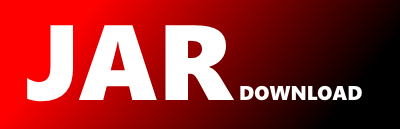
com.pulumi.gitlab.kotlin.ComplianceFramework.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [ComplianceFramework].
*/
@PulumiTagMarker
public class ComplianceFrameworkResourceBuilder internal constructor() {
public var name: String? = null
public var args: ComplianceFrameworkArgs = ComplianceFrameworkArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ComplianceFrameworkArgsBuilder.() -> Unit) {
val builder = ComplianceFrameworkArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ComplianceFramework {
val builtJavaResource = com.pulumi.gitlab.ComplianceFramework(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ComplianceFramework(builtJavaResource)
}
}
/**
* The `gitlab.ComplianceFramework` resource allows to manage the lifecycle of a compliance framework on top-level groups.
* There can be only one `default` compliance framework. Of all the configured compliance frameworks marked as default, the last one applied will be the default compliance framework.
* > This resource requires a GitLab Enterprise instance with a Premium license to create the compliance framework.
* > This resource requires a GitLab Enterprise instance with an Ultimate license to specify a compliance pipeline configuration in the compliance framework.
* **Upstream API**: [GitLab GraphQL API docs](https://docs.gitlab.com/ee/api/graphql/reference/#mutationcreatecomplianceframework)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const sample = new gitlab.ComplianceFramework("sample", {
* namespacePath: "top-level-group",
* name: "HIPAA",
* description: "A HIPAA Compliance Framework",
* color: "#87BEEF",
* "default": false,
* pipelineConfigurationFullPath: ".hipaa.yml@top-level-group/compliance-frameworks",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* sample = gitlab.ComplianceFramework("sample",
* namespace_path="top-level-group",
* name="HIPAA",
* description="A HIPAA Compliance Framework",
* color="#87BEEF",
* default=False,
* pipeline_configuration_full_path=".hipaa.yml@top-level-group/compliance-frameworks")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var sample = new GitLab.ComplianceFramework("sample", new()
* {
* NamespacePath = "top-level-group",
* Name = "HIPAA",
* Description = "A HIPAA Compliance Framework",
* Color = "#87BEEF",
* Default = false,
* PipelineConfigurationFullPath = ".hipaa.yml@top-level-group/compliance-frameworks",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewComplianceFramework(ctx, "sample", &gitlab.ComplianceFrameworkArgs{
* NamespacePath: pulumi.String("top-level-group"),
* Name: pulumi.String("HIPAA"),
* Description: pulumi.String("A HIPAA Compliance Framework"),
* Color: pulumi.String("#87BEEF"),
* Default: pulumi.Bool(false),
* PipelineConfigurationFullPath: pulumi.String(".hipaa.yml@top-level-group/compliance-frameworks"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.ComplianceFramework;
* import com.pulumi.gitlab.ComplianceFrameworkArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var sample = new ComplianceFramework("sample", ComplianceFrameworkArgs.builder()
* .namespacePath("top-level-group")
* .name("HIPAA")
* .description("A HIPAA Compliance Framework")
* .color("#87BEEF")
* .default_(false)
* .pipelineConfigurationFullPath(".hipaa.yml@top-level-group/compliance-frameworks")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* sample:
* type: gitlab:ComplianceFramework
* properties:
* namespacePath: top-level-group
* name: HIPAA
* description: A HIPAA Compliance Framework
* color: '#87BEEF'
* default: false
* pipelineConfigurationFullPath: .hipaa.yml@top-level-group/compliance-frameworks
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_compliance_framework`. For example:
* terraform
* import {
* to = gitlab_compliance_framework.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* Gitlab compliance frameworks can be imported with a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/complianceFramework:ComplianceFramework sample "top-level-group:gid://gitlab/ComplianceManagement::Framework/12345"
* ```
*/
public class ComplianceFramework internal constructor(
override val javaResource: com.pulumi.gitlab.ComplianceFramework,
) : KotlinCustomResource(javaResource, ComplianceFrameworkMapper) {
/**
* New color representation of the compliance framework in hex format. e.g. #FCA121.
*/
public val color: Output
get() = javaResource.color().applyValue({ args0 -> args0 })
/**
* Set this compliance framework as the default framework for the group. Default: `false`
*/
public val default: Output
get() = javaResource.default_().applyValue({ args0 -> args0 })
/**
* Description for the compliance framework.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* Globally unique ID of the compliance framework.
*/
public val frameworkId: Output
get() = javaResource.frameworkId().applyValue({ args0 -> args0 })
/**
* Name for the compliance framework.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Full path of the namespace to add the compliance framework to.
*/
public val namespacePath: Output
get() = javaResource.namespacePath().applyValue({ args0 -> args0 })
/**
* Full path of the compliance pipeline configuration stored in a project repository, such as `.gitlab/.compliance-gitlab-ci.yml@compliance/hipaa`. Required format: `path/file.y[a]ml@group-name/project-name` **Note**: Ultimate license required.
*/
public val pipelineConfigurationFullPath: Output?
get() = javaResource.pipelineConfigurationFullPath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object ComplianceFrameworkMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.ComplianceFramework::class == javaResource::class
override fun map(javaResource: Resource): ComplianceFramework = ComplianceFramework(
javaResource
as com.pulumi.gitlab.ComplianceFramework,
)
}
/**
* @see [ComplianceFramework].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ComplianceFramework].
*/
public suspend fun complianceFramework(
name: String,
block: suspend ComplianceFrameworkResourceBuilder.() -> Unit,
): ComplianceFramework {
val builder = ComplianceFrameworkResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ComplianceFramework].
* @param name The _unique_ name of the resulting resource.
*/
public fun complianceFramework(name: String): ComplianceFramework {
val builder = ComplianceFrameworkResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy