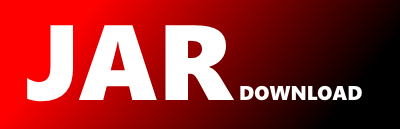
com.pulumi.gcp.vertex.kotlin.AiEndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.vertex.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.vertex.AiEndpointArgs.builder
import com.pulumi.gcp.vertex.kotlin.inputs.AiEndpointEncryptionSpecArgs
import com.pulumi.gcp.vertex.kotlin.inputs.AiEndpointEncryptionSpecArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Models are deployed into it, and afterwards Endpoint is called to obtain predictions and explanations.
* To get more information about Endpoint, see:
* * [API documentation](https://cloud.google.com/vertex-ai/docs/reference/rest/v1beta1/projects.locations.endpoints)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/vertex-ai/docs)
* ## Example Usage
* ### Vertex Ai Endpoint Network
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const vertexNetwork = new gcp.compute.Network("vertex_network", {name: "network-name"});
* const vertexRange = new gcp.compute.GlobalAddress("vertex_range", {
* name: "address-name",
* purpose: "VPC_PEERING",
* addressType: "INTERNAL",
* prefixLength: 24,
* network: vertexNetwork.id,
* });
* const vertexVpcConnection = new gcp.servicenetworking.Connection("vertex_vpc_connection", {
* network: vertexNetwork.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [vertexRange.name],
* });
* const project = gcp.organizations.getProject({});
* const endpoint = new gcp.vertex.AiEndpoint("endpoint", {
* name: "endpoint-name",
* displayName: "sample-endpoint",
* description: "A sample vertex endpoint",
* location: "us-central1",
* region: "us-central1",
* labels: {
* "label-one": "value-one",
* },
* network: pulumi.all([project, vertexNetwork.name]).apply(([project, name]) => `projects/${project.number}/global/networks/${name}`),
* encryptionSpec: {
* kmsKeyName: "kms-name",
* },
* }, {
* dependsOn: [vertexVpcConnection],
* });
* const cryptoKey = new gcp.kms.CryptoKeyIAMMember("crypto_key", {
* cryptoKeyId: "kms-name",
* role: "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member: project.then(project => `serviceAccount:service-${project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com`),
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* vertex_network = gcp.compute.Network("vertex_network", name="network-name")
* vertex_range = gcp.compute.GlobalAddress("vertex_range",
* name="address-name",
* purpose="VPC_PEERING",
* address_type="INTERNAL",
* prefix_length=24,
* network=vertex_network.id)
* vertex_vpc_connection = gcp.servicenetworking.Connection("vertex_vpc_connection",
* network=vertex_network.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[vertex_range.name])
* project = gcp.organizations.get_project()
* endpoint = gcp.vertex.AiEndpoint("endpoint",
* name="endpoint-name",
* display_name="sample-endpoint",
* description="A sample vertex endpoint",
* location="us-central1",
* region="us-central1",
* labels={
* "label-one": "value-one",
* },
* network=vertex_network.name.apply(lambda name: f"projects/{project.number}/global/networks/{name}"),
* encryption_spec={
* "kms_key_name": "kms-name",
* },
* opts = pulumi.ResourceOptions(depends_on=[vertex_vpc_connection]))
* crypto_key = gcp.kms.CryptoKeyIAMMember("crypto_key",
* crypto_key_id="kms-name",
* role="roles/cloudkms.cryptoKeyEncrypterDecrypter",
* member=f"serviceAccount:service-{project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var vertexNetwork = new Gcp.Compute.Network("vertex_network", new()
* {
* Name = "network-name",
* });
* var vertexRange = new Gcp.Compute.GlobalAddress("vertex_range", new()
* {
* Name = "address-name",
* Purpose = "VPC_PEERING",
* AddressType = "INTERNAL",
* PrefixLength = 24,
* Network = vertexNetwork.Id,
* });
* var vertexVpcConnection = new Gcp.ServiceNetworking.Connection("vertex_vpc_connection", new()
* {
* Network = vertexNetwork.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* vertexRange.Name,
* },
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var endpoint = new Gcp.Vertex.AiEndpoint("endpoint", new()
* {
* Name = "endpoint-name",
* DisplayName = "sample-endpoint",
* Description = "A sample vertex endpoint",
* Location = "us-central1",
* Region = "us-central1",
* Labels =
* {
* { "label-one", "value-one" },
* },
* Network = Output.Tuple(project, vertexNetwork.Name).Apply(values =>
* {
* var project = values.Item1;
* var name = values.Item2;
* return $"projects/{project.Apply(getProjectResult => getProjectResult.Number)}/global/networks/{name}";
* }),
* EncryptionSpec = new Gcp.Vertex.Inputs.AiEndpointEncryptionSpecArgs
* {
* KmsKeyName = "kms-name",
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* vertexVpcConnection,
* },
* });
* var cryptoKey = new Gcp.Kms.CryptoKeyIAMMember("crypto_key", new()
* {
* CryptoKeyId = "kms-name",
* Role = "roles/cloudkms.cryptoKeyEncrypterDecrypter",
* Member = $"serviceAccount:service-{project.Apply(getProjectResult => getProjectResult.Number)}@gcp-sa-aiplatform.iam.gserviceaccount.com",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/kms"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/vertex"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* vertexNetwork, err := compute.NewNetwork(ctx, "vertex_network", &compute.NetworkArgs{
* Name: pulumi.String("network-name"),
* })
* if err != nil {
* return err
* }
* vertexRange, err := compute.NewGlobalAddress(ctx, "vertex_range", &compute.GlobalAddressArgs{
* Name: pulumi.String("address-name"),
* Purpose: pulumi.String("VPC_PEERING"),
* AddressType: pulumi.String("INTERNAL"),
* PrefixLength: pulumi.Int(24),
* Network: vertexNetwork.ID(),
* })
* if err != nil {
* return err
* }
* vertexVpcConnection, err := servicenetworking.NewConnection(ctx, "vertex_vpc_connection", &servicenetworking.ConnectionArgs{
* Network: vertexNetwork.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* vertexRange.Name,
* },
* })
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, nil, nil)
* if err != nil {
* return err
* }
* _, err = vertex.NewAiEndpoint(ctx, "endpoint", &vertex.AiEndpointArgs{
* Name: pulumi.String("endpoint-name"),
* DisplayName: pulumi.String("sample-endpoint"),
* Description: pulumi.String("A sample vertex endpoint"),
* Location: pulumi.String("us-central1"),
* Region: pulumi.String("us-central1"),
* Labels: pulumi.StringMap{
* "label-one": pulumi.String("value-one"),
* },
* Network: vertexNetwork.Name.ApplyT(func(name string) (string, error) {
* return fmt.Sprintf("projects/%v/global/networks/%v", project.Number, name), nil
* }).(pulumi.StringOutput),
* EncryptionSpec: &vertex.AiEndpointEncryptionSpecArgs{
* KmsKeyName: pulumi.String("kms-name"),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* vertexVpcConnection,
* }))
* if err != nil {
* return err
* }
* _, err = kms.NewCryptoKeyIAMMember(ctx, "crypto_key", &kms.CryptoKeyIAMMemberArgs{
* CryptoKeyId: pulumi.String("kms-name"),
* Role: pulumi.String("roles/cloudkms.cryptoKeyEncrypterDecrypter"),
* Member: pulumi.Sprintf("serviceAccount:service-%[email protected]", project.Number),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.vertex.AiEndpoint;
* import com.pulumi.gcp.vertex.AiEndpointArgs;
* import com.pulumi.gcp.vertex.inputs.AiEndpointEncryptionSpecArgs;
* import com.pulumi.gcp.kms.CryptoKeyIAMMember;
* import com.pulumi.gcp.kms.CryptoKeyIAMMemberArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var vertexNetwork = new Network("vertexNetwork", NetworkArgs.builder()
* .name("network-name")
* .build());
* var vertexRange = new GlobalAddress("vertexRange", GlobalAddressArgs.builder()
* .name("address-name")
* .purpose("VPC_PEERING")
* .addressType("INTERNAL")
* .prefixLength(24)
* .network(vertexNetwork.id())
* .build());
* var vertexVpcConnection = new Connection("vertexVpcConnection", ConnectionArgs.builder()
* .network(vertexNetwork.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(vertexRange.name())
* .build());
* final var project = OrganizationsFunctions.getProject();
* var endpoint = new AiEndpoint("endpoint", AiEndpointArgs.builder()
* .name("endpoint-name")
* .displayName("sample-endpoint")
* .description("A sample vertex endpoint")
* .location("us-central1")
* .region("us-central1")
* .labels(Map.of("label-one", "value-one"))
* .network(vertexNetwork.name().applyValue(name -> String.format("projects/%s/global/networks/%s", project.applyValue(getProjectResult -> getProjectResult.number()),name)))
* .encryptionSpec(AiEndpointEncryptionSpecArgs.builder()
* .kmsKeyName("kms-name")
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(vertexVpcConnection)
* .build());
* var cryptoKey = new CryptoKeyIAMMember("cryptoKey", CryptoKeyIAMMemberArgs.builder()
* .cryptoKeyId("kms-name")
* .role("roles/cloudkms.cryptoKeyEncrypterDecrypter")
* .member(String.format("serviceAccount:service-%[email protected]", project.applyValue(getProjectResult -> getProjectResult.number())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* endpoint:
* type: gcp:vertex:AiEndpoint
* properties:
* name: endpoint-name
* displayName: sample-endpoint
* description: A sample vertex endpoint
* location: us-central1
* region: us-central1
* labels:
* label-one: value-one
* network: projects/${project.number}/global/networks/${vertexNetwork.name}
* encryptionSpec:
* kmsKeyName: kms-name
* options:
* dependson:
* - ${vertexVpcConnection}
* vertexVpcConnection:
* type: gcp:servicenetworking:Connection
* name: vertex_vpc_connection
* properties:
* network: ${vertexNetwork.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${vertexRange.name}
* vertexRange:
* type: gcp:compute:GlobalAddress
* name: vertex_range
* properties:
* name: address-name
* purpose: VPC_PEERING
* addressType: INTERNAL
* prefixLength: 24
* network: ${vertexNetwork.id}
* vertexNetwork:
* type: gcp:compute:Network
* name: vertex_network
* properties:
* name: network-name
* cryptoKey:
* type: gcp:kms:CryptoKeyIAMMember
* name: crypto_key
* properties:
* cryptoKeyId: kms-name
* role: roles/cloudkms.cryptoKeyEncrypterDecrypter
* member: serviceAccount:service-${project.number}@gcp-sa-aiplatform.iam.gserviceaccount.com
* variables:
* project:
* fn::invoke:
* Function: gcp:organizations:getProject
* Arguments: {}
* ```
*
* ## Import
* Endpoint can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/endpoints/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Endpoint can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:vertex/aiEndpoint:AiEndpoint default projects/{{project}}/locations/{{location}}/endpoints/{{name}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiEndpoint:AiEndpoint default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiEndpoint:AiEndpoint default {{location}}/{{name}}
* ```
* @property description The description of the Endpoint.
* @property displayName Required. The display name of the Endpoint. The name can be up to 128 characters long and can consist of any UTF-8 characters.
* @property encryptionSpec Customer-managed encryption key spec for an Endpoint. If set, this Endpoint and all sub-resources of this Endpoint will be secured by this key.
* Structure is documented below.
* @property labels The labels with user-defined metadata to organize your Endpoints. Label keys and values can be no longer than 64 characters (Unicode codepoints), can only contain lowercase letters, numeric characters, underscores and dashes. International characters are allowed. See https://goo.gl/xmQnxf for more information and examples of labels.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property location The location for the resource
* - - -
* @property name The resource name of the Endpoint. The name must be numeric with no leading zeros and can be at most 10 digits.
* @property network The full name of the Google Compute Engine [network](https://cloud.google.com//compute/docs/networks-and-firewalls#networks) to which the Endpoint should be peered. Private services access must already be configured for the network. If left unspecified, the Endpoint is not peered with any network. Only one of the fields, network or enable_private_service_connect, can be set. [Format](https://cloud.google.com/compute/docs/reference/rest/v1/networks/insert): `projects/{project}/global/networks/{network}`. Where `{project}` is a project number, as in `12345`, and `{network}` is network name.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property region The region for the resource
*/
public data class AiEndpointArgs(
public val description: Output? = null,
public val displayName: Output? = null,
public val encryptionSpec: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy