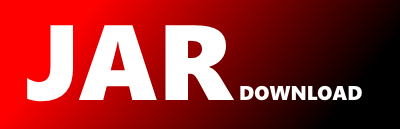
com.pulumi.gcp.networksecurity.kotlin.inputs.AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.networksecurity.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.networksecurity.inputs.AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property contains The input string must have the substring specified here. Note: empty contains match is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc.def
* @property exact The input string must match exactly the string specified here.
* Examples:
* * abc only matches the value abc.
* @property ignoreCase If true, indicates the exact/prefix/suffix/contains matching should be case insensitive. For example, the matcher data will match both input string Data and data if set to true.
* @property prefix The input string must have the prefix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value abc.xyz
* @property suffix The input string must have the suffix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc
*/
public data class AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs(
public val contains: Output? = null,
public val exact: Output? = null,
public val ignoreCase: Output? = null,
public val prefix: Output? = null,
public val suffix: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.networksecurity.inputs.AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs =
com.pulumi.gcp.networksecurity.inputs.AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs.builder()
.contains(contains?.applyValue({ args0 -> args0 }))
.exact(exact?.applyValue({ args0 -> args0 }))
.ignoreCase(ignoreCase?.applyValue({ args0 -> args0 }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.suffix(suffix?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs].
*/
@PulumiTagMarker
public class AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgsBuilder internal constructor() {
private var contains: Output? = null
private var exact: Output? = null
private var ignoreCase: Output? = null
private var prefix: Output? = null
private var suffix: Output? = null
/**
* @param value The input string must have the substring specified here. Note: empty contains match is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc.def
*/
@JvmName("edbsokslonvprsav")
public suspend fun contains(`value`: Output) {
this.contains = value
}
/**
* @param value The input string must match exactly the string specified here.
* Examples:
* * abc only matches the value abc.
*/
@JvmName("etvmknkkmdkbdimp")
public suspend fun exact(`value`: Output) {
this.exact = value
}
/**
* @param value If true, indicates the exact/prefix/suffix/contains matching should be case insensitive. For example, the matcher data will match both input string Data and data if set to true.
*/
@JvmName("nmpwmuuqvhfsgfwo")
public suspend fun ignoreCase(`value`: Output) {
this.ignoreCase = value
}
/**
* @param value The input string must have the prefix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value abc.xyz
*/
@JvmName("vwbmqhdxbkhmyqet")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value The input string must have the suffix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc
*/
@JvmName("kerrsoycdsorhdsf")
public suspend fun suffix(`value`: Output) {
this.suffix = value
}
/**
* @param value The input string must have the substring specified here. Note: empty contains match is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc.def
*/
@JvmName("oxfibjudkydhnnmx")
public suspend fun contains(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contains = mapped
}
/**
* @param value The input string must match exactly the string specified here.
* Examples:
* * abc only matches the value abc.
*/
@JvmName("qfkitspckwmthaqn")
public suspend fun exact(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exact = mapped
}
/**
* @param value If true, indicates the exact/prefix/suffix/contains matching should be case insensitive. For example, the matcher data will match both input string Data and data if set to true.
*/
@JvmName("adnmolllmuwlkwpo")
public suspend fun ignoreCase(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ignoreCase = mapped
}
/**
* @param value The input string must have the prefix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value abc.xyz
*/
@JvmName("gcrxnxjccdcltpae")
public suspend fun prefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefix = mapped
}
/**
* @param value The input string must have the suffix specified here. Note: empty prefix is not allowed, please use regex instead.
* Examples:
* * abc matches the value xyz.abc
*/
@JvmName("aogtbntpcjdqxoom")
public suspend fun suffix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.suffix = mapped
}
internal fun build(): AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs =
AuthzPolicyHttpRuleFromSourceResourceIamServiceAccountArgs(
contains = contains,
exact = exact,
ignoreCase = ignoreCase,
prefix = prefix,
suffix = suffix,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy