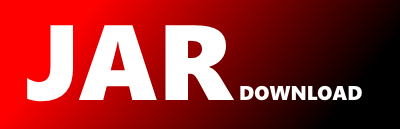
com.pulumi.gcp.vertex.kotlin.AiIndexEndpointDeployedIndexArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.vertex.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndexArgs.builder
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexAutomaticResourcesArgs
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexAutomaticResourcesArgsBuilder
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexDedicatedResourcesArgs
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexDedicatedResourcesArgsBuilder
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs
import com.pulumi.gcp.vertex.kotlin.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* An endpoint indexes are deployed into. An index endpoint can have multiple deployed indexes.
* To get more information about IndexEndpointDeployedIndex, see:
* * [API documentation](https://cloud.google.com/vertex-ai/docs/reference/rest/v1/projects.locations.indexEndpoints#DeployedIndex)
* ## Example Usage
* ### Vertex Ai Index Endpoint Deployed Index Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const sa = new gcp.serviceaccount.Account("sa", {accountId: "vertex-sa"});
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "bucket-name",
* location: "us-central1",
* uniformBucketLevelAccess: true,
* });
* const index = new gcp.vertex.AiIndex("index", {
* labels: {
* foo: "bar",
* },
* region: "us-central1",
* displayName: "test-index",
* description: "index for test",
* metadata: {
* contentsDeltaUri: pulumi.interpolate`gs://${bucket.name}/contents`,
* config: {
* dimensions: 2,
* approximateNeighborsCount: 150,
* shardSize: "SHARD_SIZE_SMALL",
* distanceMeasureType: "DOT_PRODUCT_DISTANCE",
* algorithmConfig: {
* treeAhConfig: {
* leafNodeEmbeddingCount: 500,
* leafNodesToSearchPercent: 7,
* },
* },
* },
* },
* indexUpdateMethod: "BATCH_UPDATE",
* });
* const vertexNetwork = gcp.compute.getNetwork({
* name: "network-name",
* });
* const project = gcp.organizations.getProject({});
* const vertexIndexEndpointDeployed = new gcp.vertex.AiIndexEndpoint("vertex_index_endpoint_deployed", {
* displayName: "sample-endpoint",
* description: "A sample vertex endpoint",
* region: "us-central1",
* labels: {
* "label-one": "value-one",
* },
* network: Promise.all([project, vertexNetwork]).then(([project, vertexNetwork]) => `projects/${project.number}/global/networks/${vertexNetwork.name}`),
* });
* const basicDeployedIndex = new gcp.vertex.AiIndexEndpointDeployedIndex("basic_deployed_index", {
* indexEndpoint: vertexIndexEndpointDeployed.id,
* index: index.id,
* deployedIndexId: "deployed_index_id",
* reservedIpRanges: ["vertex-ai-range"],
* enableAccessLogging: false,
* displayName: "vertex-deployed-index",
* deployedIndexAuthConfig: {
* authProvider: {
* audiences: ["123456-my-app"],
* allowedIssuers: [sa.email],
* },
* },
* }, {
* dependsOn: [
* vertexIndexEndpointDeployed,
* sa,
* ],
* });
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* const data = new gcp.storage.BucketObject("data", {
* name: "contents/data.json",
* bucket: bucket.name,
* content: `{"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* sa = gcp.serviceaccount.Account("sa", account_id="vertex-sa")
* bucket = gcp.storage.Bucket("bucket",
* name="bucket-name",
* location="us-central1",
* uniform_bucket_level_access=True)
* index = gcp.vertex.AiIndex("index",
* labels={
* "foo": "bar",
* },
* region="us-central1",
* display_name="test-index",
* description="index for test",
* metadata={
* "contents_delta_uri": bucket.name.apply(lambda name: f"gs://{name}/contents"),
* "config": {
* "dimensions": 2,
* "approximate_neighbors_count": 150,
* "shard_size": "SHARD_SIZE_SMALL",
* "distance_measure_type": "DOT_PRODUCT_DISTANCE",
* "algorithm_config": {
* "tree_ah_config": {
* "leaf_node_embedding_count": 500,
* "leaf_nodes_to_search_percent": 7,
* },
* },
* },
* },
* index_update_method="BATCH_UPDATE")
* vertex_network = gcp.compute.get_network(name="network-name")
* project = gcp.organizations.get_project()
* vertex_index_endpoint_deployed = gcp.vertex.AiIndexEndpoint("vertex_index_endpoint_deployed",
* display_name="sample-endpoint",
* description="A sample vertex endpoint",
* region="us-central1",
* labels={
* "label-one": "value-one",
* },
* network=f"projects/{project.number}/global/networks/{vertex_network.name}")
* basic_deployed_index = gcp.vertex.AiIndexEndpointDeployedIndex("basic_deployed_index",
* index_endpoint=vertex_index_endpoint_deployed.id,
* index=index.id,
* deployed_index_id="deployed_index_id",
* reserved_ip_ranges=["vertex-ai-range"],
* enable_access_logging=False,
* display_name="vertex-deployed-index",
* deployed_index_auth_config={
* "auth_provider": {
* "audiences": ["123456-my-app"],
* "allowed_issuers": [sa.email],
* },
* },
* opts = pulumi.ResourceOptions(depends_on=[
* vertex_index_endpoint_deployed,
* sa,
* ]))
* # The sample data comes from the following link:
* # https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* data = gcp.storage.BucketObject("data",
* name="contents/data.json",
* bucket=bucket.name,
* content="""{"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var sa = new Gcp.ServiceAccount.Account("sa", new()
* {
* AccountId = "vertex-sa",
* });
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "bucket-name",
* Location = "us-central1",
* UniformBucketLevelAccess = true,
* });
* var index = new Gcp.Vertex.AiIndex("index", new()
* {
* Labels =
* {
* { "foo", "bar" },
* },
* Region = "us-central1",
* DisplayName = "test-index",
* Description = "index for test",
* Metadata = new Gcp.Vertex.Inputs.AiIndexMetadataArgs
* {
* ContentsDeltaUri = bucket.Name.Apply(name => $"gs://{name}/contents"),
* Config = new Gcp.Vertex.Inputs.AiIndexMetadataConfigArgs
* {
* Dimensions = 2,
* ApproximateNeighborsCount = 150,
* ShardSize = "SHARD_SIZE_SMALL",
* DistanceMeasureType = "DOT_PRODUCT_DISTANCE",
* AlgorithmConfig = new Gcp.Vertex.Inputs.AiIndexMetadataConfigAlgorithmConfigArgs
* {
* TreeAhConfig = new Gcp.Vertex.Inputs.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs
* {
* LeafNodeEmbeddingCount = 500,
* LeafNodesToSearchPercent = 7,
* },
* },
* },
* },
* IndexUpdateMethod = "BATCH_UPDATE",
* });
* var vertexNetwork = Gcp.Compute.GetNetwork.Invoke(new()
* {
* Name = "network-name",
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var vertexIndexEndpointDeployed = new Gcp.Vertex.AiIndexEndpoint("vertex_index_endpoint_deployed", new()
* {
* DisplayName = "sample-endpoint",
* Description = "A sample vertex endpoint",
* Region = "us-central1",
* Labels =
* {
* { "label-one", "value-one" },
* },
* Network = Output.Tuple(project, vertexNetwork).Apply(values =>
* {
* var project = values.Item1;
* var vertexNetwork = values.Item2;
* return $"projects/{project.Apply(getProjectResult => getProjectResult.Number)}/global/networks/{vertexNetwork.Apply(getNetworkResult => getNetworkResult.Name)}";
* }),
* });
* var basicDeployedIndex = new Gcp.Vertex.AiIndexEndpointDeployedIndex("basic_deployed_index", new()
* {
* IndexEndpoint = vertexIndexEndpointDeployed.Id,
* Index = index.Id,
* DeployedIndexId = "deployed_index_id",
* ReservedIpRanges = new[]
* {
* "vertex-ai-range",
* },
* EnableAccessLogging = false,
* DisplayName = "vertex-deployed-index",
* DeployedIndexAuthConfig = new Gcp.Vertex.Inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs
* {
* AuthProvider = new Gcp.Vertex.Inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs
* {
* Audiences = new[]
* {
* "123456-my-app",
* },
* AllowedIssuers = new[]
* {
* sa.Email,
* },
* },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* vertexIndexEndpointDeployed,
* sa,
* },
* });
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* var data = new Gcp.Storage.BucketObject("data", new()
* {
* Name = "contents/data.json",
* Bucket = bucket.Name,
* Content = @"{""id"": ""42"", ""embedding"": [0.5, 1.0], ""restricts"": [{""namespace"": ""class"", ""allow"": [""cat"", ""pet""]},{""namespace"": ""category"", ""allow"": [""feline""]}]}
* {""id"": ""43"", ""embedding"": [0.6, 1.0], ""restricts"": [{""namespace"": ""class"", ""allow"": [""dog"", ""pet""]},{""namespace"": ""category"", ""allow"": [""canine""]}]}
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/storage"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/vertex"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* sa, err := serviceaccount.NewAccount(ctx, "sa", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("vertex-sa"),
* })
* if err != nil {
* return err
* }
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("bucket-name"),
* Location: pulumi.String("us-central1"),
* UniformBucketLevelAccess: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* index, err := vertex.NewAiIndex(ctx, "index", &vertex.AiIndexArgs{
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Region: pulumi.String("us-central1"),
* DisplayName: pulumi.String("test-index"),
* Description: pulumi.String("index for test"),
* Metadata: &vertex.AiIndexMetadataArgs{
* ContentsDeltaUri: bucket.Name.ApplyT(func(name string) (string, error) {
* return fmt.Sprintf("gs://%v/contents", name), nil
* }).(pulumi.StringOutput),
* Config: &vertex.AiIndexMetadataConfigArgs{
* Dimensions: pulumi.Int(2),
* ApproximateNeighborsCount: pulumi.Int(150),
* ShardSize: pulumi.String("SHARD_SIZE_SMALL"),
* DistanceMeasureType: pulumi.String("DOT_PRODUCT_DISTANCE"),
* AlgorithmConfig: &vertex.AiIndexMetadataConfigAlgorithmConfigArgs{
* TreeAhConfig: &vertex.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs{
* LeafNodeEmbeddingCount: pulumi.Int(500),
* LeafNodesToSearchPercent: pulumi.Int(7),
* },
* },
* },
* },
* IndexUpdateMethod: pulumi.String("BATCH_UPDATE"),
* })
* if err != nil {
* return err
* }
* vertexNetwork, err := compute.LookupNetwork(ctx, &compute.LookupNetworkArgs{
* Name: "network-name",
* }, nil)
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, &organizations.LookupProjectArgs{}, nil)
* if err != nil {
* return err
* }
* vertexIndexEndpointDeployed, err := vertex.NewAiIndexEndpoint(ctx, "vertex_index_endpoint_deployed", &vertex.AiIndexEndpointArgs{
* DisplayName: pulumi.String("sample-endpoint"),
* Description: pulumi.String("A sample vertex endpoint"),
* Region: pulumi.String("us-central1"),
* Labels: pulumi.StringMap{
* "label-one": pulumi.String("value-one"),
* },
* Network: pulumi.Sprintf("projects/%v/global/networks/%v", project.Number, vertexNetwork.Name),
* })
* if err != nil {
* return err
* }
* _, err = vertex.NewAiIndexEndpointDeployedIndex(ctx, "basic_deployed_index", &vertex.AiIndexEndpointDeployedIndexArgs{
* IndexEndpoint: vertexIndexEndpointDeployed.ID(),
* Index: index.ID(),
* DeployedIndexId: pulumi.String("deployed_index_id"),
* ReservedIpRanges: pulumi.StringArray{
* pulumi.String("vertex-ai-range"),
* },
* EnableAccessLogging: pulumi.Bool(false),
* DisplayName: pulumi.String("vertex-deployed-index"),
* DeployedIndexAuthConfig: &vertex.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs{
* AuthProvider: &vertex.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs{
* Audiences: pulumi.StringArray{
* pulumi.String("123456-my-app"),
* },
* AllowedIssuers: pulumi.StringArray{
* sa.Email,
* },
* },
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* vertexIndexEndpointDeployed,
* sa,
* }))
* if err != nil {
* return err
* }
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* _, err = storage.NewBucketObject(ctx, "data", &storage.BucketObjectArgs{
* Name: pulumi.String("contents/data.json"),
* Bucket: bucket.Name,
* Content: pulumi.String("{\"id\": \"42\", \"embedding\": [0.5, 1.0], \"restricts\": [{\"namespace\": \"class\", \"allow\": [\"cat\", \"pet\"]},{\"namespace\": \"category\", \"allow\": [\"feline\"]}]}\n{\"id\": \"43\", \"embedding\": [0.6, 1.0], \"restricts\": [{\"namespace\": \"class\", \"allow\": [\"dog\", \"pet\"]},{\"namespace\": \"category\", \"allow\": [\"canine\"]}]}\n"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.vertex.AiIndex;
* import com.pulumi.gcp.vertex.AiIndexArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigAlgorithmConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetNetworkArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.vertex.AiIndexEndpoint;
* import com.pulumi.gcp.vertex.AiIndexEndpointArgs;
* import com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndex;
* import com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndexArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs;
* import com.pulumi.gcp.storage.BucketObject;
* import com.pulumi.gcp.storage.BucketObjectArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sa = new Account("sa", AccountArgs.builder()
* .accountId("vertex-sa")
* .build());
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("bucket-name")
* .location("us-central1")
* .uniformBucketLevelAccess(true)
* .build());
* var index = new AiIndex("index", AiIndexArgs.builder()
* .labels(Map.of("foo", "bar"))
* .region("us-central1")
* .displayName("test-index")
* .description("index for test")
* .metadata(AiIndexMetadataArgs.builder()
* .contentsDeltaUri(bucket.name().applyValue(name -> String.format("gs://%s/contents", name)))
* .config(AiIndexMetadataConfigArgs.builder()
* .dimensions(2)
* .approximateNeighborsCount(150)
* .shardSize("SHARD_SIZE_SMALL")
* .distanceMeasureType("DOT_PRODUCT_DISTANCE")
* .algorithmConfig(AiIndexMetadataConfigAlgorithmConfigArgs.builder()
* .treeAhConfig(AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs.builder()
* .leafNodeEmbeddingCount(500)
* .leafNodesToSearchPercent(7)
* .build())
* .build())
* .build())
* .build())
* .indexUpdateMethod("BATCH_UPDATE")
* .build());
* final var vertexNetwork = ComputeFunctions.getNetwork(GetNetworkArgs.builder()
* .name("network-name")
* .build());
* final var project = OrganizationsFunctions.getProject();
* var vertexIndexEndpointDeployed = new AiIndexEndpoint("vertexIndexEndpointDeployed", AiIndexEndpointArgs.builder()
* .displayName("sample-endpoint")
* .description("A sample vertex endpoint")
* .region("us-central1")
* .labels(Map.of("label-one", "value-one"))
* .network(String.format("projects/%s/global/networks/%s", project.applyValue(getProjectResult -> getProjectResult.number()),vertexNetwork.applyValue(getNetworkResult -> getNetworkResult.name())))
* .build());
* var basicDeployedIndex = new AiIndexEndpointDeployedIndex("basicDeployedIndex", AiIndexEndpointDeployedIndexArgs.builder()
* .indexEndpoint(vertexIndexEndpointDeployed.id())
* .index(index.id())
* .deployedIndexId("deployed_index_id")
* .reservedIpRanges("vertex-ai-range")
* .enableAccessLogging(false)
* .displayName("vertex-deployed-index")
* .deployedIndexAuthConfig(AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs.builder()
* .authProvider(AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs.builder()
* .audiences("123456-my-app")
* .allowedIssuers(sa.email())
* .build())
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* vertexIndexEndpointDeployed,
* sa)
* .build());
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* var data = new BucketObject("data", BucketObjectArgs.builder()
* .name("contents/data.json")
* .bucket(bucket.name())
* .content("""
* {"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* sa:
* type: gcp:serviceaccount:Account
* properties:
* accountId: vertex-sa
* basicDeployedIndex:
* type: gcp:vertex:AiIndexEndpointDeployedIndex
* name: basic_deployed_index
* properties:
* indexEndpoint: ${vertexIndexEndpointDeployed.id}
* index: ${index.id}
* deployedIndexId: deployed_index_id
* reservedIpRanges:
* - vertex-ai-range
* enableAccessLogging: false
* displayName: vertex-deployed-index
* deployedIndexAuthConfig:
* authProvider:
* audiences:
* - 123456-my-app
* allowedIssuers:
* - ${sa.email}
* options:
* dependsOn:
* - ${vertexIndexEndpointDeployed}
* - ${sa}
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: bucket-name
* location: us-central1
* uniformBucketLevelAccess: true
* # The sample data comes from the following link:
* # https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* data:
* type: gcp:storage:BucketObject
* properties:
* name: contents/data.json
* bucket: ${bucket.name}
* content: |
* {"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* index:
* type: gcp:vertex:AiIndex
* properties:
* labels:
* foo: bar
* region: us-central1
* displayName: test-index
* description: index for test
* metadata:
* contentsDeltaUri: gs://${bucket.name}/contents
* config:
* dimensions: 2
* approximateNeighborsCount: 150
* shardSize: SHARD_SIZE_SMALL
* distanceMeasureType: DOT_PRODUCT_DISTANCE
* algorithmConfig:
* treeAhConfig:
* leafNodeEmbeddingCount: 500
* leafNodesToSearchPercent: 7
* indexUpdateMethod: BATCH_UPDATE
* vertexIndexEndpointDeployed:
* type: gcp:vertex:AiIndexEndpoint
* name: vertex_index_endpoint_deployed
* properties:
* displayName: sample-endpoint
* description: A sample vertex endpoint
* region: us-central1
* labels:
* label-one: value-one
* network: projects/${project.number}/global/networks/${vertexNetwork.name}
* variables:
* vertexNetwork:
* fn::invoke:
* function: gcp:compute:getNetwork
* arguments:
* name: network-name
* project:
* fn::invoke:
* function: gcp:organizations:getProject
* arguments: {}
* ```
*
* ### Vertex Ai Index Endpoint Deployed Index Basic Two
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const sa = new gcp.serviceaccount.Account("sa", {accountId: "vertex-sa"});
* const bucket = new gcp.storage.Bucket("bucket", {
* name: "bucket-name",
* location: "us-central1",
* uniformBucketLevelAccess: true,
* });
* const index = new gcp.vertex.AiIndex("index", {
* labels: {
* foo: "bar",
* },
* region: "us-central1",
* displayName: "test-index",
* description: "index for test",
* metadata: {
* contentsDeltaUri: pulumi.interpolate`gs://${bucket.name}/contents`,
* config: {
* dimensions: 2,
* approximateNeighborsCount: 150,
* shardSize: "SHARD_SIZE_SMALL",
* distanceMeasureType: "DOT_PRODUCT_DISTANCE",
* algorithmConfig: {
* treeAhConfig: {
* leafNodeEmbeddingCount: 500,
* leafNodesToSearchPercent: 7,
* },
* },
* },
* },
* indexUpdateMethod: "BATCH_UPDATE",
* });
* const vertexNetwork = gcp.compute.getNetwork({
* name: "network-name",
* });
* const project = gcp.organizations.getProject({});
* const vertexIndexEndpointDeployed = new gcp.vertex.AiIndexEndpoint("vertex_index_endpoint_deployed", {
* displayName: "sample-endpoint",
* description: "A sample vertex endpoint",
* region: "us-central1",
* labels: {
* "label-one": "value-one",
* },
* network: Promise.all([project, vertexNetwork]).then(([project, vertexNetwork]) => `projects/${project.number}/global/networks/${vertexNetwork.name}`),
* });
* const basicDeployedIndex = new gcp.vertex.AiIndexEndpointDeployedIndex("basic_deployed_index", {
* indexEndpoint: vertexIndexEndpointDeployed.id,
* index: index.id,
* deployedIndexId: "deployed_index_id",
* reservedIpRanges: ["vertex-ai-range"],
* enableAccessLogging: false,
* displayName: "vertex-deployed-index",
* deployedIndexAuthConfig: {
* authProvider: {
* audiences: ["123456-my-app"],
* allowedIssuers: [sa.email],
* },
* },
* automaticResources: {
* maxReplicaCount: 4,
* },
* }, {
* dependsOn: [
* vertexIndexEndpointDeployed,
* sa,
* ],
* });
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* const data = new gcp.storage.BucketObject("data", {
* name: "contents/data.json",
* bucket: bucket.name,
* content: `{"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* sa = gcp.serviceaccount.Account("sa", account_id="vertex-sa")
* bucket = gcp.storage.Bucket("bucket",
* name="bucket-name",
* location="us-central1",
* uniform_bucket_level_access=True)
* index = gcp.vertex.AiIndex("index",
* labels={
* "foo": "bar",
* },
* region="us-central1",
* display_name="test-index",
* description="index for test",
* metadata={
* "contents_delta_uri": bucket.name.apply(lambda name: f"gs://{name}/contents"),
* "config": {
* "dimensions": 2,
* "approximate_neighbors_count": 150,
* "shard_size": "SHARD_SIZE_SMALL",
* "distance_measure_type": "DOT_PRODUCT_DISTANCE",
* "algorithm_config": {
* "tree_ah_config": {
* "leaf_node_embedding_count": 500,
* "leaf_nodes_to_search_percent": 7,
* },
* },
* },
* },
* index_update_method="BATCH_UPDATE")
* vertex_network = gcp.compute.get_network(name="network-name")
* project = gcp.organizations.get_project()
* vertex_index_endpoint_deployed = gcp.vertex.AiIndexEndpoint("vertex_index_endpoint_deployed",
* display_name="sample-endpoint",
* description="A sample vertex endpoint",
* region="us-central1",
* labels={
* "label-one": "value-one",
* },
* network=f"projects/{project.number}/global/networks/{vertex_network.name}")
* basic_deployed_index = gcp.vertex.AiIndexEndpointDeployedIndex("basic_deployed_index",
* index_endpoint=vertex_index_endpoint_deployed.id,
* index=index.id,
* deployed_index_id="deployed_index_id",
* reserved_ip_ranges=["vertex-ai-range"],
* enable_access_logging=False,
* display_name="vertex-deployed-index",
* deployed_index_auth_config={
* "auth_provider": {
* "audiences": ["123456-my-app"],
* "allowed_issuers": [sa.email],
* },
* },
* automatic_resources={
* "max_replica_count": 4,
* },
* opts = pulumi.ResourceOptions(depends_on=[
* vertex_index_endpoint_deployed,
* sa,
* ]))
* # The sample data comes from the following link:
* # https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* data = gcp.storage.BucketObject("data",
* name="contents/data.json",
* bucket=bucket.name,
* content="""{"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var sa = new Gcp.ServiceAccount.Account("sa", new()
* {
* AccountId = "vertex-sa",
* });
* var bucket = new Gcp.Storage.Bucket("bucket", new()
* {
* Name = "bucket-name",
* Location = "us-central1",
* UniformBucketLevelAccess = true,
* });
* var index = new Gcp.Vertex.AiIndex("index", new()
* {
* Labels =
* {
* { "foo", "bar" },
* },
* Region = "us-central1",
* DisplayName = "test-index",
* Description = "index for test",
* Metadata = new Gcp.Vertex.Inputs.AiIndexMetadataArgs
* {
* ContentsDeltaUri = bucket.Name.Apply(name => $"gs://{name}/contents"),
* Config = new Gcp.Vertex.Inputs.AiIndexMetadataConfigArgs
* {
* Dimensions = 2,
* ApproximateNeighborsCount = 150,
* ShardSize = "SHARD_SIZE_SMALL",
* DistanceMeasureType = "DOT_PRODUCT_DISTANCE",
* AlgorithmConfig = new Gcp.Vertex.Inputs.AiIndexMetadataConfigAlgorithmConfigArgs
* {
* TreeAhConfig = new Gcp.Vertex.Inputs.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs
* {
* LeafNodeEmbeddingCount = 500,
* LeafNodesToSearchPercent = 7,
* },
* },
* },
* },
* IndexUpdateMethod = "BATCH_UPDATE",
* });
* var vertexNetwork = Gcp.Compute.GetNetwork.Invoke(new()
* {
* Name = "network-name",
* });
* var project = Gcp.Organizations.GetProject.Invoke();
* var vertexIndexEndpointDeployed = new Gcp.Vertex.AiIndexEndpoint("vertex_index_endpoint_deployed", new()
* {
* DisplayName = "sample-endpoint",
* Description = "A sample vertex endpoint",
* Region = "us-central1",
* Labels =
* {
* { "label-one", "value-one" },
* },
* Network = Output.Tuple(project, vertexNetwork).Apply(values =>
* {
* var project = values.Item1;
* var vertexNetwork = values.Item2;
* return $"projects/{project.Apply(getProjectResult => getProjectResult.Number)}/global/networks/{vertexNetwork.Apply(getNetworkResult => getNetworkResult.Name)}";
* }),
* });
* var basicDeployedIndex = new Gcp.Vertex.AiIndexEndpointDeployedIndex("basic_deployed_index", new()
* {
* IndexEndpoint = vertexIndexEndpointDeployed.Id,
* Index = index.Id,
* DeployedIndexId = "deployed_index_id",
* ReservedIpRanges = new[]
* {
* "vertex-ai-range",
* },
* EnableAccessLogging = false,
* DisplayName = "vertex-deployed-index",
* DeployedIndexAuthConfig = new Gcp.Vertex.Inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs
* {
* AuthProvider = new Gcp.Vertex.Inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs
* {
* Audiences = new[]
* {
* "123456-my-app",
* },
* AllowedIssuers = new[]
* {
* sa.Email,
* },
* },
* },
* AutomaticResources = new Gcp.Vertex.Inputs.AiIndexEndpointDeployedIndexAutomaticResourcesArgs
* {
* MaxReplicaCount = 4,
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* vertexIndexEndpointDeployed,
* sa,
* },
* });
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* var data = new Gcp.Storage.BucketObject("data", new()
* {
* Name = "contents/data.json",
* Bucket = bucket.Name,
* Content = @"{""id"": ""42"", ""embedding"": [0.5, 1.0], ""restricts"": [{""namespace"": ""class"", ""allow"": [""cat"", ""pet""]},{""namespace"": ""category"", ""allow"": [""feline""]}]}
* {""id"": ""43"", ""embedding"": [0.6, 1.0], ""restricts"": [{""namespace"": ""class"", ""allow"": [""dog"", ""pet""]},{""namespace"": ""category"", ""allow"": [""canine""]}]}
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/organizations"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/storage"
* "github.com/pulumi/pulumi-gcp/sdk/v8/go/gcp/vertex"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* sa, err := serviceaccount.NewAccount(ctx, "sa", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("vertex-sa"),
* })
* if err != nil {
* return err
* }
* bucket, err := storage.NewBucket(ctx, "bucket", &storage.BucketArgs{
* Name: pulumi.String("bucket-name"),
* Location: pulumi.String("us-central1"),
* UniformBucketLevelAccess: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* index, err := vertex.NewAiIndex(ctx, "index", &vertex.AiIndexArgs{
* Labels: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* Region: pulumi.String("us-central1"),
* DisplayName: pulumi.String("test-index"),
* Description: pulumi.String("index for test"),
* Metadata: &vertex.AiIndexMetadataArgs{
* ContentsDeltaUri: bucket.Name.ApplyT(func(name string) (string, error) {
* return fmt.Sprintf("gs://%v/contents", name), nil
* }).(pulumi.StringOutput),
* Config: &vertex.AiIndexMetadataConfigArgs{
* Dimensions: pulumi.Int(2),
* ApproximateNeighborsCount: pulumi.Int(150),
* ShardSize: pulumi.String("SHARD_SIZE_SMALL"),
* DistanceMeasureType: pulumi.String("DOT_PRODUCT_DISTANCE"),
* AlgorithmConfig: &vertex.AiIndexMetadataConfigAlgorithmConfigArgs{
* TreeAhConfig: &vertex.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs{
* LeafNodeEmbeddingCount: pulumi.Int(500),
* LeafNodesToSearchPercent: pulumi.Int(7),
* },
* },
* },
* },
* IndexUpdateMethod: pulumi.String("BATCH_UPDATE"),
* })
* if err != nil {
* return err
* }
* vertexNetwork, err := compute.LookupNetwork(ctx, &compute.LookupNetworkArgs{
* Name: "network-name",
* }, nil)
* if err != nil {
* return err
* }
* project, err := organizations.LookupProject(ctx, &organizations.LookupProjectArgs{}, nil)
* if err != nil {
* return err
* }
* vertexIndexEndpointDeployed, err := vertex.NewAiIndexEndpoint(ctx, "vertex_index_endpoint_deployed", &vertex.AiIndexEndpointArgs{
* DisplayName: pulumi.String("sample-endpoint"),
* Description: pulumi.String("A sample vertex endpoint"),
* Region: pulumi.String("us-central1"),
* Labels: pulumi.StringMap{
* "label-one": pulumi.String("value-one"),
* },
* Network: pulumi.Sprintf("projects/%v/global/networks/%v", project.Number, vertexNetwork.Name),
* })
* if err != nil {
* return err
* }
* _, err = vertex.NewAiIndexEndpointDeployedIndex(ctx, "basic_deployed_index", &vertex.AiIndexEndpointDeployedIndexArgs{
* IndexEndpoint: vertexIndexEndpointDeployed.ID(),
* Index: index.ID(),
* DeployedIndexId: pulumi.String("deployed_index_id"),
* ReservedIpRanges: pulumi.StringArray{
* pulumi.String("vertex-ai-range"),
* },
* EnableAccessLogging: pulumi.Bool(false),
* DisplayName: pulumi.String("vertex-deployed-index"),
* DeployedIndexAuthConfig: &vertex.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs{
* AuthProvider: &vertex.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs{
* Audiences: pulumi.StringArray{
* pulumi.String("123456-my-app"),
* },
* AllowedIssuers: pulumi.StringArray{
* sa.Email,
* },
* },
* },
* AutomaticResources: &vertex.AiIndexEndpointDeployedIndexAutomaticResourcesArgs{
* MaxReplicaCount: pulumi.Int(4),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* vertexIndexEndpointDeployed,
* sa,
* }))
* if err != nil {
* return err
* }
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* _, err = storage.NewBucketObject(ctx, "data", &storage.BucketObjectArgs{
* Name: pulumi.String("contents/data.json"),
* Bucket: bucket.Name,
* Content: pulumi.String("{\"id\": \"42\", \"embedding\": [0.5, 1.0], \"restricts\": [{\"namespace\": \"class\", \"allow\": [\"cat\", \"pet\"]},{\"namespace\": \"category\", \"allow\": [\"feline\"]}]}\n{\"id\": \"43\", \"embedding\": [0.6, 1.0], \"restricts\": [{\"namespace\": \"class\", \"allow\": [\"dog\", \"pet\"]},{\"namespace\": \"category\", \"allow\": [\"canine\"]}]}\n"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.vertex.AiIndex;
* import com.pulumi.gcp.vertex.AiIndexArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigAlgorithmConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetNetworkArgs;
* import com.pulumi.gcp.organizations.OrganizationsFunctions;
* import com.pulumi.gcp.organizations.inputs.GetProjectArgs;
* import com.pulumi.gcp.vertex.AiIndexEndpoint;
* import com.pulumi.gcp.vertex.AiIndexEndpointArgs;
* import com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndex;
* import com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndexArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs;
* import com.pulumi.gcp.vertex.inputs.AiIndexEndpointDeployedIndexAutomaticResourcesArgs;
* import com.pulumi.gcp.storage.BucketObject;
* import com.pulumi.gcp.storage.BucketObjectArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sa = new Account("sa", AccountArgs.builder()
* .accountId("vertex-sa")
* .build());
* var bucket = new Bucket("bucket", BucketArgs.builder()
* .name("bucket-name")
* .location("us-central1")
* .uniformBucketLevelAccess(true)
* .build());
* var index = new AiIndex("index", AiIndexArgs.builder()
* .labels(Map.of("foo", "bar"))
* .region("us-central1")
* .displayName("test-index")
* .description("index for test")
* .metadata(AiIndexMetadataArgs.builder()
* .contentsDeltaUri(bucket.name().applyValue(name -> String.format("gs://%s/contents", name)))
* .config(AiIndexMetadataConfigArgs.builder()
* .dimensions(2)
* .approximateNeighborsCount(150)
* .shardSize("SHARD_SIZE_SMALL")
* .distanceMeasureType("DOT_PRODUCT_DISTANCE")
* .algorithmConfig(AiIndexMetadataConfigAlgorithmConfigArgs.builder()
* .treeAhConfig(AiIndexMetadataConfigAlgorithmConfigTreeAhConfigArgs.builder()
* .leafNodeEmbeddingCount(500)
* .leafNodesToSearchPercent(7)
* .build())
* .build())
* .build())
* .build())
* .indexUpdateMethod("BATCH_UPDATE")
* .build());
* final var vertexNetwork = ComputeFunctions.getNetwork(GetNetworkArgs.builder()
* .name("network-name")
* .build());
* final var project = OrganizationsFunctions.getProject();
* var vertexIndexEndpointDeployed = new AiIndexEndpoint("vertexIndexEndpointDeployed", AiIndexEndpointArgs.builder()
* .displayName("sample-endpoint")
* .description("A sample vertex endpoint")
* .region("us-central1")
* .labels(Map.of("label-one", "value-one"))
* .network(String.format("projects/%s/global/networks/%s", project.applyValue(getProjectResult -> getProjectResult.number()),vertexNetwork.applyValue(getNetworkResult -> getNetworkResult.name())))
* .build());
* var basicDeployedIndex = new AiIndexEndpointDeployedIndex("basicDeployedIndex", AiIndexEndpointDeployedIndexArgs.builder()
* .indexEndpoint(vertexIndexEndpointDeployed.id())
* .index(index.id())
* .deployedIndexId("deployed_index_id")
* .reservedIpRanges("vertex-ai-range")
* .enableAccessLogging(false)
* .displayName("vertex-deployed-index")
* .deployedIndexAuthConfig(AiIndexEndpointDeployedIndexDeployedIndexAuthConfigArgs.builder()
* .authProvider(AiIndexEndpointDeployedIndexDeployedIndexAuthConfigAuthProviderArgs.builder()
* .audiences("123456-my-app")
* .allowedIssuers(sa.email())
* .build())
* .build())
* .automaticResources(AiIndexEndpointDeployedIndexAutomaticResourcesArgs.builder()
* .maxReplicaCount(4)
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* vertexIndexEndpointDeployed,
* sa)
* .build());
* // The sample data comes from the following link:
* // https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* var data = new BucketObject("data", BucketObjectArgs.builder()
* .name("contents/data.json")
* .bucket(bucket.name())
* .content("""
* {"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* sa:
* type: gcp:serviceaccount:Account
* properties:
* accountId: vertex-sa
* basicDeployedIndex:
* type: gcp:vertex:AiIndexEndpointDeployedIndex
* name: basic_deployed_index
* properties:
* indexEndpoint: ${vertexIndexEndpointDeployed.id}
* index: ${index.id}
* deployedIndexId: deployed_index_id
* reservedIpRanges:
* - vertex-ai-range
* enableAccessLogging: false
* displayName: vertex-deployed-index
* deployedIndexAuthConfig:
* authProvider:
* audiences:
* - 123456-my-app
* allowedIssuers:
* - ${sa.email}
* automaticResources:
* maxReplicaCount: 4
* options:
* dependsOn:
* - ${vertexIndexEndpointDeployed}
* - ${sa}
* bucket:
* type: gcp:storage:Bucket
* properties:
* name: bucket-name
* location: us-central1
* uniformBucketLevelAccess: true
* # The sample data comes from the following link:
* # https://cloud.google.com/vertex-ai/docs/matching-engine/filtering#specify-namespaces-tokens
* data:
* type: gcp:storage:BucketObject
* properties:
* name: contents/data.json
* bucket: ${bucket.name}
* content: |
* {"id": "42", "embedding": [0.5, 1.0], "restricts": [{"namespace": "class", "allow": ["cat", "pet"]},{"namespace": "category", "allow": ["feline"]}]}
* {"id": "43", "embedding": [0.6, 1.0], "restricts": [{"namespace": "class", "allow": ["dog", "pet"]},{"namespace": "category", "allow": ["canine"]}]}
* index:
* type: gcp:vertex:AiIndex
* properties:
* labels:
* foo: bar
* region: us-central1
* displayName: test-index
* description: index for test
* metadata:
* contentsDeltaUri: gs://${bucket.name}/contents
* config:
* dimensions: 2
* approximateNeighborsCount: 150
* shardSize: SHARD_SIZE_SMALL
* distanceMeasureType: DOT_PRODUCT_DISTANCE
* algorithmConfig:
* treeAhConfig:
* leafNodeEmbeddingCount: 500
* leafNodesToSearchPercent: 7
* indexUpdateMethod: BATCH_UPDATE
* vertexIndexEndpointDeployed:
* type: gcp:vertex:AiIndexEndpoint
* name: vertex_index_endpoint_deployed
* properties:
* displayName: sample-endpoint
* description: A sample vertex endpoint
* region: us-central1
* labels:
* label-one: value-one
* network: projects/${project.number}/global/networks/${vertexNetwork.name}
* variables:
* vertexNetwork:
* fn::invoke:
* function: gcp:compute:getNetwork
* arguments:
* name: network-name
* project:
* fn::invoke:
* function: gcp:organizations:getProject
* arguments: {}
* ```
*
* ## Import
* IndexEndpointDeployedIndex can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{region}}/indexEndpoints/{{index_endpoint}}/deployedIndex/{{deployed_index_id}}`
* * `{{project}}/{{region}}/{{index_endpoint}}/{{deployed_index_id}}`
* * `{{region}}/{{index_endpoint}}/{{deployed_index_id}}`
* * `{{index_endpoint}}/{{deployed_index_id}}`
* When using the `pulumi import` command, IndexEndpointDeployedIndex can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:vertex/aiIndexEndpointDeployedIndex:AiIndexEndpointDeployedIndex default projects/{{project}}/locations/{{region}}/indexEndpoints/{{index_endpoint}}/deployedIndex/{{deployed_index_id}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiIndexEndpointDeployedIndex:AiIndexEndpointDeployedIndex default {{project}}/{{region}}/{{index_endpoint}}/{{deployed_index_id}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiIndexEndpointDeployedIndex:AiIndexEndpointDeployedIndex default {{region}}/{{index_endpoint}}/{{deployed_index_id}}
* ```
* ```sh
* $ pulumi import gcp:vertex/aiIndexEndpointDeployedIndex:AiIndexEndpointDeployedIndex default {{index_endpoint}}/{{deployed_index_id}}
* ```
* @property automaticResources A description of resources that the DeployedIndex uses, which to large degree are decided by Vertex AI, and optionally allows only a modest additional configuration.
* Structure is documented below.
* @property dedicatedResources A description of resources that are dedicated to the DeployedIndex, and that need a higher degree of manual configuration. The field minReplicaCount must be set to a value strictly greater than 0, or else validation will fail. We don't provide SLA when minReplicaCount=1. If maxReplicaCount is not set, the default value is minReplicaCount. The max allowed replica count is 1000.
* Available machine types for SMALL shard: e2-standard-2 and all machine types available for MEDIUM and LARGE shard.
* Available machine types for MEDIUM shard: e2-standard-16 and all machine types available for LARGE shard.
* Available machine types for LARGE shard: e2-highmem-16, n2d-standard-32.
* n1-standard-16 and n1-standard-32 are still available, but we recommend e2-standard-16 and e2-highmem-16 for cost efficiency.
* Structure is documented below.
* @property deployedIndexAuthConfig If set, the authentication is enabled for the private endpoint.
* Structure is documented below.
* @property deployedIndexId The user specified ID of the DeployedIndex. The ID can be up to 128 characters long and must start with a letter and only contain letters, numbers, and underscores. The ID must be unique within the project it is created in.
* @property deploymentGroup The deployment group can be no longer than 64 characters (eg: 'test', 'prod'). If not set, we will use the 'default' deployment group.
* Creating deployment_groups with reserved_ip_ranges is a recommended practice when the peered network has multiple peering ranges. This creates your deployments from predictable IP spaces for easier traffic administration. Also, one deployment_group (except 'default') can only be used with the same reserved_ip_ranges which means if the deployment_group has been used with reserved_ip_ranges: [a, b, c], using it with [a, b] or [d, e] is disallowed. [See the official documentation here](https://cloud.google.com/vertex-ai/docs/reference/rest/v1/projects.locations.indexEndpoints#DeployedIndex.FIELDS.deployment_group).
* Note: we only support up to 5 deployment groups (not including 'default').
* @property displayName The display name of the Index. The name can be up to 128 characters long and can consist of any UTF-8 characters.
* @property enableAccessLogging If true, private endpoint's access logs are sent to Cloud Logging.
* @property index The name of the Index this is the deployment of.
* @property indexEndpoint Identifies the index endpoint. Must be in the format
* 'projects/{{project}}/locations/{{region}}/indexEndpoints/{{indexEndpoint}}'
* - - -
* @property reservedIpRanges A list of reserved ip ranges under the VPC network that can be used for this DeployedIndex.
* If set, we will deploy the index within the provided ip ranges. Otherwise, the index might be deployed to any ip ranges under the provided VPC network.
* The value should be the name of the address (https://cloud.google.com/compute/docs/reference/rest/v1/addresses) Example: ['vertex-ai-ip-range'].
* For more information about subnets and network IP ranges, please see https://cloud.google.com/vpc/docs/subnets#manually_created_subnet_ip_ranges.
*/
public data class AiIndexEndpointDeployedIndexArgs(
public val automaticResources: Output? = null,
public val dedicatedResources: Output? = null,
public val deployedIndexAuthConfig: Output? = null,
public val deployedIndexId: Output? = null,
public val deploymentGroup: Output? = null,
public val displayName: Output? = null,
public val enableAccessLogging: Output? = null,
public val index: Output? = null,
public val indexEndpoint: Output? = null,
public val reservedIpRanges: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndexArgs =
com.pulumi.gcp.vertex.AiIndexEndpointDeployedIndexArgs.builder()
.automaticResources(
automaticResources?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.dedicatedResources(
dedicatedResources?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.deployedIndexAuthConfig(
deployedIndexAuthConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.deployedIndexId(deployedIndexId?.applyValue({ args0 -> args0 }))
.deploymentGroup(deploymentGroup?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.enableAccessLogging(enableAccessLogging?.applyValue({ args0 -> args0 }))
.index(index?.applyValue({ args0 -> args0 }))
.indexEndpoint(indexEndpoint?.applyValue({ args0 -> args0 }))
.reservedIpRanges(reservedIpRanges?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [AiIndexEndpointDeployedIndexArgs].
*/
@PulumiTagMarker
public class AiIndexEndpointDeployedIndexArgsBuilder internal constructor() {
private var automaticResources: Output? = null
private var dedicatedResources: Output? = null
private var deployedIndexAuthConfig:
Output? = null
private var deployedIndexId: Output? = null
private var deploymentGroup: Output? = null
private var displayName: Output? = null
private var enableAccessLogging: Output? = null
private var index: Output? = null
private var indexEndpoint: Output? = null
private var reservedIpRanges: Output>? = null
/**
* @param value A description of resources that the DeployedIndex uses, which to large degree are decided by Vertex AI, and optionally allows only a modest additional configuration.
* Structure is documented below.
*/
@JvmName("gaffoluvwcpjlknb")
public suspend fun automaticResources(`value`: Output) {
this.automaticResources = value
}
/**
* @param value A description of resources that are dedicated to the DeployedIndex, and that need a higher degree of manual configuration. The field minReplicaCount must be set to a value strictly greater than 0, or else validation will fail. We don't provide SLA when minReplicaCount=1. If maxReplicaCount is not set, the default value is minReplicaCount. The max allowed replica count is 1000.
* Available machine types for SMALL shard: e2-standard-2 and all machine types available for MEDIUM and LARGE shard.
* Available machine types for MEDIUM shard: e2-standard-16 and all machine types available for LARGE shard.
* Available machine types for LARGE shard: e2-highmem-16, n2d-standard-32.
* n1-standard-16 and n1-standard-32 are still available, but we recommend e2-standard-16 and e2-highmem-16 for cost efficiency.
* Structure is documented below.
*/
@JvmName("oiyciyghkjphqynn")
public suspend fun dedicatedResources(`value`: Output) {
this.dedicatedResources = value
}
/**
* @param value If set, the authentication is enabled for the private endpoint.
* Structure is documented below.
*/
@JvmName("slrdriohbqjlglfl")
public suspend fun deployedIndexAuthConfig(`value`: Output) {
this.deployedIndexAuthConfig = value
}
/**
* @param value The user specified ID of the DeployedIndex. The ID can be up to 128 characters long and must start with a letter and only contain letters, numbers, and underscores. The ID must be unique within the project it is created in.
*/
@JvmName("dwyyjrvgxtquvahb")
public suspend fun deployedIndexId(`value`: Output) {
this.deployedIndexId = value
}
/**
* @param value The deployment group can be no longer than 64 characters (eg: 'test', 'prod'). If not set, we will use the 'default' deployment group.
* Creating deployment_groups with reserved_ip_ranges is a recommended practice when the peered network has multiple peering ranges. This creates your deployments from predictable IP spaces for easier traffic administration. Also, one deployment_group (except 'default') can only be used with the same reserved_ip_ranges which means if the deployment_group has been used with reserved_ip_ranges: [a, b, c], using it with [a, b] or [d, e] is disallowed. [See the official documentation here](https://cloud.google.com/vertex-ai/docs/reference/rest/v1/projects.locations.indexEndpoints#DeployedIndex.FIELDS.deployment_group).
* Note: we only support up to 5 deployment groups (not including 'default').
*/
@JvmName("idnvhfavckbbsynn")
public suspend fun deploymentGroup(`value`: Output) {
this.deploymentGroup = value
}
/**
* @param value The display name of the Index. The name can be up to 128 characters long and can consist of any UTF-8 characters.
*/
@JvmName("rujibgvjjewjvhcj")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value If true, private endpoint's access logs are sent to Cloud Logging.
*/
@JvmName("yxslxugibtrwekrf")
public suspend fun enableAccessLogging(`value`: Output) {
this.enableAccessLogging = value
}
/**
* @param value The name of the Index this is the deployment of.
*/
@JvmName("rgbbkyljpfquaqcm")
public suspend fun index(`value`: Output) {
this.index = value
}
/**
* @param value Identifies the index endpoint. Must be in the format
* 'projects/{{project}}/locations/{{region}}/indexEndpoints/{{indexEndpoint}}'
* - - -
*/
@JvmName("yspecrwabdtfxbae")
public suspend fun indexEndpoint(`value`: Output) {
this.indexEndpoint = value
}
/**
* @param value A list of reserved ip ranges under the VPC network that can be used for this DeployedIndex.
* If set, we will deploy the index within the provided ip ranges. Otherwise, the index might be deployed to any ip ranges under the provided VPC network.
* The value should be the name of the address (https://cloud.google.com/compute/docs/reference/rest/v1/addresses) Example: ['vertex-ai-ip-range'].
* For more information about subnets and network IP ranges, please see https://cloud.google.com/vpc/docs/subnets#manually_created_subnet_ip_ranges.
*/
@JvmName("nydwivwkmeslrcuu")
public suspend fun reservedIpRanges(`value`: Output>) {
this.reservedIpRanges = value
}
@JvmName("rvynckexqfugmenv")
public suspend fun reservedIpRanges(vararg values: Output) {
this.reservedIpRanges = Output.all(values.asList())
}
/**
* @param values A list of reserved ip ranges under the VPC network that can be used for this DeployedIndex.
* If set, we will deploy the index within the provided ip ranges. Otherwise, the index might be deployed to any ip ranges under the provided VPC network.
* The value should be the name of the address (https://cloud.google.com/compute/docs/reference/rest/v1/addresses) Example: ['vertex-ai-ip-range'].
* For more information about subnets and network IP ranges, please see https://cloud.google.com/vpc/docs/subnets#manually_created_subnet_ip_ranges.
*/
@JvmName("ivubmkhftusrdayg")
public suspend fun reservedIpRanges(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy