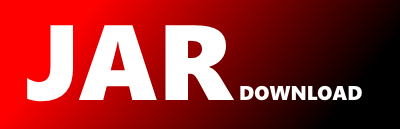
com.pulumi.gcp.transcoder.kotlin.inputs.JobConfigElementaryStreamVideoStreamH264Args.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.transcoder.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.transcoder.inputs.JobConfigElementaryStreamVideoStreamH264Args.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bitrateBps The video bitrate in bits per second.
* @property crfLevel Target CRF level. The default is '21'.
* @property entropyCoder The entropy coder to use. The default is 'cabac'.
* @property frameRate The target video frame rate in frames per second (FPS).
* @property gopDuration Select the GOP size based on the specified duration. The default is '3s'.
* @property heightPixels The height of the video in pixels.
* @property hlg HLG color format setting for H264.
* @property pixelFormat Pixel format to use. The default is 'yuv420p'.
* @property preset Enforces the specified codec preset. The default is 'veryfast'.
* @property profile Enforces the specified codec profile.
* @property rateControlMode Specify the mode. The default is 'vbr'.
* @property sdr SDR color format setting for H264.
* @property vbvFullnessBits Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* @property vbvSizeBits Size of the Video Buffering Verifier (VBV) buffer in bits.
* @property widthPixels The width of the video in pixels.
*/
public data class JobConfigElementaryStreamVideoStreamH264Args(
public val bitrateBps: Output,
public val crfLevel: Output? = null,
public val entropyCoder: Output? = null,
public val frameRate: Output,
public val gopDuration: Output? = null,
public val heightPixels: Output? = null,
public val hlg: Output? = null,
public val pixelFormat: Output? = null,
public val preset: Output? = null,
public val profile: Output? = null,
public val rateControlMode: Output? = null,
public val sdr: Output? = null,
public val vbvFullnessBits: Output? = null,
public val vbvSizeBits: Output? = null,
public val widthPixels: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.transcoder.inputs.JobConfigElementaryStreamVideoStreamH264Args =
com.pulumi.gcp.transcoder.inputs.JobConfigElementaryStreamVideoStreamH264Args.builder()
.bitrateBps(bitrateBps.applyValue({ args0 -> args0 }))
.crfLevel(crfLevel?.applyValue({ args0 -> args0 }))
.entropyCoder(entropyCoder?.applyValue({ args0 -> args0 }))
.frameRate(frameRate.applyValue({ args0 -> args0 }))
.gopDuration(gopDuration?.applyValue({ args0 -> args0 }))
.heightPixels(heightPixels?.applyValue({ args0 -> args0 }))
.hlg(hlg?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.pixelFormat(pixelFormat?.applyValue({ args0 -> args0 }))
.preset(preset?.applyValue({ args0 -> args0 }))
.profile(profile?.applyValue({ args0 -> args0 }))
.rateControlMode(rateControlMode?.applyValue({ args0 -> args0 }))
.sdr(sdr?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.vbvFullnessBits(vbvFullnessBits?.applyValue({ args0 -> args0 }))
.vbvSizeBits(vbvSizeBits?.applyValue({ args0 -> args0 }))
.widthPixels(widthPixels?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobConfigElementaryStreamVideoStreamH264Args].
*/
@PulumiTagMarker
public class JobConfigElementaryStreamVideoStreamH264ArgsBuilder internal constructor() {
private var bitrateBps: Output? = null
private var crfLevel: Output? = null
private var entropyCoder: Output? = null
private var frameRate: Output? = null
private var gopDuration: Output? = null
private var heightPixels: Output? = null
private var hlg: Output? = null
private var pixelFormat: Output? = null
private var preset: Output? = null
private var profile: Output? = null
private var rateControlMode: Output? = null
private var sdr: Output? = null
private var vbvFullnessBits: Output? = null
private var vbvSizeBits: Output? = null
private var widthPixels: Output? = null
/**
* @param value The video bitrate in bits per second.
*/
@JvmName("ergftvgwqssprlmw")
public suspend fun bitrateBps(`value`: Output) {
this.bitrateBps = value
}
/**
* @param value Target CRF level. The default is '21'.
*/
@JvmName("derrekasjyjwcujy")
public suspend fun crfLevel(`value`: Output) {
this.crfLevel = value
}
/**
* @param value The entropy coder to use. The default is 'cabac'.
*/
@JvmName("bqmgpyipcnxtbvsy")
public suspend fun entropyCoder(`value`: Output) {
this.entropyCoder = value
}
/**
* @param value The target video frame rate in frames per second (FPS).
*/
@JvmName("bmqthjkfxtpkfdpo")
public suspend fun frameRate(`value`: Output) {
this.frameRate = value
}
/**
* @param value Select the GOP size based on the specified duration. The default is '3s'.
*/
@JvmName("synhsmprtcyqvkgy")
public suspend fun gopDuration(`value`: Output) {
this.gopDuration = value
}
/**
* @param value The height of the video in pixels.
*/
@JvmName("byxfowrqhcpdawfv")
public suspend fun heightPixels(`value`: Output) {
this.heightPixels = value
}
/**
* @param value HLG color format setting for H264.
*/
@JvmName("bqomksukavemgawb")
public suspend fun hlg(`value`: Output) {
this.hlg = value
}
/**
* @param value Pixel format to use. The default is 'yuv420p'.
*/
@JvmName("sjbtvpuviouodgrt")
public suspend fun pixelFormat(`value`: Output) {
this.pixelFormat = value
}
/**
* @param value Enforces the specified codec preset. The default is 'veryfast'.
*/
@JvmName("opghukovlpodilhf")
public suspend fun preset(`value`: Output) {
this.preset = value
}
/**
* @param value Enforces the specified codec profile.
*/
@JvmName("rfoomrtjsdkmvryp")
public suspend fun profile(`value`: Output) {
this.profile = value
}
/**
* @param value Specify the mode. The default is 'vbr'.
*/
@JvmName("bbpbpvnhyuqqjjdt")
public suspend fun rateControlMode(`value`: Output) {
this.rateControlMode = value
}
/**
* @param value SDR color format setting for H264.
*/
@JvmName("ctmxjcdsoawqfseh")
public suspend fun sdr(`value`: Output) {
this.sdr = value
}
/**
* @param value Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
*/
@JvmName("ipjbpfpkheingyem")
public suspend fun vbvFullnessBits(`value`: Output) {
this.vbvFullnessBits = value
}
/**
* @param value Size of the Video Buffering Verifier (VBV) buffer in bits.
*/
@JvmName("sxdkalyhekpycncc")
public suspend fun vbvSizeBits(`value`: Output) {
this.vbvSizeBits = value
}
/**
* @param value The width of the video in pixels.
*/
@JvmName("xmiuxgfeejvamqlt")
public suspend fun widthPixels(`value`: Output) {
this.widthPixels = value
}
/**
* @param value The video bitrate in bits per second.
*/
@JvmName("jdlfatgeasrmmhuk")
public suspend fun bitrateBps(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bitrateBps = mapped
}
/**
* @param value Target CRF level. The default is '21'.
*/
@JvmName("cadriibqxahydghi")
public suspend fun crfLevel(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.crfLevel = mapped
}
/**
* @param value The entropy coder to use. The default is 'cabac'.
*/
@JvmName("qnviofjxfigqxyeq")
public suspend fun entropyCoder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entropyCoder = mapped
}
/**
* @param value The target video frame rate in frames per second (FPS).
*/
@JvmName("crequwentnxyxlds")
public suspend fun frameRate(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.frameRate = mapped
}
/**
* @param value Select the GOP size based on the specified duration. The default is '3s'.
*/
@JvmName("mmmjdnkkclfpjgsd")
public suspend fun gopDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gopDuration = mapped
}
/**
* @param value The height of the video in pixels.
*/
@JvmName("ydatuuplxclrjnrp")
public suspend fun heightPixels(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.heightPixels = mapped
}
/**
* @param value HLG color format setting for H264.
*/
@JvmName("aydxpdqniyqdrscx")
public suspend fun hlg(`value`: JobConfigElementaryStreamVideoStreamH264HlgArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hlg = mapped
}
/**
* @param argument HLG color format setting for H264.
*/
@JvmName("vqfionrbpxlqcjxm")
public suspend fun hlg(argument: suspend JobConfigElementaryStreamVideoStreamH264HlgArgsBuilder.() -> Unit) {
val toBeMapped = JobConfigElementaryStreamVideoStreamH264HlgArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.hlg = mapped
}
/**
* @param value Pixel format to use. The default is 'yuv420p'.
*/
@JvmName("fgopaitldgomybnh")
public suspend fun pixelFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pixelFormat = mapped
}
/**
* @param value Enforces the specified codec preset. The default is 'veryfast'.
*/
@JvmName("amogymspaieyquiy")
public suspend fun preset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preset = mapped
}
/**
* @param value Enforces the specified codec profile.
*/
@JvmName("wdqhwsbwohxibrjl")
public suspend fun profile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.profile = mapped
}
/**
* @param value Specify the mode. The default is 'vbr'.
*/
@JvmName("awsullfgbfbyfrer")
public suspend fun rateControlMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rateControlMode = mapped
}
/**
* @param value SDR color format setting for H264.
*/
@JvmName("rgheyxceljxtullj")
public suspend fun sdr(`value`: JobConfigElementaryStreamVideoStreamH264SdrArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sdr = mapped
}
/**
* @param argument SDR color format setting for H264.
*/
@JvmName("mlsefwwreqaxenys")
public suspend fun sdr(argument: suspend JobConfigElementaryStreamVideoStreamH264SdrArgsBuilder.() -> Unit) {
val toBeMapped = JobConfigElementaryStreamVideoStreamH264SdrArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sdr = mapped
}
/**
* @param value Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
*/
@JvmName("tlehbhdleqouajhc")
public suspend fun vbvFullnessBits(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vbvFullnessBits = mapped
}
/**
* @param value Size of the Video Buffering Verifier (VBV) buffer in bits.
*/
@JvmName("qbejwowdtknvnrgn")
public suspend fun vbvSizeBits(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vbvSizeBits = mapped
}
/**
* @param value The width of the video in pixels.
*/
@JvmName("tkbjqnjyqnmprnos")
public suspend fun widthPixels(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.widthPixels = mapped
}
internal fun build(): JobConfigElementaryStreamVideoStreamH264Args =
JobConfigElementaryStreamVideoStreamH264Args(
bitrateBps = bitrateBps ?: throw PulumiNullFieldException("bitrateBps"),
crfLevel = crfLevel,
entropyCoder = entropyCoder,
frameRate = frameRate ?: throw PulumiNullFieldException("frameRate"),
gopDuration = gopDuration,
heightPixels = heightPixels,
hlg = hlg,
pixelFormat = pixelFormat,
preset = preset,
profile = profile,
rateControlMode = rateControlMode,
sdr = sdr,
vbvFullnessBits = vbvFullnessBits,
vbvSizeBits = vbvSizeBits,
widthPixels = widthPixels,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy