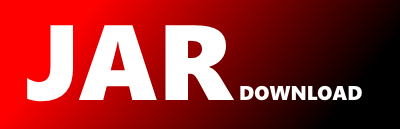
com.pulumi.gitlab.kotlin.Project.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.gitlab.kotlin.outputs.ProjectContainerExpirationPolicy
import com.pulumi.gitlab.kotlin.outputs.ProjectPushRules
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gitlab.kotlin.outputs.ProjectContainerExpirationPolicy.Companion.toKotlin as projectContainerExpirationPolicyToKotlin
import com.pulumi.gitlab.kotlin.outputs.ProjectPushRules.Companion.toKotlin as projectPushRulesToKotlin
/**
* Builder for [Project].
*/
@PulumiTagMarker
public class ProjectResourceBuilder internal constructor() {
public var name: String? = null
public var args: ProjectArgs = ProjectArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ProjectArgsBuilder.() -> Unit) {
val builder = ProjectArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Project {
val builtJavaResource = com.pulumi.gitlab.Project(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Project(builtJavaResource)
}
}
/**
* ## Example Usage
* ## Import
* ```sh
* $ pulumi import gitlab:index/project:Project You can import a project state using ` `. The
* ```
* `id` can be whatever the [get single project api][get_single_project] takes for
* its `:id` value, so for example:
* ```sh
* $ pulumi import gitlab:index/project:Project example richardc/example
* ```
* NOTE: the `import_url_username` and `import_url_password` cannot be imported.
*/
public class Project internal constructor(
override val javaResource: com.pulumi.gitlab.Project,
) : KotlinCustomResource(javaResource, ProjectMapper) {
/**
* Set to true if you want to treat skipped pipelines as if they finished with success.
*/
public val allowMergeOnSkippedPipeline: Output
get() = javaResource.allowMergeOnSkippedPipeline().applyValue({ args0 -> args0 })
/**
* Set the analytics access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val analyticsAccessLevel: Output
get() = javaResource.analyticsAccessLevel().applyValue({ args0 -> args0 })
/**
* Number of merge request approvals required for merging. Default is 0.
* This field **does not** work well in combination with the `gitlab.ProjectApprovalRule` resource
* and is most likely gonna be deprecated in a future GitLab version (see [this upstream epic](https://gitlab.com/groups/gitlab-org/-/epics/7572)).
* In the meantime we recommend against using this attribute and use `gitlab.ProjectApprovalRule` instead.
*/
public val approvalsBeforeMerge: Output?
get() = javaResource.approvalsBeforeMerge().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set to `true` to archive the project instead of deleting on destroy. If set to `true` it will entire omit the `DELETE` operation.
*/
public val archiveOnDestroy: Output?
get() = javaResource.archiveOnDestroy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether the project is in read-only mode (archived). Repositories can be archived/unarchived by toggling this parameter.
*/
public val archived: Output?
get() = javaResource.archived().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Auto-cancel pending pipelines. This isn’t a boolean, but enabled/disabled.
*/
public val autoCancelPendingPipelines: Output
get() = javaResource.autoCancelPendingPipelines().applyValue({ args0 -> args0 })
/**
* Auto Deploy strategy. Valid values are `continuous`, `manual`, `timed_incremental`.
*/
public val autoDevopsDeployStrategy: Output
get() = javaResource.autoDevopsDeployStrategy().applyValue({ args0 -> args0 })
/**
* Enable Auto DevOps for this project.
*/
public val autoDevopsEnabled: Output
get() = javaResource.autoDevopsEnabled().applyValue({ args0 -> args0 })
/**
* Set whether auto-closing referenced issues on default branch.
*/
public val autocloseReferencedIssues: Output
get() = javaResource.autocloseReferencedIssues().applyValue({ args0 -> args0 })
/**
* A local path to the avatar image to upload. **Note**: not available for imported resources.
*/
public val avatar: Output?
get() = javaResource.avatar().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The hash of the avatar image. Use `filesha256("path/to/avatar.png")` whenever possible. **Note**: this is used to trigger an update of the avatar. If it's not given, but an avatar is given, the avatar will be updated each time.
*/
public val avatarHash: Output
get() = javaResource.avatarHash().applyValue({ args0 -> args0 })
/**
* The URL of the avatar image.
*/
public val avatarUrl: Output
get() = javaResource.avatarUrl().applyValue({ args0 -> args0 })
/**
* Test coverage parsing for the project. This is deprecated feature in GitLab 15.0.
*/
@Deprecated(
message = """
build_coverage_regex is removed in GitLab 15.0.
""",
)
public val buildCoverageRegex: Output?
get() = javaResource.buildCoverageRegex().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Git strategy. Defaults to fetch. Valid values are `clone`, `fetch`.
*/
public val buildGitStrategy: Output
get() = javaResource.buildGitStrategy().applyValue({ args0 -> args0 })
/**
* The maximum amount of time, in seconds, that a job can run.
*/
public val buildTimeout: Output
get() = javaResource.buildTimeout().applyValue({ args0 -> args0 })
/**
* Set the builds access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val buildsAccessLevel: Output
get() = javaResource.buildsAccessLevel().applyValue({ args0 -> args0 })
/**
* Custom Path to CI config file.
*/
public val ciConfigPath: Output?
get() = javaResource.ciConfigPath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Default number of revisions for shallow cloning.
*/
public val ciDefaultGitDepth: Output
get() = javaResource.ciDefaultGitDepth().applyValue({ args0 -> args0 })
/**
* When a new deployment job starts, skip older deployment jobs that are still pending.
*/
public val ciForwardDeploymentEnabled: Output
get() = javaResource.ciForwardDeploymentEnabled().applyValue({ args0 -> args0 })
/**
* The role required to cancel a pipeline or job. Introduced in GitLab 16.8. Premium and Ultimate only. Valid values are `developer`, `maintainer`, `no one`
*/
public val ciRestrictPipelineCancellationRole: Output
get() = javaResource.ciRestrictPipelineCancellationRole().applyValue({ args0 -> args0 })
/**
* Use separate caches for protected branches.
*/
public val ciSeparatedCaches: Output
get() = javaResource.ciSeparatedCaches().applyValue({ args0 -> args0 })
/**
* Set the image cleanup policy for this project. **Note**: this field is sometimes named `container_expiration_policy_attributes` in the GitLab Upstream API.
*/
public val containerExpirationPolicy: Output
get() = javaResource.containerExpirationPolicy().applyValue({ args0 ->
args0.let({ args0 ->
projectContainerExpirationPolicyToKotlin(args0)
})
})
/**
* Set visibility of container registry, for this project. Valid values are `disabled`, `private`, `enabled`.
*/
public val containerRegistryAccessLevel: Output
get() = javaResource.containerRegistryAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable container registry for the project.
*/
@Deprecated(
message = """
Use `container_registry_access_level` instead.
""",
)
public val containerRegistryEnabled: Output
get() = javaResource.containerRegistryEnabled().applyValue({ args0 -> args0 })
/**
* The default branch for the project.
*/
public val defaultBranch: Output
get() = javaResource.defaultBranch().applyValue({ args0 -> args0 })
/**
* A description of the project.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Enable email notifications.
*/
public val emailsEnabled: Output
get() = javaResource.emailsEnabled().applyValue({ args0 -> args0 })
/**
* Whether the project is empty.
*/
public val emptyRepo: Output
get() = javaResource.emptyRepo().applyValue({ args0 -> args0 })
/**
* Set the environments access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val environmentsAccessLevel: Output
get() = javaResource.environmentsAccessLevel().applyValue({ args0 -> args0 })
/**
* The classification label for the project.
*/
public val externalAuthorizationClassificationLabel: Output?
get() = javaResource.externalAuthorizationClassificationLabel().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Set the feature flags access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val featureFlagsAccessLevel: Output
get() = javaResource.featureFlagsAccessLevel().applyValue({ args0 -> args0 })
/**
* The id of the project to fork. During create the project is forked and during an update the fork relation is changed.
*/
public val forkedFromProjectId: Output?
get() = javaResource.forkedFromProjectId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set the forking access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val forkingAccessLevel: Output
get() = javaResource.forkingAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable group runners for this project.
*/
public val groupRunnersEnabled: Output
get() = javaResource.groupRunnersEnabled().applyValue({ args0 -> args0 })
/**
* For group-level custom templates, specifies ID of group from which all the custom project templates are sourced. Leave empty for instance-level templates. Requires use*custom*template to be true (enterprise edition).
*/
public val groupWithProjectTemplatesId: Output?
get() = javaResource.groupWithProjectTemplatesId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* URL that can be provided to `git clone` to clone the
*/
public val httpUrlToRepo: Output
get() = javaResource.httpUrlToRepo().applyValue({ args0 -> args0 })
/**
* Git URL to a repository to be imported. Together with `mirror = true` it will setup a Pull Mirror. This can also be used together with `forked_from_project_id` to setup a Pull Mirror for a fork. The fork takes precedence over the import. Make sure to provide the credentials in `import_url_username` and `import_url_password`. GitLab never returns the credentials, thus the provider cannot detect configuration drift in the credentials. They can also not be imported using `pulumi import`. See the examples section for how to properly use it.
*/
public val importUrl: Output?
get() = javaResource.importUrl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The password for the `import_url`. The value of this field is used to construct a valid `import_url` and is only related to the provider. This field cannot be imported using `pulumi import`. See the examples section for how to properly use it.
*/
public val importUrlPassword: Output?
get() = javaResource.importUrlPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The username for the `import_url`. The value of this field is used to construct a valid `import_url` and is only related to the provider. This field cannot be imported using `pulumi import`. See the examples section for how to properly use it.
*/
public val importUrlUsername: Output?
get() = javaResource.importUrlUsername().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set the infrastructure access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val infrastructureAccessLevel: Output
get() = javaResource.infrastructureAccessLevel().applyValue({ args0 -> args0 })
/**
* Create main branch with first commit containing a README.md file.
*/
public val initializeWithReadme: Output?
get() = javaResource.initializeWithReadme().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set the issues access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val issuesAccessLevel: Output
get() = javaResource.issuesAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable issue tracking for the project.
*/
public val issuesEnabled: Output
get() = javaResource.issuesEnabled().applyValue({ args0 -> args0 })
/**
* Sets the template for new issues in the project.
*/
public val issuesTemplate: Output?
get() = javaResource.issuesTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Disable or enable the ability to keep the latest artifact for this project.
*/
public val keepLatestArtifact: Output
get() = javaResource.keepLatestArtifact().applyValue({ args0 -> args0 })
/**
* Enable LFS for the project.
*/
public val lfsEnabled: Output
get() = javaResource.lfsEnabled().applyValue({ args0 -> args0 })
/**
* Template used to create merge commit message in merge requests. (Introduced in GitLab 14.5.)
*/
public val mergeCommitTemplate: Output?
get() = javaResource.mergeCommitTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set the merge method. Valid values are `merge`, `rebase_merge`, `ff`.
*/
public val mergeMethod: Output
get() = javaResource.mergeMethod().applyValue({ args0 -> args0 })
/**
* Enable or disable merge pipelines.
*/
public val mergePipelinesEnabled: Output
get() = javaResource.mergePipelinesEnabled().applyValue({ args0 -> args0 })
/**
* Set the merge requests access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val mergeRequestsAccessLevel: Output
get() = javaResource.mergeRequestsAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable merge requests for the project.
*/
public val mergeRequestsEnabled: Output
get() = javaResource.mergeRequestsEnabled().applyValue({ args0 -> args0 })
/**
* Sets the template for new merge requests in the project.
*/
public val mergeRequestsTemplate: Output?
get() = javaResource.mergeRequestsTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Enable or disable merge trains. Requires `merge_pipelines_enabled` to be set to `true` to take effect.
*/
public val mergeTrainsEnabled: Output
get() = javaResource.mergeTrainsEnabled().applyValue({ args0 -> args0 })
/**
* Enable project pull mirror.
*/
public val mirror: Output?
get() = javaResource.mirror().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Enable overwrite diverged branches for a mirrored project.
*/
public val mirrorOverwritesDivergedBranches: Output
get() = javaResource.mirrorOverwritesDivergedBranches().applyValue({ args0 -> args0 })
/**
* Enable trigger builds on pushes for a mirrored project.
*/
public val mirrorTriggerBuilds: Output
get() = javaResource.mirrorTriggerBuilds().applyValue({ args0 -> args0 })
/**
* Set the monitor access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val monitorAccessLevel: Output
get() = javaResource.monitorAccessLevel().applyValue({ args0 -> args0 })
/**
* For forked projects, target merge requests to this project. If false, the target will be the upstream project.
*/
public val mrDefaultTargetSelf: Output?
get() = javaResource.mrDefaultTargetSelf().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the project.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace (group or user) of the project. Defaults to your user.
*/
public val namespaceId: Output
get() = javaResource.namespaceId().applyValue({ args0 -> args0 })
/**
* Set to true if you want allow merges only if all discussions are resolved.
*/
public val onlyAllowMergeIfAllDiscussionsAreResolved: Output
get() = javaResource.onlyAllowMergeIfAllDiscussionsAreResolved().applyValue({ args0 -> args0 })
/**
* Set to true if you want allow merges only if a pipeline succeeds.
*/
public val onlyAllowMergeIfPipelineSucceeds: Output
get() = javaResource.onlyAllowMergeIfPipelineSucceeds().applyValue({ args0 -> args0 })
/**
* Enable only mirror protected branches for a mirrored project.
*/
public val onlyMirrorProtectedBranches: Output
get() = javaResource.onlyMirrorProtectedBranches().applyValue({ args0 -> args0 })
/**
* Enable packages repository for the project.
*/
public val packagesEnabled: Output
get() = javaResource.packagesEnabled().applyValue({ args0 -> args0 })
/**
* Enable pages access control. Valid values are `public`, `private`, `enabled`, `disabled`.
*/
public val pagesAccessLevel: Output
get() = javaResource.pagesAccessLevel().applyValue({ args0 -> args0 })
/**
* The path of the repository.
*/
public val path: Output?
get() = javaResource.path().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The path of the repository with namespace.
*/
public val pathWithNamespace: Output
get() = javaResource.pathWithNamespace().applyValue({ args0 -> args0 })
/**
* Enable pipelines for the project. The `pipelines_enabled` field is being sent as `jobs_enabled` in the GitLab API calls.
*/
@Deprecated(
message = """
Deprecated in favor of `builds_access_level`
""",
)
public val pipelinesEnabled: Output
get() = javaResource.pipelinesEnabled().applyValue({ args0 -> args0 })
/**
* Show link to create/view merge request when pushing from the command line
*/
public val printingMergeRequestLinkEnabled: Output
get() = javaResource.printingMergeRequestLinkEnabled().applyValue({ args0 -> args0 })
/**
* If true, jobs can be viewed by non-project members.
*/
@Deprecated(
message = """
The `public_builds` attribute has been deprecated in favor of `public_jobs` and will be removed in
the next major version of the provider.
""",
)
public val publicBuilds: Output
get() = javaResource.publicBuilds().applyValue({ args0 -> args0 })
/**
* If true, jobs can be viewed by non-project members.
*/
public val publicJobs: Output
get() = javaResource.publicJobs().applyValue({ args0 -> args0 })
/**
* Push rules for the project.
*/
public val pushRules: Output
get() = javaResource.pushRules().applyValue({ args0 ->
args0.let({ args0 ->
projectPushRulesToKotlin(args0)
})
})
/**
* Set the releases access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val releasesAccessLevel: Output
get() = javaResource.releasesAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable `Delete source branch` option by default for all new merge requests.
*/
public val removeSourceBranchAfterMerge: Output
get() = javaResource.removeSourceBranchAfterMerge().applyValue({ args0 -> args0 })
/**
* Set the repository access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val repositoryAccessLevel: Output
get() = javaResource.repositoryAccessLevel().applyValue({ args0 -> args0 })
/**
* Which storage shard the repository is on. (administrator only)
*/
public val repositoryStorage: Output
get() = javaResource.repositoryStorage().applyValue({ args0 -> args0 })
/**
* Allow users to request member access.
*/
public val requestAccessEnabled: Output
get() = javaResource.requestAccessEnabled().applyValue({ args0 -> args0 })
/**
* Set the requirements access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val requirementsAccessLevel: Output
get() = javaResource.requirementsAccessLevel().applyValue({ args0 -> args0 })
/**
* Automatically resolve merge request diffs discussions on lines changed with a push.
*/
public val resolveOutdatedDiffDiscussions: Output?
get() = javaResource.resolveOutdatedDiffDiscussions().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Allow only users with the Maintainer role to pass user-defined variables when triggering a pipeline.
*/
public val restrictUserDefinedVariables: Output?
get() = javaResource.restrictUserDefinedVariables().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Registration token to use during runner setup.
*/
public val runnersToken: Output
get() = javaResource.runnersToken().applyValue({ args0 -> args0 })
/**
* Set the security and compliance access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val securityAndComplianceAccessLevel: Output
get() = javaResource.securityAndComplianceAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable shared runners for this project.
*/
public val sharedRunnersEnabled: Output
get() = javaResource.sharedRunnersEnabled().applyValue({ args0 -> args0 })
/**
* If `true`, the default behavior to wait for the default branch protection to be created is skipped.
* This is necessary if the current user is not an admin and the default branch protection is disabled on an instance-level.
* There is currently no known way to determine if the default branch protection is disabled on an instance-level for non-admin users.
* This attribute is only used during resource creation, thus changes are suppressed and the attribute cannot be imported.
*/
public val skipWaitForDefaultBranchProtection: Output?
get() = javaResource.skipWaitForDefaultBranchProtection().applyValue({ args0 ->
args0.map({ args0 -> args0 }).orElse(null)
})
/**
* Set the snippets access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val snippetsAccessLevel: Output
get() = javaResource.snippetsAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable snippets for the project.
*/
public val snippetsEnabled: Output
get() = javaResource.snippetsEnabled().applyValue({ args0 -> args0 })
/**
* Template used to create squash commit message in merge requests. (Introduced in GitLab 14.6.)
*/
public val squashCommitTemplate: Output?
get() = javaResource.squashCommitTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Squash commits when merge request. Valid values are `never`, `always`, `default_on`, or `default_off`. The default value is `default_off`. [GitLab >= 14.1]
*/
public val squashOption: Output
get() = javaResource.squashOption().applyValue({ args0 -> args0 })
/**
* URL that can be provided to `git clone` to clone the
*/
public val sshUrlToRepo: Output
get() = javaResource.sshUrlToRepo().applyValue({ args0 -> args0 })
/**
* The commit message used to apply merge request suggestions.
*/
public val suggestionCommitMessage: Output?
get() = javaResource.suggestionCommitMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The list of tags for a project; put array of tags, that should be finally assigned to a project. Use topics instead.
*/
public val tags: Output>
get() = javaResource.tags().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* When used without use*custom*template, name of a built-in project template. When used with use*custom*template, name of a custom project template. This option is mutually exclusive with `template_project_id`.
*/
public val templateName: Output?
get() = javaResource.templateName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* When used with use*custom*template, project ID of a custom project template. This is preferable to using template*name since template*name may be ambiguous (enterprise edition). This option is mutually exclusive with `template_name`. See `gitlab.GroupProjectFileTemplate` to set a project as a template project. If a project has not been set as a template, using it here will result in an error.
*/
public val templateProjectId: Output?
get() = javaResource.templateProjectId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The list of topics for the project.
*/
public val topics: Output>
get() = javaResource.topics().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Use either custom instance or group (with group*with*project*templates*id) project template (enterprise edition).
* > When using a custom template, [Group Tokens won't work](https://docs.gitlab.com/15.7/ee/user/project/settings/import_export_troubleshooting.html#import-using-the-rest-api-fails-when-using-a-group-access-token). You must use a real user's Personal Access Token.
*/
public val useCustomTemplate: Output?
get() = javaResource.useCustomTemplate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Set to `public` to create a public project. Valid values are `private`, `internal`, `public`.
*/
public val visibilityLevel: Output
get() = javaResource.visibilityLevel().applyValue({ args0 -> args0 })
/**
* URL that can be used to find the project in a browser.
*/
public val webUrl: Output
get() = javaResource.webUrl().applyValue({ args0 -> args0 })
/**
* Set the wiki access level. Valid values are `disabled`, `private`, `enabled`.
*/
public val wikiAccessLevel: Output
get() = javaResource.wikiAccessLevel().applyValue({ args0 -> args0 })
/**
* Enable wiki for the project.
*/
public val wikiEnabled: Output
get() = javaResource.wikiEnabled().applyValue({ args0 -> args0 })
}
public object ProjectMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.Project::class == javaResource::class
override fun map(javaResource: Resource): Project = Project(
javaResource as
com.pulumi.gitlab.Project,
)
}
/**
* @see [Project].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Project].
*/
public suspend fun project(name: String, block: suspend ProjectResourceBuilder.() -> Unit): Project {
val builder = ProjectResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Project].
* @param name The _unique_ name of the resulting resource.
*/
public fun project(name: String): Project {
val builder = ProjectResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy