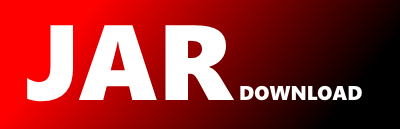
com.pulumi.gcp.backupdisasterrecovery.kotlin.BackupPlan.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.backupdisasterrecovery.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.backupdisasterrecovery.kotlin.outputs.BackupPlanBackupRule
import com.pulumi.gcp.backupdisasterrecovery.kotlin.outputs.BackupPlanBackupRule.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [BackupPlan].
*/
@PulumiTagMarker
public class BackupPlanResourceBuilder internal constructor() {
public var name: String? = null
public var args: BackupPlanArgs = BackupPlanArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BackupPlanArgsBuilder.() -> Unit) {
val builder = BackupPlanArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BackupPlan {
val builtJavaResource =
com.pulumi.gcp.backupdisasterrecovery.BackupPlan(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BackupPlan(builtJavaResource)
}
}
/**
* ## Example Usage
* ### Backup Dr Backup Plan Simple
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myBackupVault = new gcp.backupdisasterrecovery.BackupVault("my_backup_vault", {
* location: "us-central1",
* backupVaultId: "backup-vault-simple-test",
* backupMinimumEnforcedRetentionDuration: "100000s",
* });
* const my_backup_plan_1 = new gcp.backupdisasterrecovery.BackupPlan("my-backup-plan-1", {
* location: "us-central1",
* backupPlanId: "backup-plan-simple-test",
* resourceType: "compute.googleapis.com/Instance",
* backupVault: myBackupVault.id,
* backupRules: [{
* ruleId: "rule-1",
* backupRetentionDays: 5,
* standardSchedule: {
* recurrenceType: "HOURLY",
* hourlyFrequency: 6,
* timeZone: "UTC",
* backupWindow: {
* startHourOfDay: 0,
* endHourOfDay: 24,
* },
* },
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_backup_vault = gcp.backupdisasterrecovery.BackupVault("my_backup_vault",
* location="us-central1",
* backup_vault_id="backup-vault-simple-test",
* backup_minimum_enforced_retention_duration="100000s")
* my_backup_plan_1 = gcp.backupdisasterrecovery.BackupPlan("my-backup-plan-1",
* location="us-central1",
* backup_plan_id="backup-plan-simple-test",
* resource_type="compute.googleapis.com/Instance",
* backup_vault=my_backup_vault.id,
* backup_rules=[{
* "rule_id": "rule-1",
* "backup_retention_days": 5,
* "standard_schedule": {
* "recurrence_type": "HOURLY",
* "hourly_frequency": 6,
* "time_zone": "UTC",
* "backup_window": {
* "start_hour_of_day": 0,
* "end_hour_of_day": 24,
* },
* },
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myBackupVault = new Gcp.BackupDisasterRecovery.BackupVault("my_backup_vault", new()
* {
* Location = "us-central1",
* BackupVaultId = "backup-vault-simple-test",
* BackupMinimumEnforcedRetentionDuration = "100000s",
* });
* var my_backup_plan_1 = new Gcp.BackupDisasterRecovery.BackupPlan("my-backup-plan-1", new()
* {
* Location = "us-central1",
* BackupPlanId = "backup-plan-simple-test",
* ResourceType = "compute.googleapis.com/Instance",
* BackupVault = myBackupVault.Id,
* BackupRules = new[]
* {
* new Gcp.BackupDisasterRecovery.Inputs.BackupPlanBackupRuleArgs
* {
* RuleId = "rule-1",
* BackupRetentionDays = 5,
* StandardSchedule = new Gcp.BackupDisasterRecovery.Inputs.BackupPlanBackupRuleStandardScheduleArgs
* {
* RecurrenceType = "HOURLY",
* HourlyFrequency = 6,
* TimeZone = "UTC",
* BackupWindow = new Gcp.BackupDisasterRecovery.Inputs.BackupPlanBackupRuleStandardScheduleBackupWindowArgs
* {
* StartHourOfDay = 0,
* EndHourOfDay = 24,
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/backupdisasterrecovery"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* myBackupVault, err := backupdisasterrecovery.NewBackupVault(ctx, "my_backup_vault", &backupdisasterrecovery.BackupVaultArgs{
* Location: pulumi.String("us-central1"),
* BackupVaultId: pulumi.String("backup-vault-simple-test"),
* BackupMinimumEnforcedRetentionDuration: pulumi.String("100000s"),
* })
* if err != nil {
* return err
* }
* _, err = backupdisasterrecovery.NewBackupPlan(ctx, "my-backup-plan-1", &backupdisasterrecovery.BackupPlanArgs{
* Location: pulumi.String("us-central1"),
* BackupPlanId: pulumi.String("backup-plan-simple-test"),
* ResourceType: pulumi.String("compute.googleapis.com/Instance"),
* BackupVault: myBackupVault.ID(),
* BackupRules: backupdisasterrecovery.BackupPlanBackupRuleArray{
* &backupdisasterrecovery.BackupPlanBackupRuleArgs{
* RuleId: pulumi.String("rule-1"),
* BackupRetentionDays: pulumi.Int(5),
* StandardSchedule: &backupdisasterrecovery.BackupPlanBackupRuleStandardScheduleArgs{
* RecurrenceType: pulumi.String("HOURLY"),
* HourlyFrequency: pulumi.Int(6),
* TimeZone: pulumi.String("UTC"),
* BackupWindow: &backupdisasterrecovery.BackupPlanBackupRuleStandardScheduleBackupWindowArgs{
* StartHourOfDay: pulumi.Int(0),
* EndHourOfDay: pulumi.Int(24),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.backupdisasterrecovery.BackupVault;
* import com.pulumi.gcp.backupdisasterrecovery.BackupVaultArgs;
* import com.pulumi.gcp.backupdisasterrecovery.BackupPlan;
* import com.pulumi.gcp.backupdisasterrecovery.BackupPlanArgs;
* import com.pulumi.gcp.backupdisasterrecovery.inputs.BackupPlanBackupRuleArgs;
* import com.pulumi.gcp.backupdisasterrecovery.inputs.BackupPlanBackupRuleStandardScheduleArgs;
* import com.pulumi.gcp.backupdisasterrecovery.inputs.BackupPlanBackupRuleStandardScheduleBackupWindowArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myBackupVault = new BackupVault("myBackupVault", BackupVaultArgs.builder()
* .location("us-central1")
* .backupVaultId("backup-vault-simple-test")
* .backupMinimumEnforcedRetentionDuration("100000s")
* .build());
* var my_backup_plan_1 = new BackupPlan("my-backup-plan-1", BackupPlanArgs.builder()
* .location("us-central1")
* .backupPlanId("backup-plan-simple-test")
* .resourceType("compute.googleapis.com/Instance")
* .backupVault(myBackupVault.id())
* .backupRules(BackupPlanBackupRuleArgs.builder()
* .ruleId("rule-1")
* .backupRetentionDays(5)
* .standardSchedule(BackupPlanBackupRuleStandardScheduleArgs.builder()
* .recurrenceType("HOURLY")
* .hourlyFrequency(6)
* .timeZone("UTC")
* .backupWindow(BackupPlanBackupRuleStandardScheduleBackupWindowArgs.builder()
* .startHourOfDay(0)
* .endHourOfDay(24)
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myBackupVault:
* type: gcp:backupdisasterrecovery:BackupVault
* name: my_backup_vault
* properties:
* location: us-central1
* backupVaultId: backup-vault-simple-test
* backupMinimumEnforcedRetentionDuration: 100000s
* my-backup-plan-1:
* type: gcp:backupdisasterrecovery:BackupPlan
* properties:
* location: us-central1
* backupPlanId: backup-plan-simple-test
* resourceType: compute.googleapis.com/Instance
* backupVault: ${myBackupVault.id}
* backupRules:
* - ruleId: rule-1
* backupRetentionDays: 5
* standardSchedule:
* recurrenceType: HOURLY
* hourlyFrequency: 6
* timeZone: UTC
* backupWindow:
* startHourOfDay: 0
* endHourOfDay: 24
* ```
*
* ## Import
* BackupPlan can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/backupPlans/{{backup_plan_id}}`
* * `{{project}}/{{location}}/{{backup_plan_id}}`
* * `{{location}}/{{backup_plan_id}}`
* When using the `pulumi import` command, BackupPlan can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupPlan:BackupPlan default projects/{{project}}/locations/{{location}}/backupPlans/{{backup_plan_id}}
* ```
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupPlan:BackupPlan default {{project}}/{{location}}/{{backup_plan_id}}
* ```
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupPlan:BackupPlan default {{location}}/{{backup_plan_id}}
* ```
*/
public class BackupPlan internal constructor(
override val javaResource: com.pulumi.gcp.backupdisasterrecovery.BackupPlan,
) : KotlinCustomResource(javaResource, BackupPlanMapper) {
/**
* The ID of the backup plan
*/
public val backupPlanId: Output
get() = javaResource.backupPlanId().applyValue({ args0 -> args0 })
/**
* The backup rules for this `BackupPlan`. There must be at least one `BackupRule` message.
* Structure is documented below.
*/
public val backupRules: Output>
get() = javaResource.backupRules().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
})
/**
* Backup vault where the backups gets stored using this Backup plan.
*/
public val backupVault: Output
get() = javaResource.backupVault().applyValue({ args0 -> args0 })
/**
* The Google Cloud Platform Service Account to be used by the BackupVault for taking backups.
*/
public val backupVaultServiceAccount: Output
get() = javaResource.backupVaultServiceAccount().applyValue({ args0 -> args0 })
/**
* When the `BackupPlan` was created.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* The description allows for additional details about 'BackupPlan' and its use cases to be provided.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The location for the backup plan
*/
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The name of backup plan resource created
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The resource type to which the `BackupPlan` will be applied. Examples include, "compute.googleapis.com/Instance" and "storage.googleapis.com/Bucket".
*/
public val resourceType: Output
get() = javaResource.resourceType().applyValue({ args0 -> args0 })
/**
* When the `BackupPlan` was last updated.
*/
public val updateTime: Output
get() = javaResource.updateTime().applyValue({ args0 -> args0 })
}
public object BackupPlanMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.backupdisasterrecovery.BackupPlan::class == javaResource::class
override fun map(javaResource: Resource): BackupPlan = BackupPlan(
javaResource as
com.pulumi.gcp.backupdisasterrecovery.BackupPlan,
)
}
/**
* @see [BackupPlan].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [BackupPlan].
*/
public suspend fun backupPlan(name: String, block: suspend BackupPlanResourceBuilder.() -> Unit): BackupPlan {
val builder = BackupPlanResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [BackupPlan].
* @param name The _unique_ name of the resulting resource.
*/
public fun backupPlan(name: String): BackupPlan {
val builder = BackupPlanResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy