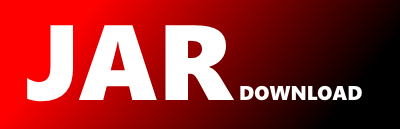
com.pulumi.gcp.transcoder.kotlin.inputs.JobTemplateConfigOverlayAnimationAnimationFadeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.transcoder.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.transcoder.inputs.JobTemplateConfigOverlayAnimationAnimationFadeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property endTimeOffset The time to end the fade animation, in seconds.
* @property fadeType Required. Type of fade animation: `FADE_IN` or `FADE_OUT`.
* The possible values are:
* * `FADE_TYPE_UNSPECIFIED`: The fade type is not specified.
* * `FADE_IN`: Fade the overlay object into view.
* * `FADE_OUT`: Fade the overlay object out of view.
* Possible values are: `FADE_TYPE_UNSPECIFIED`, `FADE_IN`, `FADE_OUT`.
* @property startTimeOffset The time to start the fade animation, in seconds.
* @property xy Normalized coordinates based on output video resolution.
* Structure is documented below.
*/
public data class JobTemplateConfigOverlayAnimationAnimationFadeArgs(
public val endTimeOffset: Output? = null,
public val fadeType: Output,
public val startTimeOffset: Output? = null,
public val xy: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.transcoder.inputs.JobTemplateConfigOverlayAnimationAnimationFadeArgs =
com.pulumi.gcp.transcoder.inputs.JobTemplateConfigOverlayAnimationAnimationFadeArgs.builder()
.endTimeOffset(endTimeOffset?.applyValue({ args0 -> args0 }))
.fadeType(fadeType.applyValue({ args0 -> args0 }))
.startTimeOffset(startTimeOffset?.applyValue({ args0 -> args0 }))
.xy(xy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [JobTemplateConfigOverlayAnimationAnimationFadeArgs].
*/
@PulumiTagMarker
public class JobTemplateConfigOverlayAnimationAnimationFadeArgsBuilder internal constructor() {
private var endTimeOffset: Output? = null
private var fadeType: Output? = null
private var startTimeOffset: Output? = null
private var xy: Output? = null
/**
* @param value The time to end the fade animation, in seconds.
*/
@JvmName("xfciqusqttkqvcjw")
public suspend fun endTimeOffset(`value`: Output) {
this.endTimeOffset = value
}
/**
* @param value Required. Type of fade animation: `FADE_IN` or `FADE_OUT`.
* The possible values are:
* * `FADE_TYPE_UNSPECIFIED`: The fade type is not specified.
* * `FADE_IN`: Fade the overlay object into view.
* * `FADE_OUT`: Fade the overlay object out of view.
* Possible values are: `FADE_TYPE_UNSPECIFIED`, `FADE_IN`, `FADE_OUT`.
*/
@JvmName("phedphloefglthwf")
public suspend fun fadeType(`value`: Output) {
this.fadeType = value
}
/**
* @param value The time to start the fade animation, in seconds.
*/
@JvmName("foapoyoawptihoce")
public suspend fun startTimeOffset(`value`: Output) {
this.startTimeOffset = value
}
/**
* @param value Normalized coordinates based on output video resolution.
* Structure is documented below.
*/
@JvmName("nqdwejuxavocpybt")
public suspend fun xy(`value`: Output) {
this.xy = value
}
/**
* @param value The time to end the fade animation, in seconds.
*/
@JvmName("biikvbhljyvusegx")
public suspend fun endTimeOffset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTimeOffset = mapped
}
/**
* @param value Required. Type of fade animation: `FADE_IN` or `FADE_OUT`.
* The possible values are:
* * `FADE_TYPE_UNSPECIFIED`: The fade type is not specified.
* * `FADE_IN`: Fade the overlay object into view.
* * `FADE_OUT`: Fade the overlay object out of view.
* Possible values are: `FADE_TYPE_UNSPECIFIED`, `FADE_IN`, `FADE_OUT`.
*/
@JvmName("mgebtkmrnxgdweyv")
public suspend fun fadeType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.fadeType = mapped
}
/**
* @param value The time to start the fade animation, in seconds.
*/
@JvmName("enrahrqacmgxwcwk")
public suspend fun startTimeOffset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTimeOffset = mapped
}
/**
* @param value Normalized coordinates based on output video resolution.
* Structure is documented below.
*/
@JvmName("vaiwdhhuckfedwsu")
public suspend fun xy(`value`: JobTemplateConfigOverlayAnimationAnimationFadeXyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.xy = mapped
}
/**
* @param argument Normalized coordinates based on output video resolution.
* Structure is documented below.
*/
@JvmName("tuxweyqcjthqvaew")
public suspend fun xy(argument: suspend JobTemplateConfigOverlayAnimationAnimationFadeXyArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateConfigOverlayAnimationAnimationFadeXyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.xy = mapped
}
internal fun build(): JobTemplateConfigOverlayAnimationAnimationFadeArgs =
JobTemplateConfigOverlayAnimationAnimationFadeArgs(
endTimeOffset = endTimeOffset,
fadeType = fadeType ?: throw PulumiNullFieldException("fadeType"),
startTimeOffset = startTimeOffset,
xy = xy,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy