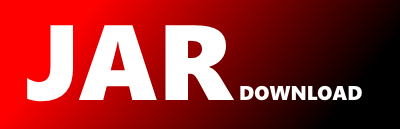
com.pulumi.gcp.dataflow.kotlin.FlexTemplateJob.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.dataflow.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [FlexTemplateJob].
*/
@PulumiTagMarker
public class FlexTemplateJobResourceBuilder internal constructor() {
public var name: String? = null
public var args: FlexTemplateJobArgs = FlexTemplateJobArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FlexTemplateJobArgsBuilder.() -> Unit) {
val builder = FlexTemplateJobArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): FlexTemplateJob {
val builtJavaResource = com.pulumi.gcp.dataflow.FlexTemplateJob(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return FlexTemplateJob(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const bigDataJob = new gcp.dataflow.FlexTemplateJob("big_data_job", {
* name: "dataflow-flextemplates-job",
* containerSpecGcsPath: "gs://my-bucket/templates/template.json",
* parameters: {
* inputSubscription: "messages",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* big_data_job = gcp.dataflow.FlexTemplateJob("big_data_job",
* name="dataflow-flextemplates-job",
* container_spec_gcs_path="gs://my-bucket/templates/template.json",
* parameters={
* "inputSubscription": "messages",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var bigDataJob = new Gcp.Dataflow.FlexTemplateJob("big_data_job", new()
* {
* Name = "dataflow-flextemplates-job",
* ContainerSpecGcsPath = "gs://my-bucket/templates/template.json",
* Parameters =
* {
* { "inputSubscription", "messages" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/dataflow"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := dataflow.NewFlexTemplateJob(ctx, "big_data_job", &dataflow.FlexTemplateJobArgs{
* Name: pulumi.String("dataflow-flextemplates-job"),
* ContainerSpecGcsPath: pulumi.String("gs://my-bucket/templates/template.json"),
* Parameters: pulumi.StringMap{
* "inputSubscription": pulumi.String("messages"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.dataflow.FlexTemplateJob;
* import com.pulumi.gcp.dataflow.FlexTemplateJobArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bigDataJob = new FlexTemplateJob("bigDataJob", FlexTemplateJobArgs.builder()
* .name("dataflow-flextemplates-job")
* .containerSpecGcsPath("gs://my-bucket/templates/template.json")
* .parameters(Map.of("inputSubscription", "messages"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bigDataJob:
* type: gcp:dataflow:FlexTemplateJob
* name: big_data_job
* properties:
* name: dataflow-flextemplates-job
* containerSpecGcsPath: gs://my-bucket/templates/template.json
* parameters:
* inputSubscription: messages
* ```
*
* ## Note on "destroy" / "apply"
* There are many types of Dataflow jobs. Some Dataflow jobs run constantly,
* getting new data from (e.g.) a GCS bucket, and outputting data continuously.
* Some jobs process a set amount of data then terminate. All jobs can fail while
* running due to programming errors or other issues. In this way, Dataflow jobs
* are different from most other provider / Google resources.
* The Dataflow resource is considered 'existing' while it is in a nonterminal
* state. If it reaches a terminal state (e.g. 'FAILED', 'COMPLETE',
* 'CANCELLED'), it will be recreated on the next 'apply'. This is as expected for
* jobs which run continuously, but may surprise users who use this resource for
* other kinds of Dataflow jobs.
* A Dataflow job which is 'destroyed' may be "cancelled" or "drained". If
* "cancelled", the job terminates - any data written remains where it is, but no
* new data will be processed. If "drained", no new data will enter the pipeline,
* but any data currently in the pipeline will finish being processed. The default
* is "cancelled", but if a user sets `on_delete` to `"drain"` in the
* configuration, you may experience a long wait for your `pulumi destroy` to
* complete.
* You can potentially short-circuit the wait by setting `skip_wait_on_job_termination`
* to `true`, but beware that unless you take active steps to ensure that the job
* `name` parameter changes between instances, the name will conflict and the launch
* of the new job will fail. One way to do this is with a
* random_id
* resource, for example:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as random from "@pulumi/random";
* const config = new pulumi.Config();
* const bigDataJobSubscriptionId = config.get("bigDataJobSubscriptionId") || "projects/myproject/subscriptions/messages";
* const bigDataJobNameSuffix = new random.RandomId("big_data_job_name_suffix", {
* byteLength: 4,
* keepers: {
* region: region,
* subscription_id: bigDataJobSubscriptionId,
* },
* });
* const bigDataJob = new gcp.dataflow.FlexTemplateJob("big_data_job", {
* name: pulumi.interpolate`dataflow-flextemplates-job-${bigDataJobNameSuffix.dec}`,
* region: region,
* containerSpecGcsPath: "gs://my-bucket/templates/template.json",
* skipWaitOnJobTermination: true,
* parameters: {
* inputSubscription: bigDataJobSubscriptionId,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_random as random
* config = pulumi.Config()
* big_data_job_subscription_id = config.get("bigDataJobSubscriptionId")
* if big_data_job_subscription_id is None:
* big_data_job_subscription_id = "projects/myproject/subscriptions/messages"
* big_data_job_name_suffix = random.RandomId("big_data_job_name_suffix",
* byte_length=4,
* keepers={
* "region": region,
* "subscription_id": big_data_job_subscription_id,
* })
* big_data_job = gcp.dataflow.FlexTemplateJob("big_data_job",
* name=big_data_job_name_suffix.dec.apply(lambda dec: f"dataflow-flextemplates-job-{dec}"),
* region=region,
* container_spec_gcs_path="gs://my-bucket/templates/template.json",
* skip_wait_on_job_termination=True,
* parameters={
* "inputSubscription": big_data_job_subscription_id,
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Random = Pulumi.Random;
* return await Deployment.RunAsync(() =>
* {
* var config = new Config();
* var bigDataJobSubscriptionId = config.Get("bigDataJobSubscriptionId") ?? "projects/myproject/subscriptions/messages";
* var bigDataJobNameSuffix = new Random.RandomId("big_data_job_name_suffix", new()
* {
* ByteLength = 4,
* Keepers =
* {
* { "region", region },
* { "subscription_id", bigDataJobSubscriptionId },
* },
* });
* var bigDataJob = new Gcp.Dataflow.FlexTemplateJob("big_data_job", new()
* {
* Name = bigDataJobNameSuffix.Dec.Apply(dec => $"dataflow-flextemplates-job-{dec}"),
* Region = region,
* ContainerSpecGcsPath = "gs://my-bucket/templates/template.json",
* SkipWaitOnJobTermination = true,
* Parameters =
* {
* { "inputSubscription", bigDataJobSubscriptionId },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/dataflow"
* "github.com/pulumi/pulumi-random/sdk/v4/go/random"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi/config"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* cfg := config.New(ctx, "")
* bigDataJobSubscriptionId := "projects/myproject/subscriptions/messages"
* if param := cfg.Get("bigDataJobSubscriptionId"); param != "" {
* bigDataJobSubscriptionId = param
* }
* bigDataJobNameSuffix, err := random.NewRandomId(ctx, "big_data_job_name_suffix", &random.RandomIdArgs{
* ByteLength: pulumi.Int(4),
* Keepers: pulumi.StringMap{
* "region": pulumi.Any(region),
* "subscription_id": pulumi.String(bigDataJobSubscriptionId),
* },
* })
* if err != nil {
* return err
* }
* _, err = dataflow.NewFlexTemplateJob(ctx, "big_data_job", &dataflow.FlexTemplateJobArgs{
* Name: bigDataJobNameSuffix.Dec.ApplyT(func(dec string) (string, error) {
* return fmt.Sprintf("dataflow-flextemplates-job-%v", dec), nil
* }).(pulumi.StringOutput),
* Region: pulumi.Any(region),
* ContainerSpecGcsPath: pulumi.String("gs://my-bucket/templates/template.json"),
* SkipWaitOnJobTermination: pulumi.Bool(true),
* Parameters: pulumi.StringMap{
* "inputSubscription": pulumi.String(bigDataJobSubscriptionId),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.random.RandomId;
* import com.pulumi.random.RandomIdArgs;
* import com.pulumi.gcp.dataflow.FlexTemplateJob;
* import com.pulumi.gcp.dataflow.FlexTemplateJobArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var config = ctx.config();
* final var bigDataJobSubscriptionId = config.get("bigDataJobSubscriptionId").orElse("projects/myproject/subscriptions/messages");
* var bigDataJobNameSuffix = new RandomId("bigDataJobNameSuffix", RandomIdArgs.builder()
* .byteLength(4)
* .keepers(Map.ofEntries(
* Map.entry("region", region),
* Map.entry("subscription_id", bigDataJobSubscriptionId)
* ))
* .build());
* var bigDataJob = new FlexTemplateJob("bigDataJob", FlexTemplateJobArgs.builder()
* .name(bigDataJobNameSuffix.dec().applyValue(dec -> String.format("dataflow-flextemplates-job-%s", dec)))
* .region(region)
* .containerSpecGcsPath("gs://my-bucket/templates/template.json")
* .skipWaitOnJobTermination(true)
* .parameters(Map.of("inputSubscription", bigDataJobSubscriptionId))
* .build());
* }
* }
* ```
* ```yaml
* configuration:
* bigDataJobSubscriptionId:
* type: string
* default: projects/myproject/subscriptions/messages
* resources:
* bigDataJobNameSuffix:
* type: random:RandomId
* name: big_data_job_name_suffix
* properties:
* byteLength: 4
* keepers:
* region: ${region}
* subscription_id: ${bigDataJobSubscriptionId}
* bigDataJob:
* type: gcp:dataflow:FlexTemplateJob
* name: big_data_job
* properties:
* name: dataflow-flextemplates-job-${bigDataJobNameSuffix.dec}
* region: ${region}
* containerSpecGcsPath: gs://my-bucket/templates/template.json
* skipWaitOnJobTermination: true
* parameters:
* inputSubscription: ${bigDataJobSubscriptionId}
* ```
*
* ## Import
* This resource does not support import.
*/
public class FlexTemplateJob internal constructor(
override val javaResource: com.pulumi.gcp.dataflow.FlexTemplateJob,
) : KotlinCustomResource(javaResource, FlexTemplateJobMapper) {
/**
* List of experiments that should be used by the job. An example value is `["enable_stackdriver_agent_metrics"]`.
*/
public val additionalExperiments: Output>
get() = javaResource.additionalExperiments().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The algorithm to use for autoscaling.
*/
public val autoscalingAlgorithm: Output
get() = javaResource.autoscalingAlgorithm().applyValue({ args0 -> args0 })
/**
* The GCS path to the Dataflow job Flex
* Template.
* - - -
*/
public val containerSpecGcsPath: Output
get() = javaResource.containerSpecGcsPath().applyValue({ args0 -> args0 })
public val effectiveLabels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy