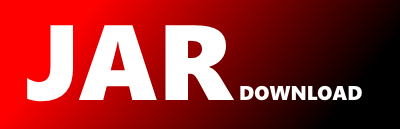
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getInstance.
* @property advancedMachineFeatures
* @property allowStoppingForUpdate
* @property attachedDisks List of disks attached to the instance. Structure is documented below.
* @property bootDisks The boot disk for the instance. Structure is documented below.
* @property canIpForward Whether sending and receiving of packets with non-matching source or destination IPs is allowed.
* @property confidentialInstanceConfigs
* @property cpuPlatform The CPU platform used by this instance.
* @property creationTimestamp Creation timestamp in RFC3339 text format.
* @property currentStatus The current status of the instance. This could be one of the following values: PROVISIONING, STAGING, RUNNING, STOPPING, SUSPENDING, SUSPENDED, REPAIRING, and TERMINATED. For more information about the status of the instance, see [Instance life cycle](https://cloud.google.com/compute/docs/instances/instance-life-cycle).
* @property deletionProtection Whether deletion protection is enabled on this instance.
* @property description A brief description of the resource.
* @property desiredStatus
* @property effectiveLabels
* @property enableDisplay Whether the instance has virtual displays enabled.
* @property guestAccelerators List of the type and count of accelerator cards attached to the instance. Structure is documented below.
* @property hostname
* @property id The provider-assigned unique ID for this managed resource.
* @property instanceId The server-assigned unique identifier of this instance.
* @property keyRevocationActionType Action to be taken when a customer's encryption key is revoked.
* @property labelFingerprint The unique fingerprint of the labels.
* @property labels A set of key/value label pairs assigned to the disk.
* @property machineType The machine type to create.
* @property metadata Metadata key/value pairs made available within the instance.
* @property metadataFingerprint The unique fingerprint of the metadata.
* @property metadataStartupScript
* @property minCpuPlatform The minimum CPU platform specified for the VM instance. Set to "AUTOMATIC" to remove a previously-set value.
* @property name
* @property networkInterfaces The networks attached to the instance. Structure is documented below.
* @property networkPerformanceConfigs The network performance configuration setting for the instance, if set. Structure is documented below.
* @property params
* @property partnerMetadata
* @property project
* @property pulumiLabels
* @property reservationAffinities
* @property resourcePolicies A list of self_links to resource policies attached to the selected `boot_disk`
* @property schedulings The scheduling strategy being used by the instance. Structure is documented below
* @property scratchDisks The scratch disks attached to the instance. Structure is documented below.
* @property selfLink The URI of the created resource.
* @property serviceAccounts The service account to attach to the instance. Structure is documented below.
* @property shieldedInstanceConfigs The shielded vm config being used by the instance. Structure is documented below.
* @property tags The list of tags attached to the instance.
* @property tagsFingerprint The unique fingerprint of the tags.
* @property zone
*/
public data class GetInstanceResult(
public val advancedMachineFeatures: List,
public val allowStoppingForUpdate: Boolean,
public val attachedDisks: List,
public val bootDisks: List,
public val canIpForward: Boolean,
public val confidentialInstanceConfigs: List,
public val cpuPlatform: String,
public val creationTimestamp: String,
public val currentStatus: String,
public val deletionProtection: Boolean,
public val description: String,
public val desiredStatus: String,
public val effectiveLabels: Map,
public val enableDisplay: Boolean,
public val guestAccelerators: List,
public val hostname: String,
public val id: String,
public val instanceId: String,
public val keyRevocationActionType: String,
public val labelFingerprint: String,
public val labels: Map,
public val machineType: String,
public val metadata: Map,
public val metadataFingerprint: String,
public val metadataStartupScript: String,
public val minCpuPlatform: String,
public val name: String? = null,
public val networkInterfaces: List,
public val networkPerformanceConfigs: List,
public val params: List,
public val partnerMetadata: Map,
public val project: String? = null,
public val pulumiLabels: Map,
public val reservationAffinities: List,
public val resourcePolicies: List,
public val schedulings: List,
public val scratchDisks: List,
public val selfLink: String? = null,
public val serviceAccounts: List,
public val shieldedInstanceConfigs: List,
public val tags: List,
public val tagsFingerprint: String,
public val zone: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetInstanceResult): GetInstanceResult = GetInstanceResult(
advancedMachineFeatures = javaType.advancedMachineFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceAdvancedMachineFeature.Companion.toKotlin(args0)
})
}),
allowStoppingForUpdate = javaType.allowStoppingForUpdate(),
attachedDisks = javaType.attachedDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceAttachedDisk.Companion.toKotlin(args0)
})
}),
bootDisks = javaType.bootDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceBootDisk.Companion.toKotlin(args0)
})
}),
canIpForward = javaType.canIpForward(),
confidentialInstanceConfigs = javaType.confidentialInstanceConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceConfidentialInstanceConfig.Companion.toKotlin(args0)
})
}),
cpuPlatform = javaType.cpuPlatform(),
creationTimestamp = javaType.creationTimestamp(),
currentStatus = javaType.currentStatus(),
deletionProtection = javaType.deletionProtection(),
description = javaType.description(),
desiredStatus = javaType.desiredStatus(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
enableDisplay = javaType.enableDisplay(),
guestAccelerators = javaType.guestAccelerators().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGuestAccelerator.Companion.toKotlin(args0)
})
}),
hostname = javaType.hostname(),
id = javaType.id(),
instanceId = javaType.instanceId(),
keyRevocationActionType = javaType.keyRevocationActionType(),
labelFingerprint = javaType.labelFingerprint(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
machineType = javaType.machineType(),
metadata = javaType.metadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
metadataFingerprint = javaType.metadataFingerprint(),
metadataStartupScript = javaType.metadataStartupScript(),
minCpuPlatform = javaType.minCpuPlatform(),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
networkInterfaces = javaType.networkInterfaces().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceNetworkInterface.Companion.toKotlin(args0)
})
}),
networkPerformanceConfigs = javaType.networkPerformanceConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceNetworkPerformanceConfig.Companion.toKotlin(args0)
})
}),
params = javaType.params().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceParam.Companion.toKotlin(args0)
})
}),
partnerMetadata = javaType.partnerMetadata().map({ args0 -> args0.key.to(args0.value) }).toMap(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
reservationAffinities = javaType.reservationAffinities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceReservationAffinity.Companion.toKotlin(args0)
})
}),
resourcePolicies = javaType.resourcePolicies().map({ args0 -> args0 }),
schedulings = javaType.schedulings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceScheduling.Companion.toKotlin(args0)
})
}),
scratchDisks = javaType.scratchDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceScratchDisk.Companion.toKotlin(args0)
})
}),
selfLink = javaType.selfLink().map({ args0 -> args0 }).orElse(null),
serviceAccounts = javaType.serviceAccounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceServiceAccount.Companion.toKotlin(args0)
})
}),
shieldedInstanceConfigs = javaType.shieldedInstanceConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceShieldedInstanceConfig.Companion.toKotlin(args0)
})
}),
tags = javaType.tags().map({ args0 -> args0 }),
tagsFingerprint = javaType.tagsFingerprint(),
zone = javaType.zone().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy