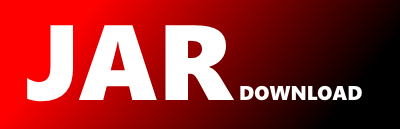
com.pulumi.gitlab.kotlin.BranchProtection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToMerge
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToPush
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToUnprotect
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToMerge.Companion.toKotlin as branchProtectionAllowedToMergeToKotlin
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToPush.Companion.toKotlin as branchProtectionAllowedToPushToKotlin
import com.pulumi.gitlab.kotlin.outputs.BranchProtectionAllowedToUnprotect.Companion.toKotlin as branchProtectionAllowedToUnprotectToKotlin
/**
* Builder for [BranchProtection].
*/
@PulumiTagMarker
public class BranchProtectionResourceBuilder internal constructor() {
public var name: String? = null
public var args: BranchProtectionArgs = BranchProtectionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BranchProtectionArgsBuilder.() -> Unit) {
val builder = BranchProtectionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BranchProtection {
val builtJavaResource = com.pulumi.gitlab.BranchProtection(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BranchProtection(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const branchProtect = new gitlab.BranchProtection("BranchProtect", {
* project: "12345",
* branch: "BranchProtected",
* pushAccessLevel: "developer",
* mergeAccessLevel: "developer",
* unprotectAccessLevel: "developer",
* allowForcePush: true,
* codeOwnerApprovalRequired: true,
* allowedToPushes: [
* {
* userId: 5,
* },
* {
* userId: 521,
* },
* ],
* allowedToMerges: [
* {
* userId: 15,
* },
* {
* userId: 37,
* },
* ],
* allowedToUnprotects: [
* {
* userId: 15,
* },
* {
* groupId: 42,
* },
* ],
* });
* // Example using dynamic block
* const main = new gitlab.BranchProtection("main", {
* allowedToPushes: [
* 50,
* 55,
* 60,
* ].map((v, k) => ({key: k, value: v})).map(entry => ({
* userId: entry.value,
* })),
* project: "12345",
* branch: "main",
* pushAccessLevel: "maintainer",
* mergeAccessLevel: "maintainer",
* unprotectAccessLevel: "maintainer",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* branch_protect = gitlab.BranchProtection("BranchProtect",
* project="12345",
* branch="BranchProtected",
* push_access_level="developer",
* merge_access_level="developer",
* unprotect_access_level="developer",
* allow_force_push=True,
* code_owner_approval_required=True,
* allowed_to_pushes=[
* {
* "user_id": 5,
* },
* {
* "user_id": 521,
* },
* ],
* allowed_to_merges=[
* {
* "user_id": 15,
* },
* {
* "user_id": 37,
* },
* ],
* allowed_to_unprotects=[
* {
* "user_id": 15,
* },
* {
* "group_id": 42,
* },
* ])
* # Example using dynamic block
* main = gitlab.BranchProtection("main",
* allowed_to_pushes=[{
* "user_id": entry["value"],
* } for entry in [{"key": k, "value": v} for k, v in [
* 50,
* 55,
* 60,
* ]]],
* project="12345",
* branch="main",
* push_access_level="maintainer",
* merge_access_level="maintainer",
* unprotect_access_level="maintainer")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var branchProtect = new GitLab.BranchProtection("BranchProtect", new()
* {
* Project = "12345",
* Branch = "BranchProtected",
* PushAccessLevel = "developer",
* MergeAccessLevel = "developer",
* UnprotectAccessLevel = "developer",
* AllowForcePush = true,
* CodeOwnerApprovalRequired = true,
* AllowedToPushes = new[]
* {
* new GitLab.Inputs.BranchProtectionAllowedToPushArgs
* {
* UserId = 5,
* },
* new GitLab.Inputs.BranchProtectionAllowedToPushArgs
* {
* UserId = 521,
* },
* },
* AllowedToMerges = new[]
* {
* new GitLab.Inputs.BranchProtectionAllowedToMergeArgs
* {
* UserId = 15,
* },
* new GitLab.Inputs.BranchProtectionAllowedToMergeArgs
* {
* UserId = 37,
* },
* },
* AllowedToUnprotects = new[]
* {
* new GitLab.Inputs.BranchProtectionAllowedToUnprotectArgs
* {
* UserId = 15,
* },
* new GitLab.Inputs.BranchProtectionAllowedToUnprotectArgs
* {
* GroupId = 42,
* },
* },
* });
* // Example using dynamic block
* var main = new GitLab.BranchProtection("main", new()
* {
* AllowedToPushes = new[]
* {
* 50,
* 55,
* 60,
* }.Select((v, k) => new { Key = k, Value = v }).Select(entry =>
* {
* return new GitLab.Inputs.BranchProtectionAllowedToPushArgs
* {
* UserId = entry.Value,
* };
* }).ToList(),
* Project = "12345",
* Branch = "main",
* PushAccessLevel = "maintainer",
* MergeAccessLevel = "maintainer",
* UnprotectAccessLevel = "maintainer",
* });
* });
* ```
*
* ## Import
* Gitlab protected branches can be imported with a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/branchProtection:BranchProtection BranchProtect "12345:main"
* ```
*/
public class BranchProtection internal constructor(
override val javaResource: com.pulumi.gitlab.BranchProtection,
) : KotlinCustomResource(javaResource, BranchProtectionMapper) {
/**
* Can be set to true to allow users with push access to force push.
*/
public val allowForcePush: Output
get() = javaResource.allowForcePush().applyValue({ args0 -> args0 })
/**
* Array of access levels and user(s)/group(s) allowed to merge to protected branch.
*/
public val allowedToMerges: Output>?
get() = javaResource.allowedToMerges().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
branchProtectionAllowedToMergeToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Array of access levels and user(s)/group(s) allowed to push to protected branch.
*/
public val allowedToPushes: Output>?
get() = javaResource.allowedToPushes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
branchProtectionAllowedToPushToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Array of access levels and user(s)/group(s) allowed to unprotect push to protected branch.
*/
public val allowedToUnprotects: Output>?
get() = javaResource.allowedToUnprotects().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
branchProtectionAllowedToUnprotectToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Name of the branch.
*/
public val branch: Output
get() = javaResource.branch().applyValue({ args0 -> args0 })
/**
* The ID of the branch protection (not the branch name).
*/
public val branchProtectionId: Output
get() = javaResource.branchProtectionId().applyValue({ args0 -> args0 })
/**
* Can be set to true to require code owner approval before merging. Only available for Premium and Ultimate instances.
*/
public val codeOwnerApprovalRequired: Output
get() = javaResource.codeOwnerApprovalRequired().applyValue({ args0 -> args0 })
/**
* Access levels allowed to merge. Valid values are: `no one`, `developer`, `maintainer`.
*/
public val mergeAccessLevel: Output
get() = javaResource.mergeAccessLevel().applyValue({ args0 -> args0 })
/**
* The id of the project.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* Access levels allowed to push. Valid values are: `no one`, `developer`, `maintainer`.
*/
public val pushAccessLevel: Output
get() = javaResource.pushAccessLevel().applyValue({ args0 -> args0 })
/**
* Access levels allowed to unprotect. Valid values are: `developer`, `maintainer`, `admin`.
*/
public val unprotectAccessLevel: Output
get() = javaResource.unprotectAccessLevel().applyValue({ args0 -> args0 })
}
public object BranchProtectionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.BranchProtection::class == javaResource::class
override fun map(javaResource: Resource): BranchProtection = BranchProtection(
javaResource as
com.pulumi.gitlab.BranchProtection,
)
}
/**
* @see [BranchProtection].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [BranchProtection].
*/
public suspend fun branchProtection(
name: String,
block: suspend BranchProtectionResourceBuilder.() -> Unit,
): BranchProtection {
val builder = BranchProtectionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [BranchProtection].
* @param name The _unique_ name of the resulting resource.
*/
public fun branchProtection(name: String): BranchProtection {
val builder = BranchProtectionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy