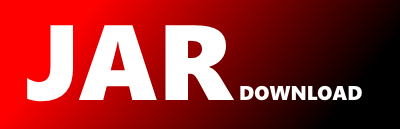
com.pulumi.gcp.compute.kotlin.RegionSslCertificate.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [RegionSslCertificate].
*/
@PulumiTagMarker
public class RegionSslCertificateResourceBuilder internal constructor() {
public var name: String? = null
public var args: RegionSslCertificateArgs = RegionSslCertificateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RegionSslCertificateArgsBuilder.() -> Unit) {
val builder = RegionSslCertificateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): RegionSslCertificate {
val builtJavaResource = com.pulumi.gcp.compute.RegionSslCertificate(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RegionSslCertificate(builtJavaResource)
}
}
/**
* A RegionSslCertificate resource, used for HTTPS load balancing. This resource
* provides a mechanism to upload an SSL key and certificate to
* the load balancer to serve secure connections from the user.
* To get more information about RegionSslCertificate, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/rest/v1/regionSslCertificates)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/load-balancing/docs/ssl-certificates)
* ## Example Usage
* ## Import
* RegionSslCertificate can be imported using any of these accepted formats:
* * `projects/{{project}}/regions/{{region}}/sslCertificates/{{name}}`
* * `{{project}}/{{region}}/{{name}}`
* * `{{region}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, RegionSslCertificate can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/regionSslCertificate:RegionSslCertificate default projects/{{project}}/regions/{{region}}/sslCertificates/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/regionSslCertificate:RegionSslCertificate default {{project}}/{{region}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/regionSslCertificate:RegionSslCertificate default {{region}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/regionSslCertificate:RegionSslCertificate default {{name}}
* ```
*/
public class RegionSslCertificate internal constructor(
override val javaResource: com.pulumi.gcp.compute.RegionSslCertificate,
) : KotlinCustomResource(javaResource, RegionSslCertificateMapper) {
/**
* The certificate in PEM format.
* The certificate chain must be no greater than 5 certs long.
* The chain must include at least one intermediate cert.
* **Note**: This property is sensitive and will not be displayed in the plan.
*/
public val certificate: Output
get() = javaResource.certificate().applyValue({ args0 -> args0 })
/**
* The unique identifier for the resource.
*/
public val certificateId: Output
get() = javaResource.certificateId().applyValue({ args0 -> args0 })
/**
* Creation timestamp in RFC3339 text format.
*/
public val creationTimestamp: Output
get() = javaResource.creationTimestamp().applyValue({ args0 -> args0 })
/**
* An optional description of this resource.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Expire time of the certificate in RFC3339 text format.
*/
public val expireTime: Output
get() = javaResource.expireTime().applyValue({ args0 -> args0 })
/**
* Name of the resource. Provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and match
* the regular expression `a-z?` which means the
* first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the last
* character, which cannot be a dash.
* These are in the same namespace as the managed SSL certificates.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Creates a unique name beginning with the
* specified prefix. Conflicts with `name`. Max length is 54 characters.
* Prefixes with lengths longer than 37 characters will use a shortened
* UUID that will be more prone to collisions.
* Resulting name for a `name_prefix` <= 37 characters:
* `name_prefix` + YYYYmmddHHSSssss + 8 digit incremental counter
* Resulting name for a `name_prefix` 38 - 54 characters:
* `name_prefix` + YYmmdd + 3 digit incremental counter
*/
public val namePrefix: Output
get() = javaResource.namePrefix().applyValue({ args0 -> args0 })
/**
* The write-only private key in PEM format.
* **Note**: This property is sensitive and will not be displayed in the plan.
* - - -
*/
public val privateKey: Output
get() = javaResource.privateKey().applyValue({ args0 -> args0 })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The Region in which the created regional ssl certificate should reside.
* If it is not provided, the provider region is used.
*/
public val region: Output
get() = javaResource.region().applyValue({ args0 -> args0 })
/**
* The URI of the created resource.
*/
public val selfLink: Output
get() = javaResource.selfLink().applyValue({ args0 -> args0 })
}
public object RegionSslCertificateMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.compute.RegionSslCertificate::class == javaResource::class
override fun map(javaResource: Resource): RegionSslCertificate = RegionSslCertificate(
javaResource
as com.pulumi.gcp.compute.RegionSslCertificate,
)
}
/**
* @see [RegionSslCertificate].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [RegionSslCertificate].
*/
public suspend fun regionSslCertificate(
name: String,
block: suspend RegionSslCertificateResourceBuilder.() -> Unit,
): RegionSslCertificate {
val builder = RegionSslCertificateResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [RegionSslCertificate].
* @param name The _unique_ name of the resulting resource.
*/
public fun regionSslCertificate(name: String): RegionSslCertificate {
val builder = RegionSslCertificateResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy