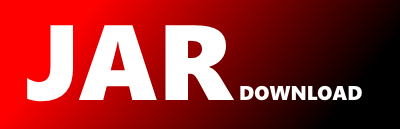
com.pulumi.gitlab.kotlin.GroupLdapLink.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [GroupLdapLink].
*/
@PulumiTagMarker
public class GroupLdapLinkResourceBuilder internal constructor() {
public var name: String? = null
public var args: GroupLdapLinkArgs = GroupLdapLinkArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend GroupLdapLinkArgsBuilder.() -> Unit) {
val builder = GroupLdapLinkArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): GroupLdapLink {
val builtJavaResource = com.pulumi.gitlab.GroupLdapLink(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return GroupLdapLink(builtJavaResource)
}
}
/**
* The `gitlab.GroupLdapLink` resource allows to manage the lifecycle of an LDAP integration with a group.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/groups.html#ldap-group-links)
* ## Example Usage
*
* ```yaml
* resources:
* test:
* type: gitlab:GroupLdapLink
* properties:
* groupId: '12345'
* cn: testuser
* groupAccess: developer
* ldapProvider: ldapmain
* ```
*
* ## Import
* GitLab group ldap links can be imported using an id made up of `group_id:ldap_provider:cn:filter`. CN and Filter are mutually exclusive, so one will be missing.
* If using the CN for the group link, the ID will end with a blank filter (":"). e.g.,
* ```sh
* $ pulumi import gitlab:index/groupLdapLink:GroupLdapLink test "12345:ldapmain:testcn:"
* ```
* If using the Filter for the group link, the ID will have two "::" in the middle due to having a blank CN. e.g.,
* ```sh
* $ pulumi import gitlab:index/groupLdapLink:GroupLdapLink test "12345:ldapmain::testfilter"
* ```
*/
public class GroupLdapLink internal constructor(
override val javaResource: com.pulumi.gitlab.GroupLdapLink,
) : KotlinCustomResource(javaResource, GroupLdapLinkMapper) {
/**
* Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
@Deprecated(
message = """
Use `group_access` instead of the `access_level` attribute.
""",
)
public val accessLevel: Output?
get() = javaResource.accessLevel().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The CN of the LDAP group to link with. Required if `filter` is not provided.
*/
public val cn: Output
get() = javaResource.cn().applyValue({ args0 -> args0 })
/**
* The LDAP filter for the group. Required if `cn` is not provided. Requires GitLab Premium or above.
*/
public val filter: Output
get() = javaResource.filter().applyValue({ args0 -> args0 })
/**
* If true, then delete and replace an existing LDAP link if one exists. Will also remove an LDAP link if the parent group is not found.
*/
public val force: Output?
get() = javaResource.force().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The ID or URL-encoded path of the group
*/
public val group: Output
get() = javaResource.group().applyValue({ args0 -> args0 })
/**
* Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
public val groupAccess: Output?
get() = javaResource.groupAccess().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the LDAP provider as stored in the GitLab database. Note that this is NOT the value of the `label` attribute as shown in the web UI. In most cases this will be `ldapmain` but you may use the [LDAP check rake task](https://docs.gitlab.com/ee/administration/raketasks/ldap.html#check) for receiving the LDAP server name: `LDAP: ... Server: ldapmain`
*/
public val ldapProvider: Output
get() = javaResource.ldapProvider().applyValue({ args0 -> args0 })
}
public object GroupLdapLinkMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.GroupLdapLink::class == javaResource::class
override fun map(javaResource: Resource): GroupLdapLink = GroupLdapLink(
javaResource as
com.pulumi.gitlab.GroupLdapLink,
)
}
/**
* @see [GroupLdapLink].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [GroupLdapLink].
*/
public suspend fun groupLdapLink(
name: String,
block: suspend GroupLdapLinkResourceBuilder.() -> Unit,
): GroupLdapLink {
val builder = GroupLdapLinkResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [GroupLdapLink].
* @param name The _unique_ name of the resulting resource.
*/
public fun groupLdapLink(name: String): GroupLdapLink {
val builder = GroupLdapLinkResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy