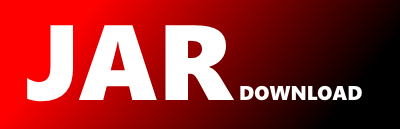
com.pulumi.gitlab.kotlin.RepositoryFile.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [RepositoryFile].
*/
@PulumiTagMarker
public class RepositoryFileResourceBuilder internal constructor() {
public var name: String? = null
public var args: RepositoryFileArgs = RepositoryFileArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RepositoryFileArgsBuilder.() -> Unit) {
val builder = RepositoryFileArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): RepositoryFile {
val builtJavaResource = com.pulumi.gitlab.RepositoryFile(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RepositoryFile(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* import * as std from "@pulumi/std";
* const _this = new gitlab.Group("this", {
* name: "example",
* path: "example",
* description: "An example group",
* });
* const thisProject = new gitlab.Project("this", {
* name: "example",
* namespaceId: _this.id,
* initializeWithReadme: true,
* });
* const thisRepositoryFile = new gitlab.RepositoryFile("this", {
* project: thisProject.id,
* filePath: "meow.txt",
* branch: "main",
* content: std.base64encode({
* input: "Meow goes the cat",
* }).then(invoke => invoke.result),
* authorEmail: "[email protected]",
* authorName: "Terraform",
* commitMessage: "feature: add meow file",
* });
* const readme = new gitlab.RepositoryFile("readme", {
* project: thisProject.id,
* filePath: "readme.txt",
* branch: "main",
* content: "Meow goes the cat",
* authorEmail: "[email protected]",
* authorName: "Terraform",
* commitMessage: "feature: add readme file",
* });
* const readmeForDogs = new gitlab.RepositoryFile("readme_for_dogs", {
* project: thisProject.id,
* filePath: "readme.txt",
* branch: "main",
* content: "Bark goes the dog",
* authorEmail: "[email protected]",
* authorName: "Terraform",
* commitMessage: "feature: update readme file",
* overwriteOnCreate: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* import pulumi_std as std
* this = gitlab.Group("this",
* name="example",
* path="example",
* description="An example group")
* this_project = gitlab.Project("this",
* name="example",
* namespace_id=this.id,
* initialize_with_readme=True)
* this_repository_file = gitlab.RepositoryFile("this",
* project=this_project.id,
* file_path="meow.txt",
* branch="main",
* content=std.base64encode(input="Meow goes the cat").result,
* author_email="[email protected]",
* author_name="Terraform",
* commit_message="feature: add meow file")
* readme = gitlab.RepositoryFile("readme",
* project=this_project.id,
* file_path="readme.txt",
* branch="main",
* content="Meow goes the cat",
* author_email="[email protected]",
* author_name="Terraform",
* commit_message="feature: add readme file")
* readme_for_dogs = gitlab.RepositoryFile("readme_for_dogs",
* project=this_project.id,
* file_path="readme.txt",
* branch="main",
* content="Bark goes the dog",
* author_email="[email protected]",
* author_name="Terraform",
* commit_message="feature: update readme file",
* overwrite_on_create=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var @this = new GitLab.Group("this", new()
* {
* Name = "example",
* Path = "example",
* Description = "An example group",
* });
* var thisProject = new GitLab.Project("this", new()
* {
* Name = "example",
* NamespaceId = @this.Id,
* InitializeWithReadme = true,
* });
* var thisRepositoryFile = new GitLab.RepositoryFile("this", new()
* {
* Project = thisProject.Id,
* FilePath = "meow.txt",
* Branch = "main",
* Content = Std.Base64encode.Invoke(new()
* {
* Input = "Meow goes the cat",
* }).Apply(invoke => invoke.Result),
* AuthorEmail = "[email protected]",
* AuthorName = "Terraform",
* CommitMessage = "feature: add meow file",
* });
* var readme = new GitLab.RepositoryFile("readme", new()
* {
* Project = thisProject.Id,
* FilePath = "readme.txt",
* Branch = "main",
* Content = "Meow goes the cat",
* AuthorEmail = "[email protected]",
* AuthorName = "Terraform",
* CommitMessage = "feature: add readme file",
* });
* var readmeForDogs = new GitLab.RepositoryFile("readme_for_dogs", new()
* {
* Project = thisProject.Id,
* FilePath = "readme.txt",
* Branch = "main",
* Content = "Bark goes the dog",
* AuthorEmail = "[email protected]",
* AuthorName = "Terraform",
* CommitMessage = "feature: update readme file",
* OverwriteOnCreate = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* this, err := gitlab.NewGroup(ctx, "this", &gitlab.GroupArgs{
* Name: pulumi.String("example"),
* Path: pulumi.String("example"),
* Description: pulumi.String("An example group"),
* })
* if err != nil {
* return err
* }
* thisProject, err := gitlab.NewProject(ctx, "this", &gitlab.ProjectArgs{
* Name: pulumi.String("example"),
* NamespaceId: this.ID(),
* InitializeWithReadme: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* invokeBase64encode, err := std.Base64encode(ctx, &std.Base64encodeArgs{
* Input: "Meow goes the cat",
* }, nil)
* if err != nil {
* return err
* }
* _, err = gitlab.NewRepositoryFile(ctx, "this", &gitlab.RepositoryFileArgs{
* Project: thisProject.ID(),
* FilePath: pulumi.String("meow.txt"),
* Branch: pulumi.String("main"),
* Content: pulumi.String(invokeBase64encode.Result),
* AuthorEmail: pulumi.String("[email protected]"),
* AuthorName: pulumi.String("Terraform"),
* CommitMessage: pulumi.String("feature: add meow file"),
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewRepositoryFile(ctx, "readme", &gitlab.RepositoryFileArgs{
* Project: thisProject.ID(),
* FilePath: pulumi.String("readme.txt"),
* Branch: pulumi.String("main"),
* Content: pulumi.String("Meow goes the cat"),
* AuthorEmail: pulumi.String("[email protected]"),
* AuthorName: pulumi.String("Terraform"),
* CommitMessage: pulumi.String("feature: add readme file"),
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewRepositoryFile(ctx, "readme_for_dogs", &gitlab.RepositoryFileArgs{
* Project: thisProject.ID(),
* FilePath: pulumi.String("readme.txt"),
* Branch: pulumi.String("main"),
* Content: pulumi.String("Bark goes the dog"),
* AuthorEmail: pulumi.String("[email protected]"),
* AuthorName: pulumi.String("Terraform"),
* CommitMessage: pulumi.String("feature: update readme file"),
* OverwriteOnCreate: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.Group;
* import com.pulumi.gitlab.GroupArgs;
* import com.pulumi.gitlab.Project;
* import com.pulumi.gitlab.ProjectArgs;
* import com.pulumi.gitlab.RepositoryFile;
* import com.pulumi.gitlab.RepositoryFileArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var this_ = new Group("this", GroupArgs.builder()
* .name("example")
* .path("example")
* .description("An example group")
* .build());
* var thisProject = new Project("thisProject", ProjectArgs.builder()
* .name("example")
* .namespaceId(this_.id())
* .initializeWithReadme(true)
* .build());
* var thisRepositoryFile = new RepositoryFile("thisRepositoryFile", RepositoryFileArgs.builder()
* .project(thisProject.id())
* .filePath("meow.txt")
* .branch("main")
* .content(StdFunctions.base64encode(Base64encodeArgs.builder()
* .input("Meow goes the cat")
* .build()).result())
* .authorEmail("[email protected]")
* .authorName("Terraform")
* .commitMessage("feature: add meow file")
* .build());
* var readme = new RepositoryFile("readme", RepositoryFileArgs.builder()
* .project(thisProject.id())
* .filePath("readme.txt")
* .branch("main")
* .content("Meow goes the cat")
* .authorEmail("[email protected]")
* .authorName("Terraform")
* .commitMessage("feature: add readme file")
* .build());
* var readmeForDogs = new RepositoryFile("readmeForDogs", RepositoryFileArgs.builder()
* .project(thisProject.id())
* .filePath("readme.txt")
* .branch("main")
* .content("Bark goes the dog")
* .authorEmail("[email protected]")
* .authorName("Terraform")
* .commitMessage("feature: update readme file")
* .overwriteOnCreate(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* this:
* type: gitlab:Group
* properties:
* name: example
* path: example
* description: An example group
* thisProject:
* type: gitlab:Project
* name: this
* properties:
* name: example
* namespaceId: ${this.id}
* initializeWithReadme: true
* thisRepositoryFile:
* type: gitlab:RepositoryFile
* name: this
* properties:
* project: ${thisProject.id}
* filePath: meow.txt
* branch: main
* content:
* fn::invoke:
* Function: std:base64encode
* Arguments:
* input: Meow goes the cat
* Return: result
* authorEmail: [email protected]
* authorName: Terraform
* commitMessage: 'feature: add meow file'
* readme:
* type: gitlab:RepositoryFile
* properties:
* project: ${thisProject.id}
* filePath: readme.txt
* branch: main
* content: Meow goes the cat
* authorEmail: [email protected]
* authorName: Terraform
* commitMessage: 'feature: add readme file'
* readmeForDogs:
* type: gitlab:RepositoryFile
* name: readme_for_dogs
* properties:
* project: ${thisProject.id}
* filePath: readme.txt
* branch: main
* content: Bark goes the dog
* authorEmail: [email protected]
* authorName: Terraform
* commitMessage: 'feature: update readme file'
* overwriteOnCreate: true
* ```
*
* ## Import
* A Repository File can be imported using an id made up of `::`, e.g.
* ```sh
* $ pulumi import gitlab:index/repositoryFile:RepositoryFile this 1:main:foo/bar.txt
* ```
*/
public class RepositoryFile internal constructor(
override val javaResource: com.pulumi.gitlab.RepositoryFile,
) : KotlinCustomResource(javaResource, RepositoryFileMapper) {
/**
* Email of the commit author.
*/
public val authorEmail: Output?
get() = javaResource.authorEmail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Name of the commit author.
*/
public val authorName: Output?
get() = javaResource.authorName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The blob id.
*/
public val blobId: Output
get() = javaResource.blobId().applyValue({ args0 -> args0 })
/**
* Name of the branch to which to commit to.
*/
public val branch: Output
get() = javaResource.branch().applyValue({ args0 -> args0 })
/**
* The commit id.
*/
public val commitId: Output
get() = javaResource.commitId().applyValue({ args0 -> args0 })
/**
* Commit message.
*/
public val commitMessage: Output?
get() = javaResource.commitMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* File content.
*/
public val content: Output
get() = javaResource.content().applyValue({ args0 -> args0 })
/**
* File content sha256 digest.
*/
public val contentSha256: Output
get() = javaResource.contentSha256().applyValue({ args0 -> args0 })
/**
* Create commit message.
*/
public val createCommitMessage: Output?
get() = javaResource.createCommitMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Delete Commit message.
*/
public val deleteCommitMessage: Output?
get() = javaResource.deleteCommitMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The file content encoding. Default value is `base64`. Valid values are: `base64`, `text`.
*/
public val encoding: Output?
get() = javaResource.encoding().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Enables or disables the execute flag on the file. **Note**: requires GitLab 14.10 or newer.
*/
public val executeFilemode: Output?
get() = javaResource.executeFilemode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The filename.
*/
public val fileName: Output
get() = javaResource.fileName().applyValue({ args0 -> args0 })
/**
* The full path of the file. It must be relative to the root of the project without a leading slash `/` or `./`.
*/
public val filePath: Output
get() = javaResource.filePath().applyValue({ args0 -> args0 })
/**
* The last known commit id.
*/
public val lastCommitId: Output
get() = javaResource.lastCommitId().applyValue({ args0 -> args0 })
/**
* Enable overwriting existing files, defaults to `false`. This attribute is only used during `create` and must be use carefully. We suggest to use `imports` whenever possible and limit the use of this attribute for when the project was imported on the same `apply`. This attribute is not supported during a resource import.
*/
public val overwriteOnCreate: Output?
get() = javaResource.overwriteOnCreate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name or ID of the project.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The name of branch, tag or commit.
*/
public val ref: Output
get() = javaResource.ref().applyValue({ args0 -> args0 })
/**
* The file size.
*/
public val size: Output
get() = javaResource.size().applyValue({ args0 -> args0 })
/**
* Name of the branch to start the new commit from.
*/
public val startBranch: Output?
get() = javaResource.startBranch().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Update commit message.
*/
public val updateCommitMessage: Output?
get() = javaResource.updateCommitMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object RepositoryFileMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.RepositoryFile::class == javaResource::class
override fun map(javaResource: Resource): RepositoryFile = RepositoryFile(
javaResource as
com.pulumi.gitlab.RepositoryFile,
)
}
/**
* @see [RepositoryFile].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [RepositoryFile].
*/
public suspend fun repositoryFile(
name: String,
block: suspend RepositoryFileResourceBuilder.() -> Unit,
): RepositoryFile {
val builder = RepositoryFileResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [RepositoryFile].
* @param name The _unique_ name of the resulting resource.
*/
public fun repositoryFile(name: String): RepositoryFile {
val builder = RepositoryFileResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy