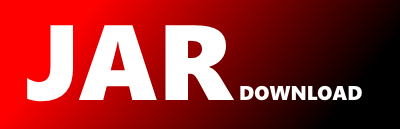
com.pulumi.gcp.compute.kotlin.inputs.RegionNetworkFirewallPolicyWithRulesRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionNetworkFirewallPolicyWithRulesRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property action The Action to perform when the client connection triggers the rule. Can currently be either
* "allow", "deny", "apply_security_profile_group" or "goto_next".
* @property description A description of the rule.
* @property direction The direction in which this rule applies. If unspecified an INGRESS rule is created.
* Possible values are: `INGRESS`, `EGRESS`.
* @property disabled Denotes whether the firewall policy rule is disabled. When set to true,
* the firewall policy rule is not enforced and traffic behaves as if it did
* not exist. If this is unspecified, the firewall policy rule will be
* enabled.
* @property enableLogging Denotes whether to enable logging for a particular rule.
* If logging is enabled, logs will be exported to the
* configured export destination in Stackdriver.
* @property match A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
* Structure is documented below.
* @property priority An integer indicating the priority of a rule in the list. The priority must be a value
* between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the
* highest priority and 2147483647 is the lowest priority.
* @property ruleName An optional name for the rule. This field is not a unique identifier
* and can be updated.
* @property securityProfileGroup A fully-qualified URL of a SecurityProfile resource instance.
* Example:
* https://networksecurity.googleapis.com/v1/projects/{project}/locations/{location}/securityProfileGroups/my-security-profile-group
* Must be specified if action is 'apply_security_profile_group'.
* @property targetSecureTags A list of secure tags that controls which instances the firewall rule
* applies to. If targetSecureTag
are specified, then the
* firewall rule applies only to instances in the VPC network that have one
* of those EFFECTIVE secure tags, if all the target_secure_tag are in
* INEFFECTIVE state, then this rule will be ignored.
* targetSecureTag
may not be set at the same time as
* targetServiceAccounts
.
* If neither targetServiceAccounts
nor
* targetSecureTag
are specified, the firewall rule applies
* to all instances on the specified network.
* Maximum number of target label tags allowed is 256.
* Structure is documented below.
* @property targetServiceAccounts A list of service accounts indicating the sets of
* instances that are applied with this rule.
* @property tlsInspect Boolean flag indicating if the traffic should be TLS decrypted.
* It can be set only if action = 'apply_security_profile_group' and cannot be set for other actions.
*/
public data class RegionNetworkFirewallPolicyWithRulesRuleArgs(
public val action: Output,
public val description: Output? = null,
public val direction: Output? = null,
public val disabled: Output? = null,
public val enableLogging: Output? = null,
public val match: Output,
public val priority: Output,
public val ruleName: Output? = null,
public val securityProfileGroup: Output? = null,
public val targetSecureTags: Output>? = null,
public val targetServiceAccounts: Output>? = null,
public val tlsInspect: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionNetworkFirewallPolicyWithRulesRuleArgs = com.pulumi.gcp.compute.inputs.RegionNetworkFirewallPolicyWithRulesRuleArgs.builder()
.action(action.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.direction(direction?.applyValue({ args0 -> args0 }))
.disabled(disabled?.applyValue({ args0 -> args0 }))
.enableLogging(enableLogging?.applyValue({ args0 -> args0 }))
.match(match.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.priority(priority.applyValue({ args0 -> args0 }))
.ruleName(ruleName?.applyValue({ args0 -> args0 }))
.securityProfileGroup(securityProfileGroup?.applyValue({ args0 -> args0 }))
.targetSecureTags(
targetSecureTags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.targetServiceAccounts(targetServiceAccounts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tlsInspect(tlsInspect?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionNetworkFirewallPolicyWithRulesRuleArgs].
*/
@PulumiTagMarker
public class RegionNetworkFirewallPolicyWithRulesRuleArgsBuilder internal constructor() {
private var action: Output? = null
private var description: Output? = null
private var direction: Output? = null
private var disabled: Output? = null
private var enableLogging: Output? = null
private var match: Output? = null
private var priority: Output? = null
private var ruleName: Output? = null
private var securityProfileGroup: Output? = null
private var targetSecureTags:
Output>? = null
private var targetServiceAccounts: Output>? = null
private var tlsInspect: Output? = null
/**
* @param value The Action to perform when the client connection triggers the rule. Can currently be either
* "allow", "deny", "apply_security_profile_group" or "goto_next".
*/
@JvmName("mqjkkrgfigtngacf")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value A description of the rule.
*/
@JvmName("hnrtivrljylmabui")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The direction in which this rule applies. If unspecified an INGRESS rule is created.
* Possible values are: `INGRESS`, `EGRESS`.
*/
@JvmName("evhiuupygypsnpro")
public suspend fun direction(`value`: Output) {
this.direction = value
}
/**
* @param value Denotes whether the firewall policy rule is disabled. When set to true,
* the firewall policy rule is not enforced and traffic behaves as if it did
* not exist. If this is unspecified, the firewall policy rule will be
* enabled.
*/
@JvmName("yjbwnbnovdlbwkqi")
public suspend fun disabled(`value`: Output) {
this.disabled = value
}
/**
* @param value Denotes whether to enable logging for a particular rule.
* If logging is enabled, logs will be exported to the
* configured export destination in Stackdriver.
*/
@JvmName("qendsaiskchwugqa")
public suspend fun enableLogging(`value`: Output) {
this.enableLogging = value
}
/**
* @param value A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
* Structure is documented below.
*/
@JvmName("mqdrgprjjkfqdrdn")
public suspend fun match(`value`: Output) {
this.match = value
}
/**
* @param value An integer indicating the priority of a rule in the list. The priority must be a value
* between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the
* highest priority and 2147483647 is the lowest priority.
*/
@JvmName("rnnfylkqcvpovwwf")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value An optional name for the rule. This field is not a unique identifier
* and can be updated.
*/
@JvmName("slphstqokweivoqq")
public suspend fun ruleName(`value`: Output) {
this.ruleName = value
}
/**
* @param value A fully-qualified URL of a SecurityProfile resource instance.
* Example:
* https://networksecurity.googleapis.com/v1/projects/{project}/locations/{location}/securityProfileGroups/my-security-profile-group
* Must be specified if action is 'apply_security_profile_group'.
*/
@JvmName("clvhxcowknqkwvgw")
public suspend fun securityProfileGroup(`value`: Output) {
this.securityProfileGroup = value
}
/**
* @param value A list of secure tags that controls which instances the firewall rule
* applies to. If targetSecureTag
are specified, then the
* firewall rule applies only to instances in the VPC network that have one
* of those EFFECTIVE secure tags, if all the target_secure_tag are in
* INEFFECTIVE state, then this rule will be ignored.
* targetSecureTag
may not be set at the same time as
* targetServiceAccounts
.
* If neither targetServiceAccounts
nor
* targetSecureTag
are specified, the firewall rule applies
* to all instances on the specified network.
* Maximum number of target label tags allowed is 256.
* Structure is documented below.
*/
@JvmName("purbgedvlkdroeke")
public suspend fun targetSecureTags(`value`: Output>) {
this.targetSecureTags = value
}
@JvmName("gbuekipbbypihvqv")
public suspend fun targetSecureTags(vararg values: Output) {
this.targetSecureTags = Output.all(values.asList())
}
/**
* @param values A list of secure tags that controls which instances the firewall rule
* applies to. If targetSecureTag
are specified, then the
* firewall rule applies only to instances in the VPC network that have one
* of those EFFECTIVE secure tags, if all the target_secure_tag are in
* INEFFECTIVE state, then this rule will be ignored.
* targetSecureTag
may not be set at the same time as
* targetServiceAccounts
.
* If neither targetServiceAccounts
nor
* targetSecureTag
are specified, the firewall rule applies
* to all instances on the specified network.
* Maximum number of target label tags allowed is 256.
* Structure is documented below.
*/
@JvmName("sxjvpecciofqdfcj")
public suspend fun targetSecureTags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy