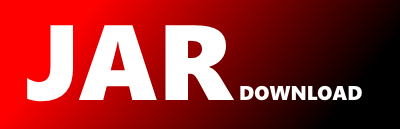
com.pulumi.gcp.securitycenter.kotlin.FolderNotificationConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.securitycenter.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.securitycenter.FolderNotificationConfigArgs.builder
import com.pulumi.gcp.securitycenter.kotlin.inputs.FolderNotificationConfigStreamingConfigArgs
import com.pulumi.gcp.securitycenter.kotlin.inputs.FolderNotificationConfigStreamingConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A Cloud Security Command Center (Cloud SCC) notification configs. A
* notification config is a Cloud SCC resource that contains the
* configuration to send notifications for create/update events of
* findings, assets and etc.
* > **Note:** In order to use Cloud SCC resources, your organization must be enrolled
* in [SCC Standard/Premium](https://cloud.google.com/security-command-center/docs/quickstart-security-command-center).
* Without doing so, you may run into errors during resource creation.
* To get more information about FolderNotificationConfig, see:
* * [API documentation](https://cloud.google.com/security-command-center/docs/reference/rest/v1/folders.notificationConfigs)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/security-command-center/docs)
* ## Example Usage
* ### Scc Folder Notification Config Basic
*
* ```yaml
* resources:
* folder:
* type: gcp:organizations:Folder
* properties:
* parent: organizations/123456789
* displayName: folder-name
* sccFolderNotificationConfig:
* type: gcp:pubsub:Topic
* name: scc_folder_notification_config
* properties:
* name: my-topic
* customNotificationConfig:
* type: gcp:securitycenter:FolderNotificationConfig
* name: custom_notification_config
* properties:
* configId: my-config
* folder: ${folder.folderId}
* location: global
* description: My custom Cloud Security Command Center Finding Notification Configuration
* pubsubTopic: ${sccFolderNotificationConfig.id}
* streamingConfig:
* filter: category = "OPEN_FIREWALL" AND state = "ACTIVE"
* ```
*
* ## Import
* FolderNotificationConfig can be imported using any of these accepted formats:
* * `folders/{{folder}}/notificationConfigs/{{config_id}}`
* * `{{folder}}/{{config_id}}`
* When using the `pulumi import` command, FolderNotificationConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:securitycenter/folderNotificationConfig:FolderNotificationConfig default folders/{{folder}}/notificationConfigs/{{config_id}}
* ```
* ```sh
* $ pulumi import gcp:securitycenter/folderNotificationConfig:FolderNotificationConfig default {{folder}}/{{config_id}}
* ```
* @property configId This must be unique within the organization.
* @property description The description of the notification config (max of 1024 characters).
* @property folder Numerical ID of the parent folder.
* @property pubsubTopic The Pub/Sub topic to send notifications to. Its format is
* "projects/[project_id]/topics/[topic]".
* @property streamingConfig The config for triggering streaming-based notifications.
* Structure is documented below.
*/
public data class FolderNotificationConfigArgs(
public val configId: Output? = null,
public val description: Output? = null,
public val folder: Output? = null,
public val pubsubTopic: Output? = null,
public val streamingConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.securitycenter.FolderNotificationConfigArgs =
com.pulumi.gcp.securitycenter.FolderNotificationConfigArgs.builder()
.configId(configId?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.folder(folder?.applyValue({ args0 -> args0 }))
.pubsubTopic(pubsubTopic?.applyValue({ args0 -> args0 }))
.streamingConfig(
streamingConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [FolderNotificationConfigArgs].
*/
@PulumiTagMarker
public class FolderNotificationConfigArgsBuilder internal constructor() {
private var configId: Output? = null
private var description: Output? = null
private var folder: Output? = null
private var pubsubTopic: Output? = null
private var streamingConfig: Output? = null
/**
* @param value This must be unique within the organization.
*/
@JvmName("ksrxgqjmjlhaupdp")
public suspend fun configId(`value`: Output) {
this.configId = value
}
/**
* @param value The description of the notification config (max of 1024 characters).
*/
@JvmName("aeudjgifalolsqxy")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Numerical ID of the parent folder.
*/
@JvmName("issjloehvnjuoesy")
public suspend fun folder(`value`: Output) {
this.folder = value
}
/**
* @param value The Pub/Sub topic to send notifications to. Its format is
* "projects/[project_id]/topics/[topic]".
*/
@JvmName("ipmhbiipxxiowolt")
public suspend fun pubsubTopic(`value`: Output) {
this.pubsubTopic = value
}
/**
* @param value The config for triggering streaming-based notifications.
* Structure is documented below.
*/
@JvmName("xqmgtkkrhpuosyqj")
public suspend fun streamingConfig(`value`: Output) {
this.streamingConfig = value
}
/**
* @param value This must be unique within the organization.
*/
@JvmName("pgfkiehqnekixsoy")
public suspend fun configId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configId = mapped
}
/**
* @param value The description of the notification config (max of 1024 characters).
*/
@JvmName("skttxaefqreqdbii")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Numerical ID of the parent folder.
*/
@JvmName("moiuxexjucuhdemh")
public suspend fun folder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.folder = mapped
}
/**
* @param value The Pub/Sub topic to send notifications to. Its format is
* "projects/[project_id]/topics/[topic]".
*/
@JvmName("buecpcgfjlgrexca")
public suspend fun pubsubTopic(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pubsubTopic = mapped
}
/**
* @param value The config for triggering streaming-based notifications.
* Structure is documented below.
*/
@JvmName("ljbfcruycgcrmopb")
public suspend fun streamingConfig(`value`: FolderNotificationConfigStreamingConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.streamingConfig = mapped
}
/**
* @param argument The config for triggering streaming-based notifications.
* Structure is documented below.
*/
@JvmName("mipbfmqdegbgopxh")
public suspend fun streamingConfig(argument: suspend FolderNotificationConfigStreamingConfigArgsBuilder.() -> Unit) {
val toBeMapped = FolderNotificationConfigStreamingConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.streamingConfig = mapped
}
internal fun build(): FolderNotificationConfigArgs = FolderNotificationConfigArgs(
configId = configId,
description = description,
folder = folder,
pubsubTopic = pubsubTopic,
streamingConfig = streamingConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy