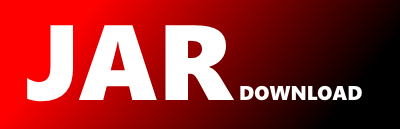
com.pulumi.gcp.bigquery.kotlin.inputs.TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.bigquery.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property encoding The encoding of the values when the type is not STRING. Acceptable encoding values are: TEXT - indicates values are alphanumeric text strings. BINARY - indicates values are encoded using HBase Bytes.toBytes family of functions. 'encoding' can also be set at the column family level. However, the setting at this level takes precedence if 'encoding' is set at both levels.
* @property fieldName If the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as the column field name and is used as field name in queries.
* @property onlyReadLatest If this is set, only the latest version of value in this column are exposed. 'onlyReadLatest' can also be set at the column family level. However, the setting at this level takes precedence if 'onlyReadLatest' is set at both levels.
* @property qualifierEncoded Qualifier of the column. Columns in the parent column family that has this exact qualifier are exposed as . field. If the qualifier is valid UTF-8 string, it can be specified in the qualifierString field. Otherwise, a base-64 encoded value must be set to qualifierEncoded. The column field name is the same as the column qualifier. However, if the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as fieldName.
* @property qualifierString Qualifier string.
* @property type The type to convert the value in cells of this column. The values are expected to be encoded using HBase Bytes.toBytes function when using the BINARY encoding value. Following BigQuery types are allowed (case-sensitive): "BYTES", "STRING", "INTEGER", "FLOAT", "BOOLEAN", "JSON", Default type is "BYTES". 'type' can also be set at the column family level. However, the setting at this level takes precedence if 'type' is set at both levels.
*/
public data class TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs(
public val encoding: Output? = null,
public val fieldName: Output? = null,
public val onlyReadLatest: Output? = null,
public val qualifierEncoded: Output? = null,
public val qualifierString: Output? = null,
public val type: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs =
com.pulumi.gcp.bigquery.inputs.TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs.builder()
.encoding(encoding?.applyValue({ args0 -> args0 }))
.fieldName(fieldName?.applyValue({ args0 -> args0 }))
.onlyReadLatest(onlyReadLatest?.applyValue({ args0 -> args0 }))
.qualifierEncoded(qualifierEncoded?.applyValue({ args0 -> args0 }))
.qualifierString(qualifierString?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs].
*/
@PulumiTagMarker
public class TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgsBuilder internal constructor() {
private var encoding: Output? = null
private var fieldName: Output? = null
private var onlyReadLatest: Output? = null
private var qualifierEncoded: Output? = null
private var qualifierString: Output? = null
private var type: Output? = null
/**
* @param value The encoding of the values when the type is not STRING. Acceptable encoding values are: TEXT - indicates values are alphanumeric text strings. BINARY - indicates values are encoded using HBase Bytes.toBytes family of functions. 'encoding' can also be set at the column family level. However, the setting at this level takes precedence if 'encoding' is set at both levels.
*/
@JvmName("vluukxnwawopqknw")
public suspend fun encoding(`value`: Output) {
this.encoding = value
}
/**
* @param value If the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as the column field name and is used as field name in queries.
*/
@JvmName("vvcrotccphufgcjj")
public suspend fun fieldName(`value`: Output) {
this.fieldName = value
}
/**
* @param value If this is set, only the latest version of value in this column are exposed. 'onlyReadLatest' can also be set at the column family level. However, the setting at this level takes precedence if 'onlyReadLatest' is set at both levels.
*/
@JvmName("xlrxhtksrefterlg")
public suspend fun onlyReadLatest(`value`: Output) {
this.onlyReadLatest = value
}
/**
* @param value Qualifier of the column. Columns in the parent column family that has this exact qualifier are exposed as . field. If the qualifier is valid UTF-8 string, it can be specified in the qualifierString field. Otherwise, a base-64 encoded value must be set to qualifierEncoded. The column field name is the same as the column qualifier. However, if the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as fieldName.
*/
@JvmName("hkhypjcegnyqtvup")
public suspend fun qualifierEncoded(`value`: Output) {
this.qualifierEncoded = value
}
/**
* @param value Qualifier string.
*/
@JvmName("fjqobunaimbfkshi")
public suspend fun qualifierString(`value`: Output) {
this.qualifierString = value
}
/**
* @param value The type to convert the value in cells of this column. The values are expected to be encoded using HBase Bytes.toBytes function when using the BINARY encoding value. Following BigQuery types are allowed (case-sensitive): "BYTES", "STRING", "INTEGER", "FLOAT", "BOOLEAN", "JSON", Default type is "BYTES". 'type' can also be set at the column family level. However, the setting at this level takes precedence if 'type' is set at both levels.
*/
@JvmName("cavvdrmuubccwokd")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The encoding of the values when the type is not STRING. Acceptable encoding values are: TEXT - indicates values are alphanumeric text strings. BINARY - indicates values are encoded using HBase Bytes.toBytes family of functions. 'encoding' can also be set at the column family level. However, the setting at this level takes precedence if 'encoding' is set at both levels.
*/
@JvmName("lsumerufgmfpuxay")
public suspend fun encoding(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encoding = mapped
}
/**
* @param value If the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as the column field name and is used as field name in queries.
*/
@JvmName("jnejamwwaarditwc")
public suspend fun fieldName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldName = mapped
}
/**
* @param value If this is set, only the latest version of value in this column are exposed. 'onlyReadLatest' can also be set at the column family level. However, the setting at this level takes precedence if 'onlyReadLatest' is set at both levels.
*/
@JvmName("rvsadrsmvvpsidiv")
public suspend fun onlyReadLatest(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.onlyReadLatest = mapped
}
/**
* @param value Qualifier of the column. Columns in the parent column family that has this exact qualifier are exposed as . field. If the qualifier is valid UTF-8 string, it can be specified in the qualifierString field. Otherwise, a base-64 encoded value must be set to qualifierEncoded. The column field name is the same as the column qualifier. However, if the qualifier is not a valid BigQuery field identifier i.e. does not match [a-zA-Z][a-zA-Z0-9_]*, a valid identifier must be provided as fieldName.
*/
@JvmName("gggogjtukqqxldmb")
public suspend fun qualifierEncoded(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.qualifierEncoded = mapped
}
/**
* @param value Qualifier string.
*/
@JvmName("xdcvpiipwpwtofdd")
public suspend fun qualifierString(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.qualifierString = mapped
}
/**
* @param value The type to convert the value in cells of this column. The values are expected to be encoded using HBase Bytes.toBytes function when using the BINARY encoding value. Following BigQuery types are allowed (case-sensitive): "BYTES", "STRING", "INTEGER", "FLOAT", "BOOLEAN", "JSON", Default type is "BYTES". 'type' can also be set at the column family level. However, the setting at this level takes precedence if 'type' is set at both levels.
*/
@JvmName("tnsvbeninvhuseip")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs =
TableExternalDataConfigurationBigtableOptionsColumnFamilyColumnArgs(
encoding = encoding,
fieldName = fieldName,
onlyReadLatest = onlyReadLatest,
qualifierEncoded = qualifierEncoded,
qualifierString = qualifierString,
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy