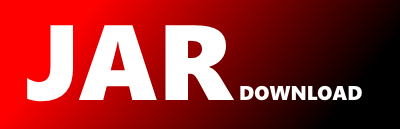
com.pulumi.gitlab.kotlin.PagesDomain.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [PagesDomain].
*/
@PulumiTagMarker
public class PagesDomainResourceBuilder internal constructor() {
public var name: String? = null
public var args: PagesDomainArgs = PagesDomainArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PagesDomainArgsBuilder.() -> Unit) {
val builder = PagesDomainArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): PagesDomain {
val builtJavaResource = com.pulumi.gitlab.PagesDomain(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return PagesDomain(builtJavaResource)
}
}
/**
* The `gitlab.PagesDomain` resource allows connecting custom domains and TLS certificates in GitLab Pages.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/pages_domains.html)
* ## Import
* GitLab pages domain can be imported using an id made up of `projectId:domain` _without_ the http protocol, e.g.
* ```sh
* $ pulumi import gitlab:index/pagesDomain:PagesDomain this 123:example.com
* ```
*/
public class PagesDomain internal constructor(
override val javaResource: com.pulumi.gitlab.PagesDomain,
) : KotlinCustomResource(javaResource, PagesDomainMapper) {
/**
* Enables [automatic generation](https://docs.gitlab.com/ee/user/project/pages/custom_domains_ssl_tls_certification/lets_encrypt_integration.html) of SSL certificates issued by Let’s Encrypt for custom domains. When this is set to "true", certificate can't be provided.
*/
public val autoSslEnabled: Output
get() = javaResource.autoSslEnabled().applyValue({ args0 -> args0 })
/**
* The certificate in PEM format with intermediates following in most specific to least specific order.
*/
public val certificate: Output
get() = javaResource.certificate().applyValue({ args0 -> args0 })
/**
* The custom domain indicated by the user.
*/
public val domain: Output
get() = javaResource.domain().applyValue({ args0 -> args0 })
/**
* Whether the certificate is expired.
*/
public val expired: Output
get() = javaResource.expired().applyValue({ args0 -> args0 })
/**
* The certificate key in PEM format.
*/
public val key: Output?
get() = javaResource.key().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The ID or [URL-encoded path of the project](https://docs.gitlab.com/ee/api/index.html#namespaced-path-encoding) owned by the authenticated user.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The URL for the given domain.
*/
public val url: Output
get() = javaResource.url().applyValue({ args0 -> args0 })
/**
* The verification code for the domain.
*/
public val verificationCode: Output
get() = javaResource.verificationCode().applyValue({ args0 -> args0 })
/**
* The certificate data.
*/
public val verified: Output
get() = javaResource.verified().applyValue({ args0 -> args0 })
}
public object PagesDomainMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.PagesDomain::class == javaResource::class
override fun map(javaResource: Resource): PagesDomain = PagesDomain(
javaResource as
com.pulumi.gitlab.PagesDomain,
)
}
/**
* @see [PagesDomain].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [PagesDomain].
*/
public suspend fun pagesDomain(name: String, block: suspend PagesDomainResourceBuilder.() -> Unit): PagesDomain {
val builder = PagesDomainResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [PagesDomain].
* @param name The _unique_ name of the resulting resource.
*/
public fun pagesDomain(name: String): PagesDomain {
val builder = PagesDomainResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy