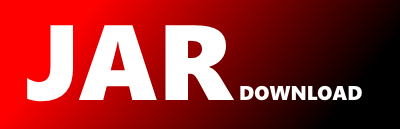
com.pulumi.gitlab.kotlin.GroupLdapLinkArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.GroupLdapLinkArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.GroupLdapLink` resource allows to manage the lifecycle of an LDAP integration with a group.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/groups.html#ldap-group-links)
* ## Example Usage
*
* ```yaml
* resources:
* test:
* type: gitlab:GroupLdapLink
* properties:
* groupId: '12345'
* cn: testuser
* groupAccess: developer
* ldapProvider: ldapmain
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_group_ldap_link`. For example:
* terraform
* import {
* to = gitlab_group_ldap_link.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* GitLab group ldap links can be imported using an id made up of `group_id:ldap_provider:cn:filter`. CN and Filter are mutually exclusive, so one will be missing.
* If using the CN for the group link, the ID will end with a blank filter (":"). e.g.,
* ```sh
* $ pulumi import gitlab:index/groupLdapLink:GroupLdapLink test "12345:ldapmain:testcn:"
* ```
* If using the Filter for the group link, the ID will have two "::" in the middle due to having a blank CN. e.g.,
* ```sh
* $ pulumi import gitlab:index/groupLdapLink:GroupLdapLink test "12345:ldapmain::testfilter"
* ```
* @property accessLevel Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
* @property cn The CN of the LDAP group to link with. Required if `filter` is not provided.
* @property filter The LDAP filter for the group. Required if `cn` is not provided. Requires GitLab Premium or above.
* @property force If true, then delete and replace an existing LDAP link if one exists. Will also remove an LDAP link if the parent group is not found.
* @property group The ID or URL-encoded path of the group
* @property groupAccess Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
* @property ldapProvider The name of the LDAP provider as stored in the GitLab database. Note that this is NOT the value of the `label` attribute as shown in the web UI. In most cases this will be `ldapmain` but you may use the [LDAP check rake task](https://docs.gitlab.com/ee/administration/raketasks/ldap.html#check) for receiving the LDAP server name: `LDAP: ... Server: ldapmain`
*/
public data class GroupLdapLinkArgs(
@Deprecated(
message = """
Use `group_access` instead of the `access_level` attribute.
""",
)
public val accessLevel: Output? = null,
public val cn: Output? = null,
public val filter: Output? = null,
public val force: Output? = null,
public val group: Output? = null,
public val groupAccess: Output? = null,
public val ldapProvider: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.GroupLdapLinkArgs =
com.pulumi.gitlab.GroupLdapLinkArgs.builder()
.accessLevel(accessLevel?.applyValue({ args0 -> args0 }))
.cn(cn?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0 }))
.force(force?.applyValue({ args0 -> args0 }))
.group(group?.applyValue({ args0 -> args0 }))
.groupAccess(groupAccess?.applyValue({ args0 -> args0 }))
.ldapProvider(ldapProvider?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GroupLdapLinkArgs].
*/
@PulumiTagMarker
public class GroupLdapLinkArgsBuilder internal constructor() {
private var accessLevel: Output? = null
private var cn: Output? = null
private var filter: Output? = null
private var force: Output? = null
private var group: Output? = null
private var groupAccess: Output? = null
private var ldapProvider: Output? = null
/**
* @param value Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
@Deprecated(
message = """
Use `group_access` instead of the `access_level` attribute.
""",
)
@JvmName("uiihlkyahojiuvib")
public suspend fun accessLevel(`value`: Output) {
this.accessLevel = value
}
/**
* @param value The CN of the LDAP group to link with. Required if `filter` is not provided.
*/
@JvmName("rcmtasufunjshplw")
public suspend fun cn(`value`: Output) {
this.cn = value
}
/**
* @param value The LDAP filter for the group. Required if `cn` is not provided. Requires GitLab Premium or above.
*/
@JvmName("sqadoarhwocprdqe")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value If true, then delete and replace an existing LDAP link if one exists. Will also remove an LDAP link if the parent group is not found.
*/
@JvmName("aclnqiiqjwvxrgmf")
public suspend fun force(`value`: Output) {
this.force = value
}
/**
* @param value The ID or URL-encoded path of the group
*/
@JvmName("jojybahfkcysschx")
public suspend fun group(`value`: Output) {
this.group = value
}
/**
* @param value Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
@JvmName("oflxyljjbmcjwort")
public suspend fun groupAccess(`value`: Output) {
this.groupAccess = value
}
/**
* @param value The name of the LDAP provider as stored in the GitLab database. Note that this is NOT the value of the `label` attribute as shown in the web UI. In most cases this will be `ldapmain` but you may use the [LDAP check rake task](https://docs.gitlab.com/ee/administration/raketasks/ldap.html#check) for receiving the LDAP server name: `LDAP: ... Server: ldapmain`
*/
@JvmName("fdygrgudjocqdpae")
public suspend fun ldapProvider(`value`: Output) {
this.ldapProvider = value
}
/**
* @param value Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
@Deprecated(
message = """
Use `group_access` instead of the `access_level` attribute.
""",
)
@JvmName("cusmhmqmlmmdnxaw")
public suspend fun accessLevel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessLevel = mapped
}
/**
* @param value The CN of the LDAP group to link with. Required if `filter` is not provided.
*/
@JvmName("kcvotatynuewpoih")
public suspend fun cn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cn = mapped
}
/**
* @param value The LDAP filter for the group. Required if `cn` is not provided. Requires GitLab Premium or above.
*/
@JvmName("qsrfhpnbavdynpbv")
public suspend fun filter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filter = mapped
}
/**
* @param value If true, then delete and replace an existing LDAP link if one exists. Will also remove an LDAP link if the parent group is not found.
*/
@JvmName("hcbquuxdcrugymei")
public suspend fun force(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.force = mapped
}
/**
* @param value The ID or URL-encoded path of the group
*/
@JvmName("xyvftpamfaxfjhge")
public suspend fun group(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.group = mapped
}
/**
* @param value Minimum access level for members of the LDAP group. Valid values are: `no one`, `minimal`, `guest`, `reporter`, `developer`, `maintainer`, `owner`
*/
@JvmName("irdswnvmkksnghra")
public suspend fun groupAccess(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupAccess = mapped
}
/**
* @param value The name of the LDAP provider as stored in the GitLab database. Note that this is NOT the value of the `label` attribute as shown in the web UI. In most cases this will be `ldapmain` but you may use the [LDAP check rake task](https://docs.gitlab.com/ee/administration/raketasks/ldap.html#check) for receiving the LDAP server name: `LDAP: ... Server: ldapmain`
*/
@JvmName("bsisxbpmnmtbtago")
public suspend fun ldapProvider(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ldapProvider = mapped
}
internal fun build(): GroupLdapLinkArgs = GroupLdapLinkArgs(
accessLevel = accessLevel,
cn = cn,
filter = filter,
force = force,
group = group,
groupAccess = groupAccess,
ldapProvider = ldapProvider,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy