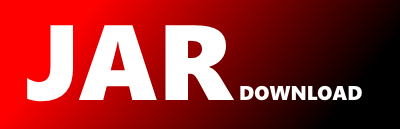
com.pulumi.gitlab.kotlin.GroupBadgeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.GroupBadgeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.GroupBadge` resource allows to manage the lifecycle of group badges.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/user/project/badges.html#group-badges)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const foo = new gitlab.Group("foo", {name: "foo-group"});
* const example = new gitlab.GroupBadge("example", {
* group: foo.id,
* linkUrl: "https://example.com/badge-123",
* imageUrl: "https://example.com/badge-123.svg",
* });
* // Pipeline status badges with placeholders will be enabled for each project
* const gitlabPipeline = new gitlab.GroupBadge("gitlab_pipeline", {
* group: foo.id,
* linkUrl: "https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}",
* imageUrl: "https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg",
* });
* // Test coverage report badges with placeholders will be enabled for each project
* const gitlabCoverage = new gitlab.GroupBadge("gitlab_coverage", {
* group: foo.id,
* linkUrl: "https://gitlab.example.com/%{project_path}/-/jobs",
* imageUrl: "https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg",
* });
* // Latest release badges with placeholders will be enabled for each project
* const gitlabRelease = new gitlab.GroupBadge("gitlab_release", {
* group: foo.id,
* linkUrl: "https://gitlab.example.com/%{project_path}/-/releases",
* imageUrl: "https://gitlab.example.com/%{project_path}/-/badges/release.svg",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* foo = gitlab.Group("foo", name="foo-group")
* example = gitlab.GroupBadge("example",
* group=foo.id,
* link_url="https://example.com/badge-123",
* image_url="https://example.com/badge-123.svg")
* # Pipeline status badges with placeholders will be enabled for each project
* gitlab_pipeline = gitlab.GroupBadge("gitlab_pipeline",
* group=foo.id,
* link_url="https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}",
* image_url="https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg")
* # Test coverage report badges with placeholders will be enabled for each project
* gitlab_coverage = gitlab.GroupBadge("gitlab_coverage",
* group=foo.id,
* link_url="https://gitlab.example.com/%{project_path}/-/jobs",
* image_url="https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg")
* # Latest release badges with placeholders will be enabled for each project
* gitlab_release = gitlab.GroupBadge("gitlab_release",
* group=foo.id,
* link_url="https://gitlab.example.com/%{project_path}/-/releases",
* image_url="https://gitlab.example.com/%{project_path}/-/badges/release.svg")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var foo = new GitLab.Group("foo", new()
* {
* Name = "foo-group",
* });
* var example = new GitLab.GroupBadge("example", new()
* {
* Group = foo.Id,
* LinkUrl = "https://example.com/badge-123",
* ImageUrl = "https://example.com/badge-123.svg",
* });
* // Pipeline status badges with placeholders will be enabled for each project
* var gitlabPipeline = new GitLab.GroupBadge("gitlab_pipeline", new()
* {
* Group = foo.Id,
* LinkUrl = "https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}",
* ImageUrl = "https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg",
* });
* // Test coverage report badges with placeholders will be enabled for each project
* var gitlabCoverage = new GitLab.GroupBadge("gitlab_coverage", new()
* {
* Group = foo.Id,
* LinkUrl = "https://gitlab.example.com/%{project_path}/-/jobs",
* ImageUrl = "https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg",
* });
* // Latest release badges with placeholders will be enabled for each project
* var gitlabRelease = new GitLab.GroupBadge("gitlab_release", new()
* {
* Group = foo.Id,
* LinkUrl = "https://gitlab.example.com/%{project_path}/-/releases",
* ImageUrl = "https://gitlab.example.com/%{project_path}/-/badges/release.svg",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* foo, err := gitlab.NewGroup(ctx, "foo", &gitlab.GroupArgs{
* Name: pulumi.String("foo-group"),
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewGroupBadge(ctx, "example", &gitlab.GroupBadgeArgs{
* Group: foo.ID(),
* LinkUrl: pulumi.String("https://example.com/badge-123"),
* ImageUrl: pulumi.String("https://example.com/badge-123.svg"),
* })
* if err != nil {
* return err
* }
* // Pipeline status badges with placeholders will be enabled for each project
* _, err = gitlab.NewGroupBadge(ctx, "gitlab_pipeline", &gitlab.GroupBadgeArgs{
* Group: foo.ID(),
* LinkUrl: pulumi.String("https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}"),
* ImageUrl: pulumi.String("https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg"),
* })
* if err != nil {
* return err
* }
* // Test coverage report badges with placeholders will be enabled for each project
* _, err = gitlab.NewGroupBadge(ctx, "gitlab_coverage", &gitlab.GroupBadgeArgs{
* Group: foo.ID(),
* LinkUrl: pulumi.String("https://gitlab.example.com/%{project_path}/-/jobs"),
* ImageUrl: pulumi.String("https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg"),
* })
* if err != nil {
* return err
* }
* // Latest release badges with placeholders will be enabled for each project
* _, err = gitlab.NewGroupBadge(ctx, "gitlab_release", &gitlab.GroupBadgeArgs{
* Group: foo.ID(),
* LinkUrl: pulumi.String("https://gitlab.example.com/%{project_path}/-/releases"),
* ImageUrl: pulumi.String("https://gitlab.example.com/%{project_path}/-/badges/release.svg"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.Group;
* import com.pulumi.gitlab.GroupArgs;
* import com.pulumi.gitlab.GroupBadge;
* import com.pulumi.gitlab.GroupBadgeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foo = new Group("foo", GroupArgs.builder()
* .name("foo-group")
* .build());
* var example = new GroupBadge("example", GroupBadgeArgs.builder()
* .group(foo.id())
* .linkUrl("https://example.com/badge-123")
* .imageUrl("https://example.com/badge-123.svg")
* .build());
* // Pipeline status badges with placeholders will be enabled for each project
* var gitlabPipeline = new GroupBadge("gitlabPipeline", GroupBadgeArgs.builder()
* .group(foo.id())
* .linkUrl("https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}")
* .imageUrl("https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg")
* .build());
* // Test coverage report badges with placeholders will be enabled for each project
* var gitlabCoverage = new GroupBadge("gitlabCoverage", GroupBadgeArgs.builder()
* .group(foo.id())
* .linkUrl("https://gitlab.example.com/%{project_path}/-/jobs")
* .imageUrl("https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg")
* .build());
* // Latest release badges with placeholders will be enabled for each project
* var gitlabRelease = new GroupBadge("gitlabRelease", GroupBadgeArgs.builder()
* .group(foo.id())
* .linkUrl("https://gitlab.example.com/%{project_path}/-/releases")
* .imageUrl("https://gitlab.example.com/%{project_path}/-/badges/release.svg")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foo:
* type: gitlab:Group
* properties:
* name: foo-group
* example:
* type: gitlab:GroupBadge
* properties:
* group: ${foo.id}
* linkUrl: https://example.com/badge-123
* imageUrl: https://example.com/badge-123.svg
* # Pipeline status badges with placeholders will be enabled for each project
* gitlabPipeline:
* type: gitlab:GroupBadge
* name: gitlab_pipeline
* properties:
* group: ${foo.id}
* linkUrl: https://gitlab.example.com/%{project_path}/-/pipelines?ref=%{default_branch}
* imageUrl: https://gitlab.example.com/%{project_path}/badges/%{default_branch}/pipeline.svg
* # Test coverage report badges with placeholders will be enabled for each project
* gitlabCoverage:
* type: gitlab:GroupBadge
* name: gitlab_coverage
* properties:
* group: ${foo.id}
* linkUrl: https://gitlab.example.com/%{project_path}/-/jobs
* imageUrl: https://gitlab.example.com/%{project_path}/badges/%{default_branch}/coverage.svg
* # Latest release badges with placeholders will be enabled for each project
* gitlabRelease:
* type: gitlab:GroupBadge
* name: gitlab_release
* properties:
* group: ${foo.id}
* linkUrl: https://gitlab.example.com/%{project_path}/-/releases
* imageUrl: https://gitlab.example.com/%{project_path}/-/badges/release.svg
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_group_badge`. For example:
* terraform
* import {
* to = gitlab_group_badge.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* GitLab group badges can be imported using an id made up of `{group_id}:{badge_id}`, e.g.
* ```sh
* $ pulumi import gitlab:index/groupBadge:GroupBadge foo 1:3
* ```
* @property group The id of the group to add the badge to.
* @property imageUrl The image url which will be presented on group overview.
* @property linkUrl The url linked with the badge.
* @property name The name of the badge.
*/
public data class GroupBadgeArgs(
public val group: Output? = null,
public val imageUrl: Output? = null,
public val linkUrl: Output? = null,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.GroupBadgeArgs =
com.pulumi.gitlab.GroupBadgeArgs.builder()
.group(group?.applyValue({ args0 -> args0 }))
.imageUrl(imageUrl?.applyValue({ args0 -> args0 }))
.linkUrl(linkUrl?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GroupBadgeArgs].
*/
@PulumiTagMarker
public class GroupBadgeArgsBuilder internal constructor() {
private var group: Output? = null
private var imageUrl: Output? = null
private var linkUrl: Output? = null
private var name: Output? = null
/**
* @param value The id of the group to add the badge to.
*/
@JvmName("jbavljttojsswavc")
public suspend fun group(`value`: Output) {
this.group = value
}
/**
* @param value The image url which will be presented on group overview.
*/
@JvmName("ivxewcrltliihdut")
public suspend fun imageUrl(`value`: Output) {
this.imageUrl = value
}
/**
* @param value The url linked with the badge.
*/
@JvmName("xlsmcjhbidruuatt")
public suspend fun linkUrl(`value`: Output) {
this.linkUrl = value
}
/**
* @param value The name of the badge.
*/
@JvmName("ftjjnsnsxmwshoyg")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The id of the group to add the badge to.
*/
@JvmName("hhnykrpkpphonybq")
public suspend fun group(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.group = mapped
}
/**
* @param value The image url which will be presented on group overview.
*/
@JvmName("mjuemnlxytctxdrd")
public suspend fun imageUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageUrl = mapped
}
/**
* @param value The url linked with the badge.
*/
@JvmName("phftpnwaogpetjmp")
public suspend fun linkUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linkUrl = mapped
}
/**
* @param value The name of the badge.
*/
@JvmName("lqusyqtwpxrmxgiw")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): GroupBadgeArgs = GroupBadgeArgs(
group = group,
imageUrl = imageUrl,
linkUrl = linkUrl,
name = name,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy