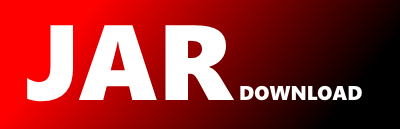
com.pulumi.gcp.cloudtasks.kotlin.inputs.QueueHttpTargetUriOverrideArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudtasks.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudtasks.inputs.QueueHttpTargetUriOverrideArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property host Host override.
* When specified, replaces the host part of the task URL.
* For example, if the task URL is "https://www.google.com", and host value
* is set to "example.net", the overridden URI will be changed to "https://example.net".
* Host value cannot be an empty string (INVALID_ARGUMENT).
* @property pathOverride URI path.
* When specified, replaces the existing path of the task URL.
* Setting the path value to an empty string clears the URI path segment.
* Structure is documented below.
* @property port Port override.
* When specified, replaces the port part of the task URI.
* For instance, for a URI http://www.google.com/foo and port=123, the overridden URI becomes http://www.google.com:123/foo.
* Note that the port value must be a positive integer.
* Setting the port to 0 (Zero) clears the URI port.
* @property queryOverride URI query.
* When specified, replaces the query part of the task URI. Setting the query value to an empty string clears the URI query segment.
* Structure is documented below.
* @property scheme Scheme override.
* When specified, the task URI scheme is replaced by the provided value (HTTP or HTTPS).
* Possible values are: `HTTP`, `HTTPS`.
* @property uriOverrideEnforceMode URI Override Enforce Mode
* When specified, determines the Target UriOverride mode. If not specified, it defaults to ALWAYS.
* Possible values are: `ALWAYS`, `IF_NOT_EXISTS`.
*/
public data class QueueHttpTargetUriOverrideArgs(
public val host: Output? = null,
public val pathOverride: Output? = null,
public val port: Output? = null,
public val queryOverride: Output? = null,
public val scheme: Output? = null,
public val uriOverrideEnforceMode: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudtasks.inputs.QueueHttpTargetUriOverrideArgs =
com.pulumi.gcp.cloudtasks.inputs.QueueHttpTargetUriOverrideArgs.builder()
.host(host?.applyValue({ args0 -> args0 }))
.pathOverride(pathOverride?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.port(port?.applyValue({ args0 -> args0 }))
.queryOverride(queryOverride?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scheme(scheme?.applyValue({ args0 -> args0 }))
.uriOverrideEnforceMode(uriOverrideEnforceMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [QueueHttpTargetUriOverrideArgs].
*/
@PulumiTagMarker
public class QueueHttpTargetUriOverrideArgsBuilder internal constructor() {
private var host: Output? = null
private var pathOverride: Output? = null
private var port: Output? = null
private var queryOverride: Output? = null
private var scheme: Output? = null
private var uriOverrideEnforceMode: Output? = null
/**
* @param value Host override.
* When specified, replaces the host part of the task URL.
* For example, if the task URL is "https://www.google.com", and host value
* is set to "example.net", the overridden URI will be changed to "https://example.net".
* Host value cannot be an empty string (INVALID_ARGUMENT).
*/
@JvmName("vurwoxfwoxmfbmfn")
public suspend fun host(`value`: Output) {
this.host = value
}
/**
* @param value URI path.
* When specified, replaces the existing path of the task URL.
* Setting the path value to an empty string clears the URI path segment.
* Structure is documented below.
*/
@JvmName("qimvdhmjosqngsem")
public suspend fun pathOverride(`value`: Output) {
this.pathOverride = value
}
/**
* @param value Port override.
* When specified, replaces the port part of the task URI.
* For instance, for a URI http://www.google.com/foo and port=123, the overridden URI becomes http://www.google.com:123/foo.
* Note that the port value must be a positive integer.
* Setting the port to 0 (Zero) clears the URI port.
*/
@JvmName("hromclgquhtqyvpf")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value URI query.
* When specified, replaces the query part of the task URI. Setting the query value to an empty string clears the URI query segment.
* Structure is documented below.
*/
@JvmName("urhxafugxkdpodan")
public suspend fun queryOverride(`value`: Output) {
this.queryOverride = value
}
/**
* @param value Scheme override.
* When specified, the task URI scheme is replaced by the provided value (HTTP or HTTPS).
* Possible values are: `HTTP`, `HTTPS`.
*/
@JvmName("eomavhfcpnvhbagi")
public suspend fun scheme(`value`: Output) {
this.scheme = value
}
/**
* @param value URI Override Enforce Mode
* When specified, determines the Target UriOverride mode. If not specified, it defaults to ALWAYS.
* Possible values are: `ALWAYS`, `IF_NOT_EXISTS`.
*/
@JvmName("cduaodfuwkyleyto")
public suspend fun uriOverrideEnforceMode(`value`: Output) {
this.uriOverrideEnforceMode = value
}
/**
* @param value Host override.
* When specified, replaces the host part of the task URL.
* For example, if the task URL is "https://www.google.com", and host value
* is set to "example.net", the overridden URI will be changed to "https://example.net".
* Host value cannot be an empty string (INVALID_ARGUMENT).
*/
@JvmName("wkgyuvmtrfmpvmhj")
public suspend fun host(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.host = mapped
}
/**
* @param value URI path.
* When specified, replaces the existing path of the task URL.
* Setting the path value to an empty string clears the URI path segment.
* Structure is documented below.
*/
@JvmName("xlxpjjdalrkkxyxb")
public suspend fun pathOverride(`value`: QueueHttpTargetUriOverridePathOverrideArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pathOverride = mapped
}
/**
* @param argument URI path.
* When specified, replaces the existing path of the task URL.
* Setting the path value to an empty string clears the URI path segment.
* Structure is documented below.
*/
@JvmName("cggirdlvlbemagld")
public suspend fun pathOverride(argument: suspend QueueHttpTargetUriOverridePathOverrideArgsBuilder.() -> Unit) {
val toBeMapped = QueueHttpTargetUriOverridePathOverrideArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pathOverride = mapped
}
/**
* @param value Port override.
* When specified, replaces the port part of the task URI.
* For instance, for a URI http://www.google.com/foo and port=123, the overridden URI becomes http://www.google.com:123/foo.
* Note that the port value must be a positive integer.
* Setting the port to 0 (Zero) clears the URI port.
*/
@JvmName("rhqlgeijoiqylaaq")
public suspend fun port(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value URI query.
* When specified, replaces the query part of the task URI. Setting the query value to an empty string clears the URI query segment.
* Structure is documented below.
*/
@JvmName("rftgwgfcmcvylyxu")
public suspend fun queryOverride(`value`: QueueHttpTargetUriOverrideQueryOverrideArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryOverride = mapped
}
/**
* @param argument URI query.
* When specified, replaces the query part of the task URI. Setting the query value to an empty string clears the URI query segment.
* Structure is documented below.
*/
@JvmName("gxjlfxoluvxyjdng")
public suspend fun queryOverride(argument: suspend QueueHttpTargetUriOverrideQueryOverrideArgsBuilder.() -> Unit) {
val toBeMapped = QueueHttpTargetUriOverrideQueryOverrideArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.queryOverride = mapped
}
/**
* @param value Scheme override.
* When specified, the task URI scheme is replaced by the provided value (HTTP or HTTPS).
* Possible values are: `HTTP`, `HTTPS`.
*/
@JvmName("ivmagbogabwfptnl")
public suspend fun scheme(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheme = mapped
}
/**
* @param value URI Override Enforce Mode
* When specified, determines the Target UriOverride mode. If not specified, it defaults to ALWAYS.
* Possible values are: `ALWAYS`, `IF_NOT_EXISTS`.
*/
@JvmName("gthtfjovsehfgtnb")
public suspend fun uriOverrideEnforceMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uriOverrideEnforceMode = mapped
}
internal fun build(): QueueHttpTargetUriOverrideArgs = QueueHttpTargetUriOverrideArgs(
host = host,
pathOverride = pathOverride,
port = port,
queryOverride = queryOverride,
scheme = scheme,
uriOverrideEnforceMode = uriOverrideEnforceMode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy