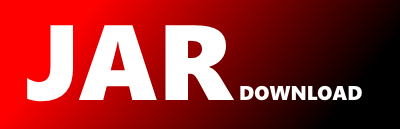
com.pulumi.gitlab.kotlin.ProjectPushRulesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.ProjectPushRulesArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const sample = new gitlab.ProjectPushRules("sample", {
* project: "42",
* authorEmailRegex: "@gitlab.com$",
* branchNameRegex: "(feat|fix)\\/*",
* commitCommitterCheck: true,
* commitCommitterNameCheck: true,
* commitMessageNegativeRegex: "ssh\\:\\/\\/",
* commitMessageRegex: "(feat|fix):.*",
* denyDeleteTag: false,
* fileNameRegex: "(jar|exe)$",
* maxFileSize: 4,
* memberCheck: true,
* preventSecrets: true,
* rejectUnsignedCommits: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* sample = gitlab.ProjectPushRules("sample",
* project="42",
* author_email_regex="@gitlab.com$",
* branch_name_regex="(feat|fix)\\/*",
* commit_committer_check=True,
* commit_committer_name_check=True,
* commit_message_negative_regex="ssh\\:\\/\\/",
* commit_message_regex="(feat|fix):.*",
* deny_delete_tag=False,
* file_name_regex="(jar|exe)$",
* max_file_size=4,
* member_check=True,
* prevent_secrets=True,
* reject_unsigned_commits=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var sample = new GitLab.ProjectPushRules("sample", new()
* {
* Project = "42",
* AuthorEmailRegex = "@gitlab.com$",
* BranchNameRegex = "(feat|fix)\\/*",
* CommitCommitterCheck = true,
* CommitCommitterNameCheck = true,
* CommitMessageNegativeRegex = "ssh\\:\\/\\/",
* CommitMessageRegex = "(feat|fix):.*",
* DenyDeleteTag = false,
* FileNameRegex = "(jar|exe)$",
* MaxFileSize = 4,
* MemberCheck = true,
* PreventSecrets = true,
* RejectUnsignedCommits = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewProjectPushRules(ctx, "sample", &gitlab.ProjectPushRulesArgs{
* Project: pulumi.String("42"),
* AuthorEmailRegex: pulumi.String("@gitlab.com$"),
* BranchNameRegex: pulumi.String("(feat|fix)\\/*"),
* CommitCommitterCheck: pulumi.Bool(true),
* CommitCommitterNameCheck: pulumi.Bool(true),
* CommitMessageNegativeRegex: pulumi.String("ssh\\:\\/\\/"),
* CommitMessageRegex: pulumi.String("(feat|fix):.*"),
* DenyDeleteTag: pulumi.Bool(false),
* FileNameRegex: pulumi.String("(jar|exe)$"),
* MaxFileSize: pulumi.Int(4),
* MemberCheck: pulumi.Bool(true),
* PreventSecrets: pulumi.Bool(true),
* RejectUnsignedCommits: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.ProjectPushRules;
* import com.pulumi.gitlab.ProjectPushRulesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sample = new ProjectPushRules("sample", ProjectPushRulesArgs.builder()
* .project(42)
* .authorEmailRegex("@gitlab.com$")
* .branchNameRegex("(feat|fix)\\/*")
* .commitCommitterCheck(true)
* .commitCommitterNameCheck(true)
* .commitMessageNegativeRegex("ssh\\:\\/\\/")
* .commitMessageRegex("(feat|fix):.*")
* .denyDeleteTag(false)
* .fileNameRegex("(jar|exe)$")
* .maxFileSize(4)
* .memberCheck(true)
* .preventSecrets(true)
* .rejectUnsignedCommits(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* sample:
* type: gitlab:ProjectPushRules
* properties:
* project: 42
* authorEmailRegex: '@gitlab.com$'
* branchNameRegex: (feat|fix)\/*
* commitCommitterCheck: true
* commitCommitterNameCheck: true
* commitMessageNegativeRegex: ssh\:\/\/
* commitMessageRegex: (feat|fix):.*
* denyDeleteTag: false
* fileNameRegex: (jar|exe)$
* maxFileSize: 4
* memberCheck: true
* preventSecrets: true
* rejectUnsignedCommits: false
* ```
*
* ## Import
* Gitlab project push rules can be imported with a key composed of ``, e.g.
* ```sh
* $ pulumi import gitlab:index/projectPushRules:ProjectPushRules sample "42"
* ```
* @property authorEmailRegex All commit author emails must match this regex, e.g. `@my-company.com$`.
* @property branchNameRegex All branch names must match this regex, e.g. `(feature|hotfix)\/*`.
* @property commitCommitterCheck Users can only push commits to this repository that were committed with one of their own verified emails.
* @property commitCommitterNameCheck Users can only push commits to this repository if the commit author name is consistent with their GitLab account name.
* @property commitMessageNegativeRegex No commit message is allowed to match this regex, e.g. `ssh\:\/\/`.
* @property commitMessageRegex All commit messages must match this regex, e.g. `Fixed \d+\..*`.
* @property denyDeleteTag Deny deleting a tag.
* @property fileNameRegex All committed filenames must not match this regex, e.g. `(jar|exe)$`.
* @property maxFileSize Maximum file size (MB).
* @property memberCheck Restrict commits by author (email) to existing GitLab users.
* @property preventSecrets GitLab will reject any files that are likely to contain secrets.
* @property project The ID or URL-encoded path of the project.
* @property rejectUnsignedCommits Reject commit when it’s not signed.
* */*/*/*/*/*/*/
*/
public data class ProjectPushRulesArgs(
public val authorEmailRegex: Output? = null,
public val branchNameRegex: Output? = null,
public val commitCommitterCheck: Output? = null,
public val commitCommitterNameCheck: Output? = null,
public val commitMessageNegativeRegex: Output? = null,
public val commitMessageRegex: Output? = null,
public val denyDeleteTag: Output? = null,
public val fileNameRegex: Output? = null,
public val maxFileSize: Output? = null,
public val memberCheck: Output? = null,
public val preventSecrets: Output? = null,
public val project: Output? = null,
public val rejectUnsignedCommits: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.ProjectPushRulesArgs =
com.pulumi.gitlab.ProjectPushRulesArgs.builder()
.authorEmailRegex(authorEmailRegex?.applyValue({ args0 -> args0 }))
.branchNameRegex(branchNameRegex?.applyValue({ args0 -> args0 }))
.commitCommitterCheck(commitCommitterCheck?.applyValue({ args0 -> args0 }))
.commitCommitterNameCheck(commitCommitterNameCheck?.applyValue({ args0 -> args0 }))
.commitMessageNegativeRegex(commitMessageNegativeRegex?.applyValue({ args0 -> args0 }))
.commitMessageRegex(commitMessageRegex?.applyValue({ args0 -> args0 }))
.denyDeleteTag(denyDeleteTag?.applyValue({ args0 -> args0 }))
.fileNameRegex(fileNameRegex?.applyValue({ args0 -> args0 }))
.maxFileSize(maxFileSize?.applyValue({ args0 -> args0 }))
.memberCheck(memberCheck?.applyValue({ args0 -> args0 }))
.preventSecrets(preventSecrets?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.rejectUnsignedCommits(rejectUnsignedCommits?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectPushRulesArgs].
*/
@PulumiTagMarker
public class ProjectPushRulesArgsBuilder internal constructor() {
private var authorEmailRegex: Output? = null
private var branchNameRegex: Output? = null
private var commitCommitterCheck: Output? = null
private var commitCommitterNameCheck: Output? = null
private var commitMessageNegativeRegex: Output? = null
private var commitMessageRegex: Output? = null
private var denyDeleteTag: Output? = null
private var fileNameRegex: Output? = null
private var maxFileSize: Output? = null
private var memberCheck: Output? = null
private var preventSecrets: Output? = null
private var project: Output? = null
private var rejectUnsignedCommits: Output? = null
/**
* @param value All commit author emails must match this regex, e.g. `@my-company.com$`.
*/
@JvmName("xxlqhkpymfxqtkvd")
public suspend fun authorEmailRegex(`value`: Output) {
this.authorEmailRegex = value
}
/**
* @param value All branch names must match this regex, e.g. `(feature|hotfix)\/*`.
* */
*/
@JvmName("fpcdgdexablvveue")
public suspend fun branchNameRegex(`value`: Output) {
this.branchNameRegex = value
}
/**
* @param value Users can only push commits to this repository that were committed with one of their own verified emails.
*/
@JvmName("xluwufhqmrsgouxy")
public suspend fun commitCommitterCheck(`value`: Output) {
this.commitCommitterCheck = value
}
/**
* @param value Users can only push commits to this repository if the commit author name is consistent with their GitLab account name.
*/
@JvmName("wiienxlshvbleeef")
public suspend fun commitCommitterNameCheck(`value`: Output) {
this.commitCommitterNameCheck = value
}
/**
* @param value No commit message is allowed to match this regex, e.g. `ssh\:\/\/`.
*/
@JvmName("hdekflxrchijyriq")
public suspend fun commitMessageNegativeRegex(`value`: Output) {
this.commitMessageNegativeRegex = value
}
/**
* @param value All commit messages must match this regex, e.g. `Fixed \d+\..*`.
*/
@JvmName("adtinfllesdouhoc")
public suspend fun commitMessageRegex(`value`: Output) {
this.commitMessageRegex = value
}
/**
* @param value Deny deleting a tag.
*/
@JvmName("mpadxoudhmynbljs")
public suspend fun denyDeleteTag(`value`: Output) {
this.denyDeleteTag = value
}
/**
* @param value All committed filenames must not match this regex, e.g. `(jar|exe)$`.
*/
@JvmName("qowyequciiqefjvr")
public suspend fun fileNameRegex(`value`: Output) {
this.fileNameRegex = value
}
/**
* @param value Maximum file size (MB).
*/
@JvmName("mvyqjvdexuigyexv")
public suspend fun maxFileSize(`value`: Output) {
this.maxFileSize = value
}
/**
* @param value Restrict commits by author (email) to existing GitLab users.
*/
@JvmName("esfbsgdfqddgibcx")
public suspend fun memberCheck(`value`: Output) {
this.memberCheck = value
}
/**
* @param value GitLab will reject any files that are likely to contain secrets.
*/
@JvmName("klnasmqeqfnnureq")
public suspend fun preventSecrets(`value`: Output) {
this.preventSecrets = value
}
/**
* @param value The ID or URL-encoded path of the project.
*/
@JvmName("rkrqkcsymrufflte")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Reject commit when it’s not signed.
*/
@JvmName("sjedikbmsaloiqmj")
public suspend fun rejectUnsignedCommits(`value`: Output) {
this.rejectUnsignedCommits = value
}
/**
* @param value All commit author emails must match this regex, e.g. `@my-company.com$`.
*/
@JvmName("uvakqflhrqjfcxlm")
public suspend fun authorEmailRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.authorEmailRegex = mapped
}
/**
* @param value All branch names must match this regex, e.g. `(feature|hotfix)\/*`.
* */
*/
@JvmName("yddfnmhlwalyyano")
public suspend fun branchNameRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.branchNameRegex = mapped
}
/**
* @param value Users can only push commits to this repository that were committed with one of their own verified emails.
*/
@JvmName("yxdmodxfgekvjvys")
public suspend fun commitCommitterCheck(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.commitCommitterCheck = mapped
}
/**
* @param value Users can only push commits to this repository if the commit author name is consistent with their GitLab account name.
*/
@JvmName("wiorhmnbmwptvptx")
public suspend fun commitCommitterNameCheck(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.commitCommitterNameCheck = mapped
}
/**
* @param value No commit message is allowed to match this regex, e.g. `ssh\:\/\/`.
*/
@JvmName("rtvlwxuyqjfmdoik")
public suspend fun commitMessageNegativeRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.commitMessageNegativeRegex = mapped
}
/**
* @param value All commit messages must match this regex, e.g. `Fixed \d+\..*`.
*/
@JvmName("iqhsexmcyhmqpkye")
public suspend fun commitMessageRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.commitMessageRegex = mapped
}
/**
* @param value Deny deleting a tag.
*/
@JvmName("byaqjmmakknlexii")
public suspend fun denyDeleteTag(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.denyDeleteTag = mapped
}
/**
* @param value All committed filenames must not match this regex, e.g. `(jar|exe)$`.
*/
@JvmName("dgfapadnouklmtpt")
public suspend fun fileNameRegex(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileNameRegex = mapped
}
/**
* @param value Maximum file size (MB).
*/
@JvmName("oawcdpgcklljklfd")
public suspend fun maxFileSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxFileSize = mapped
}
/**
* @param value Restrict commits by author (email) to existing GitLab users.
*/
@JvmName("kuwbvkapjsdacayl")
public suspend fun memberCheck(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.memberCheck = mapped
}
/**
* @param value GitLab will reject any files that are likely to contain secrets.
*/
@JvmName("dxuqchvqufwrbabi")
public suspend fun preventSecrets(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preventSecrets = mapped
}
/**
* @param value The ID or URL-encoded path of the project.
*/
@JvmName("tkpitxnigwqfknyq")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Reject commit when it’s not signed.
*/
@JvmName("oucfrpukcmbladwp")
public suspend fun rejectUnsignedCommits(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rejectUnsignedCommits = mapped
}
internal fun build(): ProjectPushRulesArgs = ProjectPushRulesArgs(
authorEmailRegex = authorEmailRegex,
branchNameRegex = branchNameRegex,
commitCommitterCheck = commitCommitterCheck,
commitCommitterNameCheck = commitCommitterNameCheck,
commitMessageNegativeRegex = commitMessageNegativeRegex,
commitMessageRegex = commitMessageRegex,
denyDeleteTag = denyDeleteTag,
fileNameRegex = fileNameRegex,
maxFileSize = maxFileSize,
memberCheck = memberCheck,
preventSecrets = preventSecrets,
project = project,
rejectUnsignedCommits = rejectUnsignedCommits,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy