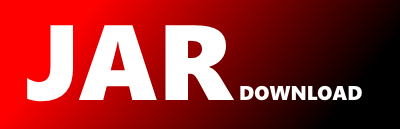
com.pulumi.gcp.cloudbuildv2.kotlin.inputs.ConnectionBitbucketDataCenterConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudbuildv2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudbuildv2.inputs.ConnectionBitbucketDataCenterConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property authorizerCredential Required. A http access token with the `REPO_ADMIN` scope access.
* Structure is documented below.
* @property hostUri The URI of the Bitbucket Data Center host this connection is for.
* @property readAuthorizerCredential Required. A http access token with the `REPO_READ` access.
* Structure is documented below.
* @property serverVersion (Output)
* Output only. Version of the Bitbucket Data Center running on the `host_uri`.
* @property serviceDirectoryConfig Configuration for using Service Directory to privately connect to a Bitbucket Data Center. This should only be set if the Bitbucket Data Center is hosted on-premises and not reachable by public internet. If this field is left empty, calls to the Bitbucket Data Center will be made over the public internet.
* Structure is documented below.
* @property sslCa SSL certificate to use for requests to the Bitbucket Data Center.
* @property webhookSecretSecretVersion Required. Immutable. SecretManager resource containing the webhook secret used to verify webhook events, formatted as `projects/*/secrets/*/versions/*`.
* */*/*/
*/
public data class ConnectionBitbucketDataCenterConfigArgs(
public val authorizerCredential: Output,
public val hostUri: Output,
public val readAuthorizerCredential: Output,
public val serverVersion: Output? = null,
public val serviceDirectoryConfig: Output? = null,
public val sslCa: Output? = null,
public val webhookSecretSecretVersion: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudbuildv2.inputs.ConnectionBitbucketDataCenterConfigArgs = com.pulumi.gcp.cloudbuildv2.inputs.ConnectionBitbucketDataCenterConfigArgs.builder()
.authorizerCredential(
authorizerCredential.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.hostUri(hostUri.applyValue({ args0 -> args0 }))
.readAuthorizerCredential(
readAuthorizerCredential.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.serverVersion(serverVersion?.applyValue({ args0 -> args0 }))
.serviceDirectoryConfig(
serviceDirectoryConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sslCa(sslCa?.applyValue({ args0 -> args0 }))
.webhookSecretSecretVersion(webhookSecretSecretVersion.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectionBitbucketDataCenterConfigArgs].
*/
@PulumiTagMarker
public class ConnectionBitbucketDataCenterConfigArgsBuilder internal constructor() {
private var authorizerCredential:
Output? = null
private var hostUri: Output? = null
private var readAuthorizerCredential:
Output? = null
private var serverVersion: Output? = null
private var serviceDirectoryConfig:
Output? = null
private var sslCa: Output? = null
private var webhookSecretSecretVersion: Output? = null
/**
* @param value Required. A http access token with the `REPO_ADMIN` scope access.
* Structure is documented below.
*/
@JvmName("vfqsbvdimcbfycdn")
public suspend fun authorizerCredential(`value`: Output) {
this.authorizerCredential = value
}
/**
* @param value The URI of the Bitbucket Data Center host this connection is for.
*/
@JvmName("fltnsxelwjaegube")
public suspend fun hostUri(`value`: Output) {
this.hostUri = value
}
/**
* @param value Required. A http access token with the `REPO_READ` access.
* Structure is documented below.
*/
@JvmName("rdpkqtnersccxajb")
public suspend fun readAuthorizerCredential(`value`: Output) {
this.readAuthorizerCredential = value
}
/**
* @param value (Output)
* Output only. Version of the Bitbucket Data Center running on the `host_uri`.
*/
@JvmName("aqfyxxnfhmjfxulk")
public suspend fun serverVersion(`value`: Output) {
this.serverVersion = value
}
/**
* @param value Configuration for using Service Directory to privately connect to a Bitbucket Data Center. This should only be set if the Bitbucket Data Center is hosted on-premises and not reachable by public internet. If this field is left empty, calls to the Bitbucket Data Center will be made over the public internet.
* Structure is documented below.
*/
@JvmName("ldipuroksmrhaowp")
public suspend fun serviceDirectoryConfig(`value`: Output) {
this.serviceDirectoryConfig = value
}
/**
* @param value SSL certificate to use for requests to the Bitbucket Data Center.
*/
@JvmName("skstnyvcywpcxhet")
public suspend fun sslCa(`value`: Output) {
this.sslCa = value
}
/**
* @param value Required. Immutable. SecretManager resource containing the webhook secret used to verify webhook events, formatted as `projects/*/secrets/*/versions/*`.
* */*/*/
*/
@JvmName("rpaohvnyckuihhvf")
public suspend fun webhookSecretSecretVersion(`value`: Output) {
this.webhookSecretSecretVersion = value
}
/**
* @param value Required. A http access token with the `REPO_ADMIN` scope access.
* Structure is documented below.
*/
@JvmName("wiavbuictvwgkgbe")
public suspend fun authorizerCredential(`value`: ConnectionBitbucketDataCenterConfigAuthorizerCredentialArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authorizerCredential = mapped
}
/**
* @param argument Required. A http access token with the `REPO_ADMIN` scope access.
* Structure is documented below.
*/
@JvmName("xlfscogfckstdgym")
public suspend fun authorizerCredential(argument: suspend ConnectionBitbucketDataCenterConfigAuthorizerCredentialArgsBuilder.() -> Unit) {
val toBeMapped =
ConnectionBitbucketDataCenterConfigAuthorizerCredentialArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.authorizerCredential = mapped
}
/**
* @param value The URI of the Bitbucket Data Center host this connection is for.
*/
@JvmName("rtowwlsyfsikwpog")
public suspend fun hostUri(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hostUri = mapped
}
/**
* @param value Required. A http access token with the `REPO_READ` access.
* Structure is documented below.
*/
@JvmName("cwhymtfdtcfbryqo")
public suspend fun readAuthorizerCredential(`value`: ConnectionBitbucketDataCenterConfigReadAuthorizerCredentialArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.readAuthorizerCredential = mapped
}
/**
* @param argument Required. A http access token with the `REPO_READ` access.
* Structure is documented below.
*/
@JvmName("xqcpjxheefomgrtw")
public suspend fun readAuthorizerCredential(argument: suspend ConnectionBitbucketDataCenterConfigReadAuthorizerCredentialArgsBuilder.() -> Unit) {
val toBeMapped =
ConnectionBitbucketDataCenterConfigReadAuthorizerCredentialArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.readAuthorizerCredential = mapped
}
/**
* @param value (Output)
* Output only. Version of the Bitbucket Data Center running on the `host_uri`.
*/
@JvmName("uruasgvolouplmpq")
public suspend fun serverVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverVersion = mapped
}
/**
* @param value Configuration for using Service Directory to privately connect to a Bitbucket Data Center. This should only be set if the Bitbucket Data Center is hosted on-premises and not reachable by public internet. If this field is left empty, calls to the Bitbucket Data Center will be made over the public internet.
* Structure is documented below.
*/
@JvmName("dguyrywcopjedlgt")
public suspend fun serviceDirectoryConfig(`value`: ConnectionBitbucketDataCenterConfigServiceDirectoryConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceDirectoryConfig = mapped
}
/**
* @param argument Configuration for using Service Directory to privately connect to a Bitbucket Data Center. This should only be set if the Bitbucket Data Center is hosted on-premises and not reachable by public internet. If this field is left empty, calls to the Bitbucket Data Center will be made over the public internet.
* Structure is documented below.
*/
@JvmName("myvqyjjrcoonojbo")
public suspend fun serviceDirectoryConfig(argument: suspend ConnectionBitbucketDataCenterConfigServiceDirectoryConfigArgsBuilder.() -> Unit) {
val toBeMapped =
ConnectionBitbucketDataCenterConfigServiceDirectoryConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.serviceDirectoryConfig = mapped
}
/**
* @param value SSL certificate to use for requests to the Bitbucket Data Center.
*/
@JvmName("tnbafspmydieaskj")
public suspend fun sslCa(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslCa = mapped
}
/**
* @param value Required. Immutable. SecretManager resource containing the webhook secret used to verify webhook events, formatted as `projects/*/secrets/*/versions/*`.
* */*/*/
*/
@JvmName("howctkygnyfrlxfr")
public suspend fun webhookSecretSecretVersion(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.webhookSecretSecretVersion = mapped
}
internal fun build(): ConnectionBitbucketDataCenterConfigArgs =
ConnectionBitbucketDataCenterConfigArgs(
authorizerCredential = authorizerCredential ?: throw
PulumiNullFieldException("authorizerCredential"),
hostUri = hostUri ?: throw PulumiNullFieldException("hostUri"),
readAuthorizerCredential = readAuthorizerCredential ?: throw
PulumiNullFieldException("readAuthorizerCredential"),
serverVersion = serverVersion,
serviceDirectoryConfig = serviceDirectoryConfig,
sslCa = sslCa,
webhookSecretSecretVersion = webhookSecretSecretVersion ?: throw
PulumiNullFieldException("webhookSecretSecretVersion"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy