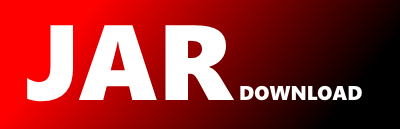
com.pulumi.gcp.securesourcemanager.kotlin.BranchRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.securesourcemanager.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.securesourcemanager.BranchRuleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* BranchRule is the protection rule to enforce pre-defined rules on designated branches within a repository.
* To get more information about BranchRule, see:
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/secure-source-manager/docs/overview)
* ## Example Usage
* ### Secure Source Manager Branch Rule Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.securesourcemanager.Instance("instance", {
* location: "us-central1",
* instanceId: "my-basic-instance",
* });
* const repository = new gcp.securesourcemanager.Repository("repository", {
* repositoryId: "my-basic-repository",
* location: instance.location,
* instance: instance.name,
* });
* const basic = new gcp.securesourcemanager.BranchRule("basic", {
* branchRuleId: "my-basic-branchrule",
* repositoryId: repository.repositoryId,
* location: repository.location,
* includePattern: "main",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.securesourcemanager.Instance("instance",
* location="us-central1",
* instance_id="my-basic-instance")
* repository = gcp.securesourcemanager.Repository("repository",
* repository_id="my-basic-repository",
* location=instance.location,
* instance=instance.name)
* basic = gcp.securesourcemanager.BranchRule("basic",
* branch_rule_id="my-basic-branchrule",
* repository_id=repository.repository_id,
* location=repository.location,
* include_pattern="main")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.SecureSourceManager.Instance("instance", new()
* {
* Location = "us-central1",
* InstanceId = "my-basic-instance",
* });
* var repository = new Gcp.SecureSourceManager.Repository("repository", new()
* {
* RepositoryId = "my-basic-repository",
* Location = instance.Location,
* Instance = instance.Name,
* });
* var basic = new Gcp.SecureSourceManager.BranchRule("basic", new()
* {
* BranchRuleId = "my-basic-branchrule",
* RepositoryId = repository.RepositoryId,
* Location = repository.Location,
* IncludePattern = "main",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/securesourcemanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* instance, err := securesourcemanager.NewInstance(ctx, "instance", &securesourcemanager.InstanceArgs{
* Location: pulumi.String("us-central1"),
* InstanceId: pulumi.String("my-basic-instance"),
* })
* if err != nil {
* return err
* }
* repository, err := securesourcemanager.NewRepository(ctx, "repository", &securesourcemanager.RepositoryArgs{
* RepositoryId: pulumi.String("my-basic-repository"),
* Location: instance.Location,
* Instance: instance.Name,
* })
* if err != nil {
* return err
* }
* _, err = securesourcemanager.NewBranchRule(ctx, "basic", &securesourcemanager.BranchRuleArgs{
* BranchRuleId: pulumi.String("my-basic-branchrule"),
* RepositoryId: repository.RepositoryId,
* Location: repository.Location,
* IncludePattern: pulumi.String("main"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.securesourcemanager.Instance;
* import com.pulumi.gcp.securesourcemanager.InstanceArgs;
* import com.pulumi.gcp.securesourcemanager.Repository;
* import com.pulumi.gcp.securesourcemanager.RepositoryArgs;
* import com.pulumi.gcp.securesourcemanager.BranchRule;
* import com.pulumi.gcp.securesourcemanager.BranchRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .location("us-central1")
* .instanceId("my-basic-instance")
* .build());
* var repository = new Repository("repository", RepositoryArgs.builder()
* .repositoryId("my-basic-repository")
* .location(instance.location())
* .instance(instance.name())
* .build());
* var basic = new BranchRule("basic", BranchRuleArgs.builder()
* .branchRuleId("my-basic-branchrule")
* .repositoryId(repository.repositoryId())
* .location(repository.location())
* .includePattern("main")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:securesourcemanager:Instance
* properties:
* location: us-central1
* instanceId: my-basic-instance
* repository:
* type: gcp:securesourcemanager:Repository
* properties:
* repositoryId: my-basic-repository
* location: ${instance.location}
* instance: ${instance.name}
* basic:
* type: gcp:securesourcemanager:BranchRule
* properties:
* branchRuleId: my-basic-branchrule
* repositoryId: ${repository.repositoryId}
* location: ${repository.location}
* includePattern: main
* ```
*
* ### Secure Source Manager Branch Rule With Fields
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const instance = new gcp.securesourcemanager.Instance("instance", {
* location: "us-central1",
* instanceId: "my-initial-instance",
* });
* const repository = new gcp.securesourcemanager.Repository("repository", {
* repositoryId: "my-initial-repository",
* instance: instance.name,
* location: instance.location,
* });
* const _default = new gcp.securesourcemanager.BranchRule("default", {
* branchRuleId: "my-initial-branchrule",
* location: repository.location,
* repositoryId: repository.repositoryId,
* includePattern: "test",
* minimumApprovalsCount: 2,
* minimumReviewsCount: 2,
* requireCommentsResolved: true,
* requireLinearHistory: true,
* requirePullRequest: true,
* disabled: false,
* allowStaleReviews: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* instance = gcp.securesourcemanager.Instance("instance",
* location="us-central1",
* instance_id="my-initial-instance")
* repository = gcp.securesourcemanager.Repository("repository",
* repository_id="my-initial-repository",
* instance=instance.name,
* location=instance.location)
* default = gcp.securesourcemanager.BranchRule("default",
* branch_rule_id="my-initial-branchrule",
* location=repository.location,
* repository_id=repository.repository_id,
* include_pattern="test",
* minimum_approvals_count=2,
* minimum_reviews_count=2,
* require_comments_resolved=True,
* require_linear_history=True,
* require_pull_request=True,
* disabled=False,
* allow_stale_reviews=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var instance = new Gcp.SecureSourceManager.Instance("instance", new()
* {
* Location = "us-central1",
* InstanceId = "my-initial-instance",
* });
* var repository = new Gcp.SecureSourceManager.Repository("repository", new()
* {
* RepositoryId = "my-initial-repository",
* Instance = instance.Name,
* Location = instance.Location,
* });
* var @default = new Gcp.SecureSourceManager.BranchRule("default", new()
* {
* BranchRuleId = "my-initial-branchrule",
* Location = repository.Location,
* RepositoryId = repository.RepositoryId,
* IncludePattern = "test",
* MinimumApprovalsCount = 2,
* MinimumReviewsCount = 2,
* RequireCommentsResolved = true,
* RequireLinearHistory = true,
* RequirePullRequest = true,
* Disabled = false,
* AllowStaleReviews = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/securesourcemanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* instance, err := securesourcemanager.NewInstance(ctx, "instance", &securesourcemanager.InstanceArgs{
* Location: pulumi.String("us-central1"),
* InstanceId: pulumi.String("my-initial-instance"),
* })
* if err != nil {
* return err
* }
* repository, err := securesourcemanager.NewRepository(ctx, "repository", &securesourcemanager.RepositoryArgs{
* RepositoryId: pulumi.String("my-initial-repository"),
* Instance: instance.Name,
* Location: instance.Location,
* })
* if err != nil {
* return err
* }
* _, err = securesourcemanager.NewBranchRule(ctx, "default", &securesourcemanager.BranchRuleArgs{
* BranchRuleId: pulumi.String("my-initial-branchrule"),
* Location: repository.Location,
* RepositoryId: repository.RepositoryId,
* IncludePattern: pulumi.String("test"),
* MinimumApprovalsCount: pulumi.Int(2),
* MinimumReviewsCount: pulumi.Int(2),
* RequireCommentsResolved: pulumi.Bool(true),
* RequireLinearHistory: pulumi.Bool(true),
* RequirePullRequest: pulumi.Bool(true),
* Disabled: pulumi.Bool(false),
* AllowStaleReviews: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.securesourcemanager.Instance;
* import com.pulumi.gcp.securesourcemanager.InstanceArgs;
* import com.pulumi.gcp.securesourcemanager.Repository;
* import com.pulumi.gcp.securesourcemanager.RepositoryArgs;
* import com.pulumi.gcp.securesourcemanager.BranchRule;
* import com.pulumi.gcp.securesourcemanager.BranchRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var instance = new Instance("instance", InstanceArgs.builder()
* .location("us-central1")
* .instanceId("my-initial-instance")
* .build());
* var repository = new Repository("repository", RepositoryArgs.builder()
* .repositoryId("my-initial-repository")
* .instance(instance.name())
* .location(instance.location())
* .build());
* var default_ = new BranchRule("default", BranchRuleArgs.builder()
* .branchRuleId("my-initial-branchrule")
* .location(repository.location())
* .repositoryId(repository.repositoryId())
* .includePattern("test")
* .minimumApprovalsCount(2)
* .minimumReviewsCount(2)
* .requireCommentsResolved(true)
* .requireLinearHistory(true)
* .requirePullRequest(true)
* .disabled(false)
* .allowStaleReviews(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* instance:
* type: gcp:securesourcemanager:Instance
* properties:
* location: us-central1
* instanceId: my-initial-instance
* repository:
* type: gcp:securesourcemanager:Repository
* properties:
* repositoryId: my-initial-repository
* instance: ${instance.name}
* location: ${instance.location}
* default:
* type: gcp:securesourcemanager:BranchRule
* properties:
* branchRuleId: my-initial-branchrule
* location: ${repository.location}
* repositoryId: ${repository.repositoryId}
* includePattern: test
* minimumApprovalsCount: 2
* minimumReviewsCount: 2
* requireCommentsResolved: true
* requireLinearHistory: true
* requirePullRequest: true
* disabled: false
* allowStaleReviews: false
* ```
*
* ## Import
* BranchRule can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/repositories/{{repository_id}}/branchRules/{{branch_rule_id}}`
* * `{{project}}/{{location}}/{{repository_id}}/{{branch_rule_id}}`
* * `{{location}}/{{repository_id}}/{{branch_rule_id}}`
* * `{{branch_rule_id}}`
* When using the `pulumi import` command, BranchRule can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:securesourcemanager/branchRule:BranchRule default projects/{{project}}/locations/{{location}}/repositories/{{repository_id}}/branchRules/{{branch_rule_id}}
* ```
* ```sh
* $ pulumi import gcp:securesourcemanager/branchRule:BranchRule default {{project}}/{{location}}/{{repository_id}}/{{branch_rule_id}}
* ```
* ```sh
* $ pulumi import gcp:securesourcemanager/branchRule:BranchRule default {{location}}/{{repository_id}}/{{branch_rule_id}}
* ```
* ```sh
* $ pulumi import gcp:securesourcemanager/branchRule:BranchRule default {{branch_rule_id}}
* ```
* @property allowStaleReviews Determines if allow stale reviews or approvals before merging to the branch.
* @property branchRuleId The ID for the BranchRule.
* @property disabled Determines if the branch rule is disabled or not.
* @property includePattern The BranchRule matches branches based on the specified regular expression. Use .* to match all branches.
* @property location The location for the Repository.
* @property minimumApprovalsCount The minimum number of approvals required for the branch rule to be matched.
* @property minimumReviewsCount The minimum number of reviews required for the branch rule to be matched.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property repositoryId The ID for the Repository.
* - - -
* @property requireCommentsResolved Determines if require comments resolved before merging to the branch.
* @property requireLinearHistory Determines if require linear history before merging to the branch.
* @property requirePullRequest Determines if the branch rule requires a pull request or not.
*/
public data class BranchRuleArgs(
public val allowStaleReviews: Output? = null,
public val branchRuleId: Output? = null,
public val disabled: Output? = null,
public val includePattern: Output? = null,
public val location: Output? = null,
public val minimumApprovalsCount: Output? = null,
public val minimumReviewsCount: Output? = null,
public val project: Output? = null,
public val repositoryId: Output? = null,
public val requireCommentsResolved: Output? = null,
public val requireLinearHistory: Output? = null,
public val requirePullRequest: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.securesourcemanager.BranchRuleArgs =
com.pulumi.gcp.securesourcemanager.BranchRuleArgs.builder()
.allowStaleReviews(allowStaleReviews?.applyValue({ args0 -> args0 }))
.branchRuleId(branchRuleId?.applyValue({ args0 -> args0 }))
.disabled(disabled?.applyValue({ args0 -> args0 }))
.includePattern(includePattern?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.minimumApprovalsCount(minimumApprovalsCount?.applyValue({ args0 -> args0 }))
.minimumReviewsCount(minimumReviewsCount?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.repositoryId(repositoryId?.applyValue({ args0 -> args0 }))
.requireCommentsResolved(requireCommentsResolved?.applyValue({ args0 -> args0 }))
.requireLinearHistory(requireLinearHistory?.applyValue({ args0 -> args0 }))
.requirePullRequest(requirePullRequest?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BranchRuleArgs].
*/
@PulumiTagMarker
public class BranchRuleArgsBuilder internal constructor() {
private var allowStaleReviews: Output? = null
private var branchRuleId: Output? = null
private var disabled: Output? = null
private var includePattern: Output? = null
private var location: Output? = null
private var minimumApprovalsCount: Output? = null
private var minimumReviewsCount: Output? = null
private var project: Output? = null
private var repositoryId: Output? = null
private var requireCommentsResolved: Output? = null
private var requireLinearHistory: Output? = null
private var requirePullRequest: Output? = null
/**
* @param value Determines if allow stale reviews or approvals before merging to the branch.
*/
@JvmName("yqnyrkugshlgvfdf")
public suspend fun allowStaleReviews(`value`: Output) {
this.allowStaleReviews = value
}
/**
* @param value The ID for the BranchRule.
*/
@JvmName("gyywdfykexnhasht")
public suspend fun branchRuleId(`value`: Output) {
this.branchRuleId = value
}
/**
* @param value Determines if the branch rule is disabled or not.
*/
@JvmName("vhcoektugrpmfswy")
public suspend fun disabled(`value`: Output) {
this.disabled = value
}
/**
* @param value The BranchRule matches branches based on the specified regular expression. Use .* to match all branches.
*/
@JvmName("rdqofsrvvetfgwsn")
public suspend fun includePattern(`value`: Output) {
this.includePattern = value
}
/**
* @param value The location for the Repository.
*/
@JvmName("mkdiamaabdtnoawe")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value The minimum number of approvals required for the branch rule to be matched.
*/
@JvmName("glhcbjxkvdxbgvcq")
public suspend fun minimumApprovalsCount(`value`: Output) {
this.minimumApprovalsCount = value
}
/**
* @param value The minimum number of reviews required for the branch rule to be matched.
*/
@JvmName("vfehtikykicfrfpr")
public suspend fun minimumReviewsCount(`value`: Output) {
this.minimumReviewsCount = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("jgdvqndyeyidcfsr")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The ID for the Repository.
* - - -
*/
@JvmName("pigrqkllgkspbikh")
public suspend fun repositoryId(`value`: Output) {
this.repositoryId = value
}
/**
* @param value Determines if require comments resolved before merging to the branch.
*/
@JvmName("rrgdtvoupeejavfm")
public suspend fun requireCommentsResolved(`value`: Output) {
this.requireCommentsResolved = value
}
/**
* @param value Determines if require linear history before merging to the branch.
*/
@JvmName("mvtpkfsujumxyakg")
public suspend fun requireLinearHistory(`value`: Output) {
this.requireLinearHistory = value
}
/**
* @param value Determines if the branch rule requires a pull request or not.
*/
@JvmName("yplqyqpfxlbhiusl")
public suspend fun requirePullRequest(`value`: Output) {
this.requirePullRequest = value
}
/**
* @param value Determines if allow stale reviews or approvals before merging to the branch.
*/
@JvmName("ledmtcgmjaqijcji")
public suspend fun allowStaleReviews(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowStaleReviews = mapped
}
/**
* @param value The ID for the BranchRule.
*/
@JvmName("ruqobunrshaxdfrw")
public suspend fun branchRuleId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.branchRuleId = mapped
}
/**
* @param value Determines if the branch rule is disabled or not.
*/
@JvmName("clrsffxfifoocgij")
public suspend fun disabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disabled = mapped
}
/**
* @param value The BranchRule matches branches based on the specified regular expression. Use .* to match all branches.
*/
@JvmName("joraovjdvpckljad")
public suspend fun includePattern(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includePattern = mapped
}
/**
* @param value The location for the Repository.
*/
@JvmName("yvebryoftugyakdu")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value The minimum number of approvals required for the branch rule to be matched.
*/
@JvmName("dxknjvcqeoreimnq")
public suspend fun minimumApprovalsCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumApprovalsCount = mapped
}
/**
* @param value The minimum number of reviews required for the branch rule to be matched.
*/
@JvmName("ubuwlnojyumgflkv")
public suspend fun minimumReviewsCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumReviewsCount = mapped
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("koorxsktpscyqjvu")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The ID for the Repository.
* - - -
*/
@JvmName("gmdtjbosnnfckkor")
public suspend fun repositoryId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repositoryId = mapped
}
/**
* @param value Determines if require comments resolved before merging to the branch.
*/
@JvmName("fbtnxtptiraqfjhk")
public suspend fun requireCommentsResolved(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireCommentsResolved = mapped
}
/**
* @param value Determines if require linear history before merging to the branch.
*/
@JvmName("qphkiprukqoeknne")
public suspend fun requireLinearHistory(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requireLinearHistory = mapped
}
/**
* @param value Determines if the branch rule requires a pull request or not.
*/
@JvmName("taugffbmwrfhacyf")
public suspend fun requirePullRequest(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requirePullRequest = mapped
}
internal fun build(): BranchRuleArgs = BranchRuleArgs(
allowStaleReviews = allowStaleReviews,
branchRuleId = branchRuleId,
disabled = disabled,
includePattern = includePattern,
location = location,
minimumApprovalsCount = minimumApprovalsCount,
minimumReviewsCount = minimumReviewsCount,
project = project,
repositoryId = repositoryId,
requireCommentsResolved = requireCommentsResolved,
requireLinearHistory = requireLinearHistory,
requirePullRequest = requirePullRequest,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy