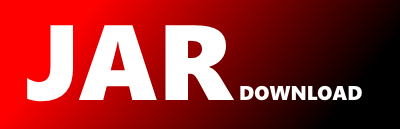
com.pulumi.gitlab.kotlin.User.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [User].
*/
@PulumiTagMarker
public class UserResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserArgs = UserArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserArgsBuilder.() -> Unit) {
val builder = UserArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): User {
val builtJavaResource = com.pulumi.gitlab.User(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return User(builtJavaResource)
}
}
/**
* The `gitlab.User` resource allows to manage the lifecycle of a user.
* > the provider needs to be configured with admin-level access for this resource to work.
* > You must specify either password or reset_password.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/users.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const example = new gitlab.User("example", {
* name: "Example Foo",
* username: "example",
* password: "superPassword",
* email: "[email protected]",
* isAdmin: true,
* projectsLimit: 4,
* canCreateGroup: false,
* isExternal: true,
* resetPassword: false,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* example = gitlab.User("example",
* name="Example Foo",
* username="example",
* password="superPassword",
* email="[email protected]",
* is_admin=True,
* projects_limit=4,
* can_create_group=False,
* is_external=True,
* reset_password=False)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var example = new GitLab.User("example", new()
* {
* Name = "Example Foo",
* Username = "example",
* Password = "superPassword",
* Email = "[email protected]",
* IsAdmin = true,
* ProjectsLimit = 4,
* CanCreateGroup = false,
* IsExternal = true,
* ResetPassword = false,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewUser(ctx, "example", &gitlab.UserArgs{
* Name: pulumi.String("Example Foo"),
* Username: pulumi.String("example"),
* Password: pulumi.String("superPassword"),
* Email: pulumi.String("[email protected]"),
* IsAdmin: pulumi.Bool(true),
* ProjectsLimit: pulumi.Int(4),
* CanCreateGroup: pulumi.Bool(false),
* IsExternal: pulumi.Bool(true),
* ResetPassword: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.User;
* import com.pulumi.gitlab.UserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new User("example", UserArgs.builder()
* .name("Example Foo")
* .username("example")
* .password("superPassword")
* .email("[email protected]")
* .isAdmin(true)
* .projectsLimit(4)
* .canCreateGroup(false)
* .isExternal(true)
* .resetPassword(false)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gitlab:User
* properties:
* name: Example Foo
* username: example
* password: superPassword
* email: [email protected]
* isAdmin: true
* projectsLimit: 4
* canCreateGroup: false
* isExternal: true
* resetPassword: false
* ```
*
* ## Import
* ```sh
* $ pulumi import gitlab:index/user:User You can import a user to terraform state using ` `.
* ```
* The `id` must be an integer for the id of the user you want to import,
* for example:
* ```sh
* $ pulumi import gitlab:index/user:User example 42
* ```
*/
public class User internal constructor(
override val javaResource: com.pulumi.gitlab.User,
) : KotlinCustomResource(javaResource, UserMapper) {
/**
* Boolean, defaults to false. Whether to allow the user to create groups.
*/
public val canCreateGroup: Output?
get() = javaResource.canCreateGroup().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The e-mail address of the user.
*/
public val email: Output
get() = javaResource.email().applyValue({ args0 -> args0 })
/**
* Boolean, defaults to false. Whether to enable administrative privileges
*/
public val isAdmin: Output?
get() = javaResource.isAdmin().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Boolean, defaults to false. Whether a user has access only to some internal or private projects. External users can only access projects to which they are explicitly granted access.
*/
public val isExternal: Output?
get() = javaResource.isExternal().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the user.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The ID of the user's namespace. Available since GitLab 14.10.
*/
public val namespaceId: Output
get() = javaResource.namespaceId().applyValue({ args0 -> args0 })
/**
* The note associated to the user.
*/
public val note: Output?
get() = javaResource.note().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The password of the user.
*/
public val password: Output?
get() = javaResource.password().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Integer, defaults to 0. Number of projects user can create.
*/
public val projectsLimit: Output?
get() = javaResource.projectsLimit().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Boolean, defaults to false. Send user password reset link.
*/
public val resetPassword: Output?
get() = javaResource.resetPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Boolean, defaults to true. Whether to skip confirmation.
*/
public val skipConfirmation: Output?
get() = javaResource.skipConfirmation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* String, defaults to 'active'. The state of the user account. Valid values are `active`, `deactivated`, `blocked`.
*/
public val state: Output?
get() = javaResource.state().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The username of the user.
*/
public val username: Output
get() = javaResource.username().applyValue({ args0 -> args0 })
}
public object UserMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.User::class == javaResource::class
override fun map(javaResource: Resource): User = User(javaResource as com.pulumi.gitlab.User)
}
/**
* @see [User].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [User].
*/
public suspend fun user(name: String, block: suspend UserResourceBuilder.() -> Unit): User {
val builder = UserResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [User].
* @param name The _unique_ name of the resulting resource.
*/
public fun user(name: String): User {
val builder = UserResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy