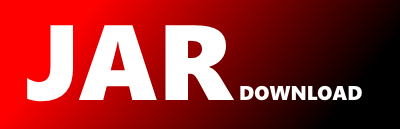
com.pulumi.gitlab.kotlin.PipelineScheduleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gitlab-kotlin Show documentation
Show all versions of pulumi-gitlab-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gitlab.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gitlab.PipelineScheduleArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The `gitlab.PipelineSchedule` resource allows to manage the lifecycle of a scheduled pipeline.
* **Upstream API**: [GitLab REST API docs](https://docs.gitlab.com/ee/api/pipeline_schedules.html)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gitlab from "@pulumi/gitlab";
* const example = new gitlab.PipelineSchedule("example", {
* project: "12345",
* description: "Used to schedule builds",
* ref: "refs/heads/main",
* cron: "0 1 * * *",
* });
* ```
* ```python
* import pulumi
* import pulumi_gitlab as gitlab
* example = gitlab.PipelineSchedule("example",
* project="12345",
* description="Used to schedule builds",
* ref="refs/heads/main",
* cron="0 1 * * *")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using GitLab = Pulumi.GitLab;
* return await Deployment.RunAsync(() =>
* {
* var example = new GitLab.PipelineSchedule("example", new()
* {
* Project = "12345",
* Description = "Used to schedule builds",
* Ref = "refs/heads/main",
* Cron = "0 1 * * *",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gitlab/sdk/v8/go/gitlab"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := gitlab.NewPipelineSchedule(ctx, "example", &gitlab.PipelineScheduleArgs{
* Project: pulumi.String("12345"),
* Description: pulumi.String("Used to schedule builds"),
* Ref: pulumi.String("refs/heads/main"),
* Cron: pulumi.String("0 1 * * *"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gitlab.PipelineSchedule;
* import com.pulumi.gitlab.PipelineScheduleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new PipelineSchedule("example", PipelineScheduleArgs.builder()
* .project("12345")
* .description("Used to schedule builds")
* .ref("refs/heads/main")
* .cron("0 1 * * *")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gitlab:PipelineSchedule
* properties:
* project: '12345'
* description: Used to schedule builds
* ref: refs/heads/main
* cron: 0 1 * * *
* ```
*
* ## Import
* Starting in Terraform v1.5.0 you can use an import block to import `gitlab_pipeline_schedule`. For example:
* terraform
* import {
* to = gitlab_pipeline_schedule.example
* id = "see CLI command below for ID"
* }
* Import using the CLI is supported using the following syntax:
* GitLab pipeline schedules can be imported using an id made up of `{project_id}:{pipeline_schedule_id}`, e.g.
* ```sh
* $ pulumi import gitlab:index/pipelineSchedule:PipelineSchedule test 1:3
* ```
* @property active The activation of pipeline schedule. If false is set, the pipeline schedule will deactivated initially.
* @property cron The cron (e.g. `0 1 * * *`).
* @property cronTimezone The timezone.
* @property description The description of the pipeline schedule.
* @property project The name or id of the project to add the schedule to.
* @property ref The branch/tag name to be triggered. This must be the full branch reference, for example: `refs/heads/main`, not `main`.
* @property takeOwnership
*/
public data class PipelineScheduleArgs(
public val active: Output? = null,
public val cron: Output? = null,
public val cronTimezone: Output? = null,
public val description: Output? = null,
public val project: Output? = null,
public val ref: Output? = null,
public val takeOwnership: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gitlab.PipelineScheduleArgs =
com.pulumi.gitlab.PipelineScheduleArgs.builder()
.active(active?.applyValue({ args0 -> args0 }))
.cron(cron?.applyValue({ args0 -> args0 }))
.cronTimezone(cronTimezone?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.ref(ref?.applyValue({ args0 -> args0 }))
.takeOwnership(takeOwnership?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PipelineScheduleArgs].
*/
@PulumiTagMarker
public class PipelineScheduleArgsBuilder internal constructor() {
private var active: Output? = null
private var cron: Output? = null
private var cronTimezone: Output? = null
private var description: Output? = null
private var project: Output? = null
private var ref: Output? = null
private var takeOwnership: Output? = null
/**
* @param value The activation of pipeline schedule. If false is set, the pipeline schedule will deactivated initially.
*/
@JvmName("awknaskdqhkddvex")
public suspend fun active(`value`: Output) {
this.active = value
}
/**
* @param value The cron (e.g. `0 1 * * *`).
*/
@JvmName("ckgpwuwuattpxggt")
public suspend fun cron(`value`: Output) {
this.cron = value
}
/**
* @param value The timezone.
*/
@JvmName("jdshvpivhprrbyov")
public suspend fun cronTimezone(`value`: Output) {
this.cronTimezone = value
}
/**
* @param value The description of the pipeline schedule.
*/
@JvmName("prvpcleyktbavtln")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name or id of the project to add the schedule to.
*/
@JvmName("mebwjiniejtwyjxu")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The branch/tag name to be triggered. This must be the full branch reference, for example: `refs/heads/main`, not `main`.
*/
@JvmName("nndskdqcaidjqlgs")
public suspend fun ref(`value`: Output) {
this.ref = value
}
/**
* @param value
*/
@JvmName("vjswxaghwdhfcdgw")
public suspend fun takeOwnership(`value`: Output) {
this.takeOwnership = value
}
/**
* @param value The activation of pipeline schedule. If false is set, the pipeline schedule will deactivated initially.
*/
@JvmName("nmabixvkiixgkwfv")
public suspend fun active(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.active = mapped
}
/**
* @param value The cron (e.g. `0 1 * * *`).
*/
@JvmName("qwvrsmgwauvlbome")
public suspend fun cron(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cron = mapped
}
/**
* @param value The timezone.
*/
@JvmName("fjhxfknrpmbyvpvs")
public suspend fun cronTimezone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cronTimezone = mapped
}
/**
* @param value The description of the pipeline schedule.
*/
@JvmName("pobewvylwnlyofur")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The name or id of the project to add the schedule to.
*/
@JvmName("vceercqgovqkosas")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The branch/tag name to be triggered. This must be the full branch reference, for example: `refs/heads/main`, not `main`.
*/
@JvmName("lymeepgxbyakamth")
public suspend fun ref(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ref = mapped
}
/**
* @param value
*/
@JvmName("qtagecsrqwvrnydy")
public suspend fun takeOwnership(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.takeOwnership = mapped
}
internal fun build(): PipelineScheduleArgs = PipelineScheduleArgs(
active = active,
cron = cron,
cronTimezone = cronTimezone,
description = description,
project = project,
ref = ref,
takeOwnership = takeOwnership,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy