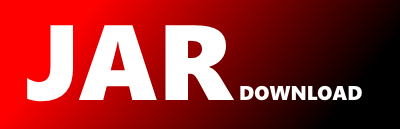
com.pulumi.gcp.storage.kotlin.StorageFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.storage.kotlin
import com.pulumi.gcp.storage.StorageFunctions.getBucketIamPolicyPlain
import com.pulumi.gcp.storage.StorageFunctions.getBucketObjectContentPlain
import com.pulumi.gcp.storage.StorageFunctions.getBucketObjectPlain
import com.pulumi.gcp.storage.StorageFunctions.getBucketObjectsPlain
import com.pulumi.gcp.storage.StorageFunctions.getBucketPlain
import com.pulumi.gcp.storage.StorageFunctions.getBucketsPlain
import com.pulumi.gcp.storage.StorageFunctions.getManagedFolderIamPolicyPlain
import com.pulumi.gcp.storage.StorageFunctions.getObjectSignedUrlPlain
import com.pulumi.gcp.storage.StorageFunctions.getProjectServiceAccountPlain
import com.pulumi.gcp.storage.StorageFunctions.getTransferProjectServiceAccountPlain
import com.pulumi.gcp.storage.StorageFunctions.getTransferProjectServieAccountPlain
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketIamPolicyPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketIamPolicyPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectContentPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectContentPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectsPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectsPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketsPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetBucketsPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetManagedFolderIamPolicyPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetManagedFolderIamPolicyPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetObjectSignedUrlPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetObjectSignedUrlPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetProjectServiceAccountPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetProjectServiceAccountPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServiceAccountPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServiceAccountPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServieAccountPlainArgs
import com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServieAccountPlainArgsBuilder
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketIamPolicyResult
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectContentResult
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectResult
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectsResult
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketResult
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketsResult
import com.pulumi.gcp.storage.kotlin.outputs.GetManagedFolderIamPolicyResult
import com.pulumi.gcp.storage.kotlin.outputs.GetObjectSignedUrlResult
import com.pulumi.gcp.storage.kotlin.outputs.GetProjectServiceAccountResult
import com.pulumi.gcp.storage.kotlin.outputs.GetTransferProjectServiceAccountResult
import com.pulumi.gcp.storage.kotlin.outputs.GetTransferProjectServieAccountResult
import kotlinx.coroutines.future.await
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketIamPolicyResult.Companion.toKotlin as getBucketIamPolicyResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectContentResult.Companion.toKotlin as getBucketObjectContentResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectResult.Companion.toKotlin as getBucketObjectResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketObjectsResult.Companion.toKotlin as getBucketObjectsResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketResult.Companion.toKotlin as getBucketResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetBucketsResult.Companion.toKotlin as getBucketsResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetManagedFolderIamPolicyResult.Companion.toKotlin as getManagedFolderIamPolicyResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetObjectSignedUrlResult.Companion.toKotlin as getObjectSignedUrlResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetProjectServiceAccountResult.Companion.toKotlin as getProjectServiceAccountResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetTransferProjectServiceAccountResult.Companion.toKotlin as getTransferProjectServiceAccountResultToKotlin
import com.pulumi.gcp.storage.kotlin.outputs.GetTransferProjectServieAccountResult.Companion.toKotlin as getTransferProjectServieAccountResultToKotlin
public object StorageFunctions {
/**
* Gets an existing bucket in Google Cloud Storage service (GCS).
* See [the official documentation](https://cloud.google.com/storage/docs/key-terms#buckets)
* and
* [API](https://cloud.google.com/storage/docs/json_api/v1/buckets).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const my-bucket = gcp.storage.getBucket({
* name: "my-bucket",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_bucket = gcp.storage.get_bucket(name="my-bucket")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var my_bucket = Gcp.Storage.GetBucket.Invoke(new()
* {
* Name = "my-bucket",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.LookupBucket(ctx, &storage.LookupBucketArgs{
* Name: "my-bucket",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var my-bucket = StorageFunctions.getBucket(GetBucketArgs.builder()
* .name("my-bucket")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* my-bucket:
* fn::invoke:
* Function: gcp:storage:getBucket
* Arguments:
* name: my-bucket
* ```
*
* @param argument A collection of arguments for invoking getBucket.
* @return A collection of values returned by getBucket.
*/
public suspend fun getBucket(argument: GetBucketPlainArgs): GetBucketResult =
getBucketResultToKotlin(getBucketPlain(argument.toJava()).await())
/**
* @see [getBucket].
* @param name The name of the bucket.
* @param project The ID of the project in which the resource belongs. If it is not provided, the provider project is used. If no value is supplied in the configuration or through provider defaults then the data source will use the Compute API to find the project id that corresponds to the project number returned from the Storage API. Supplying a value for `project` doesn't influence retrieving data about the bucket but it can be used to prevent use of the Compute API. If you do provide a `project` value ensure that it is the correct value for that bucket; the data source will not check that the project id and project number match.
* @return A collection of values returned by getBucket.
*/
public suspend fun getBucket(name: String, project: String? = null): GetBucketResult {
val argument = GetBucketPlainArgs(
name = name,
project = project,
)
return getBucketResultToKotlin(getBucketPlain(argument.toJava()).await())
}
/**
* @see [getBucket].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketPlainArgs].
* @return A collection of values returned by getBucket.
*/
public suspend fun getBucket(argument: suspend GetBucketPlainArgsBuilder.() -> Unit): GetBucketResult {
val builder = GetBucketPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketResultToKotlin(getBucketPlain(builtArgument.toJava()).await())
}
/**
* Retrieves the current IAM policy data for bucket
* ## example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const policy = gcp.storage.getBucketIamPolicy({
* bucket: _default.name,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* policy = gcp.storage.get_bucket_iam_policy(bucket=default["name"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var policy = Gcp.Storage.GetBucketIamPolicy.Invoke(new()
* {
* Bucket = @default.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.GetBucketIamPolicy(ctx, &storage.GetBucketIamPolicyArgs{
* Bucket: _default.Name,
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketIamPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var policy = StorageFunctions.getBucketIamPolicy(GetBucketIamPolicyArgs.builder()
* .bucket(default_.name())
* .build());
* }
* }
* ```
* ```yaml
* variables:
* policy:
* fn::invoke:
* Function: gcp:storage:getBucketIamPolicy
* Arguments:
* bucket: ${default.name}
* ```
*
* @param argument A collection of arguments for invoking getBucketIamPolicy.
* @return A collection of values returned by getBucketIamPolicy.
*/
public suspend fun getBucketIamPolicy(argument: GetBucketIamPolicyPlainArgs): GetBucketIamPolicyResult =
getBucketIamPolicyResultToKotlin(getBucketIamPolicyPlain(argument.toJava()).await())
/**
* @see [getBucketIamPolicy].
* @param bucket Used to find the parent resource to bind the IAM policy to
* @return A collection of values returned by getBucketIamPolicy.
*/
public suspend fun getBucketIamPolicy(bucket: String): GetBucketIamPolicyResult {
val argument = GetBucketIamPolicyPlainArgs(
bucket = bucket,
)
return getBucketIamPolicyResultToKotlin(getBucketIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getBucketIamPolicy].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketIamPolicyPlainArgs].
* @return A collection of values returned by getBucketIamPolicy.
*/
public suspend fun getBucketIamPolicy(argument: suspend GetBucketIamPolicyPlainArgsBuilder.() -> Unit): GetBucketIamPolicyResult {
val builder = GetBucketIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketIamPolicyResultToKotlin(getBucketIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* Gets an existing object inside an existing bucket in Google Cloud Storage service (GCS).
* See [the official documentation](https://cloud.google.com/storage/docs/key-terms#objects)
* and
* [API](https://cloud.google.com/storage/docs/json_api/v1/objects).
* ## Example Usage
* Example picture stored within a folder.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const picture = gcp.storage.getBucketObject({
* name: "folder/butterfly01.jpg",
* bucket: "image-store",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* picture = gcp.storage.get_bucket_object(name="folder/butterfly01.jpg",
* bucket="image-store")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var picture = Gcp.Storage.GetBucketObject.Invoke(new()
* {
* Name = "folder/butterfly01.jpg",
* Bucket = "image-store",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.LookupBucketObject(ctx, &storage.LookupBucketObjectArgs{
* Name: pulumi.StringRef("folder/butterfly01.jpg"),
* Bucket: pulumi.StringRef("image-store"),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketObjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var picture = StorageFunctions.getBucketObject(GetBucketObjectArgs.builder()
* .name("folder/butterfly01.jpg")
* .bucket("image-store")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* picture:
* fn::invoke:
* Function: gcp:storage:getBucketObject
* Arguments:
* name: folder/butterfly01.jpg
* bucket: image-store
* ```
*
* @param argument A collection of arguments for invoking getBucketObject.
* @return A collection of values returned by getBucketObject.
*/
public suspend fun getBucketObject(argument: GetBucketObjectPlainArgs): GetBucketObjectResult =
getBucketObjectResultToKotlin(getBucketObjectPlain(argument.toJava()).await())
/**
* @see [getBucketObject].
* @param bucket The name of the containing bucket.
* @param name The name of the object.
* @return A collection of values returned by getBucketObject.
*/
public suspend fun getBucketObject(bucket: String? = null, name: String? = null): GetBucketObjectResult {
val argument = GetBucketObjectPlainArgs(
bucket = bucket,
name = name,
)
return getBucketObjectResultToKotlin(getBucketObjectPlain(argument.toJava()).await())
}
/**
* @see [getBucketObject].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectPlainArgs].
* @return A collection of values returned by getBucketObject.
*/
public suspend fun getBucketObject(argument: suspend GetBucketObjectPlainArgsBuilder.() -> Unit): GetBucketObjectResult {
val builder = GetBucketObjectPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketObjectResultToKotlin(getBucketObjectPlain(builtArgument.toJava()).await())
}
/**
* Gets an existing object content inside an existing bucket in Google Cloud Storage service (GCS).
* See [the official documentation](https://cloud.google.com/storage/docs/key-terms#objects)
* and
* [API](https://cloud.google.com/storage/docs/json_api/v1/objects).
* > **Warning:** The object content will be saved in the state, and visiable to everyone who has access to the state file.
* ## Example Usage
* Example file object stored within a folder.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const key = gcp.storage.getBucketObjectContent({
* name: "encryptedkey",
* bucket: "keystore",
* });
* export const encrypted = key.then(key => key.content);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* key = gcp.storage.get_bucket_object_content(name="encryptedkey",
* bucket="keystore")
* pulumi.export("encrypted", key.content)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var key = Gcp.Storage.GetBucketObjectContent.Invoke(new()
* {
* Name = "encryptedkey",
* Bucket = "keystore",
* });
* return new Dictionary
* {
* ["encrypted"] = key.Apply(getBucketObjectContentResult => getBucketObjectContentResult.Content),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* key, err := storage.GetBucketObjectContent(ctx, &storage.GetBucketObjectContentArgs{
* Name: "encryptedkey",
* Bucket: "keystore",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("encrypted", key.Content)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketObjectContentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var key = StorageFunctions.getBucketObjectContent(GetBucketObjectContentArgs.builder()
* .name("encryptedkey")
* .bucket("keystore")
* .build());
* ctx.export("encrypted", key.applyValue(getBucketObjectContentResult -> getBucketObjectContentResult.content()));
* }
* }
* ```
* ```yaml
* variables:
* key:
* fn::invoke:
* Function: gcp:storage:getBucketObjectContent
* Arguments:
* name: encryptedkey
* bucket: keystore
* outputs:
* encrypted: ${key.content}
* ```
*
* @param argument A collection of arguments for invoking getBucketObjectContent.
* @return A collection of values returned by getBucketObjectContent.
*/
public suspend fun getBucketObjectContent(argument: GetBucketObjectContentPlainArgs): GetBucketObjectContentResult =
getBucketObjectContentResultToKotlin(getBucketObjectContentPlain(argument.toJava()).await())
/**
* @see [getBucketObjectContent].
* @param bucket The name of the containing bucket.
* @param content (Computed) [Content-Language](https://tools.ietf.org/html/rfc7231#section-3.1.3.2) of the object content.
* @param name The name of the object.
* @return A collection of values returned by getBucketObjectContent.
*/
public suspend fun getBucketObjectContent(
bucket: String,
content: String? = null,
name: String,
): GetBucketObjectContentResult {
val argument = GetBucketObjectContentPlainArgs(
bucket = bucket,
content = content,
name = name,
)
return getBucketObjectContentResultToKotlin(getBucketObjectContentPlain(argument.toJava()).await())
}
/**
* @see [getBucketObjectContent].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectContentPlainArgs].
* @return A collection of values returned by getBucketObjectContent.
*/
public suspend fun getBucketObjectContent(argument: suspend GetBucketObjectContentPlainArgsBuilder.() -> Unit): GetBucketObjectContentResult {
val builder = GetBucketObjectContentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketObjectContentResultToKotlin(getBucketObjectContentPlain(builtArgument.toJava()).await())
}
/**
* Gets existing objects inside an existing bucket in Google Cloud Storage service (GCS).
* See [the official documentation](https://cloud.google.com/storage/docs/key-terms#objects)
* and [API](https://cloud.google.com/storage/docs/json_api/v1/objects/list).
* ## Example Usage
* Example files stored within a bucket.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const files = gcp.storage.getBucketObjects({
* bucket: "file-store",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* files = gcp.storage.get_bucket_objects(bucket="file-store")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var files = Gcp.Storage.GetBucketObjects.Invoke(new()
* {
* Bucket = "file-store",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.GetBucketObjects(ctx, &storage.GetBucketObjectsArgs{
* Bucket: "file-store",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketObjectsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var files = StorageFunctions.getBucketObjects(GetBucketObjectsArgs.builder()
* .bucket("file-store")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* files:
* fn::invoke:
* Function: gcp:storage:getBucketObjects
* Arguments:
* bucket: file-store
* ```
*
* @param argument A collection of arguments for invoking getBucketObjects.
* @return A collection of values returned by getBucketObjects.
*/
public suspend fun getBucketObjects(argument: GetBucketObjectsPlainArgs): GetBucketObjectsResult =
getBucketObjectsResultToKotlin(getBucketObjectsPlain(argument.toJava()).await())
/**
* @see [getBucketObjects].
* @param bucket The name of the containing bucket.
* @param matchGlob A glob pattern used to filter results (for example, `foo*bar`).
* @param prefix Filter results to include only objects whose names begin with this prefix.
* @return A collection of values returned by getBucketObjects.
*/
public suspend fun getBucketObjects(
bucket: String,
matchGlob: String? = null,
prefix: String? = null,
): GetBucketObjectsResult {
val argument = GetBucketObjectsPlainArgs(
bucket = bucket,
matchGlob = matchGlob,
prefix = prefix,
)
return getBucketObjectsResultToKotlin(getBucketObjectsPlain(argument.toJava()).await())
}
/**
* @see [getBucketObjects].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketObjectsPlainArgs].
* @return A collection of values returned by getBucketObjects.
*/
public suspend fun getBucketObjects(argument: suspend GetBucketObjectsPlainArgsBuilder.() -> Unit): GetBucketObjectsResult {
val builder = GetBucketObjectsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketObjectsResultToKotlin(getBucketObjectsPlain(builtArgument.toJava()).await())
}
/**
* Gets a list of existing GCS buckets.
* See [the official documentation](https://cloud.google.com/storage/docs/introduction)
* and [API](https://cloud.google.com/storage/docs/json_api/v1/buckets/list).
* ## Example Usage
* Example GCS buckets.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = gcp.storage.getBuckets({
* project: "example-project",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.storage.get_buckets(project="example-project")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = Gcp.Storage.GetBuckets.Invoke(new()
* {
* Project = "example-project",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.GetBuckets(ctx, &storage.GetBucketsArgs{
* Project: pulumi.StringRef("example-project"),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetBucketsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = StorageFunctions.getBuckets(GetBucketsArgs.builder()
* .project("example-project")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: gcp:storage:getBuckets
* Arguments:
* project: example-project
* ```
*
* @param argument A collection of arguments for invoking getBuckets.
* @return A collection of values returned by getBuckets.
*/
public suspend fun getBuckets(argument: GetBucketsPlainArgs): GetBucketsResult =
getBucketsResultToKotlin(getBucketsPlain(argument.toJava()).await())
/**
* @see [getBuckets].
* @param prefix Filter results to buckets whose names begin with this prefix.
* @param project The ID of the project. If it is not provided, the provider project is used.
* @return A collection of values returned by getBuckets.
*/
public suspend fun getBuckets(prefix: String? = null, project: String? = null): GetBucketsResult {
val argument = GetBucketsPlainArgs(
prefix = prefix,
project = project,
)
return getBucketsResultToKotlin(getBucketsPlain(argument.toJava()).await())
}
/**
* @see [getBuckets].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetBucketsPlainArgs].
* @return A collection of values returned by getBuckets.
*/
public suspend fun getBuckets(argument: suspend GetBucketsPlainArgsBuilder.() -> Unit): GetBucketsResult {
val builder = GetBucketsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getBucketsResultToKotlin(getBucketsPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getManagedFolderIamPolicy.
* @return A collection of values returned by getManagedFolderIamPolicy.
*/
public suspend fun getManagedFolderIamPolicy(argument: GetManagedFolderIamPolicyPlainArgs): GetManagedFolderIamPolicyResult =
getManagedFolderIamPolicyResultToKotlin(getManagedFolderIamPolicyPlain(argument.toJava()).await())
/**
* @see [getManagedFolderIamPolicy].
* @param bucket
* @param managedFolder
* @return A collection of values returned by getManagedFolderIamPolicy.
*/
public suspend fun getManagedFolderIamPolicy(bucket: String, managedFolder: String): GetManagedFolderIamPolicyResult {
val argument = GetManagedFolderIamPolicyPlainArgs(
bucket = bucket,
managedFolder = managedFolder,
)
return getManagedFolderIamPolicyResultToKotlin(getManagedFolderIamPolicyPlain(argument.toJava()).await())
}
/**
* @see [getManagedFolderIamPolicy].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetManagedFolderIamPolicyPlainArgs].
* @return A collection of values returned by getManagedFolderIamPolicy.
*/
public suspend fun getManagedFolderIamPolicy(argument: suspend GetManagedFolderIamPolicyPlainArgsBuilder.() -> Unit): GetManagedFolderIamPolicyResult {
val builder = GetManagedFolderIamPolicyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getManagedFolderIamPolicyResultToKotlin(getManagedFolderIamPolicyPlain(builtArgument.toJava()).await())
}
/**
* The Google Cloud storage signed URL data source generates a signed URL for a given storage object. Signed URLs provide a way to give time-limited read or write access to anyone in possession of the URL, regardless of whether they have a Google account.
* For more info about signed URL's is available [here](https://cloud.google.com/storage/docs/access-control/signed-urls).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const artifact = gcp.storage.getObjectSignedUrl({
* bucket: "install_binaries",
* path: "path/to/install_file.bin",
* });
* const vm = new gcp.compute.Instance("vm", {name: "vm"});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* artifact = gcp.storage.get_object_signed_url(bucket="install_binaries",
* path="path/to/install_file.bin")
* vm = gcp.compute.Instance("vm", name="vm")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var artifact = Gcp.Storage.GetObjectSignedUrl.Invoke(new()
* {
* Bucket = "install_binaries",
* Path = "path/to/install_file.bin",
* });
* var vm = new Gcp.Compute.Instance("vm", new()
* {
* Name = "vm",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.GetObjectSignedUrl(ctx, &storage.GetObjectSignedUrlArgs{
* Bucket: "install_binaries",
* Path: "path/to/install_file.bin",
* }, nil)
* if err != nil {
* return err
* }
* _, err = compute.NewInstance(ctx, "vm", &compute.InstanceArgs{
* Name: pulumi.String("vm"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetObjectSignedUrlArgs;
* import com.pulumi.gcp.compute.Instance;
* import com.pulumi.gcp.compute.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var artifact = StorageFunctions.getObjectSignedUrl(GetObjectSignedUrlArgs.builder()
* .bucket("install_binaries")
* .path("path/to/install_file.bin")
* .build());
* var vm = new Instance("vm", InstanceArgs.builder()
* .name("vm")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* vm:
* type: gcp:compute:Instance
* properties:
* name: vm
* variables:
* artifact:
* fn::invoke:
* Function: gcp:storage:getObjectSignedUrl
* Arguments:
* bucket: install_binaries
* path: path/to/install_file.bin
* ```
*
* ## Full Example
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* import * as std from "@pulumi/std";
* const getUrl = std.file({
* input: "path/to/credentials.json",
* }).then(invoke => gcp.storage.getObjectSignedUrl({
* bucket: "fried_chicken",
* path: "path/to/file",
* contentMd5: "pRviqwS4c4OTJRTe03FD1w==",
* contentType: "text/plain",
* duration: "2d",
* credentials: invoke.result,
* extensionHeaders: {
* "x-goog-if-generation-match": "1",
* },
* }));
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* import pulumi_std as std
* get_url = gcp.storage.get_object_signed_url(bucket="fried_chicken",
* path="path/to/file",
* content_md5="pRviqwS4c4OTJRTe03FD1w==",
* content_type="text/plain",
* duration="2d",
* credentials=std.file(input="path/to/credentials.json").result,
* extension_headers={
* "x-goog-if-generation-match": "1",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var getUrl = Gcp.Storage.GetObjectSignedUrl.Invoke(new()
* {
* Bucket = "fried_chicken",
* Path = "path/to/file",
* ContentMd5 = "pRviqwS4c4OTJRTe03FD1w==",
* ContentType = "text/plain",
* Duration = "2d",
* Credentials = Std.File.Invoke(new()
* {
* Input = "path/to/credentials.json",
* }).Result,
* ExtensionHeaders =
* {
* { "x-goog-if-generation-match", "1" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := storage.GetObjectSignedUrl(ctx, &storage.GetObjectSignedUrlArgs{
* Bucket: "fried_chicken",
* Path: "path/to/file",
* ContentMd5: pulumi.StringRef("pRviqwS4c4OTJRTe03FD1w=="),
* ContentType: pulumi.StringRef("text/plain"),
* Duration: pulumi.StringRef("2d"),
* Credentials: pulumi.StringRef(std.File(ctx, &std.FileArgs{
* Input: "path/to/credentials.json",
* }, nil).Result),
* ExtensionHeaders: map[string]interface{}{
* "x-goog-if-generation-match": "1",
* },
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetObjectSignedUrlArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var getUrl = StorageFunctions.getObjectSignedUrl(GetObjectSignedUrlArgs.builder()
* .bucket("fried_chicken")
* .path("path/to/file")
* .contentMd5("pRviqwS4c4OTJRTe03FD1w==")
* .contentType("text/plain")
* .duration("2d")
* .credentials(StdFunctions.file(FileArgs.builder()
* .input("path/to/credentials.json")
* .build()).result())
* .extensionHeaders(Map.of("x-goog-if-generation-match", 1))
* .build());
* }
* }
* ```
* ```yaml
* variables:
* getUrl:
* fn::invoke:
* Function: gcp:storage:getObjectSignedUrl
* Arguments:
* bucket: fried_chicken
* path: path/to/file
* contentMd5: pRviqwS4c4OTJRTe03FD1w==
* contentType: text/plain
* duration: 2d
* credentials:
* fn::invoke:
* Function: std:file
* Arguments:
* input: path/to/credentials.json
* Return: result
* extensionHeaders:
* x-goog-if-generation-match: 1
* ```
*
* @param argument A collection of arguments for invoking getObjectSignedUrl.
* @return A collection of values returned by getObjectSignedUrl.
*/
public suspend fun getObjectSignedUrl(argument: GetObjectSignedUrlPlainArgs): GetObjectSignedUrlResult =
getObjectSignedUrlResultToKotlin(getObjectSignedUrlPlain(argument.toJava()).await())
/**
* @see [getObjectSignedUrl].
* @param bucket The name of the bucket to read the object from
* @param contentMd5 The [MD5 digest](https://cloud.google.com/storage/docs/hashes-etags#_MD5) value in Base64.
* Typically retrieved from `google_storage_bucket_object.object.md5hash` attribute.
* If you provide this in the datasource, the client (e.g. browser, curl) must provide the `Content-MD5` HTTP header with this same value in its request.
* @param contentType If you specify this in the datasource, the client must provide the `Content-Type` HTTP header with the same value in its request.
* @param credentials What Google service account credentials json should be used to sign the URL.
* This data source checks the following locations for credentials, in order of preference: data source `credentials` attribute, provider `credentials` attribute and finally the GOOGLE_APPLICATION_CREDENTIALS environment variable.
* > **NOTE** the default google credentials configured by `gcloud` sdk or the service account associated with a compute instance cannot be used, because these do not include the private key required to sign the URL. A valid `json` service account credentials key file must be used, as generated via Google cloud console.
* @param duration For how long shall the signed URL be valid (defaults to 1 hour - i.e. `1h`).
* See [here](https://golang.org/pkg/time/#ParseDuration) for info on valid duration formats.
* @param extensionHeaders As needed. The server checks to make sure that the client provides matching values in requests using the signed URL.
* Any header starting with `x-goog-` is accepted but see the [Google Docs](https://cloud.google.com/storage/docs/xml-api/reference-headers) for list of headers that are supported by Google.
* @param httpMethod What HTTP Method will the signed URL allow (defaults to `GET`)
* @param path The full path to the object inside the bucket
* @return A collection of values returned by getObjectSignedUrl.
*/
public suspend fun getObjectSignedUrl(
bucket: String,
contentMd5: String? = null,
contentType: String? = null,
credentials: String? = null,
duration: String? = null,
extensionHeaders: Map? = null,
httpMethod: String? = null,
path: String,
): GetObjectSignedUrlResult {
val argument = GetObjectSignedUrlPlainArgs(
bucket = bucket,
contentMd5 = contentMd5,
contentType = contentType,
credentials = credentials,
duration = duration,
extensionHeaders = extensionHeaders,
httpMethod = httpMethod,
path = path,
)
return getObjectSignedUrlResultToKotlin(getObjectSignedUrlPlain(argument.toJava()).await())
}
/**
* @see [getObjectSignedUrl].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetObjectSignedUrlPlainArgs].
* @return A collection of values returned by getObjectSignedUrl.
*/
public suspend fun getObjectSignedUrl(argument: suspend GetObjectSignedUrlPlainArgsBuilder.() -> Unit): GetObjectSignedUrlResult {
val builder = GetObjectSignedUrlPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getObjectSignedUrlResultToKotlin(getObjectSignedUrlPlain(builtArgument.toJava()).await())
}
/**
* Get the email address of a project's unique [automatic Google Cloud Storage service account](https://cloud.google.com/storage/docs/projects#service-accounts).
* For each Google Cloud project, Google maintains a unique service account which
* is used as the identity for various Google Cloud Storage operations, including
* operations involving
* [customer-managed encryption keys](https://cloud.google.com/storage/docs/encryption/customer-managed-keys)
* and those involving
* [storage notifications to pub/sub](https://cloud.google.com/storage/docs/gsutil/commands/notification).
* This automatic Google service account requires access to the relevant Cloud KMS keys or pub/sub topics, respectively, in order for Cloud Storage to use
* these customer-managed resources.
* The service account has a well-known, documented naming format which is parameterised on the numeric Google project ID.
* However, as noted in [the docs](https://cloud.google.com/storage/docs/projects#service-accounts), it is only created when certain relevant actions occur which
* presuppose its existence.
* These actions include calling a [Cloud Storage API endpoint](https://cloud.google.com/storage/docs/json_api/v1/projects/serviceAccount/get) to yield the
* service account's identity, or performing some operations in the UI which must use the service account's identity, such as attempting to list Cloud KMS keys
* on the bucket creation page.
* Use of this data source calls the relevant API endpoint to obtain the service account's identity and thus ensures it exists prior to any API operations
* which demand its existence, such as specifying it in Cloud IAM policy.
* Always prefer to use this data source over interpolating the project ID into the well-known format for this service account, as the latter approach may cause
* provider update errors in cases where the service account does not yet exist.
* > When you write provider code which uses features depending on this service account *and* your provider code adds the service account in IAM policy on other resources,
* you must take care for race conditions between the establishment of the IAM policy and creation of the relevant Cloud Storage resource.
* Cloud Storage APIs will require permissions on resources such as pub/sub topics or Cloud KMS keys to exist *before* the attempt to utilise them in a
* bucket configuration, otherwise the API calls will fail.
* You may need to use `depends_on` to create an explicit dependency between the IAM policy resource and the Cloud Storage resource which depends on it.
* See the examples here and in the `gcp.storage.Notification` resource.
* For more information see
* [the API reference](https://cloud.google.com/storage/docs/json_api/v1/projects/serviceAccount).
* ## Example Usage
* ### Pub/Sub Notifications
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const gcsAccount = gcp.storage.getProjectServiceAccount({});
* const binding = new gcp.pubsub.TopicIAMBinding("binding", {
* topic: topic.name,
* role: "roles/pubsub.publisher",
* members: [gcsAccount.then(gcsAccount => `serviceAccount:${gcsAccount.emailAddress}`)],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* gcs_account = gcp.storage.get_project_service_account()
* binding = gcp.pubsub.TopicIAMBinding("binding",
* topic=topic["name"],
* role="roles/pubsub.publisher",
* members=[f"serviceAccount:{gcs_account.email_address}"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var gcsAccount = Gcp.Storage.GetProjectServiceAccount.Invoke();
* var binding = new Gcp.PubSub.TopicIAMBinding("binding", new()
* {
* Topic = topic.Name,
* Role = "roles/pubsub.publisher",
* Members = new[]
* {
* $"serviceAccount:{gcsAccount.Apply(getProjectServiceAccountResult => getProjectServiceAccountResult.EmailAddress)}",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/pubsub"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* gcsAccount, err := storage.GetProjectServiceAccount(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = pubsub.NewTopicIAMBinding(ctx, "binding", &pubsub.TopicIAMBindingArgs{
* Topic: pulumi.Any(topic.Name),
* Role: pulumi.String("roles/pubsub.publisher"),
* Members: pulumi.StringArray{
* pulumi.Sprintf("serviceAccount:%v", gcsAccount.EmailAddress),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetProjectServiceAccountArgs;
* import com.pulumi.gcp.pubsub.TopicIAMBinding;
* import com.pulumi.gcp.pubsub.TopicIAMBindingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var gcsAccount = StorageFunctions.getProjectServiceAccount();
* var binding = new TopicIAMBinding("binding", TopicIAMBindingArgs.builder()
* .topic(topic.name())
* .role("roles/pubsub.publisher")
* .members(String.format("serviceAccount:%s", gcsAccount.applyValue(getProjectServiceAccountResult -> getProjectServiceAccountResult.emailAddress())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* binding:
* type: gcp:pubsub:TopicIAMBinding
* properties:
* topic: ${topic.name}
* role: roles/pubsub.publisher
* members:
* - serviceAccount:${gcsAccount.emailAddress}
* variables:
* gcsAccount:
* fn::invoke:
* Function: gcp:storage:getProjectServiceAccount
* Arguments: {}
* ```
*
* ### Cloud KMS Keys
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const gcsAccount = gcp.storage.getProjectServiceAccount({});
* const binding = new gcp.kms.CryptoKeyIAMBinding("binding", {
* cryptoKeyId: "your-crypto-key-id",
* role: "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* members: [gcsAccount.then(gcsAccount => `serviceAccount:${gcsAccount.emailAddress}`)],
* });
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "kms-protected-bucket",
* location: "US",
* encryption: {
* defaultKmsKeyName: "your-crypto-key-id",
* },
* }, {
* dependsOn: [binding],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* gcs_account = gcp.storage.get_project_service_account()
* binding = gcp.kms.CryptoKeyIAMBinding("binding",
* crypto_key_id="your-crypto-key-id",
* role="roles/cloudkms.cryptoKeyEncrypterDecrypter",
* members=[f"serviceAccount:{gcs_account.email_address}"])
* bucket = gcp.storage.Bucket("bucket",
* name="kms-protected-bucket",
* location="US",
* encryption={
* "default_kms_key_name": "your-crypto-key-id",
* },
* opts = pulumi.ResourceOptions(depends_on=[binding]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var gcsAccount = Gcp.Storage.GetProjectServiceAccount.Invoke();
* var binding = new Gcp.Kms.CryptoKeyIAMBinding("binding", new()
* {
* CryptoKeyId = "your-crypto-key-id",
* Role = "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* Members = new[]
* {
* $"serviceAccount:{gcsAccount.Apply(getProjectServiceAccountResult => getProjectServiceAccountResult.EmailAddress)}",
* },
* });
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "kms-protected-bucket",
* Location = "US",
* Encryption = new Gcp.Storage.Inputs.BucketEncryptionArgs
* {
* DefaultKmsKeyName = "your-crypto-key-id",
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* binding,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* gcsAccount, err := storage.GetProjectServiceAccount(ctx, nil, nil)
* if err != nil {
* return err
* }
* binding, err := kms.NewCryptoKeyIAMBinding(ctx, "binding", &kms.CryptoKeyIAMBindingArgs{
* CryptoKeyId: pulumi.String("your-crypto-key-id"),
* Role: pulumi.String("roles/cloudkms.cryptoKeyEncrypterDecrypter"),
* Members: pulumi.StringArray{
* pulumi.Sprintf("serviceAccount:%v", gcsAccount.EmailAddress),
* },
* })
* if err != nil {
* return err
* }
* _, err = storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("kms-protected-bucket"),
* Location: pulumi.String("US"),
* Encryption: &storage.BucketEncryptionArgs{
* DefaultKmsKeyName: pulumi.String("your-crypto-key-id"),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* binding,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetProjectServiceAccountArgs;
* import com.pulumi.gcp.kms.CryptoKeyIAMBinding;
* import com.pulumi.gcp.kms.CryptoKeyIAMBindingArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.storage.inputs.BucketEncryptionArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var gcsAccount = StorageFunctions.getProjectServiceAccount();
* var binding = new CryptoKeyIAMBinding("binding", CryptoKeyIAMBindingArgs.builder()
* .cryptoKeyId("your-crypto-key-id")
* .role("roles/cloudkms.cryptoKeyEncrypterDecrypter")
* .members(String.format("serviceAccount:%s", gcsAccount.applyValue(getProjectServiceAccountResult -> getProjectServiceAccountResult.emailAddress())))
* .build());
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("kms-protected-bucket")
* .location("US")
* .encryption(BucketEncryptionArgs.builder()
* .defaultKmsKeyName("your-crypto-key-id")
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(binding)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* binding:
* type: gcp:kms:CryptoKeyIAMBinding
* properties:
* cryptoKeyId: your-crypto-key-id
* role: roles/cloudkms.cryptoKeyEncrypterDecrypter
* members:
* - serviceAccount:${gcsAccount.emailAddress}
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: kms-protected-bucket
* location: US
* encryption:
* defaultKmsKeyName: your-crypto-key-id
* options:
* dependson:
* - ${binding}
* variables:
* gcsAccount:
* fn::invoke:
* Function: gcp:storage:getProjectServiceAccount
* Arguments: {}
* ```
*
* @param argument A collection of arguments for invoking getProjectServiceAccount.
* @return A collection of values returned by getProjectServiceAccount.
*/
public suspend fun getProjectServiceAccount(argument: GetProjectServiceAccountPlainArgs): GetProjectServiceAccountResult =
getProjectServiceAccountResultToKotlin(getProjectServiceAccountPlain(argument.toJava()).await())
/**
* @see [getProjectServiceAccount].
* @param project The project the unique service account was created for. If it is not provided, the provider project is used.
* @param userProject The project the lookup originates from. This field is used if you are making the request
* from a different account than the one you are finding the service account for.
* @return A collection of values returned by getProjectServiceAccount.
*/
public suspend fun getProjectServiceAccount(project: String? = null, userProject: String? = null): GetProjectServiceAccountResult {
val argument = GetProjectServiceAccountPlainArgs(
project = project,
userProject = userProject,
)
return getProjectServiceAccountResultToKotlin(getProjectServiceAccountPlain(argument.toJava()).await())
}
/**
* @see [getProjectServiceAccount].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetProjectServiceAccountPlainArgs].
* @return A collection of values returned by getProjectServiceAccount.
*/
public suspend fun getProjectServiceAccount(argument: suspend GetProjectServiceAccountPlainArgsBuilder.() -> Unit): GetProjectServiceAccountResult {
val builder = GetProjectServiceAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProjectServiceAccountResultToKotlin(getProjectServiceAccountPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to retrieve Storage Transfer service account for this project
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const default = gcp.storage.getTransferProjectServiceAccount({});
* export const defaultAccount = _default.then(_default => _default.email);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.storage.get_transfer_project_service_account()
* pulumi.export("defaultAccount", default.email)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Storage.GetTransferProjectServiceAccount.Invoke();
* return new Dictionary
* {
* ["defaultAccount"] = @default.Apply(@default => @default.Apply(getTransferProjectServiceAccountResult => getTransferProjectServiceAccountResult.Email)),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _default, err := storage.GetTransferProjectServiceAccount(ctx, nil, nil)
* if err != nil {
* return err
* }
* ctx.Export("defaultAccount", _default.Email)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetTransferProjectServiceAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = StorageFunctions.getTransferProjectServiceAccount();
* ctx.export("defaultAccount", default_.email());
* }
* }
* ```
* ```yaml
* variables:
* default:
* fn::invoke:
* Function: gcp:storage:getTransferProjectServiceAccount
* Arguments: {}
* outputs:
* defaultAccount: ${default.email}
* ```
*
* @param argument A collection of arguments for invoking getTransferProjectServiceAccount.
* @return A collection of values returned by getTransferProjectServiceAccount.
*/
public suspend fun getTransferProjectServiceAccount(argument: GetTransferProjectServiceAccountPlainArgs): GetTransferProjectServiceAccountResult =
getTransferProjectServiceAccountResultToKotlin(getTransferProjectServiceAccountPlain(argument.toJava()).await())
/**
* @see [getTransferProjectServiceAccount].
* @param project The project ID. If it is not provided, the provider project is used.
* @return A collection of values returned by getTransferProjectServiceAccount.
*/
public suspend fun getTransferProjectServiceAccount(project: String? = null): GetTransferProjectServiceAccountResult {
val argument = GetTransferProjectServiceAccountPlainArgs(
project = project,
)
return getTransferProjectServiceAccountResultToKotlin(getTransferProjectServiceAccountPlain(argument.toJava()).await())
}
/**
* @see [getTransferProjectServiceAccount].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServiceAccountPlainArgs].
* @return A collection of values returned by getTransferProjectServiceAccount.
*/
public suspend fun getTransferProjectServiceAccount(argument: suspend GetTransferProjectServiceAccountPlainArgsBuilder.() -> Unit): GetTransferProjectServiceAccountResult {
val builder = GetTransferProjectServiceAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTransferProjectServiceAccountResultToKotlin(getTransferProjectServiceAccountPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to retrieve Storage Transfer service account for this project
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const default = gcp.storage.getTransferProjectServiceAccount({});
* export const defaultAccount = _default.then(_default => _default.email);
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.storage.get_transfer_project_service_account()
* pulumi.export("defaultAccount", default.email)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = Gcp.Storage.GetTransferProjectServiceAccount.Invoke();
* return new Dictionary
* {
* ["defaultAccount"] = @default.Apply(@default => @default.Apply(getTransferProjectServiceAccountResult => getTransferProjectServiceAccountResult.Email)),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _default, err := storage.GetTransferProjectServiceAccount(ctx, nil, nil)
* if err != nil {
* return err
* }
* ctx.Export("defaultAccount", _default.Email)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.storage.StorageFunctions;
* import com.pulumi.gcp.storage.inputs.GetTransferProjectServiceAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var default = StorageFunctions.getTransferProjectServiceAccount();
* ctx.export("defaultAccount", default_.email());
* }
* }
* ```
* ```yaml
* variables:
* default:
* fn::invoke:
* Function: gcp:storage:getTransferProjectServiceAccount
* Arguments: {}
* outputs:
* defaultAccount: ${default.email}
* ```
*
* @param argument A collection of arguments for invoking getTransferProjectServieAccount.
* @return A collection of values returned by getTransferProjectServieAccount.
*/
@Deprecated(
message = """
gcp.storage.getTransferProjectServieAccount has been deprecated in favor of
gcp.storage.getTransferProjectServiceAccount
""",
)
public suspend fun getTransferProjectServieAccount(argument: GetTransferProjectServieAccountPlainArgs): GetTransferProjectServieAccountResult =
getTransferProjectServieAccountResultToKotlin(getTransferProjectServieAccountPlain(argument.toJava()).await())
/**
* @see [getTransferProjectServieAccount].
* @param project The project ID. If it is not provided, the provider project is used.
* @return A collection of values returned by getTransferProjectServieAccount.
*/
@Deprecated(
message = """
gcp.storage.getTransferProjectServieAccount has been deprecated in favor of
gcp.storage.getTransferProjectServiceAccount
""",
)
public suspend fun getTransferProjectServieAccount(project: String? = null): GetTransferProjectServieAccountResult {
val argument = GetTransferProjectServieAccountPlainArgs(
project = project,
)
return getTransferProjectServieAccountResultToKotlin(getTransferProjectServieAccountPlain(argument.toJava()).await())
}
/**
* @see [getTransferProjectServieAccount].
* @param argument Builder for [com.pulumi.gcp.storage.kotlin.inputs.GetTransferProjectServieAccountPlainArgs].
* @return A collection of values returned by getTransferProjectServieAccount.
*/
@Deprecated(
message = """
gcp.storage.getTransferProjectServieAccount has been deprecated in favor of
gcp.storage.getTransferProjectServiceAccount
""",
)
public suspend fun getTransferProjectServieAccount(argument: suspend GetTransferProjectServieAccountPlainArgsBuilder.() -> Unit): GetTransferProjectServieAccountResult {
val builder = GetTransferProjectServieAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTransferProjectServieAccountResultToKotlin(getTransferProjectServieAccountPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy