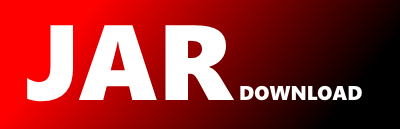
com.pulumi.gcp.vmwareengine.kotlin.inputs.PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.vmwareengine.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.vmwareengine.inputs.PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property autoscalePolicyId The identifier for this object. Format specified above.
* @property consumedMemoryThresholds Utilization thresholds pertaining to amount of consumed memory.
* Structure is documented below.
* @property cpuThresholds Utilization thresholds pertaining to CPU utilization.
* Structure is documented below.
* @property nodeTypeId The canonical identifier of the node type to add or remove.
* @property scaleOutSize Number of nodes to add to a cluster during a scale-out operation.
* Must be divisible by 2 for stretched clusters.
* @property storageThresholds Utilization thresholds pertaining to amount of consumed storage.
* Structure is documented below.
*/
public data class PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs(
public val autoscalePolicyId: Output,
public val consumedMemoryThresholds: Output? =
null,
public val cpuThresholds: Output? =
null,
public val nodeTypeId: Output,
public val scaleOutSize: Output,
public val storageThresholds: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.vmwareengine.inputs.PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs =
com.pulumi.gcp.vmwareengine.inputs.PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs.builder()
.autoscalePolicyId(autoscalePolicyId.applyValue({ args0 -> args0 }))
.consumedMemoryThresholds(
consumedMemoryThresholds?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.cpuThresholds(cpuThresholds?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.nodeTypeId(nodeTypeId.applyValue({ args0 -> args0 }))
.scaleOutSize(scaleOutSize.applyValue({ args0 -> args0 }))
.storageThresholds(
storageThresholds?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs].
*/
@PulumiTagMarker
public class PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgsBuilder internal constructor() {
private var autoscalePolicyId: Output? = null
private var consumedMemoryThresholds:
Output? =
null
private var cpuThresholds:
Output? =
null
private var nodeTypeId: Output? = null
private var scaleOutSize: Output? = null
private var storageThresholds:
Output? =
null
/**
* @param value The identifier for this object. Format specified above.
*/
@JvmName("caucslqeohujnike")
public suspend fun autoscalePolicyId(`value`: Output) {
this.autoscalePolicyId = value
}
/**
* @param value Utilization thresholds pertaining to amount of consumed memory.
* Structure is documented below.
*/
@JvmName("aabwqnouatkmvetn")
public suspend fun consumedMemoryThresholds(`value`: Output) {
this.consumedMemoryThresholds = value
}
/**
* @param value Utilization thresholds pertaining to CPU utilization.
* Structure is documented below.
*/
@JvmName("nvbivwkwdtipnirk")
public suspend fun cpuThresholds(`value`: Output) {
this.cpuThresholds = value
}
/**
* @param value The canonical identifier of the node type to add or remove.
*/
@JvmName("wapgtsdinvctyrte")
public suspend fun nodeTypeId(`value`: Output) {
this.nodeTypeId = value
}
/**
* @param value Number of nodes to add to a cluster during a scale-out operation.
* Must be divisible by 2 for stretched clusters.
*/
@JvmName("gsngiufexegnrbrj")
public suspend fun scaleOutSize(`value`: Output) {
this.scaleOutSize = value
}
/**
* @param value Utilization thresholds pertaining to amount of consumed storage.
* Structure is documented below.
*/
@JvmName("nwpgugrqvnmiatdw")
public suspend fun storageThresholds(`value`: Output) {
this.storageThresholds = value
}
/**
* @param value The identifier for this object. Format specified above.
*/
@JvmName("vsdtsyqgogpgnkww")
public suspend fun autoscalePolicyId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.autoscalePolicyId = mapped
}
/**
* @param value Utilization thresholds pertaining to amount of consumed memory.
* Structure is documented below.
*/
@JvmName("dawacwqcrwfensct")
public suspend fun consumedMemoryThresholds(`value`: PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyConsumedMemoryThresholdsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consumedMemoryThresholds = mapped
}
/**
* @param argument Utilization thresholds pertaining to amount of consumed memory.
* Structure is documented below.
*/
@JvmName("rnqdhviacceefwkh")
public suspend fun consumedMemoryThresholds(argument: suspend PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyConsumedMemoryThresholdsArgsBuilder.() -> Unit) {
val toBeMapped =
PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyConsumedMemoryThresholdsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.consumedMemoryThresholds = mapped
}
/**
* @param value Utilization thresholds pertaining to CPU utilization.
* Structure is documented below.
*/
@JvmName("wpfuskvalnjumhcr")
public suspend fun cpuThresholds(`value`: PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyCpuThresholdsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cpuThresholds = mapped
}
/**
* @param argument Utilization thresholds pertaining to CPU utilization.
* Structure is documented below.
*/
@JvmName("odkwujpweoqjgsvk")
public suspend fun cpuThresholds(argument: suspend PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyCpuThresholdsArgsBuilder.() -> Unit) {
val toBeMapped =
PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyCpuThresholdsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cpuThresholds = mapped
}
/**
* @param value The canonical identifier of the node type to add or remove.
*/
@JvmName("obimlxgthdhimmdo")
public suspend fun nodeTypeId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.nodeTypeId = mapped
}
/**
* @param value Number of nodes to add to a cluster during a scale-out operation.
* Must be divisible by 2 for stretched clusters.
*/
@JvmName("dlojadpdfloslsim")
public suspend fun scaleOutSize(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.scaleOutSize = mapped
}
/**
* @param value Utilization thresholds pertaining to amount of consumed storage.
* Structure is documented below.
*/
@JvmName("wxmftiwnktxkupmf")
public suspend fun storageThresholds(`value`: PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyStorageThresholdsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageThresholds = mapped
}
/**
* @param argument Utilization thresholds pertaining to amount of consumed storage.
* Structure is documented below.
*/
@JvmName("wuisxklyfiaeshcc")
public suspend fun storageThresholds(argument: suspend PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyStorageThresholdsArgsBuilder.() -> Unit) {
val toBeMapped =
PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyStorageThresholdsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.storageThresholds = mapped
}
internal fun build(): PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs =
PrivateCloudManagementClusterAutoscalingSettingsAutoscalingPolicyArgs(
autoscalePolicyId = autoscalePolicyId ?: throw PulumiNullFieldException("autoscalePolicyId"),
consumedMemoryThresholds = consumedMemoryThresholds,
cpuThresholds = cpuThresholds,
nodeTypeId = nodeTypeId ?: throw PulumiNullFieldException("nodeTypeId"),
scaleOutSize = scaleOutSize ?: throw PulumiNullFieldException("scaleOutSize"),
storageThresholds = storageThresholds,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy