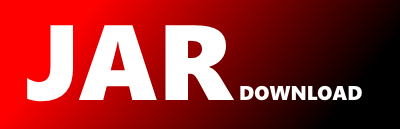
com.pulumi.gcp.clouddeploy.kotlin.Target.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.clouddeploy.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetAnthosCluster
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetAssociatedEntity
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetCustomTarget
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetExecutionConfig
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetGke
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetMultiTarget
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetRun
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetAnthosCluster.Companion.toKotlin as targetAnthosClusterToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetAssociatedEntity.Companion.toKotlin as targetAssociatedEntityToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetCustomTarget.Companion.toKotlin as targetCustomTargetToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetExecutionConfig.Companion.toKotlin as targetExecutionConfigToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetGke.Companion.toKotlin as targetGkeToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetMultiTarget.Companion.toKotlin as targetMultiTargetToKotlin
import com.pulumi.gcp.clouddeploy.kotlin.outputs.TargetRun.Companion.toKotlin as targetRunToKotlin
/**
* Builder for [Target].
*/
@PulumiTagMarker
public class TargetResourceBuilder internal constructor() {
public var name: String? = null
public var args: TargetArgs = TargetArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TargetArgsBuilder.() -> Unit) {
val builder = TargetArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Target {
val builtJavaResource = com.pulumi.gcp.clouddeploy.Target(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Target(builtJavaResource)
}
}
/**
* The Cloud Deploy `Target` resource
* ## Example Usage
* ### Multi_target
* tests creating and updating a multi-target
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const primary = new gcp.clouddeploy.Target("primary", {
* location: "us-west1",
* name: "target",
* deployParameters: {},
* description: "multi-target description",
* executionConfigs: [{
* usages: [
* "RENDER",
* "DEPLOY",
* ],
* executionTimeout: "3600s",
* }],
* multiTarget: {
* targetIds: [
* "1",
* "2",
* ],
* },
* project: "my-project-name",
* requireApproval: false,
* annotations: {
* my_first_annotation: "example-annotation-1",
* my_second_annotation: "example-annotation-2",
* },
* labels: {
* my_first_label: "example-label-1",
* my_second_label: "example-label-2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* primary = gcp.clouddeploy.Target("primary",
* location="us-west1",
* name="target",
* deploy_parameters={},
* description="multi-target description",
* execution_configs=[{
* "usages": [
* "RENDER",
* "DEPLOY",
* ],
* "execution_timeout": "3600s",
* }],
* multi_target={
* "target_ids": [
* "1",
* "2",
* ],
* },
* project="my-project-name",
* require_approval=False,
* annotations={
* "my_first_annotation": "example-annotation-1",
* "my_second_annotation": "example-annotation-2",
* },
* labels={
* "my_first_label": "example-label-1",
* "my_second_label": "example-label-2",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Gcp.CloudDeploy.Target("primary", new()
* {
* Location = "us-west1",
* Name = "target",
* DeployParameters = null,
* Description = "multi-target description",
* ExecutionConfigs = new[]
* {
* new Gcp.CloudDeploy.Inputs.TargetExecutionConfigArgs
* {
* Usages = new[]
* {
* "RENDER",
* "DEPLOY",
* },
* ExecutionTimeout = "3600s",
* },
* },
* MultiTarget = new Gcp.CloudDeploy.Inputs.TargetMultiTargetArgs
* {
* TargetIds = new[]
* {
* "1",
* "2",
* },
* },
* Project = "my-project-name",
* RequireApproval = false,
* Annotations =
* {
* { "my_first_annotation", "example-annotation-1" },
* { "my_second_annotation", "example-annotation-2" },
* },
* Labels =
* {
* { "my_first_label", "example-label-1" },
* { "my_second_label", "example-label-2" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/clouddeploy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := clouddeploy.NewTarget(ctx, "primary", &clouddeploy.TargetArgs{
* Location: pulumi.String("us-west1"),
* Name: pulumi.String("target"),
* DeployParameters: pulumi.StringMap{},
* Description: pulumi.String("multi-target description"),
* ExecutionConfigs: clouddeploy.TargetExecutionConfigArray{
* &clouddeploy.TargetExecutionConfigArgs{
* Usages: pulumi.StringArray{
* pulumi.String("RENDER"),
* pulumi.String("DEPLOY"),
* },
* ExecutionTimeout: pulumi.String("3600s"),
* },
* },
* MultiTarget: &clouddeploy.TargetMultiTargetArgs{
* TargetIds: pulumi.StringArray{
* pulumi.String("1"),
* pulumi.String("2"),
* },
* },
* Project: pulumi.String("my-project-name"),
* RequireApproval: pulumi.Bool(false),
* Annotations: pulumi.StringMap{
* "my_first_annotation": pulumi.String("example-annotation-1"),
* "my_second_annotation": pulumi.String("example-annotation-2"),
* },
* Labels: pulumi.StringMap{
* "my_first_label": pulumi.String("example-label-1"),
* "my_second_label": pulumi.String("example-label-2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.clouddeploy.Target;
* import com.pulumi.gcp.clouddeploy.TargetArgs;
* import com.pulumi.gcp.clouddeploy.inputs.TargetExecutionConfigArgs;
* import com.pulumi.gcp.clouddeploy.inputs.TargetMultiTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new Target("primary", TargetArgs.builder()
* .location("us-west1")
* .name("target")
* .deployParameters()
* .description("multi-target description")
* .executionConfigs(TargetExecutionConfigArgs.builder()
* .usages(
* "RENDER",
* "DEPLOY")
* .executionTimeout("3600s")
* .build())
* .multiTarget(TargetMultiTargetArgs.builder()
* .targetIds(
* "1",
* "2")
* .build())
* .project("my-project-name")
* .requireApproval(false)
* .annotations(Map.ofEntries(
* Map.entry("my_first_annotation", "example-annotation-1"),
* Map.entry("my_second_annotation", "example-annotation-2")
* ))
* .labels(Map.ofEntries(
* Map.entry("my_first_label", "example-label-1"),
* Map.entry("my_second_label", "example-label-2")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:clouddeploy:Target
* properties:
* location: us-west1
* name: target
* deployParameters: {}
* description: multi-target description
* executionConfigs:
* - usages:
* - RENDER
* - DEPLOY
* executionTimeout: 3600s
* multiTarget:
* targetIds:
* - '1'
* - '2'
* project: my-project-name
* requireApproval: false
* annotations:
* my_first_annotation: example-annotation-1
* my_second_annotation: example-annotation-2
* labels:
* my_first_label: example-label-1
* my_second_label: example-label-2
* ```
*
* ### Run_target
* tests creating and updating a cloud run target
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const primary = new gcp.clouddeploy.Target("primary", {
* location: "us-west1",
* name: "target",
* deployParameters: {},
* description: "basic description",
* executionConfigs: [{
* usages: [
* "RENDER",
* "DEPLOY",
* ],
* executionTimeout: "3600s",
* }],
* project: "my-project-name",
* requireApproval: false,
* run: {
* location: "projects/my-project-name/locations/us-west1",
* },
* annotations: {
* my_first_annotation: "example-annotation-1",
* my_second_annotation: "example-annotation-2",
* },
* labels: {
* my_first_label: "example-label-1",
* my_second_label: "example-label-2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* primary = gcp.clouddeploy.Target("primary",
* location="us-west1",
* name="target",
* deploy_parameters={},
* description="basic description",
* execution_configs=[{
* "usages": [
* "RENDER",
* "DEPLOY",
* ],
* "execution_timeout": "3600s",
* }],
* project="my-project-name",
* require_approval=False,
* run={
* "location": "projects/my-project-name/locations/us-west1",
* },
* annotations={
* "my_first_annotation": "example-annotation-1",
* "my_second_annotation": "example-annotation-2",
* },
* labels={
* "my_first_label": "example-label-1",
* "my_second_label": "example-label-2",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Gcp.CloudDeploy.Target("primary", new()
* {
* Location = "us-west1",
* Name = "target",
* DeployParameters = null,
* Description = "basic description",
* ExecutionConfigs = new[]
* {
* new Gcp.CloudDeploy.Inputs.TargetExecutionConfigArgs
* {
* Usages = new[]
* {
* "RENDER",
* "DEPLOY",
* },
* ExecutionTimeout = "3600s",
* },
* },
* Project = "my-project-name",
* RequireApproval = false,
* Run = new Gcp.CloudDeploy.Inputs.TargetRunArgs
* {
* Location = "projects/my-project-name/locations/us-west1",
* },
* Annotations =
* {
* { "my_first_annotation", "example-annotation-1" },
* { "my_second_annotation", "example-annotation-2" },
* },
* Labels =
* {
* { "my_first_label", "example-label-1" },
* { "my_second_label", "example-label-2" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/clouddeploy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := clouddeploy.NewTarget(ctx, "primary", &clouddeploy.TargetArgs{
* Location: pulumi.String("us-west1"),
* Name: pulumi.String("target"),
* DeployParameters: pulumi.StringMap{},
* Description: pulumi.String("basic description"),
* ExecutionConfigs: clouddeploy.TargetExecutionConfigArray{
* &clouddeploy.TargetExecutionConfigArgs{
* Usages: pulumi.StringArray{
* pulumi.String("RENDER"),
* pulumi.String("DEPLOY"),
* },
* ExecutionTimeout: pulumi.String("3600s"),
* },
* },
* Project: pulumi.String("my-project-name"),
* RequireApproval: pulumi.Bool(false),
* Run: &clouddeploy.TargetRunArgs{
* Location: pulumi.String("projects/my-project-name/locations/us-west1"),
* },
* Annotations: pulumi.StringMap{
* "my_first_annotation": pulumi.String("example-annotation-1"),
* "my_second_annotation": pulumi.String("example-annotation-2"),
* },
* Labels: pulumi.StringMap{
* "my_first_label": pulumi.String("example-label-1"),
* "my_second_label": pulumi.String("example-label-2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.clouddeploy.Target;
* import com.pulumi.gcp.clouddeploy.TargetArgs;
* import com.pulumi.gcp.clouddeploy.inputs.TargetExecutionConfigArgs;
* import com.pulumi.gcp.clouddeploy.inputs.TargetRunArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new Target("primary", TargetArgs.builder()
* .location("us-west1")
* .name("target")
* .deployParameters()
* .description("basic description")
* .executionConfigs(TargetExecutionConfigArgs.builder()
* .usages(
* "RENDER",
* "DEPLOY")
* .executionTimeout("3600s")
* .build())
* .project("my-project-name")
* .requireApproval(false)
* .run(TargetRunArgs.builder()
* .location("projects/my-project-name/locations/us-west1")
* .build())
* .annotations(Map.ofEntries(
* Map.entry("my_first_annotation", "example-annotation-1"),
* Map.entry("my_second_annotation", "example-annotation-2")
* ))
* .labels(Map.ofEntries(
* Map.entry("my_first_label", "example-label-1"),
* Map.entry("my_second_label", "example-label-2")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:clouddeploy:Target
* properties:
* location: us-west1
* name: target
* deployParameters: {}
* description: basic description
* executionConfigs:
* - usages:
* - RENDER
* - DEPLOY
* executionTimeout: 3600s
* project: my-project-name
* requireApproval: false
* run:
* location: projects/my-project-name/locations/us-west1
* annotations:
* my_first_annotation: example-annotation-1
* my_second_annotation: example-annotation-2
* labels:
* my_first_label: example-label-1
* my_second_label: example-label-2
* ```
*
* ### Target
* Creates a basic Cloud Deploy target
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const primary = new gcp.clouddeploy.Target("primary", {
* location: "us-west1",
* name: "target",
* deployParameters: {
* deployParameterKey: "deployParameterValue",
* },
* description: "basic description",
* gke: {
* cluster: "projects/my-project-name/locations/us-west1/clusters/example-cluster-name",
* },
* project: "my-project-name",
* requireApproval: false,
* annotations: {
* my_first_annotation: "example-annotation-1",
* my_second_annotation: "example-annotation-2",
* },
* labels: {
* my_first_label: "example-label-1",
* my_second_label: "example-label-2",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* primary = gcp.clouddeploy.Target("primary",
* location="us-west1",
* name="target",
* deploy_parameters={
* "deployParameterKey": "deployParameterValue",
* },
* description="basic description",
* gke={
* "cluster": "projects/my-project-name/locations/us-west1/clusters/example-cluster-name",
* },
* project="my-project-name",
* require_approval=False,
* annotations={
* "my_first_annotation": "example-annotation-1",
* "my_second_annotation": "example-annotation-2",
* },
* labels={
* "my_first_label": "example-label-1",
* "my_second_label": "example-label-2",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Gcp.CloudDeploy.Target("primary", new()
* {
* Location = "us-west1",
* Name = "target",
* DeployParameters =
* {
* { "deployParameterKey", "deployParameterValue" },
* },
* Description = "basic description",
* Gke = new Gcp.CloudDeploy.Inputs.TargetGkeArgs
* {
* Cluster = "projects/my-project-name/locations/us-west1/clusters/example-cluster-name",
* },
* Project = "my-project-name",
* RequireApproval = false,
* Annotations =
* {
* { "my_first_annotation", "example-annotation-1" },
* { "my_second_annotation", "example-annotation-2" },
* },
* Labels =
* {
* { "my_first_label", "example-label-1" },
* { "my_second_label", "example-label-2" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/clouddeploy"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := clouddeploy.NewTarget(ctx, "primary", &clouddeploy.TargetArgs{
* Location: pulumi.String("us-west1"),
* Name: pulumi.String("target"),
* DeployParameters: pulumi.StringMap{
* "deployParameterKey": pulumi.String("deployParameterValue"),
* },
* Description: pulumi.String("basic description"),
* Gke: &clouddeploy.TargetGkeArgs{
* Cluster: pulumi.String("projects/my-project-name/locations/us-west1/clusters/example-cluster-name"),
* },
* Project: pulumi.String("my-project-name"),
* RequireApproval: pulumi.Bool(false),
* Annotations: pulumi.StringMap{
* "my_first_annotation": pulumi.String("example-annotation-1"),
* "my_second_annotation": pulumi.String("example-annotation-2"),
* },
* Labels: pulumi.StringMap{
* "my_first_label": pulumi.String("example-label-1"),
* "my_second_label": pulumi.String("example-label-2"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.clouddeploy.Target;
* import com.pulumi.gcp.clouddeploy.TargetArgs;
* import com.pulumi.gcp.clouddeploy.inputs.TargetGkeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new Target("primary", TargetArgs.builder()
* .location("us-west1")
* .name("target")
* .deployParameters(Map.of("deployParameterKey", "deployParameterValue"))
* .description("basic description")
* .gke(TargetGkeArgs.builder()
* .cluster("projects/my-project-name/locations/us-west1/clusters/example-cluster-name")
* .build())
* .project("my-project-name")
* .requireApproval(false)
* .annotations(Map.ofEntries(
* Map.entry("my_first_annotation", "example-annotation-1"),
* Map.entry("my_second_annotation", "example-annotation-2")
* ))
* .labels(Map.ofEntries(
* Map.entry("my_first_label", "example-label-1"),
* Map.entry("my_second_label", "example-label-2")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:clouddeploy:Target
* properties:
* location: us-west1
* name: target
* deployParameters:
* deployParameterKey: deployParameterValue
* description: basic description
* gke:
* cluster: projects/my-project-name/locations/us-west1/clusters/example-cluster-name
* project: my-project-name
* requireApproval: false
* annotations:
* my_first_annotation: example-annotation-1
* my_second_annotation: example-annotation-2
* labels:
* my_first_label: example-label-1
* my_second_label: example-label-2
* ```
*
* ## Import
* Target can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/targets/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Target can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:clouddeploy/target:Target default projects/{{project}}/locations/{{location}}/targets/{{name}}
* ```
* ```sh
* $ pulumi import gcp:clouddeploy/target:Target default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:clouddeploy/target:Target default {{location}}/{{name}}
* ```
*/
public class Target internal constructor(
override val javaResource: com.pulumi.gcp.clouddeploy.Target,
) : KotlinCustomResource(javaResource, TargetMapper) {
/**
* Optional. User annotations. These attributes can only be set and used by the user, and not by Google Cloud Deploy. See https://google.aip.dev/128#annotations for more details such as format and size limitations.
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
*/
public val annotations: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy