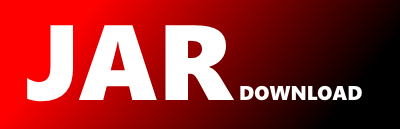
com.pulumi.gcp.oracledatabase.kotlin.outputs.CloudExadataInfrastructureProperties.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.oracledatabase.kotlin.outputs
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property activatedStorageCount (Output)
* The requested number of additional storage servers activated for the
* Exadata Infrastructure.
* @property additionalStorageCount (Output)
* The requested number of additional storage servers for the Exadata
* Infrastructure.
* @property availableStorageSizeGb (Output)
* The available storage can be allocated to the Exadata Infrastructure
* resource, in gigabytes (GB).
* @property computeCount The number of compute servers for the Exadata Infrastructure.
* @property cpuCount (Output)
* The number of enabled CPU cores.
* @property customerContacts The list of customer contacts.
* Structure is documented below.
* @property dataStorageSizeTb (Output)
* Size, in terabytes, of the DATA disk group.
* @property dbNodeStorageSizeGb (Output)
* The local node storage allocated in GBs.
* @property dbServerVersion (Output)
* The software version of the database servers (dom0) in the Exadata
* Infrastructure.
* @property maintenanceWindow Maintenance window as defined by Oracle.
* https://docs.oracle.com/en-us/iaas/api/#/en/database/20160918/datatypes/MaintenanceWindow
* Structure is documented below.
* @property maxCpuCount (Output)
* The total number of CPU cores available.
* @property maxDataStorageTb (Output)
* The total available DATA disk group size.
* @property maxDbNodeStorageSizeGb (Output)
* The total local node storage available in GBs.
* @property maxMemoryGb (Output)
* The total memory available in GBs.
* @property memorySizeGb (Output)
* The memory allocated in GBs.
* @property monthlyDbServerVersion (Output)
* The monthly software version of the database servers (dom0)
* in the Exadata Infrastructure. Example: 20.1.15
* @property monthlyStorageServerVersion (Output)
* The monthly software version of the storage servers (cells)
* in the Exadata Infrastructure. Example: 20.1.15
* @property nextMaintenanceRunId (Output)
* The OCID of the next maintenance run.
* @property nextMaintenanceRunTime (Output)
* The time when the next maintenance run will occur.
* @property nextSecurityMaintenanceRunTime (Output)
* The time when the next security maintenance run will occur.
* @property ociUrl (Output)
* Deep link to the OCI console to view this resource.
* @property ocid (Output)
* OCID of created infra.
* https://docs.oracle.com/en-us/iaas/Content/General/Concepts/identifiers.htm#Oracle
* @property shape The shape of the Exadata Infrastructure. The shape determines the
* amount of CPU, storage, and memory resources allocated to the instance.
* @property state (Output)
* The current lifecycle state of the Exadata Infrastructure.
* Possible values:
* STATE_UNSPECIFIED
* PROVISIONING
* AVAILABLE
* UPDATING
* TERMINATING
* TERMINATED
* FAILED
* MAINTENANCE_IN_PROGRESS
* @property storageCount The number of Cloud Exadata storage servers for the Exadata Infrastructure.
* @property storageServerVersion (Output)
* The software version of the storage servers (cells) in the Exadata
* Infrastructure.
* @property totalStorageSizeGb The total storage allocated to the Exadata Infrastructure
* resource, in gigabytes (GB).
*/
public data class CloudExadataInfrastructureProperties(
public val activatedStorageCount: Int? = null,
public val additionalStorageCount: Int? = null,
public val availableStorageSizeGb: Int? = null,
public val computeCount: Int? = null,
public val cpuCount: Int? = null,
public val customerContacts: List? = null,
public val dataStorageSizeTb: Double? = null,
public val dbNodeStorageSizeGb: Int? = null,
public val dbServerVersion: String? = null,
public val maintenanceWindow: CloudExadataInfrastructurePropertiesMaintenanceWindow? = null,
public val maxCpuCount: Int? = null,
public val maxDataStorageTb: Double? = null,
public val maxDbNodeStorageSizeGb: Int? = null,
public val maxMemoryGb: Int? = null,
public val memorySizeGb: Int? = null,
public val monthlyDbServerVersion: String? = null,
public val monthlyStorageServerVersion: String? = null,
public val nextMaintenanceRunId: String? = null,
public val nextMaintenanceRunTime: String? = null,
public val nextSecurityMaintenanceRunTime: String? = null,
public val ociUrl: String? = null,
public val ocid: String? = null,
public val shape: String,
public val state: String? = null,
public val storageCount: Int? = null,
public val storageServerVersion: String? = null,
public val totalStorageSizeGb: Int? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.oracledatabase.outputs.CloudExadataInfrastructureProperties): CloudExadataInfrastructureProperties = CloudExadataInfrastructureProperties(
activatedStorageCount = javaType.activatedStorageCount().map({ args0 -> args0 }).orElse(null),
additionalStorageCount = javaType.additionalStorageCount().map({ args0 -> args0 }).orElse(null),
availableStorageSizeGb = javaType.availableStorageSizeGb().map({ args0 -> args0 }).orElse(null),
computeCount = javaType.computeCount().map({ args0 -> args0 }).orElse(null),
cpuCount = javaType.cpuCount().map({ args0 -> args0 }).orElse(null),
customerContacts = javaType.customerContacts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.oracledatabase.kotlin.outputs.CloudExadataInfrastructurePropertiesCustomerContact.Companion.toKotlin(args0)
})
}),
dataStorageSizeTb = javaType.dataStorageSizeTb().map({ args0 -> args0 }).orElse(null),
dbNodeStorageSizeGb = javaType.dbNodeStorageSizeGb().map({ args0 -> args0 }).orElse(null),
dbServerVersion = javaType.dbServerVersion().map({ args0 -> args0 }).orElse(null),
maintenanceWindow = javaType.maintenanceWindow().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.oracledatabase.kotlin.outputs.CloudExadataInfrastructurePropertiesMaintenanceWindow.Companion.toKotlin(args0)
})
}).orElse(null),
maxCpuCount = javaType.maxCpuCount().map({ args0 -> args0 }).orElse(null),
maxDataStorageTb = javaType.maxDataStorageTb().map({ args0 -> args0 }).orElse(null),
maxDbNodeStorageSizeGb = javaType.maxDbNodeStorageSizeGb().map({ args0 -> args0 }).orElse(null),
maxMemoryGb = javaType.maxMemoryGb().map({ args0 -> args0 }).orElse(null),
memorySizeGb = javaType.memorySizeGb().map({ args0 -> args0 }).orElse(null),
monthlyDbServerVersion = javaType.monthlyDbServerVersion().map({ args0 -> args0 }).orElse(null),
monthlyStorageServerVersion = javaType.monthlyStorageServerVersion().map({ args0 ->
args0
}).orElse(null),
nextMaintenanceRunId = javaType.nextMaintenanceRunId().map({ args0 -> args0 }).orElse(null),
nextMaintenanceRunTime = javaType.nextMaintenanceRunTime().map({ args0 -> args0 }).orElse(null),
nextSecurityMaintenanceRunTime = javaType.nextSecurityMaintenanceRunTime().map({ args0 ->
args0
}).orElse(null),
ociUrl = javaType.ociUrl().map({ args0 -> args0 }).orElse(null),
ocid = javaType.ocid().map({ args0 -> args0 }).orElse(null),
shape = javaType.shape(),
state = javaType.state().map({ args0 -> args0 }).orElse(null),
storageCount = javaType.storageCount().map({ args0 -> args0 }).orElse(null),
storageServerVersion = javaType.storageServerVersion().map({ args0 -> args0 }).orElse(null),
totalStorageSizeGb = javaType.totalStorageSizeGb().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy