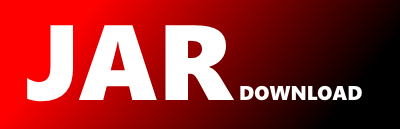
com.pulumi.gcp.securitycenter.kotlin.V2FolderNotificationConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.securitycenter.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.securitycenter.kotlin.outputs.V2FolderNotificationConfigStreamingConfig
import com.pulumi.gcp.securitycenter.kotlin.outputs.V2FolderNotificationConfigStreamingConfig.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [V2FolderNotificationConfig].
*/
@PulumiTagMarker
public class V2FolderNotificationConfigResourceBuilder internal constructor() {
public var name: String? = null
public var args: V2FolderNotificationConfigArgs = V2FolderNotificationConfigArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend V2FolderNotificationConfigArgsBuilder.() -> Unit) {
val builder = V2FolderNotificationConfigArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): V2FolderNotificationConfig {
val builtJavaResource =
com.pulumi.gcp.securitycenter.V2FolderNotificationConfig(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return V2FolderNotificationConfig(builtJavaResource)
}
}
/**
* A Cloud Security Command Center (Cloud SCC) notification configs. A
* notification config is a Cloud SCC resource that contains the
* configuration to send notifications for create/update events of
* findings, assets and etc.
* > **Note:** In order to use Cloud SCC resources, your organization must be enrolled
* in [SCC Standard/Premium](https://cloud.google.com/security-command-center/docs/quickstart-security-command-center).
* Without doing so, you may run into errors during resource creation.
* To get more information about FolderNotificationConfig, see:
* * [API documentation](https://cloud.google.com/security-command-center/docs/reference/rest/v2/folders.locations.notificationConfigs)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/security-command-center/docs)
* ## Example Usage
* ### Scc V2 Folder Notification Config Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const folder = new gcp.organizations.Folder("folder", {
* parent: "organizations/123456789",
* displayName: "folder-name",
* });
* const sccV2FolderNotificationConfig = new gcp.pubsub.Topic("scc_v2_folder_notification_config", {name: "my-topic"});
* const customNotificationConfig = new gcp.securitycenter.V2FolderNotificationConfig("custom_notification_config", {
* configId: "my-config",
* folder: folder.folderId,
* location: "global",
* description: "My custom Cloud Security Command Center Finding Notification Configuration",
* pubsubTopic: sccV2FolderNotificationConfig.id,
* streamingConfig: {
* filter: "category = \"OPEN_FIREWALL\" AND state = \"ACTIVE\"",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* folder = gcp.organizations.Folder("folder",
* parent="organizations/123456789",
* display_name="folder-name")
* scc_v2_folder_notification_config = gcp.pubsub.Topic("scc_v2_folder_notification_config", name="my-topic")
* custom_notification_config = gcp.securitycenter.V2FolderNotificationConfig("custom_notification_config",
* config_id="my-config",
* folder=folder.folder_id,
* location="global",
* description="My custom Cloud Security Command Center Finding Notification Configuration",
* pubsub_topic=scc_v2_folder_notification_config.id,
* streaming_config={
* "filter": "category = \"OPEN_FIREWALL\" AND state = \"ACTIVE\"",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var folder = new Gcp.Organizations.Folder("folder", new()
* {
* Parent = "organizations/123456789",
* DisplayName = "folder-name",
* });
* var sccV2FolderNotificationConfig = new Gcp.PubSub.Topic("scc_v2_folder_notification_config", new()
* {
* Name = "my-topic",
* });
* var customNotificationConfig = new Gcp.SecurityCenter.V2FolderNotificationConfig("custom_notification_config", new()
* {
* ConfigId = "my-config",
* Folder = folder.FolderId,
* Location = "global",
* Description = "My custom Cloud Security Command Center Finding Notification Configuration",
* PubsubTopic = sccV2FolderNotificationConfig.Id,
* StreamingConfig = new Gcp.SecurityCenter.Inputs.V2FolderNotificationConfigStreamingConfigArgs
* {
* Filter = "category = \"OPEN_FIREWALL\" AND state = \"ACTIVE\"",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/pubsub"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/securitycenter"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* folder, err := organizations.NewFolder(ctx, "folder", &organizations.FolderArgs{
* Parent: pulumi.String("organizations/123456789"),
* DisplayName: pulumi.String("folder-name"),
* })
* if err != nil {
* return err
* }
* sccV2FolderNotificationConfig, err := pubsub.NewTopic(ctx, "scc_v2_folder_notification_config", &pubsub.TopicArgs{
* Name: pulumi.String("my-topic"),
* })
* if err != nil {
* return err
* }
* _, err = securitycenter.NewV2FolderNotificationConfig(ctx, "custom_notification_config", &securitycenter.V2FolderNotificationConfigArgs{
* ConfigId: pulumi.String("my-config"),
* Folder: folder.FolderId,
* Location: pulumi.String("global"),
* Description: pulumi.String("My custom Cloud Security Command Center Finding Notification Configuration"),
* PubsubTopic: sccV2FolderNotificationConfig.ID(),
* StreamingConfig: &securitycenter.V2FolderNotificationConfigStreamingConfigArgs{
* Filter: pulumi.String("category = \"OPEN_FIREWALL\" AND state = \"ACTIVE\""),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.pubsub.Topic;
* import com.pulumi.gcp.pubsub.TopicArgs;
* import com.pulumi.gcp.securitycenter.V2FolderNotificationConfig;
* import com.pulumi.gcp.securitycenter.V2FolderNotificationConfigArgs;
* import com.pulumi.gcp.securitycenter.inputs.V2FolderNotificationConfigStreamingConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var folder = new Folder("folder", FolderArgs.builder()
* .parent("organizations/123456789")
* .displayName("folder-name")
* .build());
* var sccV2FolderNotificationConfig = new Topic("sccV2FolderNotificationConfig", TopicArgs.builder()
* .name("my-topic")
* .build());
* var customNotificationConfig = new V2FolderNotificationConfig("customNotificationConfig", V2FolderNotificationConfigArgs.builder()
* .configId("my-config")
* .folder(folder.folderId())
* .location("global")
* .description("My custom Cloud Security Command Center Finding Notification Configuration")
* .pubsubTopic(sccV2FolderNotificationConfig.id())
* .streamingConfig(V2FolderNotificationConfigStreamingConfigArgs.builder()
* .filter("category = \"OPEN_FIREWALL\" AND state = \"ACTIVE\"")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* folder:
* type: gcp:organizations:Folder
* properties:
* parent: organizations/123456789
* displayName: folder-name
* sccV2FolderNotificationConfig:
* type: gcp:pubsub:Topic
* name: scc_v2_folder_notification_config
* properties:
* name: my-topic
* customNotificationConfig:
* type: gcp:securitycenter:V2FolderNotificationConfig
* name: custom_notification_config
* properties:
* configId: my-config
* folder: ${folder.folderId}
* location: global
* description: My custom Cloud Security Command Center Finding Notification Configuration
* pubsubTopic: ${sccV2FolderNotificationConfig.id}
* streamingConfig:
* filter: category = "OPEN_FIREWALL" AND state = "ACTIVE"
* ```
*
* ## Import
* FolderNotificationConfig can be imported using any of these accepted formats:
* * `folders/{{folder}}/locations/{{location}}/notificationConfigs/{{config_id}}`
* * `{{folder}}/{{location}}/{{config_id}}`
* When using the `pulumi import` command, FolderNotificationConfig can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:securitycenter/v2FolderNotificationConfig:V2FolderNotificationConfig default folders/{{folder}}/locations/{{location}}/notificationConfigs/{{config_id}}
* ```
* ```sh
* $ pulumi import gcp:securitycenter/v2FolderNotificationConfig:V2FolderNotificationConfig default {{folder}}/{{location}}/{{config_id}}
* ```
*/
public class V2FolderNotificationConfig internal constructor(
override val javaResource: com.pulumi.gcp.securitycenter.V2FolderNotificationConfig,
) : KotlinCustomResource(javaResource, V2FolderNotificationConfigMapper) {
/**
* This must be unique within the organization.
*/
public val configId: Output
get() = javaResource.configId().applyValue({ args0 -> args0 })
/**
* The description of the notification config (max of 1024 characters).
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Numerical ID of the parent folder.
*/
public val folder: Output
get() = javaResource.folder().applyValue({ args0 -> args0 })
/**
* Location ID of the parent organization. If not provided, 'global' will be used as the default location.
*/
public val location: Output?
get() = javaResource.location().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The resource name of this notification config, in the format
* `folders/{{folder}}/locations/{{location}}/notificationConfigs/{{config_id}}`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The Pub/Sub topic to send notifications to. Its format is
* "projects/[project_id]/topics/[topic]".
*/
public val pubsubTopic: Output
get() = javaResource.pubsubTopic().applyValue({ args0 -> args0 })
/**
* The service account that needs "pubsub.topics.publish" permission to
* publish to the Pub/Sub topic.
*/
public val serviceAccount: Output
get() = javaResource.serviceAccount().applyValue({ args0 -> args0 })
/**
* The config for triggering streaming-based notifications.
* Structure is documented below.
*/
public val streamingConfig: Output
get() = javaResource.streamingConfig().applyValue({ args0 ->
args0.let({ args0 ->
toKotlin(args0)
})
})
}
public object V2FolderNotificationConfigMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.securitycenter.V2FolderNotificationConfig::class == javaResource::class
override fun map(javaResource: Resource): V2FolderNotificationConfig =
V2FolderNotificationConfig(
javaResource as
com.pulumi.gcp.securitycenter.V2FolderNotificationConfig,
)
}
/**
* @see [V2FolderNotificationConfig].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [V2FolderNotificationConfig].
*/
public suspend fun v2FolderNotificationConfig(
name: String,
block: suspend V2FolderNotificationConfigResourceBuilder.() -> Unit,
): V2FolderNotificationConfig {
val builder = V2FolderNotificationConfigResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [V2FolderNotificationConfig].
* @param name The _unique_ name of the resulting resource.
*/
public fun v2FolderNotificationConfig(name: String): V2FolderNotificationConfig {
val builder = V2FolderNotificationConfigResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy