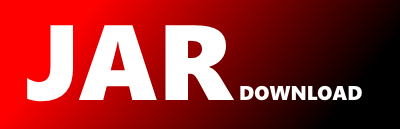
com.pulumi.gitlab.kotlin.ProjectMirrorArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.ProjectMirrorArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.ProjectMirror` resource allows to manage the lifecycle of a project mirror.
* This is for *pushing* changes to a remote repository. *Pull Mirroring* can be configured using a combination of the
* import_url, mirror, and mirror_trigger_builds properties on the gitlab.Project resource.
* > **Warning** By default, the provider sets the `keep_divergent_refs` argument to `True`.
* If you manually set `keep_divergent_refs` to `False`, GitLab mirroring removes branches in the target that aren't in the source.
* This action can result in unexpected branch deletions.
* > **Destroy Behavior** GitLab 14.10 introduced an API endpoint to delete a project mirror.
* Therefore, for GitLab 14.10 and newer the project mirror will be destroyed when the resource is destroyed.
* For older versions, the mirror will be disabled and the resource will be destroyed.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/remote_mirrors.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const foo = new gitlab.ProjectMirror("foo", {
* project: "1",
* url: "https://username:[email protected]/org/repository.git",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* foo = gitlab.ProjectMirror("foo",
* project="1",
* url="https://username:[email protected]/org/repository.git")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var foo = new GitLab.ProjectMirror("foo", new()
* {
* Project = "1",
* Url = "https://username:[email protected]/org/repository.git",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewProjectMirror(ctx, "foo", &gitlab.ProjectMirrorArgs{
* Project: pulumi.String("1"),
* Url: pulumi.String("https://username:[email protected]/org/repository.git"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.ProjectMirror;
* import com.pulumi.gitlab.ProjectMirrorArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new ProjectMirror("foo", ProjectMirrorArgs.builder()
* .project("1")
* .url("https://username:[email protected]/org/repository.git")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: gitlab:ProjectMirror
* properties:
* project: '1'
* url: https://username:[email protected]/org/repository.git
* ```
*
* ## Import
* GitLab project mirror can be imported using an id made up of `project_id:mirror_id`, e.g.
* ```sh
* $ pulumi import gitlab:index/projectMirror:ProjectMirror foo "12345:1337"
* ```
* @property enabled Determines if the mirror is enabled.
* @property keepDivergentRefs Determines if divergent refs are skipped.
* @property onlyProtectedBranches Determines if only protected branches are mirrored.
* @property project The id of the project.
* @property url The URL of the remote repository to be mirrored.
*/
public data class ProjectMirrorArgs(
public val enabled: Output? = null,
public val keepDivergentRefs: Output? = null,
public val onlyProtectedBranches: Output? = null,
public val project: Output? = null,
public val url: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.ProjectMirrorArgs =
com.pulumi.gitlab.ProjectMirrorArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.keepDivergentRefs(keepDivergentRefs?.applyValue({ args0 -> args0 }))
.onlyProtectedBranches(onlyProtectedBranches?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.url(url?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectMirrorArgs].
*/
@PulumiTagMarker
public class ProjectMirrorArgsBuilder internal constructor() {
private var enabled: Output? = null
private var keepDivergentRefs: Output? = null
private var onlyProtectedBranches: Output? = null
private var project: Output? = null
private var url: Output? = null
/**
* @param value Determines if the mirror is enabled.
*/
@JvmName("hbgffkmnnnphrshc")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Determines if divergent refs are skipped.
*/
@JvmName("efnolxcksasufcwh")
public suspend fun keepDivergentRefs(`value`: Output) {
this.keepDivergentRefs = value
}
/**
* @param value Determines if only protected branches are mirrored.
*/
@JvmName("osarenogxnlsrgpg")
public suspend fun onlyProtectedBranches(`value`: Output) {
this.onlyProtectedBranches = value
}
/**
* @param value The id of the project.
*/
@JvmName("hxxhdaigapvneeha")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The URL of the remote repository to be mirrored.
*/
@JvmName("xwehdhghcubyhtkv")
public suspend fun url(`value`: Output) {
this.url = value
}
/**
* @param value Determines if the mirror is enabled.
*/
@JvmName("hyrbmnycyadelhfa")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Determines if divergent refs are skipped.
*/
@JvmName("pxbqfdfniwqwdppa")
public suspend fun keepDivergentRefs(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keepDivergentRefs = mapped
}
/**
* @param value Determines if only protected branches are mirrored.
*/
@JvmName("fqlwlocdhjgdkcrx")
public suspend fun onlyProtectedBranches(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.onlyProtectedBranches = mapped
}
/**
* @param value The id of the project.
*/
@JvmName("sdldsodvyssuyxtv")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The URL of the remote repository to be mirrored.
*/
@JvmName("icfqhhrfwmkflimk")
public suspend fun url(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.url = mapped
}
internal fun build(): ProjectMirrorArgs = ProjectMirrorArgs(
enabled = enabled,
keepDivergentRefs = keepDivergentRefs,
onlyProtectedBranches = onlyProtectedBranches,
project = project,
url = url,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy