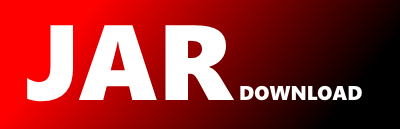
com.pulumi.gcp.eventarc.kotlin.TriggerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.eventarc.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.eventarc.TriggerArgs.builder
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerDestinationArgs
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerDestinationArgsBuilder
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerMatchingCriteriaArgs
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerMatchingCriteriaArgsBuilder
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerTransportArgs
import com.pulumi.gcp.eventarc.kotlin.inputs.TriggerTransportArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The Eventarc Trigger resource
* ## Example Usage
* ### Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.cloudrun.Service("default", {
* name: "eventarc-service",
* location: "europe-west1",
* metadata: {
* namespace: "my-project-name",
* },
* template: {
* spec: {
* containers: [{
* image: "gcr.io/cloudrun/hello",
* ports: [{
* containerPort: 8080,
* }],
* }],
* containerConcurrency: 50,
* timeoutSeconds: 100,
* },
* },
* traffics: [{
* percent: 100,
* latestRevision: true,
* }],
* });
* const primary = new gcp.eventarc.Trigger("primary", {
* name: "name",
* location: "europe-west1",
* matchingCriterias: [{
* attribute: "type",
* value: "google.cloud.pubsub.topic.v1.messagePublished",
* }],
* destination: {
* cloudRunService: {
* service: _default.name,
* region: "europe-west1",
* },
* },
* labels: {
* foo: "bar",
* },
* });
* const foo = new gcp.pubsub.Topic("foo", {name: "topic"});
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.cloudrun.Service("default",
* name="eventarc-service",
* location="europe-west1",
* metadata={
* "namespace": "my-project-name",
* },
* template={
* "spec": {
* "containers": [{
* "image": "gcr.io/cloudrun/hello",
* "ports": [{
* "container_port": 8080,
* }],
* }],
* "container_concurrency": 50,
* "timeout_seconds": 100,
* },
* },
* traffics=[{
* "percent": 100,
* "latest_revision": True,
* }])
* primary = gcp.eventarc.Trigger("primary",
* name="name",
* location="europe-west1",
* matching_criterias=[{
* "attribute": "type",
* "value": "google.cloud.pubsub.topic.v1.messagePublished",
* }],
* destination={
* "cloud_run_service": {
* "service": default.name,
* "region": "europe-west1",
* },
* },
* labels={
* "foo": "bar",
* })
* foo = gcp.pubsub.Topic("foo", name="topic")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.CloudRun.Service("default", new()
* {
* Name = "eventarc-service",
* Location = "europe-west1",
* Metadata = new Gcp.CloudRun.Inputs.ServiceMetadataArgs
* {
* Namespace = "my-project-name",
* },
* Template = new Gcp.CloudRun.Inputs.ServiceTemplateArgs
* {
* Spec = new Gcp.CloudRun.Inputs.ServiceTemplateSpecArgs
* {
* Containers = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTemplateSpecContainerArgs
* {
* Image = "gcr.io/cloudrun/hello",
* Ports = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTemplateSpecContainerPortArgs
* {
* ContainerPort = 8080,
* },
* },
* },
* },
* ContainerConcurrency = 50,
* TimeoutSeconds = 100,
* },
* },
* Traffics = new[]
* {
* new Gcp.CloudRun.Inputs.ServiceTrafficArgs
* {
* Percent = 100,
* LatestRevision = true,
* },
* },
* });
* var primary = new Gcp.Eventarc.Trigger("primary", new()
* {
* Name = "name",
* Location = "europe-west1",
* MatchingCriterias = new[]
* {
* new Gcp.Eventarc.Inputs.TriggerMatchingCriteriaArgs
* {
* Attribute = "type",
* Value = "google.cloud.pubsub.topic.v1.messagePublished",
* },
* },
* Destination = new Gcp.Eventarc.Inputs.TriggerDestinationArgs
* {
* CloudRunService = new Gcp.Eventarc.Inputs.TriggerDestinationCloudRunServiceArgs
* {
* Service = @default.Name,
* Region = "europe-west1",
* },
* },
* Labels =
* {
* { "foo", "bar" },
* },
* });
* var foo = new Gcp.PubSub.Topic("foo", new()
* {
* Name = "topic",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/cloudrun"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/eventarc"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/pubsub"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudrun.NewService(ctx, "default", &cloudrun.ServiceArgs{
* Name: pulumi.String("eventarc-service"),
* Location: pulumi.String("europe-west1"),
* Metadata: &cloudrun.ServiceMetadataArgs{
* Namespace: pulumi.String("my-project-name"),
* },
* Template: &cloudrun.ServiceTemplateArgs{
* Spec: &cloudrun.ServiceTemplateSpecArgs{
* Containers: cloudrun.ServiceTemplateSpecContainerArray{
* &cloudrun.ServiceTemplateSpecContainerArgs{
* Image: pulumi.String("gcr.io/cloudrun/hello"),
* Ports: cloudrun.ServiceTemplateSpecContainerPortArray{
* &cloudrun.ServiceTemplateSpecContainerPortArgs{
* ContainerPort: pulumi.Int(8080),
* },
* },
* },
* },
* ContainerConcurrency: pulumi.Int(50),
* TimeoutSeconds: pulumi.Int(100),
* },
* },
* Traffics: cloudrun.ServiceTrafficArray{
* &cloudrun.ServiceTrafficArgs{
* Percent: pulumi.Int(100),
* LatestRevision: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = eventarc.NewTrigger(ctx, "primary", &eventarc.TriggerArgs{
* Name: pulumi.String("name"),
* Location: pulumi.String("europe-west1"),
* MatchingCriterias: eventarc.TriggerMatchingCriteriaArray{
* &eventarc.TriggerMatchingCriteriaArgs{
* Attribute: pulumi.String("type"),
* Value: pulumi.String("google.cloud.pubsub.topic.v1.messagePublished"),
* },
* },
* Destination: &eventarc.TriggerDestinationArgs{
* CloudRunService: &eventarc.TriggerDestinationCloudRunServiceArgs{
* Service: _default.Name,
* Region: pulumi.String("europe-west1"),
* },
* },
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* _, err = pubsub.NewTopic(ctx, "foo", &pubsub.TopicArgs{
* Name: pulumi.String("topic"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudrun.Service;
* import com.pulumi.gcp.cloudrun.ServiceArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceMetadataArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecArgs;
* import com.pulumi.gcp.cloudrun.inputs.ServiceTrafficArgs;
* import com.pulumi.gcp.eventarc.Trigger;
* import com.pulumi.gcp.eventarc.TriggerArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerMatchingCriteriaArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerDestinationArgs;
* import com.pulumi.gcp.eventarc.inputs.TriggerDestinationCloudRunServiceArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Service("default", ServiceArgs.builder()
* .name("eventarc-service")
* .location("europe-west1")
* .metadata(ServiceMetadataArgs.builder()
* .namespace("my-project-name")
* .build())
* .template(ServiceTemplateArgs.builder()
* .spec(ServiceTemplateSpecArgs.builder()
* .containers(ServiceTemplateSpecContainerArgs.builder()
* .image("gcr.io/cloudrun/hello")
* .ports(ServiceTemplateSpecContainerPortArgs.builder()
* .containerPort(8080)
* .build())
* .build())
* .containerConcurrency(50)
* .timeoutSeconds(100)
* .build())
* .build())
* .traffics(ServiceTrafficArgs.builder()
* .percent(100)
* .latestRevision(true)
* .build())
* .build());
* var primary = new Trigger("primary", TriggerArgs.builder()
* .name("name")
* .location("europe-west1")
* .matchingCriterias(TriggerMatchingCriteriaArgs.builder()
* .attribute("type")
* .value("google.cloud.pubsub.topic.v1.messagePublished")
* .build())
* .destination(TriggerDestinationArgs.builder()
* .cloudRunService(TriggerDestinationCloudRunServiceArgs.builder()
* .service(default_.name())
* .region("europe-west1")
* .build())
* .build())
* .labels(Map.of("foo", "bar"))
* .build());
* var foo = new Topic("foo", TopicArgs.builder()
* .name("topic")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: gcp:eventarc:Trigger
* properties:
* name: name
* location: europe-west1
* matchingCriterias:
* - attribute: type
* value: google.cloud.pubsub.topic.v1.messagePublished
* destination:
* cloudRunService:
* service: ${default.name}
* region: europe-west1
* labels:
* foo: bar
* foo:
* type: gcp:pubsub:Topic
* properties:
* name: topic
* default:
* type: gcp:cloudrun:Service
* properties:
* name: eventarc-service
* location: europe-west1
* metadata:
* namespace: my-project-name
* template:
* spec:
* containers:
* - image: gcr.io/cloudrun/hello
* ports:
* - containerPort: 8080
* containerConcurrency: 50
* timeoutSeconds: 100
* traffics:
* - percent: 100
* latestRevision: true
* ```
*
* ## Import
* Trigger can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/triggers/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Trigger can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default projects/{{project}}/locations/{{location}}/triggers/{{name}}
* ```
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:eventarc/trigger:Trigger default {{location}}/{{name}}
* ```
* @property channel Optional. The name of the channel associated with the trigger in
* `projects/{project}/locations/{location}/channels/{channel}` format. You must provide a channel to receive events from
* Eventarc SaaS partners.
* @property destination Required. Destination specifies where the events should be sent to.
* @property eventDataContentType Optional. EventDataContentType specifies the type of payload in MIME format that is expected from the CloudEvent data
* field. This is set to `application/json` if the value is not defined.
* @property labels Optional. User labels attached to the triggers that can be used to group resources. **Note**: This field is
* non-authoritative, and will only manage the labels present in your configuration. Please refer to the field
* `effective_labels` for all of the labels present on the resource.
* @property location The location for the resource
* @property matchingCriterias Required. null The list of filters that applies to event attributes. Only events that match all the provided filters will be sent to the destination.
* @property name Required. The resource name of the trigger. Must be unique within the location on the project.
* @property project The project for the resource
* @property serviceAccount Optional. The IAM service account email associated with the trigger. The service account represents the identity of the
* trigger. The principal who calls this API must have `iam.serviceAccounts.actAs` permission in the service account. See
* https://cloud.google.com/iam/docs/understanding-service-accounts#sa_common for more information. For Cloud Run
* destinations, this service account is used to generate identity tokens when invoking the service. See
* https://cloud.google.com/run/docs/triggering/pubsub-push#create-service-account for information on how to invoke
* authenticated Cloud Run services. In order to create Audit Log triggers, the service account should also have
* `roles/eventarc.eventReceiver` IAM role.
* @property transport Optional. In order to deliver messages, Eventarc may use other GCP products as transport intermediary. This field
* contains a reference to that transport intermediary. This information can be used for debugging purposes.
*/
public data class TriggerArgs(
public val channel: Output? = null,
public val destination: Output? = null,
public val eventDataContentType: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy