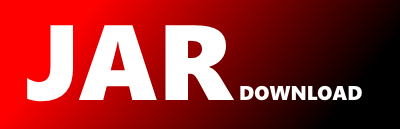
com.pulumi.gitlab.kotlin.BranchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.BranchArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.Branch` resource allows to manage the lifecycle of a repository branch.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/branches.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* // Create a project for the branch to use
* const example = new gitlab.Project("example", {
* name: "example",
* description: "An example project",
* namespaceId: exampleGitlabGroup.id,
* });
* const exampleBranch = new gitlab.Branch("example", {
* name: "example",
* ref: "main",
* project: example.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* # Create a project for the branch to use
* example = gitlab.Project("example",
* name="example",
* description="An example project",
* namespace_id=example_gitlab_group["id"])
* example_branch = gitlab.Branch("example",
* name="example",
* ref="main",
* project=example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* // Create a project for the branch to use
* var example = new GitLab.Project("example", new()
* {
* Name = "example",
* Description = "An example project",
* NamespaceId = exampleGitlabGroup.Id,
* });
* var exampleBranch = new GitLab.Branch("example", new()
* {
* Name = "example",
* Ref = "main",
* Project = example.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* // Create a project for the branch to use
* example, err := gitlab.NewProject(ctx, "example", &gitlab.ProjectArgs{
* Name: pulumi.String("example"),
* Description: pulumi.String("An example project"),
* NamespaceId: pulumi.Any(exampleGitlabGroup.Id),
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewBranch(ctx, "example", &gitlab.BranchArgs{
* Name: pulumi.String("example"),
* Ref: pulumi.String("main"),
* Project: example.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.Project;
* import com.pulumi.gitlab.ProjectArgs;
* import com.pulumi.gitlab.Branch;
* import com.pulumi.gitlab.BranchArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* // Create a project for the branch to use
* var example = new Project("example", ProjectArgs.builder()
* .name("example")
* .description("An example project")
* .namespaceId(exampleGitlabGroup.id())
* .build());
* var exampleBranch = new Branch("exampleBranch", BranchArgs.builder()
* .name("example")
* .ref("main")
* .project(example.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* # Create a project for the branch to use
* example:
* type: gitlab:Project
* properties:
* name: example
* description: An example project
* namespaceId: ${exampleGitlabGroup.id}
* exampleBranch:
* type: gitlab:Branch
* name: example
* properties:
* name: example
* ref: main
* project: ${example.id}
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_branch`. For example:
* terraform
* import {
* to = gitlab_branch.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* Gitlab branches can be imported with a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/branch:Branch example "12345:develop"
* ```
* @property name The name for this branch.
* @property project The ID or full path of the project which the branch is created against.
* @property ref The ref which the branch is created from.
*/
public data class BranchArgs(
public val name: Output? = null,
public val project: Output? = null,
public val ref: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.BranchArgs = com.pulumi.gitlab.BranchArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.ref(ref?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BranchArgs].
*/
@PulumiTagMarker
public class BranchArgsBuilder internal constructor() {
private var name: Output? = null
private var project: Output? = null
private var ref: Output? = null
/**
* @param value The name for this branch.
*/
@JvmName("rwplfqmfjjvhhrbh")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The ID or full path of the project which the branch is created against.
*/
@JvmName("boatwghfxqjslxwh")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The ref which the branch is created from.
*/
@JvmName("qlpqmxidpmvrbsfi")
public suspend fun ref(`value`: Output) {
this.ref = value
}
/**
* @param value The name for this branch.
*/
@JvmName("lqoqviraylekaius")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The ID or full path of the project which the branch is created against.
*/
@JvmName("arkmkrgltcrclqng")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The ref which the branch is created from.
*/
@JvmName("cjfgaqnqhvibkucl")
public suspend fun ref(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ref = mapped
}
internal fun build(): BranchArgs = BranchArgs(
name = name,
project = project,
ref = ref,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy