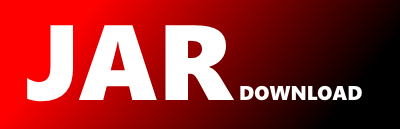
com.pulumi.gcp.compute.kotlin.outputs.RegionNetworkFirewallPolicyWithRulesPredefinedRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property action (Output)
* The Action to perform when the client connection triggers the rule. Can currently be either
* "allow", "deny", "apply_security_profile_group" or "goto_next".
* @property description (Output)
* A description of the rule.
* @property direction (Output)
* The direction in which this rule applies. If unspecified an INGRESS rule is created.
* @property disabled (Output)
* Denotes whether the firewall policy rule is disabled. When set to true,
* the firewall policy rule is not enforced and traffic behaves as if it did
* not exist. If this is unspecified, the firewall policy rule will be
* enabled.
* @property enableLogging (Output)
* Denotes whether to enable logging for a particular rule.
* If logging is enabled, logs will be exported to the
* configured export destination in Stackdriver.
* @property matches (Output)
* A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
* Structure is documented below.
* @property priority (Output)
* An integer indicating the priority of a rule in the list. The priority must be a value
* between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the
* highest priority and 2147483647 is the lowest priority.
* @property ruleName (Output)
* An optional name for the rule. This field is not a unique identifier
* and can be updated.
* @property securityProfileGroup (Output)
* A fully-qualified URL of a SecurityProfile resource instance.
* Example:
* https://networksecurity.googleapis.com/v1/projects/{project}/locations/{location}/securityProfileGroups/my-security-profile-group
* Must be specified if action is 'apply_security_profile_group'.
* @property targetSecureTags (Output)
* A list of secure tags that controls which instances the firewall rule
* applies to. If targetSecureTag
are specified, then the
* firewall rule applies only to instances in the VPC network that have one
* of those EFFECTIVE secure tags, if all the target_secure_tag are in
* INEFFECTIVE state, then this rule will be ignored.
* targetSecureTag
may not be set at the same time as
* targetServiceAccounts
.
* If neither targetServiceAccounts
nor
* targetSecureTag
are specified, the firewall rule applies
* to all instances on the specified network.
* Maximum number of target label tags allowed is 256.
* Structure is documented below.
* @property targetServiceAccounts (Output)
* A list of service accounts indicating the sets of
* instances that are applied with this rule.
* @property tlsInspect (Output)
* Boolean flag indicating if the traffic should be TLS decrypted.
* It can be set only if action = 'apply_security_profile_group' and cannot be set for other actions.
*/
public data class RegionNetworkFirewallPolicyWithRulesPredefinedRule(
public val action: String? = null,
public val description: String? = null,
public val direction: String? = null,
public val disabled: Boolean? = null,
public val enableLogging: Boolean? = null,
public val matches: List? = null,
public val priority: Int? = null,
public val ruleName: String? = null,
public val securityProfileGroup: String? = null,
public val targetSecureTags: List? = null,
public val targetServiceAccounts: List? = null,
public val tlsInspect: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.RegionNetworkFirewallPolicyWithRulesPredefinedRule): RegionNetworkFirewallPolicyWithRulesPredefinedRule =
RegionNetworkFirewallPolicyWithRulesPredefinedRule(
action = javaType.action().map({ args0 -> args0 }).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
direction = javaType.direction().map({ args0 -> args0 }).orElse(null),
disabled = javaType.disabled().map({ args0 -> args0 }).orElse(null),
enableLogging = javaType.enableLogging().map({ args0 -> args0 }).orElse(null),
matches = javaType.matches().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.RegionNetworkFirewallPolicyWithRulesPredefinedRuleMatch.Companion.toKotlin(args0)
})
}),
priority = javaType.priority().map({ args0 -> args0 }).orElse(null),
ruleName = javaType.ruleName().map({ args0 -> args0 }).orElse(null),
securityProfileGroup = javaType.securityProfileGroup().map({ args0 -> args0 }).orElse(null),
targetSecureTags = javaType.targetSecureTags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.RegionNetworkFirewallPolicyWithRulesPredefinedRuleTargetSecureTag.Companion.toKotlin(args0)
})
}),
targetServiceAccounts = javaType.targetServiceAccounts().map({ args0 -> args0 }),
tlsInspect = javaType.tlsInspect().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy