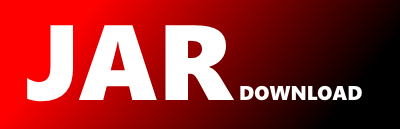
com.pulumi.gcp.organizations.kotlin.ProjectArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.organizations.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.organizations.ProjectArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Allows creation and management of a Google Cloud Platform project.
* Projects created with this resource must be associated with an Organization.
* See the [Organization documentation](https://cloud.google.com/resource-manager/docs/quickstarts) for more details.
* The user or service account that is running this provider when creating a `gcp.organizations.Project`
* resource must have `roles/resourcemanager.projectCreator` on the specified organization. See the
* [Access Control for Organizations Using IAM](https://cloud.google.com/resource-manager/docs/access-control-org)
* doc for more information.
* > This resource reads the specified billing account on every pulumi up and plan operation so you must have permissions on the specified billing account.
* > It is recommended to use the `constraints/compute.skipDefaultNetworkCreation` [constraint](https://www.terraform.io/docs/providers/google/r/google_organization_policy.html) to remove the default network instead of setting `auto_create_network` to false, when possible.
* > It may take a while for the attached tag bindings to be deleted after the project is scheduled to be deleted.
* To get more information about projects, see:
* * [API documentation](https://cloud.google.com/resource-manager/reference/rest/v1/projects)
* * How-to Guides
* * [Creating and managing projects](https://cloud.google.com/resource-manager/docs/creating-managing-projects)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myProject = new gcp.organizations.Project("my_project", {
* name: "My Project",
* projectId: "your-project-id",
* orgId: "1234567",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_project = gcp.organizations.Project("my_project",
* name="My Project",
* project_id="your-project-id",
* org_id="1234567")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myProject = new Gcp.Organizations.Project("my_project", new()
* {
* Name = "My Project",
* ProjectId = "your-project-id",
* OrgId = "1234567",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := organizations.NewProject(ctx, "my_project", &organizations.ProjectArgs{
* Name: pulumi.String("My Project"),
* ProjectId: pulumi.String("your-project-id"),
* OrgId: pulumi.String("1234567"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myProject = new Project("myProject", ProjectArgs.builder()
* .name("My Project")
* .projectId("your-project-id")
* .orgId("1234567")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myProject:
* type: gcp:organizations:Project
* name: my_project
* properties:
* name: My Project
* projectId: your-project-id
* orgId: '1234567'
* ```
*
* To create a project under a specific folder
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const department1 = new gcp.organizations.Folder("department1", {
* displayName: "Department 1",
* parent: "organizations/1234567",
* });
* const myProject_in_a_folder = new gcp.organizations.Project("my_project-in-a-folder", {
* name: "My Project",
* projectId: "your-project-id",
* folderId: department1.name,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* department1 = gcp.organizations.Folder("department1",
* display_name="Department 1",
* parent="organizations/1234567")
* my_project_in_a_folder = gcp.organizations.Project("my_project-in-a-folder",
* name="My Project",
* project_id="your-project-id",
* folder_id=department1.name)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var department1 = new Gcp.Organizations.Folder("department1", new()
* {
* DisplayName = "Department 1",
* Parent = "organizations/1234567",
* });
* var myProject_in_a_folder = new Gcp.Organizations.Project("my_project-in-a-folder", new()
* {
* Name = "My Project",
* ProjectId = "your-project-id",
* FolderId = department1.Name,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* department1, err := organizations.NewFolder(ctx, "department1", &organizations.FolderArgs{
* DisplayName: pulumi.String("Department 1"),
* Parent: pulumi.String("organizations/1234567"),
* })
* if err != nil {
* return err
* }
* _, err = organizations.NewProject(ctx, "my_project-in-a-folder", &organizations.ProjectArgs{
* Name: pulumi.String("My Project"),
* ProjectId: pulumi.String("your-project-id"),
* FolderId: department1.Name,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var department1 = new Folder("department1", FolderArgs.builder()
* .displayName("Department 1")
* .parent("organizations/1234567")
* .build());
* var myProject_in_a_folder = new Project("myProject-in-a-folder", ProjectArgs.builder()
* .name("My Project")
* .projectId("your-project-id")
* .folderId(department1.name())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myProject-in-a-folder:
* type: gcp:organizations:Project
* name: my_project-in-a-folder
* properties:
* name: My Project
* projectId: your-project-id
* folderId: ${department1.name}
* department1:
* type: gcp:organizations:Folder
* properties:
* displayName: Department 1
* parent: organizations/1234567
* ```
*
* To create a project with a tag
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const myProject = new gcp.organizations.Project("my_project", {
* name: "My Project",
* projectId: "your-project-id",
* orgId: "1234567",
* tags: {
* "1234567/env": "staging",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* my_project = gcp.organizations.Project("my_project",
* name="My Project",
* project_id="your-project-id",
* org_id="1234567",
* tags={
* "1234567/env": "staging",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var myProject = new Gcp.Organizations.Project("my_project", new()
* {
* Name = "My Project",
* ProjectId = "your-project-id",
* OrgId = "1234567",
* Tags =
* {
* { "1234567/env", "staging" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := organizations.NewProject(ctx, "my_project", &organizations.ProjectArgs{
* Name: pulumi.String("My Project"),
* ProjectId: pulumi.String("your-project-id"),
* OrgId: pulumi.String("1234567"),
* Tags: pulumi.StringMap{
* "1234567/env": pulumi.String("staging"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myProject = new Project("myProject", ProjectArgs.builder()
* .name("My Project")
* .projectId("your-project-id")
* .orgId("1234567")
* .tags(Map.of("1234567/env", "staging"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myProject:
* type: gcp:organizations:Project
* name: my_project
* properties:
* name: My Project
* projectId: your-project-id
* orgId: '1234567'
* tags:
* 1234567/env: staging
* ```
*
* ## Import
* Projects can be imported using the `project_id`, e.g.
* * `{{project_id}}`
* When using the `pulumi import` command, Projects can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:organizations/project:Project default {{project_id}}
* ```
* @property autoCreateNetwork Create the 'default' network automatically. Default true. If set to false, the default network will be deleted. Note
* that, for quota purposes, you will still need to have 1 network slot available to create the project successfully, even
* if you set auto_create_network to false, since the network will exist momentarily.
* @property billingAccount The alphanumeric ID of the billing account this project
* belongs to. The user or service account performing this operation with the provider
* must have at mininum Billing Account User privileges (`roles/billing.user`) on the billing account.
* See [Google Cloud Billing API Access Control](https://cloud.google.com/billing/docs/how-to/billing-access)
* for more details.
* @property deletionPolicy
* @property folderId The numeric ID of the folder this project should be
* created under. Only one of `org_id` or `folder_id` may be
* specified. If the `folder_id` is specified, then the project is
* created under the specified folder. Changing this forces the
* project to be migrated to the newly specified folder.
* @property labels A set of key/value label pairs to assign to the project.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field 'effective_labels' for all of the labels present on the resource.
* @property name The display name of the project.
* @property orgId The numeric ID of the organization this project belongs to.
* Changing this forces a new project to be created. Only one of
* `org_id` or `folder_id` may be specified. If the `org_id` is
* specified then the project is created at the top level. Changing
* this forces the project to be migrated to the newly specified
* organization.
* @property projectId The project ID. Changing this forces a new project to be created.
* @property tags A map of resource manager tags. Resource manager tag keys and values have the same definition as resource manager tags. Keys must be in the format tagKeys/{tag_key_id}, and values are in the format tagValues/456. The field is ignored when empty. The field is immutable and causes resource replacement when mutated. This field is only set at create time and modifying this field after creation will trigger recreation. To apply tags to an existing resource, see the `gcp.tags.TagValue` resource.
*/
public data class ProjectArgs(
public val autoCreateNetwork: Output? = null,
public val billingAccount: Output? = null,
public val deletionPolicy: Output? = null,
public val folderId: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy