
args0.map({ args0 ->
args0
})
})
/**
* Node management configuration, wherein auto-repair and
* auto-upgrade is configured. Structure is documented below.
*/
public val management: Output
get() = javaResource.management().applyValue({ args0 ->
args0.let({ args0 ->
nodePoolManagementToKotlin(args0)
})
})
/**
* The maximum number of pods per node in this node pool.
* Note that this does not work on node pools which are "route-based" - that is, node
* pools belonging to clusters that do not have IP Aliasing enabled.
* See the [official documentation](https://cloud.google.com/kubernetes-engine/docs/how-to/flexible-pod-cidr)
* for more information.
*/
public val maxPodsPerNode: Output
get() = javaResource.maxPodsPerNode().applyValue({ args0 -> args0 })
/**
* The name of the node pool. If left blank, the provider will
* auto-generate a unique name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Creates a unique name for the node pool beginning
* with the specified prefix. Conflicts with `name`.
*/
public val namePrefix: Output
get() = javaResource.namePrefix().applyValue({ args0 -> args0 })
/**
* The network configuration of the pool. Such as
* configuration for [Adding Pod IP address ranges](https://cloud.google.com/kubernetes-engine/docs/how-to/multi-pod-cidr)) to the node pool. Or enabling private nodes. Structure is
* documented below
*/
public val networkConfig: Output
get() = javaResource.networkConfig().applyValue({ args0 ->
args0.let({ args0 ->
nodePoolNetworkConfigToKotlin(args0)
})
})
/**
* Parameters used in creating the node pool. See
* gcp.container.Cluster for schema.
*/
public val nodeConfig: Output
get() = javaResource.nodeConfig().applyValue({ args0 ->
args0.let({ args0 ->
nodePoolNodeConfigToKotlin(args0)
})
})
/**
* The number of nodes per instance group. This field can be used to
* update the number of nodes per instance group but should not be used alongside `autoscaling`.
*/
public val nodeCount: Output
get() = javaResource.nodeCount().applyValue({ args0 -> args0 })
/**
* The list of zones in which the node pool's nodes should be located. Nodes must
* be in the region of their regional cluster or in the same region as their
* cluster's zone for zonal clusters. If unspecified, the cluster-level
* `node_locations` will be used.
* > Note: `node_locations` will not revert to the cluster's default set of zones
* upon being unset. You must manually reconcile the list of zones with your
* cluster.
*/
public val nodeLocations: Output>
get() = javaResource.nodeLocations().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
public val operation: Output
get() = javaResource.operation().applyValue({ args0 -> args0 })
/**
* Specifies a custom placement policy for the
* nodes.
*/
public val placementPolicy: Output?
get() = javaResource.placementPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> nodePoolPlacementPolicyToKotlin(args0) })
}).orElse(null)
})
/**
* The ID of the project in which to create the node pool. If blank,
* the provider-configured project will be used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Specifies node pool-level settings of queued provisioning.
* Structure is documented below.
*/
public val queuedProvisioning: Output?
get() = javaResource.queuedProvisioning().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> nodePoolQueuedProvisioningToKotlin(args0) })
}).orElse(null)
})
/**
* Specify node upgrade settings to change how GKE upgrades nodes.
* The maximum number of nodes upgraded simultaneously is limited to 20. Structure is documented below.
*/
public val upgradeSettings: Output
get() = javaResource.upgradeSettings().applyValue({ args0 ->
args0.let({ args0 ->
nodePoolUpgradeSettingsToKotlin(args0)
})
})
/**
* The Kubernetes version for the nodes in this pool. Note that if this field
* and `auto_upgrade` are both specified, they will fight each other for what the node version should
* be, so setting both is highly discouraged. While a fuzzy version can be specified, it's
* recommended that you specify explicit versions as the provider will see spurious diffs
* when fuzzy versions are used. See the `gcp.container.getEngineVersions` data source's
* `version_prefix` field to approximate fuzzy versions in a provider-compatible way.
*/
public val version: Output
get() = javaResource.version().applyValue({ args0 -> args0 })
}
public object NodePoolMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.container.NodePool::class == javaResource::class
override fun map(javaResource: Resource): NodePool = NodePool(
javaResource as
com.pulumi.gcp.container.NodePool,
)
}
/**
* @see [NodePool].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [NodePool].
*/
public suspend fun nodePool(name: String, block: suspend NodePoolResourceBuilder.() -> Unit): NodePool {
val builder = NodePoolResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [NodePool].
* @param name The _unique_ name of the resulting resource.
*/
public fun nodePool(name: String): NodePool {
val builder = NodePoolResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy