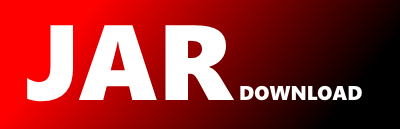
com.pulumi.gitlab.kotlin.MemberRole.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [MemberRole].
*/
@PulumiTagMarker
public class MemberRoleResourceBuilder internal constructor() {
public var name: String? = null
public var args: MemberRoleArgs = MemberRoleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend MemberRoleArgsBuilder.() -> Unit) {
val builder = MemberRoleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): MemberRole {
val builtJavaResource = com.pulumi.gitlab.MemberRole(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return MemberRole(builtJavaResource)
}
}
/**
* The `gitlab.MemberRole` resource allows to manage the lifecycle of a custom member role.
* Custom roles allow an organization to create user roles with the precise privileges and permissions required for that organization’s needs.
* > This resource requires an Ultimate license.
* > Most custom roles are considered billable users that use a seat. [Custom roles billing and seat usage](https://docs.gitlab.com/ee/user/custom_roles.html#billing-and-seat-usage)
* > There can be only 10 custom roles on your instance or namespace. See [issue 450929](https://gitlab.com/gitlab-org/gitlab/-/issues/450929) for more details.
* **Upstream API**: [GitLab GraphQL API docs](https://docs.gitlab.com/ee/api/graphql/reference/#mutationmemberrolecreate)
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_member_role`. For example:
* terraform
* import {
* to = gitlab_member_role.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* GitLab member role can be imported using the id made up of `gid://gitlab/MemberRole/` e.g.
* ```sh
* $ pulumi import gitlab:index/memberRole:MemberRole example 'gid://gitlab/MemberRole/123'
* ```
*/
public class MemberRole internal constructor(
override val javaResource: com.pulumi.gitlab.MemberRole,
) : KotlinCustomResource(javaResource, MemberRoleMapper) {
/**
* The base access level for the custom role. Valid values are: `DEVELOPER`, `GUEST`, `MAINTAINER`, `MINIMAL_ACCESS`, `OWNER`, `REPORTER`
*/
public val baseAccessLevel: Output
get() = javaResource.baseAccessLevel().applyValue({ args0 -> args0 })
/**
* Timestamp of when the member role was created. Only available with GitLab version 17.3 or higher.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* Description for the member role.
*/
public val description: Output
get() = javaResource.description().applyValue({ args0 -> args0 })
/**
* The Web UI path to edit the member role
*/
public val editPath: Output
get() = javaResource.editPath().applyValue({ args0 -> args0 })
/**
* All permissions enabled for the custom role. Valid values are: `ADMIN_CICD_VARIABLES`, `ADMIN_COMPLIANCE_FRAMEWORK`, `ADMIN_GROUP_MEMBER`, `ADMIN_INTEGRATIONS`, `ADMIN_MERGE_REQUEST`, `ADMIN_PUSH_RULES`, `ADMIN_RUNNERS`, `ADMIN_TERRAFORM_STATE`, `ADMIN_VULNERABILITY`, `ADMIN_WEB_HOOK`, `ARCHIVE_PROJECT`, `MANAGE_DEPLOY_TOKENS`, `MANAGE_GROUP_ACCESS_TOKENS`, `MANAGE_MERGE_REQUEST_SETTINGS`, `MANAGE_PROJECT_ACCESS_TOKENS`, `MANAGE_SECURITY_POLICY_LINK`, `READ_CODE`, `READ_CRM_CONTACT`, `READ_DEPENDENCY`, `READ_RUNNERS`, `READ_VULNERABILITY`, `REMOVE_GROUP`, `REMOVE_PROJECT`
*/
public val enabledPermissions: Output>
get() = javaResource.enabledPermissions().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Full path of the namespace to create the member role in. **Required for SAAS** **Not allowed for self-managed**
*/
public val groupPath: Output
get() = javaResource.groupPath().applyValue({ args0 -> args0 })
/**
* The id integer value extracted from the `id` attribute
*/
public val iid: Output
get() = javaResource.iid().applyValue({ args0 -> args0 })
/**
* Name for the member role.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
}
public object MemberRoleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.MemberRole::class == javaResource::class
override fun map(javaResource: Resource): MemberRole = MemberRole(
javaResource as
com.pulumi.gitlab.MemberRole,
)
}
/**
* @see [MemberRole].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [MemberRole].
*/
public suspend fun memberRole(name: String, block: suspend MemberRoleResourceBuilder.() -> Unit): MemberRole {
val builder = MemberRoleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [MemberRole].
* @param name The _unique_ name of the resulting resource.
*/
public fun memberRole(name: String): MemberRole {
val builder = MemberRoleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy