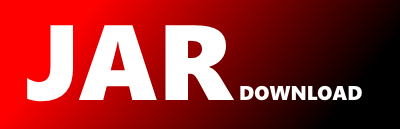
com.pulumi.gitlab.kotlin.ProviderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.ProviderArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The provider type for the gitlab package. By default, resources use package-wide configuration
* settings, however an explicit `Provider` instance may be created and passed during resource
* construction to achieve fine-grained programmatic control over provider settings. See the
* [documentation](https://www.pulumi.com/docs/reference/programming-model/#providers) for more information.
* @property baseUrl This is the target GitLab base API endpoint. Providing a value is a requirement when working with GitLab CE or GitLab
* Enterprise e.g. `https://my.gitlab.server/api/v4/`. It is optional to provide this value and it can also be sourced from
* the `GITLAB_BASE_URL` environment variable. The value must end with a slash.
* @property cacertFile This is a file containing the ca cert to verify the gitlab instance. This is available for use when working with GitLab
* CE or Gitlab Enterprise with a locally-issued or self-signed certificate chain.
* @property clientCert File path to client certificate when GitLab instance is behind company proxy. File must contain PEM encoded data.
* @property clientKey File path to client key when GitLab instance is behind company proxy. File must contain PEM encoded data. Required when
* `client_cert` is set.
* @property earlyAuthCheck
* @property insecure When set to true this disables SSL verification of the connection to the GitLab instance.
* @property token The OAuth2 Token, Project, Group, Personal Access Token or CI Job Token used to connect to GitLab. The OAuth method is
* used in this provider for authentication (using Bearer authorization token). See
* https://docs.gitlab.com/ee/api/#authentication for details. It may be sourced from the `GITLAB_TOKEN` environment
* variable.
*/
public data class ProviderArgs(
public val baseUrl: Output? = null,
public val cacertFile: Output? = null,
public val clientCert: Output? = null,
public val clientKey: Output? = null,
public val earlyAuthCheck: Output? = null,
public val insecure: Output? = null,
public val token: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.ProviderArgs = com.pulumi.gitlab.ProviderArgs.builder()
.baseUrl(baseUrl?.applyValue({ args0 -> args0 }))
.cacertFile(cacertFile?.applyValue({ args0 -> args0 }))
.clientCert(clientCert?.applyValue({ args0 -> args0 }))
.clientKey(clientKey?.applyValue({ args0 -> args0 }))
.earlyAuthCheck(earlyAuthCheck?.applyValue({ args0 -> args0 }))
.insecure(insecure?.applyValue({ args0 -> args0 }))
.token(token?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProviderArgs].
*/
@PulumiTagMarker
public class ProviderArgsBuilder internal constructor() {
private var baseUrl: Output? = null
private var cacertFile: Output? = null
private var clientCert: Output? = null
private var clientKey: Output? = null
private var earlyAuthCheck: Output? = null
private var insecure: Output? = null
private var token: Output? = null
/**
* @param value This is the target GitLab base API endpoint. Providing a value is a requirement when working with GitLab CE or GitLab
* Enterprise e.g. `https://my.gitlab.server/api/v4/`. It is optional to provide this value and it can also be sourced from
* the `GITLAB_BASE_URL` environment variable. The value must end with a slash.
*/
@JvmName("cfkheyruqaennbun")
public suspend fun baseUrl(`value`: Output) {
this.baseUrl = value
}
/**
* @param value This is a file containing the ca cert to verify the gitlab instance. This is available for use when working with GitLab
* CE or Gitlab Enterprise with a locally-issued or self-signed certificate chain.
*/
@JvmName("cjqoxdtbeijlimww")
public suspend fun cacertFile(`value`: Output) {
this.cacertFile = value
}
/**
* @param value File path to client certificate when GitLab instance is behind company proxy. File must contain PEM encoded data.
*/
@JvmName("oubxgcbmcwdgwcxq")
public suspend fun clientCert(`value`: Output) {
this.clientCert = value
}
/**
* @param value File path to client key when GitLab instance is behind company proxy. File must contain PEM encoded data. Required when
* `client_cert` is set.
*/
@JvmName("ymabndkwdlejvgja")
public suspend fun clientKey(`value`: Output) {
this.clientKey = value
}
/**
* @param value
*/
@JvmName("gjucexhkgyfhvelv")
public suspend fun earlyAuthCheck(`value`: Output) {
this.earlyAuthCheck = value
}
/**
* @param value When set to true this disables SSL verification of the connection to the GitLab instance.
*/
@JvmName("ffrqttqrfbytcbbm")
public suspend fun insecure(`value`: Output) {
this.insecure = value
}
/**
* @param value The OAuth2 Token, Project, Group, Personal Access Token or CI Job Token used to connect to GitLab. The OAuth method is
* used in this provider for authentication (using Bearer authorization token). See
* https://docs.gitlab.com/ee/api/#authentication for details. It may be sourced from the `GITLAB_TOKEN` environment
* variable.
*/
@JvmName("exupyfdqdoepjfou")
public suspend fun token(`value`: Output) {
this.token = value
}
/**
* @param value This is the target GitLab base API endpoint. Providing a value is a requirement when working with GitLab CE or GitLab
* Enterprise e.g. `https://my.gitlab.server/api/v4/`. It is optional to provide this value and it can also be sourced from
* the `GITLAB_BASE_URL` environment variable. The value must end with a slash.
*/
@JvmName("icrahenparkejpgb")
public suspend fun baseUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseUrl = mapped
}
/**
* @param value This is a file containing the ca cert to verify the gitlab instance. This is available for use when working with GitLab
* CE or Gitlab Enterprise with a locally-issued or self-signed certificate chain.
*/
@JvmName("nkeyjmodmlbbcsjk")
public suspend fun cacertFile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cacertFile = mapped
}
/**
* @param value File path to client certificate when GitLab instance is behind company proxy. File must contain PEM encoded data.
*/
@JvmName("exbstuljmyuxunpa")
public suspend fun clientCert(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientCert = mapped
}
/**
* @param value File path to client key when GitLab instance is behind company proxy. File must contain PEM encoded data. Required when
* `client_cert` is set.
*/
@JvmName("lctvvbgwltieelnf")
public suspend fun clientKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientKey = mapped
}
/**
* @param value
*/
@JvmName("fxgqeiteikxxrvec")
public suspend fun earlyAuthCheck(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.earlyAuthCheck = mapped
}
/**
* @param value When set to true this disables SSL verification of the connection to the GitLab instance.
*/
@JvmName("fmpgdxiqsisrpnjn")
public suspend fun insecure(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.insecure = mapped
}
/**
* @param value The OAuth2 Token, Project, Group, Personal Access Token or CI Job Token used to connect to GitLab. The OAuth method is
* used in this provider for authentication (using Bearer authorization token). See
* https://docs.gitlab.com/ee/api/#authentication for details. It may be sourced from the `GITLAB_TOKEN` environment
* variable.
*/
@JvmName("mypwnuuxmekvhnly")
public suspend fun token(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.token = mapped
}
internal fun build(): ProviderArgs = ProviderArgs(
baseUrl = baseUrl,
cacertFile = cacertFile,
clientCert = clientCert,
clientKey = clientKey,
earlyAuthCheck = earlyAuthCheck,
insecure = insecure,
token = token,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy