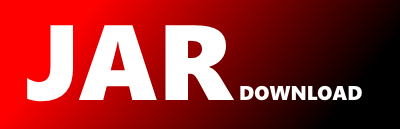
com.pulumi.gcp.backupdisasterrecovery.kotlin.BackupVault.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.backupdisasterrecovery.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [BackupVault].
*/
@PulumiTagMarker
public class BackupVaultResourceBuilder internal constructor() {
public var name: String? = null
public var args: BackupVaultArgs = BackupVaultArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BackupVaultArgsBuilder.() -> Unit) {
val builder = BackupVaultArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BackupVault {
val builtJavaResource =
com.pulumi.gcp.backupdisasterrecovery.BackupVault(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BackupVault(builtJavaResource)
}
}
/**
* Container to store and organize immutable and indelible backups.
* ## Example Usage
* ### Backup Dr Backup Vault Full
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const backup_vault_test = new gcp.backupdisasterrecovery.BackupVault("backup-vault-test", {
* location: "us-central1",
* backupVaultId: "backup-vault-test",
* description: "This is a second backup vault built by Terraform.",
* backupMinimumEnforcedRetentionDuration: "100000s",
* annotations: {
* annotations1: "bar1",
* annotations2: "baz1",
* },
* labels: {
* foo: "bar1",
* bar: "baz1",
* },
* forceUpdate: true,
* accessRestriction: "WITHIN_ORGANIZATION",
* ignoreInactiveDatasources: true,
* ignoreBackupPlanReferences: true,
* allowMissing: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* backup_vault_test = gcp.backupdisasterrecovery.BackupVault("backup-vault-test",
* location="us-central1",
* backup_vault_id="backup-vault-test",
* description="This is a second backup vault built by Terraform.",
* backup_minimum_enforced_retention_duration="100000s",
* annotations={
* "annotations1": "bar1",
* "annotations2": "baz1",
* },
* labels={
* "foo": "bar1",
* "bar": "baz1",
* },
* force_update=True,
* access_restriction="WITHIN_ORGANIZATION",
* ignore_inactive_datasources=True,
* ignore_backup_plan_references=True,
* allow_missing=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var backup_vault_test = new Gcp.BackupDisasterRecovery.BackupVault("backup-vault-test", new()
* {
* Location = "us-central1",
* BackupVaultId = "backup-vault-test",
* Description = "This is a second backup vault built by Terraform.",
* BackupMinimumEnforcedRetentionDuration = "100000s",
* Annotations =
* {
* { "annotations1", "bar1" },
* { "annotations2", "baz1" },
* },
* Labels =
* {
* { "foo", "bar1" },
* { "bar", "baz1" },
* },
* ForceUpdate = true,
* AccessRestriction = "WITHIN_ORGANIZATION",
* IgnoreInactiveDatasources = true,
* IgnoreBackupPlanReferences = true,
* AllowMissing = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/backupdisasterrecovery"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := backupdisasterrecovery.NewBackupVault(ctx, "backup-vault-test", &backupdisasterrecovery.BackupVaultArgs{
* Location: pulumi.String("us-central1"),
* BackupVaultId: pulumi.String("backup-vault-test"),
* Description: pulumi.String("This is a second backup vault built by Terraform."),
* BackupMinimumEnforcedRetentionDuration: pulumi.String("100000s"),
* Annotations: pulumi.StringMap{
* "annotations1": pulumi.String("bar1"),
* "annotations2": pulumi.String("baz1"),
* },
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar1"),
* "bar": pulumi.String("baz1"),
* },
* ForceUpdate: pulumi.Bool(true),
* AccessRestriction: pulumi.String("WITHIN_ORGANIZATION"),
* IgnoreInactiveDatasources: pulumi.Bool(true),
* IgnoreBackupPlanReferences: pulumi.Bool(true),
* AllowMissing: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.backupdisasterrecovery.BackupVault;
* import com.pulumi.gcp.backupdisasterrecovery.BackupVaultArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var backup_vault_test = new BackupVault("backup-vault-test", BackupVaultArgs.builder()
* .location("us-central1")
* .backupVaultId("backup-vault-test")
* .description("This is a second backup vault built by Terraform.")
* .backupMinimumEnforcedRetentionDuration("100000s")
* .annotations(Map.ofEntries(
* Map.entry("annotations1", "bar1"),
* Map.entry("annotations2", "baz1")
* ))
* .labels(Map.ofEntries(
* Map.entry("foo", "bar1"),
* Map.entry("bar", "baz1")
* ))
* .forceUpdate("true")
* .accessRestriction("WITHIN_ORGANIZATION")
* .ignoreInactiveDatasources("true")
* .ignoreBackupPlanReferences("true")
* .allowMissing("true")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* backup-vault-test:
* type: gcp:backupdisasterrecovery:BackupVault
* properties:
* location: us-central1
* backupVaultId: backup-vault-test
* description: This is a second backup vault built by Terraform.
* backupMinimumEnforcedRetentionDuration: 100000s
* annotations:
* annotations1: bar1
* annotations2: baz1
* labels:
* foo: bar1
* bar: baz1
* forceUpdate: 'true'
* accessRestriction: WITHIN_ORGANIZATION
* ignoreInactiveDatasources: 'true'
* ignoreBackupPlanReferences: 'true'
* allowMissing: 'true'
* ```
*
* ## Import
* BackupVault can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/backupVaults/{{backup_vault_id}}`
* * `{{project}}/{{location}}/{{backup_vault_id}}`
* * `{{location}}/{{backup_vault_id}}`
* When using the `pulumi import` command, BackupVault can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupVault:BackupVault default projects/{{project}}/locations/{{location}}/backupVaults/{{backup_vault_id}}
* ```
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupVault:BackupVault default {{project}}/{{location}}/{{backup_vault_id}}
* ```
* ```sh
* $ pulumi import gcp:backupdisasterrecovery/backupVault:BackupVault default {{location}}/{{backup_vault_id}}
* ```
*/
public class BackupVault internal constructor(
override val javaResource: com.pulumi.gcp.backupdisasterrecovery.BackupVault,
) : KotlinCustomResource(javaResource, BackupVaultMapper) {
/**
* Access restriction for the backup vault. Default value is `WITHIN_ORGANIZATION` if not provided during creation.
* Default value is `WITHIN_ORGANIZATION`.
* Possible values are: `ACCESS_RESTRICTION_UNSPECIFIED`, `WITHIN_PROJECT`, `WITHIN_ORGANIZATION`, `UNRESTRICTED`, `WITHIN_ORG_BUT_UNRESTRICTED_FOR_BA`.
*/
public val accessRestriction: Output?
get() = javaResource.accessRestriction().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Allow idempotent deletion of backup vault. The request will still succeed in case the backup vault does not exist.
*/
public val allowMissing: Output?
get() = javaResource.allowMissing().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Optional. User annotations. See https://google.aip.dev/128#annotations
* Stores small amounts of arbitrary data.
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
*/
public val annotations: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy