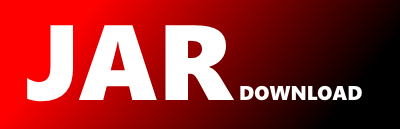
com.pulumi.gcp.compute.kotlin.outputs.FirewallPolicyWithRulesRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property action The Action to perform when the client connection triggers the rule. Can currently be either
* "allow", "deny", "apply_security_profile_group" or "goto_next".
* @property description A description of the rule.
* @property direction The direction in which this rule applies. If unspecified an INGRESS rule is created.
* Possible values are: `INGRESS`, `EGRESS`.
* @property disabled Denotes whether the firewall policy rule is disabled. When set to true,
* the firewall policy rule is not enforced and traffic behaves as if it did
* not exist. If this is unspecified, the firewall policy rule will be
* enabled.
* @property enableLogging Denotes whether to enable logging for a particular rule.
* If logging is enabled, logs will be exported to the
* configured export destination in Stackdriver.
* @property match A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
* Structure is documented below.
* @property priority An integer indicating the priority of a rule in the list. The priority must be a value
* between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the
* highest priority and 2147483647 is the lowest priority.
* @property ruleName An optional name for the rule. This field is not a unique identifier
* and can be updated.
* @property securityProfileGroup A fully-qualified URL of a SecurityProfile resource instance.
* Example:
* https://networksecurity.googleapis.com/v1/projects/{project}/locations/{location}/securityProfileGroups/my-security-profile-group
* Must be specified if action is 'apply_security_profile_group'.
* @property targetResources A list of network resource URLs to which this rule applies.
* This field allows you to control which network's VMs get
* this rule. If this field is left blank, all VMs
* within the organization will receive the rule.
* @property targetServiceAccounts A list of service accounts indicating the sets of
* instances that are applied with this rule.
* @property tlsInspect Boolean flag indicating if the traffic should be TLS decrypted.
* It can be set only if action = 'apply_security_profile_group' and cannot be set for other actions.
*/
public data class FirewallPolicyWithRulesRule(
public val action: String,
public val description: String? = null,
public val direction: String? = null,
public val disabled: Boolean? = null,
public val enableLogging: Boolean? = null,
public val match: FirewallPolicyWithRulesRuleMatch,
public val priority: Int,
public val ruleName: String? = null,
public val securityProfileGroup: String? = null,
public val targetResources: List? = null,
public val targetServiceAccounts: List? = null,
public val tlsInspect: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.FirewallPolicyWithRulesRule): FirewallPolicyWithRulesRule = FirewallPolicyWithRulesRule(
action = javaType.action(),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
direction = javaType.direction().map({ args0 -> args0 }).orElse(null),
disabled = javaType.disabled().map({ args0 -> args0 }).orElse(null),
enableLogging = javaType.enableLogging().map({ args0 -> args0 }).orElse(null),
match = javaType.match().let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.FirewallPolicyWithRulesRuleMatch.Companion.toKotlin(args0)
}),
priority = javaType.priority(),
ruleName = javaType.ruleName().map({ args0 -> args0 }).orElse(null),
securityProfileGroup = javaType.securityProfileGroup().map({ args0 -> args0 }).orElse(null),
targetResources = javaType.targetResources().map({ args0 -> args0 }),
targetServiceAccounts = javaType.targetServiceAccounts().map({ args0 -> args0 }),
tlsInspect = javaType.tlsInspect().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy