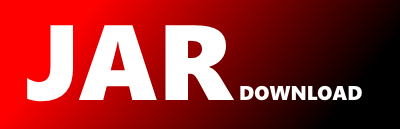
com.pulumi.gcp.kms.kotlin.CryptoKey.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.kms.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyKeyAccessJustificationsPolicy
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyPrimary
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyVersionTemplate
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyKeyAccessJustificationsPolicy.Companion.toKotlin as cryptoKeyKeyAccessJustificationsPolicyToKotlin
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyPrimary.Companion.toKotlin as cryptoKeyPrimaryToKotlin
import com.pulumi.gcp.kms.kotlin.outputs.CryptoKeyVersionTemplate.Companion.toKotlin as cryptoKeyVersionTemplateToKotlin
/**
* Builder for [CryptoKey].
*/
@PulumiTagMarker
public class CryptoKeyResourceBuilder internal constructor() {
public var name: String? = null
public var args: CryptoKeyArgs = CryptoKeyArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend CryptoKeyArgsBuilder.() -> Unit) {
val builder = CryptoKeyArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): CryptoKey {
val builtJavaResource = com.pulumi.gcp.kms.CryptoKey(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return CryptoKey(builtJavaResource)
}
}
/**
* A `CryptoKey` represents a logical key that can be used for cryptographic operations.
* > **Note:** CryptoKeys cannot be deleted from Google Cloud Platform.
* Destroying a provider-managed CryptoKey will remove it from state
* and delete all CryptoKeyVersions, rendering the key unusable, but *will
* not delete the resource from the project.* When the provider destroys these keys,
* any data previously encrypted with these keys will be irrecoverable.
* For this reason, it is strongly recommended that you use Pulumi's [protect resource option](https://www.pulumi.com/docs/concepts/options/protect/).
* To get more information about CryptoKey, see:
* * [API documentation](https://cloud.google.com/kms/docs/reference/rest/v1/projects.locations.keyRings.cryptoKeys)
* * How-to Guides
* * [Creating a key](https://cloud.google.com/kms/docs/creating-keys#create_a_key)
* ## Example Usage
* ### Kms Crypto Key Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const keyring = new gcp.kms.KeyRing("keyring", {
* name: "keyring-example",
* location: "global",
* });
* const example_key = new gcp.kms.CryptoKey("example-key", {
* name: "crypto-key-example",
* keyRing: keyring.id,
* rotationPeriod: "7776000s",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* keyring = gcp.kms.KeyRing("keyring",
* name="keyring-example",
* location="global")
* example_key = gcp.kms.CryptoKey("example-key",
* name="crypto-key-example",
* key_ring=keyring.id,
* rotation_period="7776000s")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var keyring = new Gcp.Kms.KeyRing("keyring", new()
* {
* Name = "keyring-example",
* Location = "global",
* });
* var example_key = new Gcp.Kms.CryptoKey("example-key", new()
* {
* Name = "crypto-key-example",
* KeyRing = keyring.Id,
* RotationPeriod = "7776000s",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* keyring, err := kms.NewKeyRing(ctx, "keyring", &kms.KeyRingArgs{
* Name: pulumi.String("keyring-example"),
* Location: pulumi.String("global"),
* })
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKey(ctx, "example-key", &kms.CryptoKeyArgs{
* Name: pulumi.String("crypto-key-example"),
* KeyRing: keyring.ID(),
* RotationPeriod: pulumi.String("7776000s"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.kms.KeyRing;
* import com.pulumi.gcp.kms.KeyRingArgs;
* import com.pulumi.gcp.kms.CryptoKey;
* import com.pulumi.gcp.kms.CryptoKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var keyring = new KeyRing("keyring", KeyRingArgs.builder()
* .name("keyring-example")
* .location("global")
* .build());
* var example_key = new CryptoKey("example-key", CryptoKeyArgs.builder()
* .name("crypto-key-example")
* .keyRing(keyring.id())
* .rotationPeriod("7776000s")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* keyring:
* type: gcp:kms:KeyRing
* properties:
* name: keyring-example
* location: global
* example-key:
* type: gcp:kms:CryptoKey
* properties:
* name: crypto-key-example
* keyRing: ${keyring.id}
* rotationPeriod: 7776000s
* ```
*
* ### Kms Crypto Key Asymmetric Sign
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const keyring = new gcp.kms.KeyRing("keyring", {
* name: "keyring-example",
* location: "global",
* });
* const example_asymmetric_sign_key = new gcp.kms.CryptoKey("example-asymmetric-sign-key", {
* name: "crypto-key-example",
* keyRing: keyring.id,
* purpose: "ASYMMETRIC_SIGN",
* versionTemplate: {
* algorithm: "EC_SIGN_P384_SHA384",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* keyring = gcp.kms.KeyRing("keyring",
* name="keyring-example",
* location="global")
* example_asymmetric_sign_key = gcp.kms.CryptoKey("example-asymmetric-sign-key",
* name="crypto-key-example",
* key_ring=keyring.id,
* purpose="ASYMMETRIC_SIGN",
* version_template={
* "algorithm": "EC_SIGN_P384_SHA384",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var keyring = new Gcp.Kms.KeyRing("keyring", new()
* {
* Name = "keyring-example",
* Location = "global",
* });
* var example_asymmetric_sign_key = new Gcp.Kms.CryptoKey("example-asymmetric-sign-key", new()
* {
* Name = "crypto-key-example",
* KeyRing = keyring.Id,
* Purpose = "ASYMMETRIC_SIGN",
* VersionTemplate = new Gcp.Kms.Inputs.CryptoKeyVersionTemplateArgs
* {
* Algorithm = "EC_SIGN_P384_SHA384",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* keyring, err := kms.NewKeyRing(ctx, "keyring", &kms.KeyRingArgs{
* Name: pulumi.String("keyring-example"),
* Location: pulumi.String("global"),
* })
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKey(ctx, "example-asymmetric-sign-key", &kms.CryptoKeyArgs{
* Name: pulumi.String("crypto-key-example"),
* KeyRing: keyring.ID(),
* Purpose: pulumi.String("ASYMMETRIC_SIGN"),
* VersionTemplate: &kms.CryptoKeyVersionTemplateArgs{
* Algorithm: pulumi.String("EC_SIGN_P384_SHA384"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.kms.KeyRing;
* import com.pulumi.gcp.kms.KeyRingArgs;
* import com.pulumi.gcp.kms.CryptoKey;
* import com.pulumi.gcp.kms.CryptoKeyArgs;
* import com.pulumi.gcp.kms.inputs.CryptoKeyVersionTemplateArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var keyring = new KeyRing("keyring", KeyRingArgs.builder()
* .name("keyring-example")
* .location("global")
* .build());
* var example_asymmetric_sign_key = new CryptoKey("example-asymmetric-sign-key", CryptoKeyArgs.builder()
* .name("crypto-key-example")
* .keyRing(keyring.id())
* .purpose("ASYMMETRIC_SIGN")
* .versionTemplate(CryptoKeyVersionTemplateArgs.builder()
* .algorithm("EC_SIGN_P384_SHA384")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* keyring:
* type: gcp:kms:KeyRing
* properties:
* name: keyring-example
* location: global
* example-asymmetric-sign-key:
* type: gcp:kms:CryptoKey
* properties:
* name: crypto-key-example
* keyRing: ${keyring.id}
* purpose: ASYMMETRIC_SIGN
* versionTemplate:
* algorithm: EC_SIGN_P384_SHA384
* ```
*
* ## Import
* CryptoKey can be imported using any of these accepted formats:
* * `{{key_ring}}/cryptoKeys/{{name}}`
* * `{{key_ring}}/{{name}}`
* When using the `pulumi import` command, CryptoKey can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:kms/cryptoKey:CryptoKey default {{key_ring}}/cryptoKeys/{{name}}
* ```
* ```sh
* $ pulumi import gcp:kms/cryptoKey:CryptoKey default {{key_ring}}/{{name}}
* ```
*/
public class CryptoKey internal constructor(
override val javaResource: com.pulumi.gcp.kms.CryptoKey,
) : KotlinCustomResource(javaResource, CryptoKeyMapper) {
/**
* The resource name of the backend environment associated with all CryptoKeyVersions within this CryptoKey.
* The resource name is in the format "projects/*/locations/*/ekmConnections/*" and only applies to "EXTERNAL_VPC" keys.
* */*/*/
*/
public val cryptoKeyBackend: Output
get() = javaResource.cryptoKeyBackend().applyValue({ args0 -> args0 })
/**
* The period of time that versions of this key spend in the DESTROY_SCHEDULED state before transitioning to DESTROYED.
* If not specified at creation time, the default duration is 30 days.
*/
public val destroyScheduledDuration: Output
get() = javaResource.destroyScheduledDuration().applyValue({ args0 -> args0 })
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy