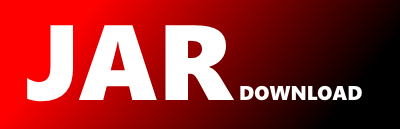
com.pulumi.gitlab.kotlin.PersonalAccessToken.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.gitlab.kotlin.outputs.PersonalAccessTokenRotationConfiguration
import com.pulumi.gitlab.kotlin.outputs.PersonalAccessTokenRotationConfiguration.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [PersonalAccessToken].
*/
@PulumiTagMarker
public class PersonalAccessTokenResourceBuilder internal constructor() {
public var name: String? = null
public var args: PersonalAccessTokenArgs = PersonalAccessTokenArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend PersonalAccessTokenArgsBuilder.() -> Unit) {
val builder = PersonalAccessTokenArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): PersonalAccessToken {
val builtJavaResource = com.pulumi.gitlab.PersonalAccessToken(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return PersonalAccessToken(builtJavaResource)
}
}
/**
* The `gitlab.PersonalAccessToken` resource allows to manage the lifecycle of a personal access token.
* > This resource requires administration privileges.
* > Use of the `timestamp()` function with expires_at will cause the resource to be re-created with every apply, it's recommended to use `plantimestamp()` or a static value instead.
* > Observability scopes are in beta and may not work on all instances. See more details in [the documentation](https://docs.gitlab.com/ee/operations/tracing.html)
* > Use `rotation_configuration` to automatically rotate tokens instead of using `timestamp()` as timestamp will cause changes with every plan. `pulumi up` must still be run to rotate the token.
* > Due to [Automatic reuse detection](https://docs.gitlab.com/ee/api/personal_access_tokens.html#automatic-reuse-detection) it's possible that a new Personal Access Token will immediately be revoked. Check if an old process using the old token is running if this happens.
* **Upstream API**: [GitLab API docs](https://docs.gitlab.com/ee/api/personal_access_tokens.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const example = new gitlab.PersonalAccessToken("example", {
* userId: 25,
* name: "Example personal access token",
* expiresAt: "2020-03-14",
* scopes: ["api"],
* });
* const exampleProjectVariable = new gitlab.ProjectVariable("example", {
* project: exampleGitlabProject.id,
* key: "pat",
* value: example.token,
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* example = gitlab.PersonalAccessToken("example",
* user_id=25,
* name="Example personal access token",
* expires_at="2020-03-14",
* scopes=["api"])
* example_project_variable = gitlab.ProjectVariable("example",
* project=example_gitlab_project["id"],
* key="pat",
* value=example.token)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var example = new GitLab.PersonalAccessToken("example", new()
* {
* UserId = 25,
* Name = "Example personal access token",
* ExpiresAt = "2020-03-14",
* Scopes = new[]
* {
* "api",
* },
* });
* var exampleProjectVariable = new GitLab.ProjectVariable("example", new()
* {
* Project = exampleGitlabProject.Id,
* Key = "pat",
* Value = example.Token,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := gitlab.NewPersonalAccessToken(ctx, "example", &gitlab.PersonalAccessTokenArgs{
* UserId: pulumi.Int(25),
* Name: pulumi.String("Example personal access token"),
* ExpiresAt: pulumi.String("2020-03-14"),
* Scopes: pulumi.StringArray{
* pulumi.String("api"),
* },
* })
* if err != nil {
* return err
* }
* _, err = gitlab.NewProjectVariable(ctx, "example", &gitlab.ProjectVariableArgs{
* Project: pulumi.Any(exampleGitlabProject.Id),
* Key: pulumi.String("pat"),
* Value: example.Token,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.PersonalAccessToken;
* import com.pulumi.gitlab.PersonalAccessTokenArgs;
* import com.pulumi.gitlab.ProjectVariable;
* import com.pulumi.gitlab.ProjectVariableArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new PersonalAccessToken("example", PersonalAccessTokenArgs.builder()
* .userId("25")
* .name("Example personal access token")
* .expiresAt("2020-03-14")
* .scopes("api")
* .build());
* var exampleProjectVariable = new ProjectVariable("exampleProjectVariable", ProjectVariableArgs.builder()
* .project(exampleGitlabProject.id())
* .key("pat")
* .value(example.token())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gitlab:PersonalAccessToken
* properties:
* userId: '25'
* name: Example personal access token
* expiresAt: 2020-03-14
* scopes:
* - api
* exampleProjectVariable:
* type: gitlab:ProjectVariable
* name: example
* properties:
* project: ${exampleGitlabProject.id}
* key: pat
* value: ${example.token}
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_personal_access_token`. For example:
* terraform
* import {
* to = gitlab_personal_access_token.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* A GitLab Personal Access Token can be imported using a key composed of `:`, e.g.
* ```sh
* $ pulumi import gitlab:index/personalAccessToken:PersonalAccessToken example "12345:1"
* ```
* NOTE: the `token` resource attribute is not available for imported resources as this information cannot be read from the GitLab API.
*/
public class PersonalAccessToken internal constructor(
override val javaResource: com.pulumi.gitlab.PersonalAccessToken,
) : KotlinCustomResource(javaResource, PersonalAccessTokenMapper) {
/**
* True if the token is active.
*/
public val active: Output
get() = javaResource.active().applyValue({ args0 -> args0 })
/**
* Time the token has been created, RFC3339 format.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* When the token will expire, YYYY-MM-DD format. Is automatically set when `rotation_configuration` is used.
*/
public val expiresAt: Output
get() = javaResource.expiresAt().applyValue({ args0 -> args0 })
/**
* The name of the personal access token.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* True if the token is revoked.
*/
public val revoked: Output
get() = javaResource.revoked().applyValue({ args0 -> args0 })
/**
* The configuration for when to rotate a token automatically. Will not rotate a token until `pulumi up` is run.
*/
public val rotationConfiguration: Output?
get() = javaResource.rotationConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
}).orElse(null)
})
/**
* The scopes of the personal access token. valid values are: `api`, `read_user`, `read_api`, `read_repository`, `write_repository`, `read_registry`, `write_registry`, `sudo`, `admin_mode`, `create_runner`, `manage_runner`, `ai_features`, `k8s_proxy`, `read_service_ping`
*/
public val scopes: Output>
get() = javaResource.scopes().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The token of the personal access token. **Note**: the token is not available for imported resources.
*/
public val token: Output
get() = javaResource.token().applyValue({ args0 -> args0 })
/**
* The ID of the user.
*/
public val userId: Output
get() = javaResource.userId().applyValue({ args0 -> args0 })
}
public object PersonalAccessTokenMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gitlab.PersonalAccessToken::class == javaResource::class
override fun map(javaResource: Resource): PersonalAccessToken = PersonalAccessToken(
javaResource
as com.pulumi.gitlab.PersonalAccessToken,
)
}
/**
* @see [PersonalAccessToken].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [PersonalAccessToken].
*/
public suspend fun personalAccessToken(
name: String,
block: suspend PersonalAccessTokenResourceBuilder.() -> Unit,
): PersonalAccessToken {
val builder = PersonalAccessTokenResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [PersonalAccessToken].
* @param name The _unique_ name of the resulting resource.
*/
public fun personalAccessToken(name: String): PersonalAccessToken {
val builder = PersonalAccessTokenResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy