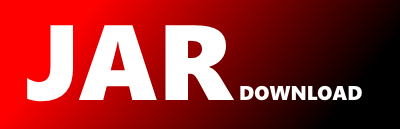
com.pulumi.gcp.bigquery.kotlin.inputs.TableExternalDataConfigurationBigtableOptionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property columnFamilies A list of column families to expose in the table schema along with their types. This list restricts the column families that can be referenced in queries and specifies their value types. You can use this list to do type conversions - see the 'type' field for more details. If you leave this list empty, all column families are present in the table schema and their values are read as BYTES. During a query only the column families referenced in that query are read from Bigtable. Structure is documented below.
* @property ignoreUnspecifiedColumnFamilies If field is true, then the column families that are not specified in columnFamilies list are not exposed in the table schema. Otherwise, they are read with BYTES type values. The default value is false.
* @property outputColumnFamiliesAsJson If field is true, then each column family will be read as a single JSON column. Otherwise they are read as a repeated cell structure containing timestamp/value tuples. The default value is false.
* @property readRowkeyAsString If field is true, then the rowkey column families will be read and converted to string. Otherwise they are read with BYTES type values and users need to manually cast them with CAST if necessary. The default value is false.
*/
public data class TableExternalDataConfigurationBigtableOptionsArgs(
public val columnFamilies: Output>? = null,
public val ignoreUnspecifiedColumnFamilies: Output? = null,
public val outputColumnFamiliesAsJson: Output? = null,
public val readRowkeyAsString: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsArgs =
com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsArgs.builder()
.columnFamilies(
columnFamilies?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.ignoreUnspecifiedColumnFamilies(ignoreUnspecifiedColumnFamilies?.applyValue({ args0 -> args0 }))
.outputColumnFamiliesAsJson(outputColumnFamiliesAsJson?.applyValue({ args0 -> args0 }))
.readRowkeyAsString(readRowkeyAsString?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableExternalDataConfigurationBigtableOptionsArgs].
*/
@PulumiTagMarker
public class TableExternalDataConfigurationBigtableOptionsArgsBuilder internal constructor() {
private var columnFamilies:
Output>? = null
private var ignoreUnspecifiedColumnFamilies: Output? = null
private var outputColumnFamiliesAsJson: Output? = null
private var readRowkeyAsString: Output? = null
/**
* @param value A list of column families to expose in the table schema along with their types. This list restricts the column families that can be referenced in queries and specifies their value types. You can use this list to do type conversions - see the 'type' field for more details. If you leave this list empty, all column families are present in the table schema and their values are read as BYTES. During a query only the column families referenced in that query are read from Bigtable. Structure is documented below.
*/
@JvmName("ggtnqnogewajjejt")
public suspend fun columnFamilies(`value`: Output>) {
this.columnFamilies = value
}
@JvmName("lcxavmcxgwynivmt")
public suspend fun columnFamilies(vararg values: Output) {
this.columnFamilies = Output.all(values.asList())
}
/**
* @param values A list of column families to expose in the table schema along with their types. This list restricts the column families that can be referenced in queries and specifies their value types. You can use this list to do type conversions - see the 'type' field for more details. If you leave this list empty, all column families are present in the table schema and their values are read as BYTES. During a query only the column families referenced in that query are read from Bigtable. Structure is documented below.
*/
@JvmName("grfhygaidhdijysd")
public suspend fun columnFamilies(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy